A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
dnslabel.c
00001 #include <stdbool.h> 00002 #include <string.h> 00003 00004 #include "dns.h" 00005 #include "dnslabel.h" 00006 #include "dhcp.h" 00007 00008 bool DnsLabelIsExternal(char* p) 00009 { 00010 while (true) 00011 { 00012 if (*p == '\0') return false; 00013 if (*p == '.') return true; 00014 p++; 00015 } 00016 } 00017 00018 void DnsLabelCopy(char* dst, char* src) 00019 { 00020 strncpy(dst, src, DNS_MAX_LABEL_LENGTH); //Copies up to 63 bytes 00021 dst[DNS_MAX_LABEL_LENGTH] = 0; //dst[63] is the 64th position from 0..63 is set to null. All labels must be declared as a size of 64 00022 } 00023 00024 bool DnsLabelIsSame(char* pA, char* pB) 00025 { 00026 while(true) 00027 { 00028 char a = *pA++; 00029 char b = *pB++; 00030 if (a >= 'A' && a <= 'Z') a |= 0x20; //Make lower case 00031 if (b >= 'A' && b <= 'Z') b |= 0x20; //Make lower case 00032 if (a != b) return false; //If different then stop and return the fact 00033 if (!a) break; //No need to check 'b' too as it will necessarily be equal to 'a' at this point. 00034 } 00035 return true; //If we get here the strings must equate. 00036 } 00037 00038 int DnsLabelMakeFullNameFromName(int protocol, const char* p, int size, char* result) 00039 { 00040 int i = 0; 00041 char c; 00042 bool isExternal = false; 00043 00044 while (i < size - 1) 00045 { 00046 c = *p++; 00047 if (c == '.') isExternal = true; 00048 if (!c) break; 00049 *result++ = c; 00050 i++; 00051 } 00052 if (protocol == DNS_PROTOCOL_MDNS) 00053 { 00054 p = ".local"; 00055 while (i < size - 1) 00056 { 00057 c = *p++; 00058 if (!c) break; 00059 *result++ = c; 00060 i++; 00061 } 00062 } 00063 if (protocol == DNS_PROTOCOL_UDNS && !isExternal && DhcpDomainName[0]) //Shouldn't do this in IPv6 as DHCP is IPv4 only 00064 { 00065 if (i < size - 1) 00066 { 00067 *result++ = '.'; 00068 i++; 00069 } 00070 p = DhcpDomainName; 00071 while (i < size - 1) 00072 { 00073 c = *p++; 00074 if (!c) break; 00075 *result++ = c; 00076 i++; 00077 } 00078 } 00079 *result = 0; //Terminate the resulting string 00080 return i; 00081 } 00082 int DnsLabelStripNameFromFullName(int protocol, char* p, int size, char* result) 00083 { 00084 int i = 0; 00085 char c; 00086 00087 while (i < size - 1) 00088 { 00089 c = *p++; 00090 if (c == 0) break; //End of the fqdn so stop 00091 if (c == '.') 00092 { 00093 if (protocol == DNS_PROTOCOL_UDNS) 00094 { 00095 if (strcmp(p, DhcpDomainName) == 0) break; //Strip the domain from a UDNS fqdn if, and only if, it matches the domain given in DHCP. IPv4 only. 00096 } 00097 else 00098 { 00099 break; //Strip the domain from an LLMNR (there shouldn't be one) or MDNS (it should always be '.local') fqdn 00100 } 00101 } 00102 *result++ = c; 00103 i++; 00104 } 00105 *result = 0; //Terminate the copied string 00106 return i; 00107 }
Generated on Tue Jul 12 2022 18:53:40 by
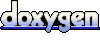