
sending data to DeviceHub.net
Dependencies: EthernetInterface MQTT mbed-rtos mbed
main.cpp
00001 #include "MQTTClient.h" 00002 #include "MQTTEthernet.h" 00003 #include "rtos.h" 00004 #include <stdlib.h> 00005 00006 #define __APP_SW_REVISION__ "1" 00007 00008 #define MQTT_PORT 1883 00009 00010 #define MQTT_MAX_PACKET_SIZE 250 00011 00012 #include "K64F.h" 00013 00014 bool quickstartMode = true; 00015 00016 bool connected = false; 00017 bool mqttConnecting = false; 00018 bool netConnected = false; 00019 bool netConnecting = false; 00020 bool ethernetInitialising = true; 00021 int connack_rc = 0; // MQTT connack return code 00022 int retryAttempt = 0; 00023 00024 int blink_interval = 0; 00025 00026 char* ip_addr = ""; 00027 char* gateway_addr = ""; 00028 char* host_addr = ""; 00029 int connectTimeout = 1000; 00030 00031 #define API_KEY "ba8b7a2d-b690-4351-8521-100b45c09dd8" 00032 #define PROJECT_ID "4505" 00033 #define AN_SENSOR_NAME "test" 00034 #define DEVICE_UUID "1deffe91-f938-48c5-aaea-3aef29af7869" 00035 #define ID "aaa" 00036 00037 char* pubTopic = "/a/"API_KEY"/p/"PROJECT_ID"/d/"DEVICE_UUID"/sensor/"AN_SENSOR_NAME"/data"; 00038 char id[30] = ID; 00039 00040 int connect(MQTT::Client<MQTTEthernet, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTEthernet* ipstack) 00041 { 00042 const char* host = "mqtt.devicehub.net"; 00043 00044 char hostname[strlen(host) + 1]; 00045 sprintf(hostname, "%s", host); 00046 EthernetInterface& eth = ipstack->getEth(); 00047 ip_addr = eth.getIPAddress(); 00048 gateway_addr = eth.getGateway(); 00049 00050 char clientId[strlen(id)]; 00051 sprintf(clientId, "%s",id); 00052 00053 // Network debug statements 00054 LOG("=====================================\n"); 00055 LOG("Connecting Ethernet.\n"); 00056 LOG("IP ADDRESS: %s\n", eth.getIPAddress()); 00057 LOG("MAC ADDRESS: %s\n", eth.getMACAddress()); 00058 LOG("Gateway: %s\n", eth.getGateway()); 00059 LOG("Network Mask: %s\n", eth.getNetworkMask()); 00060 LOG("Server Hostname: %s\n", hostname); 00061 LOG("Client ID: %s\n", clientId); 00062 LOG("=====================================\n"); 00063 00064 netConnecting = true; 00065 int rc = ipstack->connect(hostname, MQTT_PORT, connectTimeout); 00066 if (rc != 0) 00067 { 00068 WARN("IP Stack connect returned: %d\n", rc); 00069 return rc; 00070 } 00071 netConnected = true; 00072 netConnecting = false; 00073 00074 // MQTT Connect 00075 mqttConnecting = true; 00076 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00077 data.MQTTVersion = 3; 00078 data.clientID.cstring = clientId; 00079 00080 00081 if ((rc = client->connect(data)) == 0) 00082 { 00083 connected = true; 00084 } 00085 else 00086 WARN("MQTT connect returned %d\n", rc); 00087 if (rc >= 0) 00088 connack_rc = rc; 00089 mqttConnecting = false; 00090 return rc; 00091 } 00092 00093 00094 int getConnTimeout(int attemptNumber) 00095 { // First 10 attempts try within 3 seconds, next 10 attempts retry after every 1 minute 00096 // after 20 attempts, retry every 10 minutes 00097 return (attemptNumber < 10) ? 3 : (attemptNumber < 20) ? 60 : 600; 00098 } 00099 00100 00101 void attemptConnect(MQTT::Client<MQTTEthernet, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTEthernet* ipstack) 00102 { 00103 connected = false; 00104 00105 while (!linkStatus()) 00106 { 00107 wait(1.0f); 00108 WARN("Ethernet link not present. Check cable connection\n"); 00109 } 00110 00111 while (connect(client, ipstack) != MQTT_CONNECTION_ACCEPTED) 00112 { 00113 00114 int timeout = getConnTimeout(++retryAttempt); 00115 WARN("Retry attempt number %d waiting %d\n", retryAttempt, timeout); 00116 00117 // this works - reset the system when the retry count gets to a threshold 00118 if (retryAttempt == 5) 00119 NVIC_SystemReset(); 00120 else 00121 wait(timeout); 00122 } 00123 } 00124 00125 00126 int publish(MQTT::Client<MQTTEthernet, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTEthernet* ipstack) 00127 { 00128 MQTT::Message message; 00129 00130 int analog_sensor = rand() % 100; 00131 char buf[250]; 00132 sprintf(buf,"{\"value\": %d }", analog_sensor); 00133 message.qos = MQTT::QOS0; 00134 message.retained = false; 00135 message.dup = false; 00136 message.payload = (void*)buf; 00137 message.payloadlen = strlen(buf); 00138 00139 LOG("Publishing %s\n", buf); 00140 return client->publish(pubTopic, message); 00141 } 00142 00143 00144 char* getMac(EthernetInterface& eth, char* buf, int buflen) // Obtain MAC address 00145 { 00146 strncpy(buf, eth.getMACAddress(), buflen); 00147 00148 char* pos; // Remove colons from mac address 00149 while ((pos = strchr(buf, ':')) != NULL) 00150 memmove(pos, pos + 1, strlen(pos) + 1); 00151 return buf; 00152 } 00153 00154 int main() 00155 { 00156 00157 led2 = LED2_OFF; // K64F: turn off the main board LED 00158 00159 LOG("***** DeviceHub.net example *****\n"); 00160 MQTTEthernet ipstack; 00161 ethernetInitialising = false; 00162 MQTT::Client<MQTTEthernet, Countdown, MQTT_MAX_PACKET_SIZE> client(ipstack); 00163 LOG("Ethernet Initialized\n"); 00164 00165 if (quickstartMode) 00166 getMac(ipstack.getEth(), id, sizeof(id)); 00167 00168 attemptConnect(&client, &ipstack); 00169 00170 00171 blink_interval = 0; 00172 int count = 0; 00173 while (true) 00174 { 00175 if (++count == 100) 00176 { // Publish a message every second 00177 if (publish(&client, &ipstack) != 0) 00178 attemptConnect(&client, &ipstack); // if we have lost the connection 00179 count = 0; 00180 } 00181 00182 if (blink_interval == 0) 00183 led2 = LED2_OFF; 00184 else if (count % blink_interval == 0) 00185 led2 = !led2; 00186 client.yield(10); // allow the MQTT client to receive messages 00187 } 00188 }
Generated on Tue Jul 12 2022 17:58:36 by
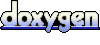