
dev
Dependencies: MTS-Serial libmDot-mbed5
Fork of Dot-AT-Firmware by
CmdLinkCheckThreshold.cpp
00001 #include "CmdLinkCheckThreshold.h" 00002 00003 CmdLinkCheckThreshold::CmdLinkCheckThreshold() : 00004 Command("Link Check Threshold", "AT+LCT", "Set threshold for number of link check or ACK failures to tolerate, (0: off, N: number of failures)", "(0-255)") 00005 { 00006 _queryable = true; 00007 } 00008 00009 uint32_t CmdLinkCheckThreshold::action(std::vector<std::string> args) 00010 { 00011 if (args.size() == 1) 00012 { 00013 CommandTerminal::Serial()->writef("%u\r\n", CommandTerminal::Dot()->getLinkCheckThreshold()); 00014 } 00015 else if (args.size() == 2) 00016 { 00017 int count; 00018 sscanf(args[1].c_str(), "%d", &count); 00019 00020 if (CommandTerminal::Dot()->setLinkCheckThreshold(count) != mDot::MDOT_OK) 00021 { 00022 CommandTerminal::setErrorMessage(CommandTerminal::Dot()->getLastError());; 00023 return 1; 00024 } 00025 } 00026 00027 return 0; 00028 } 00029 00030 bool CmdLinkCheckThreshold::verify(std::vector<std::string> args) 00031 { 00032 if (args.size() == 1) 00033 return true; 00034 00035 if (args.size() == 2) 00036 { 00037 int count; 00038 sscanf(args[1].c_str(), "%d", &count); 00039 00040 if (count < 0 || count > 255) { 00041 CommandTerminal::setErrorMessage("Invalid count, expects (0-255))"); 00042 return false; 00043 } 00044 00045 return true; 00046 } 00047 00048 CommandTerminal::setErrorMessage("Invalid arguments"); 00049 return false; 00050 }
Generated on Tue Jul 12 2022 13:49:31 by
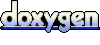