
a
Embed:
(wiki syntax)
Show/hide line numbers
move.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file MOVE.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class declaration for the moving 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Include guard */ 00013 #ifndef MOVE_HPP 00014 #define MOVE_HPP 00015 00016 #include <Drivers/servo.hpp> 00017 #include <Drivers/vnh.hpp> 00018 00019 /** 00020 * @brief Move class 00021 * It is used for executing move commands. 00022 * 00023 */ 00024 class Move 00025 { 00026 public: 00027 /* Constructor */ 00028 Move(PinName, PinName, PinName, PinName, PinName);//A0 00029 /* Destructor */ 00030 ~Move(); 00031 /* Steer */ 00032 void Steer(float angle);// -25 to + 25 degrees, - (left), + (right) 00033 /* Speed */ 00034 void Speed(float speed);//-100 to + 100 -(back), + (front) 00035 /* Brake */ 00036 void Brake(); 00037 /* Inverse */ 00038 void Inverse(float f_speed); 00039 /* Test */ 00040 void TestCar(); 00041 /* Reset */ 00042 void ResetCar(); 00043 /* Return bridge object */ 00044 drivers::VNH& getVNH(); 00045 00046 private: 00047 /* Servo pbject */ 00048 drivers::SERVO servo; 00049 /* Bridge object */ 00050 drivers::VNH vnh; 00051 }; 00052 00053 #endif
Generated on Tue Jul 12 2022 22:40:50 by
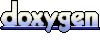