
a
Embed:
(wiki syntax)
Show/hide line numbers
servo.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file SERVO.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-2017 00007 * @brief This file contains the class declaration for the steering SERVO 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Include guard */ 00013 #ifndef SERVO_HPP 00014 #define SERVO_HPP 00015 00016 #include <mbed.h> 00017 00018 00019 namespace drivers{ 00020 /** 00021 * @brief It is used for implementing SERVO functionality. 00022 * 00023 */ 00024 class SERVO 00025 { 00026 public: 00027 /* Constructor */ 00028 SERVO(PinName _pwm); 00029 /* Destructor */ 00030 ~SERVO(); 00031 /* Set angle */ 00032 void SetAngle(float angle); //-25 to 25 degr 00033 private: 00034 /* convert angle to duty cycle */ 00035 float conversion(float angle); //angle to duty cycle 00036 /* PWM value */ 00037 PwmOut pwm; 00038 /* Current angle */ 00039 float current_angle; 00040 }; 00041 }; // namespace drivers 00042 00043 00044 #endif
Generated on Tue Jul 12 2022 22:40:51 by
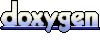