for EVK odin mbed os 5.5
Dependents: Mbed-Connect-Cloud-Demo Mbed-Connect-Cloud-Demo HTTP-all-sensors mbed-cloud-connect-sensor-light ... more
Fork of TSL2561 by
TSL2561.cpp
00001 #include "TSL2561.h" 00002 #include "mbed.h" 00003 TSL2561::TSL2561():i2c(TSL2561_I2C_PINNAME_SDA,TSL2561_I2C_PINNAME_SCL){ 00004 i2c.frequency (300); 00005 _addr = TSL2561_ADDR_FLOAT; 00006 _initialized = false; 00007 _integration = TSL2561_INTEGRATIONTIME_13MS; 00008 _gain = TSL2561_GAIN_16X; 00009 } 00010 TSL2561::TSL2561(uint8_t addr):i2c(TSL2561_I2C_PINNAME_SDA,TSL2561_I2C_PINNAME_SCL) { 00011 _addr = addr; 00012 _initialized = false; 00013 _integration = TSL2561_INTEGRATIONTIME_13MS; 00014 _gain = TSL2561_GAIN_16X; 00015 // we cant do wire initialization till later, because we havent loaded Wire yet 00016 } 00017 TSL2561::TSL2561(PinName sda, PinName scl):i2c(sda, scl) { 00018 _addr = TSL2561_ADDR_FLOAT; 00019 _initialized = false; 00020 _integration = TSL2561_INTEGRATIONTIME_13MS; 00021 _gain = TSL2561_GAIN_16X; 00022 // we cant do wire initialization till later, because we havent loaded Wire yet 00023 } 00024 TSL2561::TSL2561(PinName sda, PinName scl, uint8_t addr):i2c(sda, scl) { 00025 _addr = addr; 00026 _initialized = false; 00027 _integration = TSL2561_INTEGRATIONTIME_13MS; 00028 _gain = TSL2561_GAIN_16X; 00029 // we cant do wire initialization till later, because we havent loaded Wire yet 00030 } 00031 uint16_t TSL2561::getLuminosity (uint8_t channel) { 00032 uint32_t x = getFullLuminosity(); 00033 if (channel == 0) { 00034 // Reads two byte value from channel 0 (visible + infrared) 00035 return (x & 0xFFFF); 00036 } else if (channel == 1) { 00037 // Reads two byte value from channel 1 (infrared) 00038 return (x >> 16); 00039 } else if (channel == 2) { 00040 // Reads all and subtracts out just the visible! 00041 return ( (x & 0xFFFF) - (x >> 16) ); 00042 } 00043 // unknown channel! 00044 return 0; 00045 } 00046 uint32_t TSL2561::getFullLuminosity (void) 00047 { 00048 if (!_initialized) begin(); 00049 // Enable the device by setting the control bit to 0x03 00050 enable(); 00051 // Wait x ms for ADC to complete 00052 switch (_integration) 00053 { 00054 case TSL2561_INTEGRATIONTIME_13MS: 00055 wait_ms(14); 00056 break; 00057 case TSL2561_INTEGRATIONTIME_101MS: 00058 wait_ms(102); 00059 break; 00060 default: 00061 wait_ms(450); 00062 break; 00063 } 00064 uint32_t x; 00065 x = read16(TSL2561_COMMAND_BIT | TSL2561_WORD_BIT | TSL2561_REGISTER_CHAN1_LOW); 00066 x <<= 16; 00067 x |= read16(TSL2561_COMMAND_BIT | TSL2561_WORD_BIT | TSL2561_REGISTER_CHAN0_LOW); 00068 //wait(3); 00069 disable(); 00070 return x; 00071 } 00072 bool TSL2561::begin(void) { 00073 char reg[1]; 00074 reg[0] = TSL2561_REGISTER_ID; 00075 char receivedata[1]; 00076 char read; 00077 i2c.write(_addr<<1, reg, 1); 00078 i2c.read(_addr<<1, receivedata, 1); 00079 read=receivedata[0]; 00080 if (read & 0x0A ) { 00081 } else { 00082 return false; 00083 } 00084 _initialized = true; 00085 // Set default integration time and gain 00086 setTiming(_integration); 00087 setGain(_gain); 00088 // Note: by default, the device is in power down mode on bootup 00089 disable(); 00090 return true; 00091 } 00092 uint16_t TSL2561::read16(uint8_t reg) 00093 { 00094 uint16_t x; 00095 uint16_t t; 00096 char _x; 00097 char _t; 00098 char r[1]; 00099 r[0] = reg; 00100 char receivedata[2]; 00101 i2c.write(_addr<<1, r, 1); 00102 i2c.read(_addr<<1, receivedata, 2); 00103 _t=receivedata[0]; 00104 _x=receivedata[1]; 00105 t=(uint16_t)_t; 00106 x=(uint16_t)_x; 00107 x <<= 8; 00108 x |= t; 00109 return x; 00110 } 00111 void TSL2561::write8 (uint8_t reg, uint8_t value) 00112 { 00113 i2c.start(); 00114 i2c.write(_addr<<1); 00115 i2c.write(reg); 00116 i2c.write(value); 00117 i2c.stop(); 00118 } 00119 void TSL2561::setTiming(tsl2561IntegrationTime_t integration){ 00120 if (!_initialized) begin(); 00121 enable(); 00122 _integration = integration; 00123 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _integration | _gain); 00124 disable(); 00125 //wait(1); 00126 } 00127 void TSL2561::setGain(tsl2561Gain_t gain) { 00128 if (!_initialized) begin(); 00129 enable(); 00130 _gain = gain; 00131 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _integration | _gain); 00132 //write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _gain); 00133 disable(); 00134 //wait(1); 00135 } 00136 void TSL2561::enable(void) 00137 { 00138 if (!_initialized) begin(); 00139 // Enable the device by setting the control bit to 0x03 00140 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_CONTROL, TSL2561_CONTROL_POWERON); 00141 } 00142 void TSL2561::disable(void) 00143 { 00144 if (!_initialized) begin(); 00145 // Disable the device by setting the control bit to 0x03 00146 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_CONTROL, TSL2561_CONTROL_POWEROFF); 00147 }
Generated on Fri Jul 29 2022 02:00:46 by
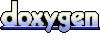