A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
jack.c
00001 #include <stdbool.h> 00002 #include "gpio.h" 00003 #include "net-jack-leds.h" 00004 #include "mstimer.h" 00005 00006 #define BLINK_DURATION_MS 50 00007 00008 void JackLeds(bool phyLink, bool phySpeed, bool activity) 00009 { 00010 static int blinkTimer = 0; 00011 00012 if (activity) blinkTimer = MsTimerCount; 00013 if (MsTimerRelative(blinkTimer, BLINK_DURATION_MS)) 00014 { 00015 if (phyLink) LED_GR_L_SET; else LED_GR_L_CLR; 00016 if (phySpeed) LED_YE_R_SET; else LED_YE_R_CLR; 00017 } 00018 else 00019 { 00020 LED_GR_L_CLR; 00021 LED_YE_R_CLR; 00022 } 00023 } 00024 00025 void JackInit() 00026 { 00027 LED_GR_L_DIR = 1; //Set the direction to 1 == output 00028 LED_YE_R_DIR = 1; //Set the direction to 1 == output 00029 }
Generated on Tue Jul 12 2022 18:53:40 by
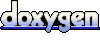