
a
Dependencies: HMC6352 PID mbed
uart1.cpp
00001 00002 #include "mbed.h" 00003 #include "uart1.h" 00004 #include "HMC6352.h" 00005 00006 extern Serial device2; 00007 extern HMC6352 compass; 00008 00009 extern uint8_t state; 00010 00011 float ultrasonicVal[4]; 00012 uint8_t direction; 00013 uint8_t Distance; 00014 uint8_t IR_found; 00015 uint8_t xbee; 00016 00017 void dev_rx() 00018 { 00019 static uint8_t count; 00020 static uint8_t RecData[RECEIVE_DATA_NUM]; 00021 00022 RecData[count] = device2.getc(); 00023 00024 if(RecData[KEY] == KEYCODE){ 00025 count++; 00026 }else{ 00027 count = 0; 00028 } 00029 if(count >= RECEIVE_DATA_NUM){ 00030 if(RecData[CHECK] == CHECKCODE){ 00031 //mbedleds = 1; 00032 direction = RecData[DIRECTION]; 00033 Distance = RecData[DISTANCE]; 00034 ultrasonicVal[0] = (RecData[SONIC1_1] + (RecData[SONIC1_2] << 8)) / 10.0; 00035 ultrasonicVal[1] = (RecData[SONIC2_1] + (RecData[SONIC2_2] << 8)) / 10.0; 00036 ultrasonicVal[2] = (RecData[SONIC3_1] + (RecData[SONIC3_2] << 8)) / 10.0; 00037 ultrasonicVal[3] = (RecData[SONIC4_1] + (RecData[SONIC4_2] << 8)) / 10.0; 00038 xbee = RecData[XBEE]; 00039 IR_found = xbee; 00040 } 00041 count = 0; 00042 } 00043 } 00044 00045 void dev_tx() 00046 { 00047 static uint8_t count2; 00048 static uint8_t SendData[SEND_DATA_NUM]; 00049 00050 //mbedleds = 2; 00051 00052 if(count2 >= SEND_DATA_NUM){ 00053 SendData[KEY2] = KEYCODE2; 00054 SendData[DATA1] = ((int)(compass.sample())) >> 8 ; 00055 SendData[DATA2] = (int)(compass.sample()); 00056 SendData[DATA3] = state; 00057 SendData[DATA4] = 1; 00058 SendData[CHECK2] = CHECKCODE2; 00059 00060 count2 = 0; 00061 00062 } 00063 device2.putc(SendData[count2]); 00064 00065 count2++; 00066 }
Generated on Fri Jul 15 2022 06:47:45 by
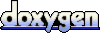