
added
Dependencies: BNO055_fusion PowerControl mbed
Fork of TEAM_G_FLOW_RIDA by
SAFETY.cpp
00001 #include "SAFETY.h" 00002 00003 #define USR_POWERDOWN (0x104) 00004 00005 // "kick" or "feed" the dog - reset the watchdog timer 00006 // by writing this required bit pattern 00007 void SAFETY::kick(void) 00008 { 00009 LPC_WDT->WDFEED = 0xAA; 00010 LPC_WDT->WDFEED = 0x55; 00011 } 00012 00013 void SAFETY::kick(float s) 00014 { 00015 LPC_WDT->WDCLKSEL = 0x1; // Set CLK src to PCLK 00016 uint32_t clk = SystemCoreClock / 16; // WD has a fixed /4 prescaler, PCLK default is /4 00017 LPC_WDT->WDTC = s * (float)clk; 00018 LPC_WDT->WDMOD = 0x3; // Enabled and Reset 00019 kick(); 00020 } 00021 00022 int SAFETY::semihost_powerdown() 00023 { 00024 uint32_t arg; 00025 return __semihost(USR_POWERDOWN, &arg); 00026 } 00027 00028 void SAFETY::init(uint32_t brownOutRoutine) 00029 { 00030 //Power Down Ethernet 00031 PHY_PowerDown(); 00032 //Power Down USB Chip 00033 semihost_powerdown(); 00034 //Setup Brown Out Interrupt 00035 NVIC_SetVector(BOD_IRQn, brownOutRoutine); 00036 //Enable Brown Out Interrupt 00037 NVIC_EnableIRQ(BOD_IRQn); 00038 }
Generated on Tue Jul 12 2022 22:21:03 by
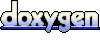