A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
ip4addr.c
00001 #include <stdint.h> 00002 #include <stdio.h> 00003 00004 #include "log.h" 00005 #include "http.h" 00006 #include "action.h" 00007 #include "ip4addr.h" 00008 #include "dhcp.h" 00009 #include "ntpclient.h" 00010 #include "tftp.h" 00011 #include "user.h" 00012 00013 int Ip4AddrToString(const uint32_t ip, const int size, char* text) 00014 { 00015 int a0 = (ip & 0xFF000000) >> 24; 00016 int a1 = (ip & 0x00FF0000) >> 16; 00017 int a2 = (ip & 0x0000FF00) >> 8; 00018 int a3 = (ip & 0x000000FF); 00019 return snprintf(text, size, "%d.%d.%d.%d", a3, a2, a1, a0); 00020 } 00021 int Ip4AddrLog(const uint32_t ip) 00022 { 00023 int a0 = (ip & 0xFF000000) >> 24; 00024 int a1 = (ip & 0x00FF0000) >> 16; 00025 int a2 = (ip & 0x0000FF00) >> 8; 00026 int a3 = (ip & 0x000000FF); 00027 return LogF("%d.%d.%d.%d", a3, a2, a1, a0); 00028 } 00029 int Ip4AddrHttp(const uint32_t ip) 00030 { 00031 int a0 = (ip & 0xFF000000) >> 24; 00032 int a1 = (ip & 0x00FF0000) >> 16; 00033 int a2 = (ip & 0x0000FF00) >> 8; 00034 int a3 = (ip & 0x000000FF); 00035 return HttpAddF("%d.%d.%d.%d", a3, a2, a1, a0); 00036 } 00037 00038 uint32_t Ip4AddrParse(const char* pText) //Returns 0 on error 00039 { 00040 const char* p = pText; 00041 int ints[4]; 00042 int field = 0; 00043 int word = 0; 00044 while(true) 00045 { 00046 switch (*p) 00047 { 00048 case '.': 00049 ints[field] = word; 00050 field++; 00051 if (field > 3) return 0; 00052 word = 0; 00053 break; 00054 case '0': word *= 10; word += 0; break; 00055 case '1': word *= 10; word += 1; break; 00056 case '2': word *= 10; word += 2; break; 00057 case '3': word *= 10; word += 3; break; 00058 case '4': word *= 10; word += 4; break; 00059 case '5': word *= 10; word += 5; break; 00060 case '6': word *= 10; word += 6; break; 00061 case '7': word *= 10; word += 7; break; 00062 case '8': word *= 10; word += 8; break; 00063 case '9': word *= 10; word += 9; break; 00064 case 0: 00065 ints[field] = word; 00066 uint32_t addr4 = (ints[0] << 0) + (ints[1] << 8) + (ints[2] << 16) + (ints[3] << 24); 00067 return addr4; 00068 default: return 0; 00069 } 00070 p++; 00071 } 00072 } 00073 void Ip4AddrFromDest(const int dest, uint32_t* pDstIp) 00074 { 00075 switch (dest) 00076 { 00077 case UNICAST: /*No change*/ break; 00078 case UNICAST_DNS: *pDstIp = DhcpDnsServerIp; break; 00079 case UNICAST_DHCP: *pDstIp = DhcpServerIp; break; 00080 case UNICAST_NTP: *pDstIp = NtpClientQueryServerIp4; break; 00081 case UNICAST_TFTP: *pDstIp = TftpServerIp4; break; 00082 case UNICAST_USER: *pDstIp = UserIp4; break; 00083 case MULTICAST_NODE: *pDstIp = IP4_MULTICAST_ALL_HOSTS; break; 00084 case MULTICAST_ROUTER: *pDstIp = IP4_MULTICAST_ALL_ROUTERS; break; 00085 case MULTICAST_MDNS: *pDstIp = IP4_MULTICAST_DNS_ADDRESS; break; 00086 case MULTICAST_LLMNR: *pDstIp = IP4_MULTICAST_LLMNR_ADDRESS; break; 00087 case MULTICAST_NTP: *pDstIp = IP4_MULTICAST_NTP_ADDRESS; break; 00088 case BROADCAST: *pDstIp = IP4_BROADCAST_ADDRESS; break; 00089 default: 00090 LogTimeF("Ip4AddrFromDest unknown destination %d\r\n", dest); 00091 break; 00092 } 00093 } 00094 bool Ip4AddrIsExternal(uint32_t ip) 00095 //Logic is if it isn't local and it isn't one of the three types of broadcast then it must be external. 00096 { 00097 if ((ip & DhcpSubnetMask) == (DhcpLocalIp & DhcpSubnetMask)) return false; // Ip is same as local ip in the unmasked area 00098 if ( ip == (DhcpLocalIp | 0xFF000000) ) return false; // Ip == 192.168.0.255; '|' is lower precendence than '==' 00099 if ( ip == IP4_BROADCAST_ADDRESS ) return false; // dstIp == 255.255.255.255 00100 if ((ip & 0xE0) == 0xE0 ) return false; // 224.x.x.x == 1110 0000 == E0.xx.xx.xx == xx.xx.xx.E0 in little endian 00101 00102 return true; 00103 }
Generated on Tue Jul 12 2022 18:53:40 by
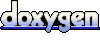