
This program is communication with MMA7660 and LM75B. First receive sensor value of MMA7660 and LM75B. And MMA7660 value is control LED 2, 3, 4. LM75B is send another device.
Dependencies: C12832 LM75B MMA7660 mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "LM75B.h" 00003 #include "C12832.h" 00004 #include "rtos.h" 00005 #include "MMA7660.h" 00006 00007 //3-Axis Sensor port setting 00008 MMA7660 MMA(p28, p27); 00009 //Temperature Sensor port setting 00010 LM75B sensor(p28,p27); 00011 //LED setting connecting 3-Axis Sensor 00012 DigitalOut MMA_X(LED2); 00013 DigitalOut MMA_Y(LED3); 00014 DigitalOut MMA_Z(LED4); 00015 //Serial port setting with PC 00016 Serial pc(USBTX,USBRX); 00017 //LCD port setting 00018 C12832 lcd(p5, p7, p6, p8, p11); 00019 //LED setting connecting Temperature Sensor 00020 DigitalOut led1(LED1); 00021 //CAN port setting 00022 CAN can1(p9, p10); 00023 00024 00025 00026 char counter, counter1, enter; 00027 float temp; 00028 float sensor_1[3]; 00029 //X-Axis thread 00030 void led1_thread(void const *args){ 00031 00032 MMA_X = 0; 00033 while(1){ 00034 sensor_1[0]=(float)MMA.x(); 00035 if(sensor_1[0]>0.5) { 00036 MMA_X = 1; 00037 wait(1); 00038 MMA_X = 0; 00039 } 00040 Thread::wait(100); 00041 } 00042 } 00043 //Y-Axis thread 00044 void led2_thread(void const *args){ 00045 00046 MMA_Y = 0; 00047 while(1){ 00048 sensor_1[1]=(float)MMA.y(); 00049 if(sensor_1[1]>0.5) { 00050 MMA_Y = 1; 00051 wait(1); 00052 MMA_Y = 0; 00053 } 00054 Thread::wait(100); 00055 } 00056 } 00057 //Z-Axis thread 00058 void led3_thread(void const *args){ 00059 00060 MMA_Z = 0; 00061 while(1){ 00062 sensor_1[2]=(float)MMA.z(); 00063 if(sensor_1[2]<0.5) { 00064 MMA_Z = 1; 00065 wait(1); 00066 MMA_Z = 0; 00067 } 00068 Thread::wait(100); 00069 } 00070 } 00071 //Float to Integer 00072 int getInteger(float n) 00073 { 00074 return (int)n; 00075 } 00076 //Getting Fracting of Float 00077 int getFraction(float n) 00078 { 00079 float a; 00080 a = n-getInteger(n); 00081 a = a*100; 00082 return (int)a; 00083 } 00084 00085 int main() { 00086 //Declare thread of 3-Axis 00087 Thread thread1(led1_thread); 00088 Thread thread2(led2_thread); 00089 Thread thread3(led3_thread); 00090 //Check connecting of 3-Axis 00091 if (MMA.testConnection()) printf("Sensor connect \n"); 00092 while(1){ 00093 temp=sensor.read(); 00094 counter=getInteger(temp); 00095 counter1=getFraction(temp); 00096 enter='1'; 00097 lcd.cls(); 00098 lcd.locate(0,3); 00099 lcd.printf("Temp = %.3f\n", temp); 00100 00101 can1.write(CANMessage(1337, &counter, 1)); 00102 wait(0.2); 00103 00104 can1.write(CANMessage(1337, &counter1, 1)); 00105 wait(0.2); 00106 00107 can1.write(CANMessage(1337, &enter, 1)); 00108 00109 printf("Message sent : %d.%d \n", counter, counter1); 00110 led1 = !led1; 00111 wait(0.2); 00112 } 00113 00114 }
Generated on Wed Aug 3 2022 03:02:19 by
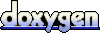