A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
ip.c
00001 #include <stdint.h> 00002 #include <string.h> 00003 #include <stdio.h> 00004 00005 #include "log.h" 00006 #include "ip.h" 00007 00008 void IpProtocolString(uint8_t protocol, int size, char* text) 00009 { 00010 switch (protocol) 00011 { 00012 case ICMP: strncpy(text, "ICMP" , size); break; 00013 case IGMP: strncpy(text, "IGMP" , size); break; 00014 case ICMP6: strncpy(text, "ICMP6" , size); break; 00015 case TCP: strncpy(text, "TCP" , size); break; 00016 case UDP: strncpy(text, "UDP" , size); break; 00017 case IP6IN4: strncpy(text, "IP6IN4", size); break; 00018 default: snprintf(text, size, "%d", protocol); break; 00019 } 00020 } 00021 void IpProtocolLog(uint8_t protocol) 00022 { 00023 switch (protocol) 00024 { 00025 case ICMP: Log("ICMP" ); break; 00026 case IGMP: Log("IGMP" ); break; 00027 case ICMP6: Log("ICMP6" ); break; 00028 case TCP: Log("TCP" ); break; 00029 case UDP: Log("UDP" ); break; 00030 case IP6IN4: Log("IP6IN4"); break; 00031 default: LogF("%d", protocol); break; 00032 } 00033 }
Generated on Tue Jul 12 2022 18:53:40 by
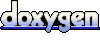