arduino library for mbed
Dependents: Gameduino2 MemoryGame arduino2
arduino.h
00001 #ifndef _ARDUINO_H_ 00002 #define _ARDUINO_H_ 00003 00004 #include "mbed.h" 00005 #include "math.h" 00006 // Macros 00007 00008 #define PI 3.1415926535897932384626433832795 00009 #define HALF_PI 1.5707963267948966192313216916398 00010 #define TWO_PI 6.283185307179586476925286766559 00011 #define DEG_TO_RAD 0.017453292519943295769236907684886 00012 #define RAD_TO_DEG 57.295779513082320876798154814105 00013 00014 #define radians(deg) ((deg)*DEG_TO_RAD) 00015 #define degrees(rad) ((rad)*RAD_TO_DEG) 00016 #define sq(x) ((x)*(x)) 00017 00018 #define pgm_read_word(x) (*(const short int*)x) 00019 #define pgm_read_dword_near(x) (*(const int*)x) 00020 #define pgm_read_word_near(x) (*(const unsigned int*)x) 00021 #define pgm_read_int_near(x) (*(const int*)x) 00022 #define pgm_read_int(x) (*(const int*)x) 00023 #define pgm_read_byte(x) (*(const char*)x) 00024 #define pgm_read_byte_near(x) (*(const char*)x) 00025 #define PROGMEM const 00026 #define char(x) ((char)x) 00027 #define byte(x) ((byte)x) 00028 #define int(x) ((int)x) 00029 #define word(x) ((word)x) 00030 #define long(x) ((long)x) 00031 #define float(x) ((float)x) 00032 00033 #define in_range(c, lo, up) ((uint8_t)c >= lo && (uint8_t)c <= up) 00034 #define isprint(c) in_range(c, 0x20, 0x7f) 00035 #define isdigit(c) in_range(c, '0', '9') 00036 #define isxdigit(c) (isdigit(c) || in_range(c, 'a', 'f') || in_range(c, 'A', 'F')) 00037 #define islower(c) in_range(c, 'a', 'z') 00038 #define isspace(c) (c == ' ' || c == '\f' || c == '\n' || c == '\r' || c == '\t' || c == '\v') 00039 00040 /** Macro for delay() 00041 * 00042 * @param void 00043 */ 00044 #define delay(x) (wait_ms(x)) 00045 /** Macro for delayMicroseconds() 00046 * 00047 * @param void 00048 */ 00049 #define delayMicroseconds(x) (wait_us(x)) 00050 00051 /** Macro for min() 00052 * 00053 * @param any 00054 */ 00055 #define min(a,b) ((a)<(b)?(a):(b)) 00056 /** Macro for max() 00057 * 00058 * @param any 00059 */ 00060 #define max(a,b) ((a)>(b)?(a):(b)) 00061 /** Macro for abs() 00062 * 00063 * @param any 00064 */ 00065 #define abs(x) ((x)>0?(x):(x*-1)) 00066 00067 /** Macro for randomSeed() 00068 * 00069 * @param int 00070 */ 00071 #define randomSeed(x) srand(x) 00072 00073 #define bitRead(value, bit) (((value) >> (bit)) & 0x01) 00074 #define bitSet(value, bit) ((value) |= (1UL << (bit))) 00075 #define bitClear(value, bit) ((value) &= ~(1UL << (bit))) 00076 #define bitWrite(value, bit, bitvalue) (bitvalue ? bitSet(value, bit) : bitClear(value, bit)) 00077 00078 // typedefs 00079 00080 typedef unsigned char prog_uchar; 00081 typedef unsigned char prog_uint8_t; 00082 typedef unsigned int prog_uint16_t; 00083 typedef unsigned int prog_uint32_t; 00084 typedef unsigned char byte; 00085 typedef bool boolean; 00086 typedef unsigned char prog_uchar; 00087 typedef signed char prog_char; 00088 typedef signed long int word; 00089 00090 // function prototypes 00091 00092 void timer_start(void); 00093 long millis(void); 00094 long micros(void); 00095 byte lowByte(short int low); 00096 byte highByte(short int high); 00097 00098 int random(int number); 00099 int random(int numberone, int numbertwo); 00100 #endif
Generated on Thu Aug 4 2022 10:28:31 by
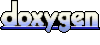