Template for group 4
Fork of RT2_P3_students by
Embed:
(wiki syntax)
Show/hide line numbers
EncoderCounter.cpp
00001 /* 00002 * EncoderCounter.cpp 00003 * Copyright (c) 2017, ZHAW 00004 * All rights reserved. 00005 */ 00006 00007 #include "EncoderCounter.h" 00008 00009 using namespace std; 00010 00011 /** 00012 * Creates and initializes the driver to read the quadrature 00013 * encoder counter of the STM32 microcontroller. 00014 * @param a the input pin for the channel A. 00015 * @param b the input pin for the channel B. 00016 */ 00017 EncoderCounter::EncoderCounter(PinName a, PinName b) { 00018 00019 // check pins 00020 00021 if ((a == PA_6) && (b == PC_7)) { 00022 00023 // pinmap OK for TIM3 CH1 and CH2 00024 00025 TIM = TIM3; 00026 00027 // configure reset and clock control registers 00028 00029 RCC->AHB1ENR |= RCC_AHB1ENR_GPIOCEN; // manually enable port C (port A enabled by mbed library) 00030 00031 // configure general purpose I/O registers 00032 00033 GPIOA->MODER &= ~GPIO_MODER_MODER6; // reset port A6 00034 GPIOA->MODER |= GPIO_MODER_MODER6_1; // set alternate mode of port A6 00035 GPIOA->PUPDR &= ~GPIO_PUPDR_PUPDR6; // reset pull-up/pull-down on port A6 00036 GPIOA->PUPDR |= GPIO_PUPDR_PUPDR6_1; // set input as pull-down 00037 GPIOA->AFR[0] &= ~(0xF << 4*6); // reset alternate function of port A6 00038 GPIOA->AFR[0] |= 2 << 4*6; // set alternate funtion 2 of port A6 00039 00040 GPIOC->MODER &= ~GPIO_MODER_MODER7; // reset port C7 00041 GPIOC->MODER |= GPIO_MODER_MODER7_1; // set alternate mode of port C7 00042 GPIOC->PUPDR &= ~GPIO_PUPDR_PUPDR7; // reset pull-up/pull-down on port C7 00043 GPIOC->PUPDR |= GPIO_PUPDR_PUPDR7_1; // set input as pull-down 00044 GPIOC->AFR[0] &= ~0xF0000000; // reset alternate function of port C7 00045 GPIOC->AFR[0] |= 2 << 4*7; // set alternate funtion 2 of port C7 00046 00047 // configure reset and clock control registers 00048 00049 RCC->APB1RSTR |= RCC_APB1RSTR_TIM3RST; //reset TIM3 controller 00050 RCC->APB1RSTR &= ~RCC_APB1RSTR_TIM3RST; 00051 00052 RCC->APB1ENR |= RCC_APB1ENR_TIM3EN; // TIM3 clock enable 00053 00054 } else if ((a == PB_6) && (b == PB_7)) { 00055 00056 // pinmap OK for TIM4 CH1 and CH2 00057 00058 TIM = TIM4; 00059 00060 // configure reset and clock control registers 00061 00062 RCC->AHB1ENR |= RCC_AHB1ENR_GPIOBEN; // manually enable port B (port A enabled by mbed library) 00063 00064 // configure general purpose I/O registers 00065 00066 GPIOB->MODER &= ~GPIO_MODER_MODER6; // reset port B6 00067 GPIOB->MODER |= GPIO_MODER_MODER6_1; // set alternate mode of port B6 00068 GPIOB->PUPDR &= ~GPIO_PUPDR_PUPDR6; // reset pull-up/pull-down on port B6 00069 GPIOB->PUPDR |= GPIO_PUPDR_PUPDR6_1; // set input as pull-down 00070 GPIOB->AFR[0] &= ~(0xF << 4*6); // reset alternate function of port B6 00071 GPIOB->AFR[0] |= 2 << 4*6; // set alternate funtion 2 of port B6 00072 00073 GPIOB->MODER &= ~GPIO_MODER_MODER7; // reset port B7 00074 GPIOB->MODER |= GPIO_MODER_MODER7_1; // set alternate mode of port B7 00075 GPIOB->PUPDR &= ~GPIO_PUPDR_PUPDR7; // reset pull-up/pull-down on port B7 00076 GPIOB->PUPDR |= GPIO_PUPDR_PUPDR7_1; // set input as pull-down 00077 GPIOB->AFR[0] &= ~0xF0000000; // reset alternate function of port B7 00078 GPIOB->AFR[0] |= 2 << 4*7; // set alternate funtion 2 of port B7 00079 00080 // configure reset and clock control registers 00081 00082 RCC->APB1RSTR |= RCC_APB1RSTR_TIM4RST; //reset TIM4 controller 00083 RCC->APB1RSTR &= ~RCC_APB1RSTR_TIM4RST; 00084 00085 RCC->APB1ENR |= RCC_APB1ENR_TIM4EN; // TIM4 clock enable 00086 00087 } else { 00088 00089 printf("pinmap not found for peripheral\n"); 00090 } 00091 00092 // configure general purpose timer 3 or 4 00093 00094 TIM->CR1 = 0x0000; // counter disable 00095 TIM->CR2 = 0x0000; // reset master mode selection 00096 TIM->SMCR = TIM_SMCR_SMS_1 | TIM_SMCR_SMS_0; // counting on both TI1 & TI2 edges 00097 TIM->CCMR1 = TIM_CCMR1_CC2S_0 | TIM_CCMR1_CC1S_0; 00098 TIM->CCMR2 = 0x0000; // reset capture mode register 2 00099 TIM->CCER = TIM_CCER_CC2E | TIM_CCER_CC1E; 00100 TIM->CNT = 0x0000; // reset counter value 00101 TIM->ARR = 0xFFFF; // auto reload register 00102 TIM->CR1 = TIM_CR1_CEN; // counter enable 00103 } 00104 00105 EncoderCounter::~EncoderCounter() {} 00106 00107 /** 00108 * Resets the counter value to zero. 00109 */ 00110 void EncoderCounter::reset() { 00111 00112 TIM->CNT = 0x0000; 00113 } 00114 00115 /** 00116 * Resets the counter value to a given offset value. 00117 * @param offset the offset value to reset the counter to. 00118 */ 00119 void EncoderCounter::reset(short offset) { 00120 00121 TIM->CNT = -offset; 00122 } 00123 00124 /** 00125 * Reads the quadrature encoder counter value. 00126 * @return the quadrature encoder counter as a signed 16-bit integer value. 00127 */ 00128 short EncoderCounter::read() { 00129 00130 return (short)(-TIM->CNT); 00131 } 00132 00133 /** 00134 * The empty operator is a shorthand notation of the <code>read()</code> method. 00135 */ 00136 EncoderCounter::operator short() { 00137 00138 return read(); 00139 }
Generated on Fri Jul 15 2022 05:28:02 by
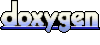