
a
Embed:
(wiki syntax)
Show/hide line numbers
serialmonitor.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file SerialMonitor.cpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-2017 00007 * @brief This file contains the class definition for the serial communication 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 #include <SerialMonitor/serialmonitor.hpp> 00013 00014 00015 namespace serial{ 00016 00017 /** @brief CSerialMonitor class constructor 00018 * 00019 * 00020 * @param f_serialPort reference to serial object 00021 * @param f_serialSubscriberMap sensor mounteed on left front part 00022 */ 00023 CSerialMonitor::CSerialMonitor(Serial& f_serialPort 00024 ,CSerialSubscriberMap f_serialSubscriberMap) 00025 :task::CTask(0) 00026 , m_serialPort(f_serialPort) 00027 , m_RxBuffer() 00028 , m_TxBuffer() 00029 , m_parseBuffer() 00030 , m_parseIt(m_parseBuffer.begin()) 00031 , m_serialSubscriberMap(f_serialSubscriberMap) 00032 { 00033 m_serialPort.attach(mbed::callback(&CSerialMonitor::RxCallback, this), Serial::RxIrq); 00034 m_serialPort.attach(mbed::callback(&CSerialMonitor::TxCallback, this), Serial::TxIrq); 00035 } 00036 00037 /** @brief Rx callback 00038 * 00039 * @param thisPointer the object pointer 00040 * 00041 */ 00042 void CSerialMonitor::RxCallback(void *thisPointer) 00043 { 00044 CSerialMonitor* self = static_cast<CSerialMonitor*>(thisPointer); 00045 self->serialRxCallback(); 00046 } 00047 00048 /** @brief Tx callback 00049 * 00050 * @param thisPointer the object pointer 00051 * 00052 */ 00053 void CSerialMonitor::TxCallback(void *thisPointer) 00054 { 00055 CSerialMonitor* self = static_cast<CSerialMonitor*>(thisPointer); 00056 self->serialTxCallback(); 00057 } 00058 00059 /** @brief Rx callback actions 00060 * 00061 * @param None 00062 * 00063 */ 00064 void CSerialMonitor::serialRxCallback() 00065 { 00066 __disable_irq(); 00067 while ((m_serialPort.readable()) && (!m_RxBuffer.isFull())) { 00068 char l_c = m_serialPort.getc(); 00069 m_RxBuffer.push(l_c); 00070 } 00071 __enable_irq(); 00072 return; 00073 } 00074 00075 /** @brief Tx callback actions 00076 * 00077 * @param None 00078 * 00079 */ 00080 void CSerialMonitor::serialTxCallback() 00081 { 00082 __disable_irq(); 00083 while ((m_serialPort.writeable()) && (!m_TxBuffer.isEmpty())) { 00084 m_serialPort.putc(m_TxBuffer.pop()); 00085 } 00086 __enable_irq(); 00087 return; 00088 } 00089 00090 /** @brief Run method 00091 * 00092 * @param None 00093 * @param None 00094 */ 00095 void CSerialMonitor::_run() 00096 { 00097 if ((!m_RxBuffer.isEmpty())) 00098 { 00099 char l_c = m_RxBuffer.pop(); 00100 // m_serialPort.printf("%c",l_c); 00101 if ('#' == l_c) 00102 { 00103 m_parseIt = m_parseBuffer.begin(); 00104 m_parseIt[0] = l_c; 00105 m_parseIt++; 00106 return; 00107 } 00108 if (m_parseIt != m_parseBuffer.end()) 00109 { 00110 if (l_c == '\n') 00111 { 00112 if ((';' == m_parseIt[-3]) && (';' == m_parseIt[-2]) && ('\r' == m_parseIt[-1])) 00113 { 00114 char l_msgID[5]; 00115 char l_msg[256]; 00116 00117 uint32_t res = sscanf(m_parseBuffer.data(),"#%4s:%s;;",l_msgID,l_msg); 00118 if (res == 2) 00119 { 00120 auto l_pair = m_serialSubscriberMap.find(l_msgID); 00121 if (l_pair != m_serialSubscriberMap.end()) 00122 { 00123 char l_resp[256] = "no response given"; 00124 string s(l_resp); 00125 l_pair->second(l_msg,l_resp); 00126 m_serialPort.printf("@%s:%s\r\n",l_msgID,l_resp); 00127 } 00128 } 00129 m_parseIt = m_parseBuffer.begin(); 00130 } 00131 } 00132 m_parseIt[0] = l_c; 00133 m_parseIt++; 00134 return; 00135 } 00136 } 00137 } 00138 00139 }; // namespace serial
Generated on Tue Jul 12 2022 22:40:51 by
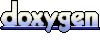