
Controls the central heating system and the lights.
Dependencies: 1-wire clock crypto fram log lpc1768 net web wiz mbed
hall-led.c
00001 #include <stdbool.h> 00002 00003 00004 #include "gpio.h" 00005 #include "radiator.h" 00006 #include "program.h" 00007 #include "mstimer.h" 00008 #include "hall-pb.h" 00009 00010 #define HALL_LED_DIR FIO0DIR(10) // P0.10 == p28; 00011 #define HALL_LED_PIN FIO0PIN(10) 00012 #define HALL_LED_SET FIO0SET(10) 00013 #define HALL_LED_CLR FIO0CLR(10) 00014 00015 #define POSITIVE_FLASH_MS 100 00016 #define NEGATIVE_FLASH_MS 500 00017 00018 void HallLedInit() 00019 { 00020 HALL_LED_DIR = 1; //Set the direction to 1 == output 00021 } 00022 void HallLedMain() 00023 { 00024 static uint32_t hallLedFlashMsTimer = 0; 00025 if (HallPbOverrideMode) 00026 { 00027 if (RadiatorsOn) HALL_LED_SET; else HALL_LED_CLR; 00028 hallLedFlashMsTimer = MsTimerCount; 00029 } 00030 else 00031 { 00032 int flashRate = RadiatorGetHotWaterProtectOn() ? POSITIVE_FLASH_MS : NEGATIVE_FLASH_MS; 00033 if (MsTimerRepetitive(&hallLedFlashMsTimer, flashRate)) 00034 { 00035 static bool flash = false; 00036 flash = !flash; 00037 if (flash) HALL_LED_SET; else HALL_LED_CLR; 00038 } 00039 } 00040 }
Generated on Sat Nov 12 2022 10:03:51 by
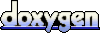