.
Embed:
(wiki syntax)
Show/hide line numbers
Unwrapper_2pi.cpp
00001 /* 00002 */ 00003 00004 #include "Unwrapper_2pi.h" 00005 #define pi 3.141592653589793 00006 using namespace std; 00007 00008 Unwrapper_2pi::Unwrapper_2pi(void) 00009 { 00010 last_value = 0.0; 00011 turns = 0; 00012 } 00013 00014 Unwrapper_2pi::~Unwrapper_2pi() {} 00015 00016 void Unwrapper_2pi::reset(void) 00017 { 00018 last_value = 0.0; 00019 turns = 0; 00020 } 00021 00022 float Unwrapper_2pi::doStep(float in) 00023 { 00024 float temp = in + 2*pi*(float)turns; 00025 if((temp - last_value) > pi){ 00026 temp -= 2*pi; 00027 turns--; 00028 } 00029 else if((temp - last_value) < -pi){ 00030 temp += 2*pi; 00031 turns++; 00032 } 00033 last_value = temp; 00034 return (temp); 00035 }
Generated on Wed Jul 13 2022 23:05:32 by
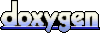