A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
dest6.c
00001 #include <stdint.h> 00002 #include <stdbool.h> 00003 00004 #include "log.h" 00005 #include "net.h" 00006 #include "action.h" 00007 00008 bool Dest6Trace = false; 00009 00010 static void logCode(uint8_t code) 00011 { 00012 switch (code) 00013 { 00014 case 0: Log ("No route to destination" ); break; 00015 case 1: Log ("Communication with destination administratively prohibited"); break; 00016 case 2: Log ("Beyond scope of source address" ); break; 00017 case 3: Log ("Address unreachable" ); break; 00018 case 4: Log ("Port unreachable" ); break; 00019 case 5: Log ("Source address failed ingress/egress policy" ); break; 00020 case 6: Log ("Reject route to destination" ); break; 00021 default: LogF("Unknown code %u", code ); break; 00022 } 00023 } 00024 int Dest6HandleRequest(void (*traceback)(void), uint8_t* pType, uint8_t* pCode) 00025 { 00026 if (Dest6Trace) 00027 { 00028 if (NetTraceNewLine) Log("\r\n"); 00029 LogTime("Dest6 - Destination unreacheable - "); logCode(*pCode); Log("\r\n"); 00030 if (NetTraceStack) traceback(); 00031 } 00032 return DO_NOTHING; 00033 }
Generated on Tue Jul 12 2022 18:53:40 by
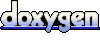