.
Embed:
(wiki syntax)
Show/hide line numbers
LinearCharacteristics.cpp
00001 #include "LinearCharacteristics.h" 00002 00003 using namespace std; 00004 00005 LinearCharacteristics::LinearCharacteristics(float gain,float offset){ // standard lin characteristics 00006 this->gain = gain; 00007 this->offset = offset; 00008 } 00009 00010 LinearCharacteristics::LinearCharacteristics(float xmin,float xmax, float ymin, float ymax){ // standard lin characteristics 00011 this->gain = (ymax - ymin)/(xmax - xmin); 00012 this->offset = xmax - ymax/this->gain; 00013 this->ulim = 999999.0; 00014 this->llim = -999999.0; 00015 00016 } 00017 LinearCharacteristics::LinearCharacteristics(float xmin,float xmax, float ymin, float ymax,float ll, float ul){ // standard lin characteristics 00018 this->gain = (ymax - ymin)/(xmax - xmin); 00019 this->offset = xmax - ymax/this->gain; 00020 this->llim = ll; 00021 this->ulim = ul; 00022 00023 } 00024 00025 LinearCharacteristics::~LinearCharacteristics() {} 00026 00027 00028 float LinearCharacteristics::evaluate(float x) 00029 { 00030 float dum = this->gain*(x - this->offset); 00031 if(dum > this->ulim) 00032 dum = this->ulim; 00033 if(dum < this->llim) 00034 dum = this->llim; 00035 return dum; 00036 } 00037 00038 void LinearCharacteristics::setup(float xmin,float xmax, float ymin, float ymax){ // standard lin characteristics 00039 this->gain = (ymax - ymin)/(xmax - xmin); 00040 this->offset = xmax - ymax/this->gain; 00041 this->ulim = 999999.0; 00042 this->llim = -999999.0; 00043 } 00044 void LinearCharacteristics::setup(float xmin,float xmax, float ymin, float ymax,float ll, float ul){ // standard lin characteristics 00045 this->gain = (ymax - ymin)/(xmax - xmin); 00046 this->offset = xmax - ymax/this->gain; 00047 this->llim = ll; 00048 this->ulim = ul; 00049 }
Generated on Wed Jul 13 2022 23:05:32 by
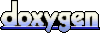