
a
Embed:
(wiki syntax)
Show/hide line numbers
serialmonitor.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file SerialMonitor.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-2017 00007 * @brief This file contains the class declaration for the serial communication 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Inclusion guard */ 00013 #ifndef SERIAL_MONITOR_HPP 00014 #define SERIAL_MONITOR_HPP 00015 00016 /* The mbed library */ 00017 #include <mbed.h> 00018 #include <map> 00019 #include <array> 00020 /* Function objects */ 00021 #include <functional> 00022 #include<TaskManager/taskmanager.hpp> 00023 #include <Queue/queue.hpp> 00024 00025 00026 namespace serial{ 00027 00028 /** 00029 * @brief It is used for implementing serial communciation. 00030 * 00031 */ 00032 class CSerialMonitor : public task::CTask 00033 { 00034 public: 00035 typedef mbed::Callback<void(char const *, char *)> FCallback; 00036 typedef std::map<string,FCallback> CSerialSubscriberMap; 00037 00038 /* Constructor */ 00039 CSerialMonitor(Serial& f_serialPort 00040 ,CSerialSubscriberMap f_serialSubscriberMap); 00041 private: 00042 /* Rx callback */ 00043 static void RxCallback(void *thisPointer); 00044 /* Tx callback */ 00045 static void TxCallback(void *thisPointer); 00046 /* Rx callback actions */ 00047 void serialRxCallback(); 00048 /* Tx callback actions */ 00049 void serialTxCallback(); 00050 /* Run method */ 00051 virtual void _run(); 00052 00053 /* Reference to serial object */ 00054 Serial& m_serialPort; 00055 /* Rx buffer */ 00056 CQueue<char,255> m_RxBuffer; 00057 /* Tx buffer */ 00058 CQueue<char,255> m_TxBuffer; 00059 /* Data buffer */ 00060 array<char,256> m_parseBuffer; 00061 /* Parse iterator */ 00062 array<char,256>::iterator m_parseIt; 00063 /* Serial subscriber */ 00064 CSerialSubscriberMap m_serialSubscriberMap; 00065 }; 00066 00067 }; // namespace serial 00068 00069 #endif // SERIAL_MONITOR_HPP 00070
Generated on Tue Jul 12 2022 22:40:51 by
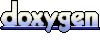