
a
Embed:
(wiki syntax)
Show/hide line numbers
sensortask.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file SensorTask.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class declaration for the sensor task 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Include guard */ 00013 #ifndef SENSOR_TASK_HPP 00014 #define SENSOR_TASK_HPP 00015 00016 /* Standard C library for basic mathematical operations */ 00017 #include <math.h> 00018 #include<TaskManager/taskmanager.hpp> 00019 #include <Drivers/vnh.hpp> 00020 #include <SerialMonitor/serialmonitor.hpp> 00021 #include <Controllers/controller.hpp> 00022 #include <SystemModels/systemmodels.hpp> 00023 00024 #include <Encoders/encoderinterface.hpp > 00025 00026 namespace examples 00027 { 00028 00029 namespace sensors{ 00030 00031 00032 //! CDISTFTest class. 00033 /*! 00034 * It inherits class task::CTask. 00035 * It is used for testing distance sensors operation. 00036 */ 00037 class CDISTFTest:public task::CTask 00038 { 00039 public: 00040 /* Constructor */ 00041 CDISTFTest(uint32_t f_period 00042 ,Serial& f_serial); 00043 00044 private: 00045 /* Run method */ 00046 void _run(); 00047 00048 /* Reference to serial object */ 00049 Serial& m_serial; 00050 /* Transfer function */ 00051 systemmodels::lti::siso::CDiscreteTransferFucntion<float,3,3> m_tf; 00052 }; 00053 00054 //! CEncoderSender class. 00055 /*! 00056 * It inherits class task::CTask. 00057 * It is used for sending acquired data. 00058 */ 00059 class CEncoderSender:public task::CTask 00060 { 00061 public: 00062 /* Constructor */ 00063 CEncoderSender(uint32_t f_period 00064 ,encoders::IEncoderGetter& f_encoder 00065 ,Serial& f_serial); 00066 /* Serial callback */ 00067 static void staticSerialCallback(void* obj,char const * a, char * b); 00068 private: 00069 /* Serial callback implementation */ 00070 void serialCallback(char const * a, char * b); 00071 /* Run method */ 00072 void _run(); 00073 00074 /* Active flag */ 00075 bool m_isActived; 00076 /* Encoder value getter */ 00077 encoders::IEncoderGetter& m_encoder; 00078 /* Serial communication member*/ 00079 Serial& m_serial; 00080 }; 00081 }; // namespace sensors 00082 }; // namespace examples 00083 00084 00085 #endif
Generated on Tue Jul 12 2022 22:40:51 by
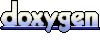