PIR sensor 2
Fork of PIRSensorWithTwitter by
Embed:
(wiki syntax)
Show/hide line numbers
WizFi310_msg.cpp
00001 /* 00002 * Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi250 00021 */ 00022 /* Copyright (C) 2017 Wiznet, MIT License 00023 * port to the Wiznet Module WizFi310 00024 */ 00025 00026 #include "WizFi310.h" 00027 00028 #ifdef CFG_ENABLE_RTOS 00029 #undef WIZ_DBG 00030 #define WIZ_DBG(x, ...) 00031 #endif 00032 00033 //daniel 00034 char g_asyncbuf[256]; 00035 00036 // This function is operating in ISR. So you can't use debug message. 00037 void WizFi310::recvData ( char c ) 00038 { 00039 static int cid, sub, len, count; 00040 static int is_mqtt_data = 0; 00041 char tbf[10]; 00042 00043 switch(_state.mode) 00044 { 00045 case MODE_COMMAND: 00046 switch(c) 00047 { 00048 case 0: 00049 case 0x0a: // LF 00050 case 0x0d: // CR 00051 break; 00052 00053 case '{': 00054 _state.buf->flush(); 00055 _state.mode = MODE_DATA_RX; 00056 sub = 0; 00057 break; 00058 00059 default: 00060 _state.buf->flush(); 00061 _state.buf->queue(c); 00062 _state.mode = MODE_CMDRESP; 00063 break; 00064 } 00065 break; 00066 00067 case MODE_CMDRESP: 00068 switch(c) 00069 { 00070 case 0: 00071 break; 00072 case 0x0a: // LF 00073 break; 00074 case 0x0d: // CR 00075 if (_flow == 2) setRts(false); // block 00076 _state.mode = MODE_COMMAND; 00077 parseMessage(); 00078 if (_flow == 2) setRts(true); // release 00079 break; 00080 default: 00081 _state.buf->queue(c); 00082 break; 00083 } 00084 break; 00085 00086 case MODE_DATA_RX: 00087 00088 switch(sub) 00089 { 00090 case 0: 00091 // cid 00092 if( (c >= '0') && (c <= '9') ) 00093 { 00094 cid = x2i(c); 00095 } 00096 else if ( c == ',' ) 00097 { 00098 sub++; 00099 count = 0; 00100 len = 0; 00101 } 00102 //daniel add for mqtt 00103 else if ( c == 'Q' ) 00104 { 00105 cid = 0; 00106 is_mqtt_data = 1; 00107 } 00108 // 00109 else 00110 { 00111 _state.mode = MODE_COMMAND; 00112 } 00113 break; 00114 00115 case 1: 00116 // ip 00117 // if ((c >= '0' && c <= '9') || c == '.') 00118 if (((c >= '0' && c <= '9') || c == '.') && is_mqtt_data == 0 ) 00119 { 00120 _con[cid].ip[count] = c; 00121 count++; 00122 } 00123 else if( c == ',' ) 00124 { 00125 _con[cid].ip[count] = '\0'; 00126 _con[cid].port = 0; 00127 sub++; 00128 } 00129 //daniel for mqtt 00130 else if( is_mqtt_data == 1) 00131 { 00132 rcvd_mqtt_topic[count] = c; 00133 count++; 00134 } 00135 // else 00136 else if( is_mqtt_data == 0 ) 00137 { 00138 _state.mode = MODE_COMMAND; 00139 } 00140 break; 00141 00142 case 2: 00143 // port 00144 if ( c >= '0' && c <= '9' ) 00145 { 00146 _con[cid].port = (_con[cid].port * 10) + ( c - '0' ); 00147 } 00148 else if( c == ',') 00149 { 00150 sub++; 00151 count = 0; 00152 } 00153 else 00154 { 00155 _state.mode = MODE_COMMAND; 00156 } 00157 break; 00158 00159 case 3: 00160 // data length 00161 if ( c >= '0' && c <= '9' ) 00162 { 00163 //_con[cid].recv_length = (_con[cid].recv_length * 10) + (c - '0'); 00164 len = (len * 10) + (c - '0'); 00165 } 00166 else if( c == '}' ) 00167 { 00168 sub++; 00169 count = 0; 00170 _con[cid].recv_length = len; 00171 } 00172 else 00173 { 00174 _state.mode = MODE_COMMAND; 00175 } 00176 break; 00177 00178 default: 00179 00180 if(_con[cid].buf != NULL) 00181 { 00182 _con[cid].buf->queue(c); 00183 if(_con[cid].buf->available() > CFG_DATA_SIZE - 16 ) 00184 { 00185 setRts(false); // blcok 00186 _con[cid].received = true; 00187 WIZ_WARN("buf full"); 00188 } 00189 } 00190 _con[cid].recv_length--; 00191 if(_con[cid].recv_length == 0) 00192 { 00193 //WIZ_DBG("recv cid: %d, count : %d, len : %d",cid, count, len); 00194 //sprintf(tbf, "recv cid: %d, count : %d, len : %d",cid, count, len); 00195 //strcat(g_asyncbuf, tbf); 00196 _con[cid].received = true; 00197 _state.mode = MODE_COMMAND; 00198 } 00199 break; 00200 } 00201 break; 00202 } 00203 } 00204 00205 00206 //#define MSG_TABLE_NUM 6 00207 //daniel 00208 #define MSG_TABLE_NUM 8 00209 #define RES_TABLE_NUM 7 00210 int WizFi310::parseMessage () { 00211 int i; 00212 char buf[128]; 00213 00214 static const struct MSG_TABLE { 00215 const char msg[24]; 00216 void (WizFi310::*func)(const char *); 00217 } msg_table[MSG_TABLE_NUM] = { 00218 {"[OK]", &WizFi310::msgOk}, 00219 {"[ERROR]", &WizFi310::msgError}, 00220 {"[ERROR:INVALIDINPUT]", &WizFi310::msgError}, 00221 {"[CONNECT ", &WizFi310::msgConnect}, 00222 {"[DISCONNECT ", &WizFi310::msgDisconnect}, 00223 {"[LISTEN ", &WizFi310::msgListen}, 00224 //daniel 00225 {"[MQTT CONNECT]", &WizFi310::msgMQTTConnect}, 00226 {"[MQTT DISCONNECT]", &WizFi310::msgMQTTDisconnect}, 00227 }; 00228 static const struct RES_TABLE{ 00229 const Response res; 00230 void (WizFi310::*func)(const char *); 00231 }res_table[RES_TABLE_NUM]={ 00232 {RES_NULL, NULL}, 00233 {RES_MACADDRESS, &WizFi310::resMacAddress}, 00234 // {RES_WJOIN, &WizFi310::resWJOIN}, 00235 {RES_CONNECT, &WizFi310::resConnect}, 00236 {RES_SSEND, &WizFi310::resSSEND}, 00237 {RES_FDNS, &WizFi310::resFDNS}, 00238 {RES_SMGMT, &WizFi310::resSMGMT}, 00239 {RES_WSTATUS, &WizFi310::resWSTATUS}, 00240 }; 00241 00242 00243 for( i=0; i<sizeof(buf); i++ ) 00244 { 00245 if( _state.buf->dequeue(&buf[i]) == false ) break; 00246 } 00247 00248 buf[i] = '\0'; 00249 //strncpy(_state.dbgRespBuf, buf, sizeof(buf) ); 00250 //WIZ_DBG("%s\r\n",_state.dbgRespBuf); 00251 00252 if(_state.res != RES_NULL) 00253 { 00254 for( i=0; i<RES_TABLE_NUM; i++) 00255 { 00256 if(res_table[i].res == _state.res) 00257 { 00258 //WIZ_DBG("parse res %d '%s'\r\n", i, buf); 00259 if(res_table[i].func != NULL) 00260 { 00261 (this->*(res_table[i].func))(buf); 00262 } 00263 00264 if(res_table[i].res == RES_CONNECT && _state.n < 2) 00265 return -1; 00266 } 00267 } 00268 } 00269 00270 for( i=0; i<MSG_TABLE_NUM; i++) 00271 { 00272 if( strncmp(buf, msg_table[i].msg, strlen(msg_table[i].msg)) == 0 ) 00273 { 00274 //WIZ_DBG("parse msg '%s'\r\n", buf); 00275 if(msg_table[i].func != NULL) 00276 { 00277 (this->*(msg_table[i].func))(buf); 00278 } 00279 return 0; 00280 } 00281 } 00282 00283 return -1; 00284 } 00285 00286 00287 void WizFi310::msgOk (const char *buf) 00288 { 00289 _state.ok = true; 00290 } 00291 00292 void WizFi310::msgError (const char *buf) 00293 { 00294 _state.failure = true; 00295 } 00296 00297 void WizFi310::msgConnect (const char *buf) 00298 { 00299 int cid; 00300 00301 if (buf[9] < '0' || buf[9] > '8' || buf[10] != ']') return; 00302 00303 cid = x2i(buf[9]); 00304 00305 initCon(cid, true); 00306 _state.cid = cid; 00307 _con[cid].accept = true; 00308 _con[cid].parent = cid; 00309 } 00310 00311 void WizFi310::msgDisconnect (const char *buf) 00312 { 00313 int cid; 00314 00315 if(buf[12] < '0' || buf[12] > '8' || buf[13] != ']') return; 00316 00317 cid = x2i(buf[12]); 00318 _con[cid].connected = false; 00319 } 00320 00321 00322 void WizFi310::msgMQTTConnect (const char *buf) 00323 { 00324 int cid = 0; 00325 00326 //if (buf[9] < '0' || buf[9] > '8' || buf[10] != ']') return; 00327 00328 //cid = x2i(buf[9]); 00329 initCon(cid, true); 00330 _state.cid = cid; 00331 _con[cid].accept = true; 00332 _con[cid].parent = cid; 00333 00334 _con[cid].connected = true; 00335 _state.res = RES_NULL; 00336 _state.ok = true; 00337 } 00338 00339 void WizFi310::msgMQTTDisconnect (const char *buf) 00340 { 00341 int cid = 0; 00342 00343 //if(buf[12] < '0' || buf[12] > '8' || buf[13] != ']') return; 00344 00345 //cid = x2i(buf[12]); 00346 _con[cid].connected = false; 00347 } 00348 00349 00350 void WizFi310::msgListen (const char *buf) 00351 { 00352 int cid; 00353 00354 if(buf[8] < '0' || buf[8] > '8' || buf[9] != ']') return; 00355 00356 cid = x2i(buf[8]); 00357 _state.cid = cid; 00358 } 00359 00360 void WizFi310::resMacAddress (const char *buf) 00361 { 00362 if( buf[2] == ':' && buf[5] == ':') 00363 { 00364 strncpy(_state.mac, buf, sizeof(_state.mac)); 00365 _state.mac[17] = 0; 00366 _state.res = RES_NULL; 00367 00368 if(strncmp(_state.mac,CFG_DEFAULT_MAC,sizeof(CFG_DEFAULT_MAC)) == 0) 00369 _state.ok = false; 00370 _state.ok = true; 00371 } 00372 } 00373 00374 void WizFi310::resConnect (const char *buf) 00375 { 00376 int cid; 00377 00378 if (buf[0] == '[' && buf[1] == 'O' && buf[2] == 'K' && buf[3] == ']') 00379 { 00380 _state.n++; 00381 } 00382 else if( buf[0] == '[' && buf[1] == 'C' && buf[2] == 'O' && buf[3] == 'N' && 00383 buf[4] == 'N' && buf[5] == 'E' && buf[6] == 'C' && buf[7] == 'T') 00384 { 00385 cid = x2i(buf[9]); 00386 _state.cid = cid; 00387 _state.n++; 00388 } 00389 00390 if(_state.n >= 2) 00391 { 00392 _state.res = RES_NULL; 00393 _state.ok = true; 00394 } 00395 } 00396 00397 void WizFi310::resSSEND (const char *buf) 00398 { 00399 if(_state.cid != -1) 00400 { 00401 _state.res = RES_NULL; 00402 _state.ok = true; 00403 } 00404 } 00405 00406 void WizFi310::resFDNS (const char *buf) 00407 { 00408 int i; 00409 00410 for(i=0; i<strlen(buf); i++) 00411 { 00412 if( (buf[i] < '0' || buf[i] > '9') && buf[i] != '.' ) 00413 { 00414 return; 00415 } 00416 } 00417 00418 strncpy(_state.resolv, buf, sizeof(_state.resolv)); 00419 _state.res = RES_NULL; 00420 } 00421 00422 void WizFi310::resSMGMT (const char *buf) 00423 { 00424 int cid, i; 00425 char *c; 00426 00427 if( (buf[0] < '0' || buf[0] > '8') ) return; 00428 00429 cid = x2i(buf[0]); 00430 if( cid != _state.cid ) return; 00431 00432 // IP 00433 c = (char*)(buf+6); 00434 for( i=0; i<16; i++ ) 00435 { 00436 if( *(c+i) == ':') 00437 { 00438 _con[cid].ip[i] = '\0'; 00439 i++; 00440 break; 00441 } 00442 if( ( *(c+i) < '0' || *(c+i) > '9') && *(c+i) != '.' ) return; 00443 _con[cid].ip[i] = *(c+i); 00444 } 00445 00446 // Port 00447 c = (c+i); 00448 _con[cid].port = 0; 00449 for( i=0; i<5; i++ ) 00450 { 00451 if( *(c+i) == '/') break; 00452 if( *(c+i) < '0' || *(c+i) > '9' ) return; 00453 00454 _con[cid].port = (_con[cid].port * 10) + ( *(c+i) - '0' ); 00455 } 00456 00457 _state.res = RES_NULL; 00458 } 00459 00460 void WizFi310::resWSTATUS (const char *buf) 00461 { 00462 int idx=0,sep_cnt=0; 00463 int ip_idx=0,gw_idx=0; 00464 00465 if(_state.n == 0) 00466 { 00467 _state.n++; 00468 } 00469 else if(_state.n == 1) 00470 { 00471 for(idx=0;buf[idx]!='\r';idx++) 00472 { 00473 if(buf[idx] =='/') 00474 { 00475 sep_cnt++; 00476 continue; 00477 } 00478 00479 if( sep_cnt == 2) // IP Address 00480 { 00481 _state.ip[ip_idx++] = buf[idx]; 00482 } 00483 else if(sep_cnt == 3) 00484 { 00485 _state.gateway[gw_idx++] = buf[idx]; 00486 } 00487 } 00488 _state.ip[ip_idx] = '\0'; 00489 _state.gateway[gw_idx] = '\0'; 00490 _state.res = RES_NULL; 00491 } 00492 }
Generated on Wed Jul 13 2022 23:19:10 by
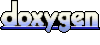