A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
checksum.c
00001 #include <stdint.h> 00002 00003 /* 00004 Checksum is calculated on 2 byte chunks 00005 All the routines below rely on the start being at a 2 byte boundary 00006 It makes no sence for any, other than the last, call to accumulate the checksum to have an odd number of bytes 00007 00008 Start the first call with a sum of zero. 00009 Thereafter propagate it to each subsequent call. 00010 Always call the Fin routine for the last call to roll back in any carries, to convert to 16 bit and to take the ones complement. 00011 The Fin call can have an odd number of byes but cannot invert. 00012 00013 The simple CheckSum is for when there is just one lump of data to calculate the checksum over. 00014 00015 If the lump of data starts at an odd address the 2 byte cast will result in an unaligned access. 00016 This is ok for the LPC1768 M3, it just takes it a bit longer, but could cause other processors to fault. 00017 It should never happen as no protocol is not at least aligned on even bytes and the buffers all start aligned. 00018 */ 00019 00020 uint32_t CheckSumAddDirect(uint32_t sum, int count, void* pData) 00021 { 00022 uint16_t* p = (uint16_t*)pData; //Set up a 16 bit pointer for the data 00023 00024 while(count > 1) 00025 { 00026 sum += *p++; // Add each pair of bytes into 32 bit accumulator 00027 count -= 2; 00028 } 00029 if (count) sum += *(uint8_t*) p; // Add left-over byte, if any 00030 return sum; 00031 } 00032 uint32_t CheckSumAddInvert(uint32_t sum, int count, void* pData) 00033 { 00034 uint16_t* p = (uint16_t*)pData; //Set up a 16 bit pointer for the data 00035 00036 while(count > 1) 00037 { 00038 uint16_t value = (*p & 0xFF00) >> 8; // Invert the value 00039 value |= (*p & 0x00FF) << 8; 00040 p++; 00041 sum += value; // Add each pair of bytes into 32 bit accumulator 00042 count -= 2; 00043 } 00044 if (count) sum += *(uint8_t*) p; // Add left-over byte, if any 00045 return sum; 00046 } 00047 uint16_t CheckSumFinDirect(uint32_t sum, int count, void* pData) 00048 { 00049 sum = CheckSumAddDirect(sum, count, pData); 00050 while (sum>>16) sum = (sum & 0xffff) + (sum >> 16); // Add any carries from the sum back into the sum to make it ones complement 00051 return ~sum; 00052 } 00053 uint16_t CheckSum(int count, void* pData) 00054 { 00055 return CheckSumFinDirect(0, count, pData); 00056 }
Generated on Tue Jul 12 2022 18:53:40 by
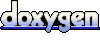