Yet another DS1820 lib, but this one is make in sweat of struggling with other ones. It's a fork really of <insert original as mbed doesn't track idk why> with *added capability* of not blocking.
DS1820.h
00001 /* mbed DS1820 Library, for the Dallas (Maxim) 1-Wire Digital Thermometer 00002 * Copyright (c) 2010, Michael Hagberg Michael@RedBoxCode.com 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_DS1820_H 00024 #define MBED_DS1820_H 00025 00026 #include "mbed.h" 00027 00028 // ****** THIS GLOBAL VARIABLES MUST BE DEFINED IN main.cpp 00029 00030 // Global variables shared between all DS1820 objects 00031 //bool DS1820_done_flag; 00032 //int DS1820_last_descrepancy; 00033 //char DS1820_search_ROM[8]; 00034 00035 /** DS1820 Dallas 1-Wire Temperature Probe 00036 * 00037 * Example: 00038 * @code 00039 * #include "mbed.h" 00040 * 00041 * #include "TextLCD.h" 00042 * #include "DS1820.h" 00043 * 00044 * TextLCD lcd(p25, p26, p21, p22, p23, p24, TextLCD::LCD16x2); // rs, e, d0-d3, layout 00045 * 00046 * const int MAX_PROBES = 16; 00047 * DS1820* probe[MAX_PROBES]; 00048 * 00049 * int main() { 00050 * int i; 00051 * int devices_found=0; 00052 * // Initialize the probe array to DS1820 objects 00053 * for (i = 0; i < MAX_PROBES; i++) 00054 * probe[i] = new DS1820(p27); 00055 * // Initialize global state variables 00056 * probe[0]->search_ROM_setup(); 00057 * // Loop to find all devices on the data line 00058 * while (probe[devices_found]->search_ROM() and devices_found<MAX_PROBES-1) 00059 * devices_found++; 00060 * // If maximum number of probes are found, 00061 * // bump the counter to include the last array entry 00062 * if (probe[devices_found]->ROM[0] != 0xFF) 00063 * devices_found++; 00064 * 00065 * lcd.cls(); 00066 * if (devices_found==0) 00067 * lcd.printf("No devices found"); 00068 * else { 00069 * while (true) { 00070 * probe[0]->convert_temperature(DS1820::all_devices); 00071 * lcd.cls(); 00072 * for (i=0; i<devices_found; i++) { 00073 * lcd.printf("%3.1f ",probe[i]->temperature('f')); 00074 * } 00075 * } 00076 * } 00077 * } 00078 * @endcode 00079 */ 00080 00081 class DS1820 00082 { 00083 public: 00084 enum devices { 00085 this_device, // command applies to only this device 00086 all_devices 00087 }; // command applies to all devices 00088 00089 /** Create a probe object connected to the specified pins 00090 * 00091 * @param data_pin DigitalInOut pin for the data bus 00092 * @param power_pin DigitalOut pin to control the power MOSFET 00093 */ 00094 DS1820(PinName data_pin, PinName power_pin); // Constructor with parasite power pin 00095 00096 /** Create a probe object connected to the specified pin 00097 * this is used when all probes are externally powered 00098 * 00099 * @param data_pin DigitalInOut pin for the data bus 00100 */ 00101 DS1820(PinName data_pin); 00102 00103 /** ROM is a copy of the internal DS1820's ROM 00104 * It's created during the search_ROM() or search_alarm() commands 00105 * 00106 * ROM[0] is the Dallas Family Code 00107 * ROM[1] thru ROM[6] is the 48-bit unique serial number 00108 * ROM[7] is the device xCRC 00109 */ 00110 char ROM[8]; 00111 #define FAMILY_CODE ROM[0] 00112 #define FAMILY_CODE_DS1820 0x10 00113 #define FAMILY_CODE_DS18S20 0x10 00114 #define FAMILY_CODE_DS18B20 0x28 00115 00116 /** RAM is a copy of the internal DS1820's RAM 00117 * It's updated during the read_RAM() command 00118 * which is automaticaly called from any function 00119 * using the RAM values. 00120 */ 00121 char RAM[9]; 00122 00123 /* This function copies the DS1820's RAM into the object's 00124 * RAM[]. 00125 */ 00126 void read_RAM(); 00127 00128 /** This routine initializes the global variables used in 00129 * the search_ROM() and search_alarm() funtions. It should 00130 * be called once before looping to find devices. 00131 */ 00132 void search_ROM_setup(); 00133 00134 /** This routine will search for an unidentified device 00135 * on the bus. It uses the variables in search_ROM_setup 00136 * to remember the pervious ROM address found. 00137 * It will return FALSE if there were no new devices 00138 * discovered on the bus. 00139 */ 00140 bool search_ROM(); 00141 00142 /** This routine will search for an unidentified device 00143 * which has the temperature alarm bit set. It uses the 00144 * variables in search_ROM_setup to remember the pervious 00145 * ROM address found. It will return FALSE if there were 00146 * no new devices with alarms discovered on the bus. 00147 */ 00148 bool search_alarm(); 00149 00150 /** This routine will read the ROM (Family code, serial number 00151 * and Checksum) from a dedicated device on the bus. 00152 * 00153 * NOTE: This command can only be used when there is only one 00154 * DS1820 on the bus. If this command is used when there 00155 * is more than one slave present on the bus, a data 00156 * collision will occur when all the DS1820s attempt to 00157 * respond at the same time. 00158 */ 00159 void read_ROM(); 00160 00161 /** This routine will initiate the temperature conversion within 00162 * a DS1820. There is a built in 750ms delay to allow the 00163 * conversion to complete. 00164 * 00165 * To update all probes on the bus, use a statement such as this: 00166 * probe[0]->convert_temperature(DS1820::all_devices); 00167 * 00168 * @param allows the fnction to apply to a specific device or 00169 * to all devices on the 1-Wire bus. 00170 */ 00171 void convert_temperature(bool blocking = false, devices device=this_device); 00172 00173 /** This function will return the probe temperature. This function 00174 * uses the count remainding values to interpolate the temperature 00175 * to about 1/150th of a degree. Whereas the probe is not spec to 00176 * that precision. It does seem to give a smooth reading to the 00177 * tenth of a degree. 00178 * 00179 * @param scale, may be either 'c' or 'f' 00180 * @returns temperature for that scale 00181 */ 00182 float temperature(char scale='c'); 00183 00184 /** This function calculates the ROM checksum and compares it to the 00185 * xCRC value stored in ROM[7]. 00186 * 00187 * @returns true if the checksum matches, otherwise false. 00188 */ 00189 bool ROM_checksum_error(); 00190 00191 /** This function calculates the RAM checksum and compares it to the 00192 * xCRC value stored in RAM[8]. 00193 * 00194 * @returns true if the checksum matches, otherwise false. 00195 */ 00196 bool RAM_checksum_error(); 00197 00198 /** This function returns the values stored in the temperature 00199 * alarm registers. 00200 * 00201 * @returns a 16 bit integer of TH (upper byte) and TL (lower byte). 00202 */ 00203 bool set_configuration_bits(unsigned int resolution, devices device = this_device); 00204 00205 /** This function sets the temperature resolution for the DS18B20 00206 * in the configuration register. 00207 * 00208 * @param a number between 9 and 12 to specify the resolution 00209 * @returns true if successful 00210 */ 00211 int read_scratchpad(); 00212 00213 /** This function will store the passed data into the DS1820's RAM. 00214 * Note: It does NOT save the data to the EEPROM for retention 00215 * during cycling the power off and on. 00216 * 00217 * @param a 16 bit integer of TH (upper byte) and TL (lower byte). 00218 */ 00219 void write_scratchpad(int data); 00220 00221 /** This function will transfer the TH and TL registers from the 00222 * DS1820's RAM into the EEPROM. 00223 * Note: There is a built in 10ms delay to allow for the 00224 * completion of the EEPROM write cycle. 00225 * 00226 * @param allows the fnction to apply to a specific device or 00227 * to all devices on the 1-Wire bus. 00228 */ 00229 void store_scratchpad(devices device=this_device); 00230 00231 /** This function will copy the stored values from the EEPROM 00232 * into the DS1820's RAM locations for TH and TL. 00233 * 00234 * @param allows the function to apply to a specific device or 00235 * to all devices on the 1-Wire bus. 00236 */ 00237 int recall_scratchpad(devices device=this_device); 00238 00239 /** This function will return the type of power supply for 00240 * a specific device. It can also be used to query all devices 00241 * looking for any device that is parasite powered. 00242 * 00243 * @returns true if the device (or all devices) are Vcc powered, 00244 * returns false if the device (or ANY device) is parasite powered. 00245 */ 00246 bool read_power_supply(devices device=this_device); 00247 00248 void enable_auto_convert(float interval); 00249 private: 00250 Timeout auto_get_new_temperature; 00251 Ticker _autoConvert; 00252 bool _parasite_power; 00253 bool _autoConvertEnabled; 00254 char CRC_byte (char xCRC, char byte ); 00255 bool onewire_reset(); 00256 void match_ROM(); 00257 void skip_ROM(); 00258 bool search_ROM_routine(char command); 00259 void onewire_bit_out (bool bit_data); 00260 void onewire_byte_out(char data); 00261 bool onewire_bit_in(); 00262 char onewire_byte_in(); 00263 void _temperature_raw(); 00264 void _auto_convert_temperature(); 00265 float _last_temperature; 00266 00267 protected: 00268 DigitalInOut _datapin; 00269 DigitalOut _parasitepin; 00270 }; 00271 00272 00273 #endif
Generated on Sat Jul 16 2022 06:07:07 by
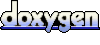