
a
Embed:
(wiki syntax)
Show/hide line numbers
distancesensors.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file DistanceSensors.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date 08-January-2018 00007 * @brief This file contains the class definition for the distance sensors read 00008 * methods. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Include guard */ 00013 #ifndef DISTANCESENSORS_HPP 00014 #define DISTANCESENSORS_HPP 00015 00016 #include <mbed.h> 00017 #include <array> 00018 #include <string> 00019 #include<TaskManager/taskmanager.hpp> 00020 #include <SHARP_IR_distance\DistanceSensors\distancesensors.hpp> 00021 using namespace std; 00022 00023 /** 00024 * @brief It is used for reading the attached distance sensors. 00025 * 00026 */ 00027 template<class T> 00028 class CDistanceSensors: public task::CTask 00029 { 00030 private: 00031 /* The sensors list */ 00032 T m_sensor_list; 00033 /* Index of current sensor */ 00034 uint8_t m_index; 00035 /* The maximum number of sensors that are used */ 00036 const uint8_t m_N; 00037 /* Flags for enabloing/disabling timeout */ 00038 Timeout m_disableTimeOut; 00039 Timeout m_enableTimeOut; 00040 /* Message that contains the read values */ 00041 string m_message; 00042 00043 public: 00044 /* Construnctor */ 00045 CDistanceSensors(uint32_t f_period, 00046 float f_baseTick, 00047 T& f_sensor_list); 00048 /* Destructor */ 00049 ~CDistanceSensors(); 00050 /* Callback for reading sensor value */ 00051 void ReadCallback(void); 00052 /* Run method */ 00053 void _run(void); 00054 }; 00055 00056 #include "DistanceSensors.inl" 00057 00058 /* Include guard */ 00059 #endif
Generated on Tue Jul 12 2022 22:40:50 by
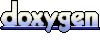