PIR sensor 2
Fork of PIRSensorWithTwitter by
Embed:
(wiki syntax)
Show/hide line numbers
TCPSocketServer.h
00001 /* 00002 * Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi250 00021 */ 00022 /* Copyright (C) 2017 Wiznet, MIT License 00023 * port to the Wiznet Module WizFi310 00024 */ 00025 00026 #ifndef TCPSOCKETSERVER_H 00027 #define TCPSOCKETSERVER_H 00028 00029 #include "Socket/Socket.h" 00030 #include "TCPSocketConnection.h" 00031 00032 /** TCP Server. 00033 */ 00034 class TCPSocketServer : public Socket { 00035 public: 00036 /** Instantiate a TCP Server. 00037 */ 00038 TCPSocketServer(); 00039 00040 /** Bind a socket to a specific port. 00041 \param port The port to listen for incoming connections on. 00042 \return 0 on success, -1 on failure. 00043 */ 00044 int bind(int port); 00045 00046 /** Start listening for incoming connections. 00047 \param backlog number of pending connections that can be queued up at any 00048 one time [Default: 1]. 00049 \return 0 on success, -1 on failure. 00050 */ 00051 int listen(int backlog=1); 00052 00053 /** Accept a new connection. 00054 \param connection A TCPSocketConnection instance that will handle the incoming connection. 00055 \return 0 on success, -1 on failure. 00056 */ 00057 00058 int accept(TCPSocketConnection& connection); 00059 }; 00060 00061 #endif
Generated on Wed Jul 13 2022 23:19:10 by
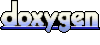