PingPong-ClickButtonToWork
Dependents: Lora_SX1272_Coragem-dev-shared Lora_SX1272_serial
SX1272.h
00001 /* 00002 * Library for LoRa 868 / 915MHz SX1272 LoRa module 00003 * 00004 * Copyright (C) Libelium Comunicaciones Distribuidas S.L. 00005 * http://www.libelium.com 00006 * 00007 * This program is free software: you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation, either version 3 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * This program is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program. If not, see http://www.gnu.org/licenses/. 00019 * 00020 * Version: 1.1 00021 * Design: David Gascón 00022 * Implementation: Covadonga Albiñana & Victor Boria 00023 */ 00024 00025 //Adaptation for NUCLEO STM32 by C.Dupaty. According to a program by C.Pham 00026 // Tested on NUCLEO L073RZ with SX1272 MB2xAS Arduino shield 00027 // please visit http://cpham.perso.univ-pau.fr/LORA/LoRaDevices.html 00028 // for original version for ARDUINO 00029 00030 00031 #ifndef SX1272_h 00032 #define SX1272_h 00033 00034 /****************************************************************************** 00035 * Includes 00036 ******************************************************************************/ 00037 00038 #include <stdlib.h> 00039 #include <stdint.h> 00040 #include <ArduinoLike.h> 00041 #include "mbed.h" 00042 00043 #ifndef inttypes_h 00044 #include <inttypes.h> 00045 #endif 00046 00047 /****************************************************************************** 00048 * Definitions & Declarations 00049 *****************************************************************************/ 00050 00051 // added by C. Pham 00052 // do not remove! 00053 #define W_REQUESTED_ACK 00054 //#define W_NET_KEY 00055 //#define W_INITIALIZATION 00056 00057 #define SX1272Chip 0 00058 #define SX1276Chip 1 00059 00060 #define SX1272_debug_mode 0 // 0 1 2 00061 //#define DEBUG_CAD // 00062 00063 //! MACROS // 00064 #define bitRead(value, bit) (((value) >> (bit)) & 0x01) // read a bit 00065 #define bitSet(value, bit) ((value) |= (1UL << (bit))) // set bit to '1' 00066 #define bitClear(value, bit) ((value) &= ~(1UL << (bit))) // set bit to '0' 00067 00068 //! REGISTERS // 00069 00070 #define REG_FIFO 0x00 00071 #define REG_OP_MODE 0x01 00072 #define REG_BITRATE_MSB 0x02 00073 #define REG_BITRATE_LSB 0x03 00074 #define REG_FDEV_MSB 0x04 00075 #define REG_FDEV_LSB 0x05 00076 #define REG_FRF_MSB 0x06 00077 #define REG_FRF_MID 0x07 00078 #define REG_FRF_LSB 0x08 00079 #define REG_PA_CONFIG 0x09 00080 #define REG_PA_RAMP 0x0A 00081 #define REG_OCP 0x0B 00082 #define REG_LNA 0x0C 00083 #define REG_RX_CONFIG 0x0D 00084 #define REG_FIFO_ADDR_PTR 0x0D 00085 #define REG_RSSI_CONFIG 0x0E 00086 #define REG_FIFO_TX_BASE_ADDR 0x0E 00087 #define REG_RSSI_COLLISION 0x0F 00088 #define REG_FIFO_RX_BASE_ADDR 0x0F 00089 #define REG_RSSI_THRESH 0x10 00090 #define REG_FIFO_RX_CURRENT_ADDR 0x10 00091 #define REG_RSSI_VALUE_FSK 0x11 00092 #define REG_IRQ_FLAGS_MASK 0x11 00093 #define REG_RX_BW 0x12 00094 #define REG_IRQ_FLAGS 0x12 00095 #define REG_AFC_BW 0x13 00096 #define REG_RX_NB_BYTES 0x13 00097 #define REG_OOK_PEAK 0x14 00098 #define REG_RX_HEADER_CNT_VALUE_MSB 0x14 00099 #define REG_OOK_FIX 0x15 00100 #define REG_RX_HEADER_CNT_VALUE_LSB 0x15 00101 #define REG_OOK_AVG 0x16 00102 #define REG_RX_PACKET_CNT_VALUE_MSB 0x16 00103 #define REG_RX_PACKET_CNT_VALUE_LSB 0x17 00104 #define REG_MODEM_STAT 0x18 00105 #define REG_PKT_SNR_VALUE 0x19 00106 #define REG_AFC_FEI 0x1A 00107 #define REG_PKT_RSSI_VALUE 0x1A 00108 #define REG_AFC_MSB 0x1B 00109 #define REG_RSSI_VALUE_LORA 0x1B 00110 #define REG_AFC_LSB 0x1C 00111 #define REG_HOP_CHANNEL 0x1C 00112 #define REG_FEI_MSB 0x1D 00113 #define REG_MODEM_CONFIG1 0x1D 00114 #define REG_FEI_LSB 0x1E 00115 #define REG_MODEM_CONFIG2 0x1E 00116 #define REG_PREAMBLE_DETECT 0x1F 00117 #define REG_SYMB_TIMEOUT_LSB 0x1F 00118 #define REG_RX_TIMEOUT1 0x20 00119 #define REG_PREAMBLE_MSB_LORA 0x20 00120 #define REG_RX_TIMEOUT2 0x21 00121 #define REG_PREAMBLE_LSB_LORA 0x21 00122 #define REG_RX_TIMEOUT3 0x22 00123 #define REG_PAYLOAD_LENGTH_LORA 0x22 00124 #define REG_RX_DELAY 0x23 00125 #define REG_MAX_PAYLOAD_LENGTH 0x23 00126 #define REG_OSC 0x24 00127 #define REG_HOP_PERIOD 0x24 00128 #define REG_PREAMBLE_MSB_FSK 0x25 00129 #define REG_FIFO_RX_BYTE_ADDR 0x25 00130 #define REG_PREAMBLE_LSB_FSK 0x26 00131 // added by C. Pham 00132 #define REG_MODEM_CONFIG3 0x26 00133 // end 00134 #define REG_SYNC_CONFIG 0x27 00135 #define REG_SYNC_VALUE1 0x28 00136 #define REG_SYNC_VALUE2 0x29 00137 #define REG_SYNC_VALUE3 0x2A 00138 #define REG_SYNC_VALUE4 0x2B 00139 #define REG_SYNC_VALUE5 0x2C 00140 #define REG_SYNC_VALUE6 0x2D 00141 #define REG_SYNC_VALUE7 0x2E 00142 #define REG_SYNC_VALUE8 0x2F 00143 #define REG_PACKET_CONFIG1 0x30 00144 #define REG_PACKET_CONFIG2 0x31 00145 #define REG_DETECT_OPTIMIZE 0x31 00146 #define REG_PAYLOAD_LENGTH_FSK 0x32 00147 #define REG_NODE_ADRS 0x33 00148 #define REG_BROADCAST_ADRS 0x34 00149 #define REG_FIFO_THRESH 0x35 00150 #define REG_SEQ_CONFIG1 0x36 00151 #define REG_SEQ_CONFIG2 0x37 00152 #define REG_DETECTION_THRESHOLD 0x37 00153 #define REG_TIMER_RESOL 0x38 00154 // added by C. Pham 00155 #define REG_SYNC_WORD 0x39 00156 //end 00157 #define REG_TIMER1_COEF 0x39 00158 #define REG_TIMER2_COEF 0x3A 00159 #define REG_IMAGE_CAL 0x3B 00160 #define REG_TEMP 0x3C 00161 #define REG_LOW_BAT 0x3D 00162 #define REG_IRQ_FLAGS1 0x3E 00163 #define REG_IRQ_FLAGS2 0x3F 00164 #define REG_DIO_MAPPING1 0x40 00165 #define REG_DIO_MAPPING2 0x41 00166 #define REG_VERSION 0x42 00167 #define REG_AGC_REF 0x43 00168 #define REG_AGC_THRESH1 0x44 00169 #define REG_AGC_THRESH2 0x45 00170 #define REG_AGC_THRESH3 0x46 00171 #define REG_PLL_HOP 0x4B 00172 #define REG_TCXO 0x58 00173 #define REG_PA_DAC 0x5A 00174 #define REG_PLL 0x5C 00175 #define REG_PLL_LOW_PN 0x5E 00176 #define REG_FORMER_TEMP 0x6C 00177 #define REG_BIT_RATE_FRAC 0x70 00178 00179 // added by C. Pham 00180 // copied from LoRaMAC-Node 00181 /*! 00182 * RegImageCal 00183 */ 00184 #define RF_IMAGECAL_AUTOIMAGECAL_MASK 0x7F 00185 #define RF_IMAGECAL_AUTOIMAGECAL_ON 0x80 00186 #define RF_IMAGECAL_AUTOIMAGECAL_OFF 0x00 // Default 00187 00188 #define RF_IMAGECAL_IMAGECAL_MASK 0xBF 00189 #define RF_IMAGECAL_IMAGECAL_START 0x40 00190 00191 #define RF_IMAGECAL_IMAGECAL_RUNNING 0x20 00192 #define RF_IMAGECAL_IMAGECAL_DONE 0x00 // Default 00193 00194 #define RF_IMAGECAL_TEMPCHANGE_HIGHER 0x08 00195 #define RF_IMAGECAL_TEMPCHANGE_LOWER 0x00 00196 00197 #define RF_IMAGECAL_TEMPTHRESHOLD_MASK 0xF9 00198 #define RF_IMAGECAL_TEMPTHRESHOLD_05 0x00 00199 #define RF_IMAGECAL_TEMPTHRESHOLD_10 0x02 // Default 00200 #define RF_IMAGECAL_TEMPTHRESHOLD_15 0x04 00201 #define RF_IMAGECAL_TEMPTHRESHOLD_20 0x06 00202 00203 #define RF_IMAGECAL_TEMPMONITOR_MASK 0xFE 00204 #define RF_IMAGECAL_TEMPMONITOR_ON 0x00 // Default 00205 #define RF_IMAGECAL_TEMPMONITOR_OFF 0x01 00206 00207 // added by C. Pham 00208 // The crystal oscillator frequency of the module 00209 #define RH_LORA_FXOSC 32000000.0 00210 00211 // The Frequency Synthesizer step = RH_LORA_FXOSC / 2^^19 00212 #define RH_LORA_FCONVERT (524288 / RH_LORA_FXOSC) 00213 00214 // Frf = frf(Hz)*2^19/RH_LORA_FXOSC 00215 00216 ///// 00217 00218 //FREQUENCY CHANNELS: 00219 // added by C. Pham for Senegal 00220 const uint32_t CH_04_868 = 0xD7CCCC; // channel 04, central freq = 863.20MHz 00221 const uint32_t CH_05_868 = 0xD7E000; // channel 05, central freq = 863.50MHz 00222 const uint32_t CH_06_868 = 0xD7F333; // channel 06, central freq = 863.80MHz 00223 const uint32_t CH_07_868 = 0xD80666; // channel 07, central freq = 864.10MHz 00224 const uint32_t CH_08_868 = 0xD81999; // channel 08, central freq = 864.40MHz 00225 const uint32_t CH_09_868 = 0xD82CCC; // channel 09, central freq = 864.70MHz 00226 // 00227 const uint32_t CH_10_868 = 0xD84CCC; // channel 10, central freq = 865.20MHz, = 865200000*RH_LORA_FCONVERT 00228 const uint32_t CH_11_868 = 0xD86000; // channel 11, central freq = 865.50MHz 00229 const uint32_t CH_12_868 = 0xD87333; // channel 12, central freq = 865.80MHz 00230 const uint32_t CH_13_868 = 0xD88666; // channel 13, central freq = 866.10MHz 00231 const uint32_t CH_14_868 = 0xD89999; // channel 14, central freq = 866.40MHz 00232 const uint32_t CH_15_868 = 0xD8ACCC; // channel 15, central freq = 866.70MHz 00233 const uint32_t CH_16_868 = 0xD8C000; // channel 16, central freq = 867.00MHz 00234 const uint32_t CH_17_868 = 0xD90000; // channel 17, central freq = 868.00MHz 00235 00236 // added by C. Pham 00237 const uint32_t CH_18_868 = 0xD90666; // 868.1MHz for LoRaWAN test 00238 // end 00239 const uint32_t CH_00_900 = 0xE1C51E; // channel 00, central freq = 903.08MHz 00240 const uint32_t CH_01_900 = 0xE24F5C; // channel 01, central freq = 905.24MHz 00241 const uint32_t CH_02_900 = 0xE2D999; // channel 02, central freq = 907.40MHz 00242 const uint32_t CH_03_900 = 0xE363D7; // channel 03, central freq = 909.56MHz 00243 const uint32_t CH_04_900 = 0xE3EE14; // channel 04, central freq = 911.72MHz 00244 const uint32_t CH_05_900 = 0xE47851; // channel 05, central freq = 913.88MHz 00245 const uint32_t CH_06_900 = 0xE5028F; // channel 06, central freq = 916.04MHz 00246 const uint32_t CH_07_900 = 0xE58CCC; // channel 07, central freq = 918.20MHz 00247 const uint32_t CH_08_900 = 0xE6170A; // channel 08, central freq = 920.36MHz 00248 const uint32_t CH_09_900 = 0xE6A147; // channel 09, central freq = 922.52MHz 00249 const uint32_t CH_10_900 = 0xE72B85; // channel 10, central freq = 924.68MHz 00250 const uint32_t CH_11_900 = 0xE7B5C2; // channel 11, central freq = 926.84MHz 00251 const uint32_t CH_12_900 = 0xE4C000; // default channel 915MHz, the module is configured with it 00252 00253 // added by C. Pham 00254 const uint32_t CH_00_433 = 0x6C5333; // 433.3MHz 00255 const uint32_t CH_01_433 = 0x6C6666; // 433.6MHz 00256 const uint32_t CH_02_433 = 0x6C7999; // 433.9MHz 00257 const uint32_t CH_03_433 = 0x6C9333; // 434.3MHz 00258 // end 00259 00260 //LORA BANDWIDTH: 00261 // modified by C. Pham 00262 const uint8_t SX1272_BW_125 = 0x00; 00263 const uint8_t SX1272_BW_250 = 0x01; 00264 const uint8_t SX1272_BW_500 = 0x02; 00265 00266 // use the following constants with setBW() 00267 const uint8_t BW_7_8 = 0x00; 00268 const uint8_t BW_10_4 = 0x01; 00269 const uint8_t BW_15_6 = 0x02; 00270 const uint8_t BW_20_8 = 0x03; 00271 const uint8_t BW_31_25 = 0x04; 00272 const uint8_t BW_41_7 = 0x05; 00273 const uint8_t BW_62_5 = 0x06; 00274 const uint8_t BW_125 = 0x07; 00275 const uint8_t BW_250 = 0x08; 00276 const uint8_t BW_500 = 0x09; 00277 // end 00278 00279 const double SignalBwLog[] = 00280 { 00281 5.0969100130080564143587833158265, 00282 5.397940008672037609572522210551, 00283 5.6989700043360188047862611052755 00284 }; 00285 00286 //LORA CODING RATE: 00287 const uint8_t CR_5 = 0x01; 00288 const uint8_t CR_6 = 0x02; 00289 const uint8_t CR_7 = 0x03; 00290 const uint8_t CR_8 = 0x04; 00291 00292 //LORA SPREADING FACTOR: 00293 const uint8_t SF_6 = 0x06; 00294 const uint8_t SF_7 = 0x07; 00295 const uint8_t SF_8 = 0x08; 00296 const uint8_t SF_9 = 0x09; 00297 const uint8_t SF_10 = 0x0A; 00298 const uint8_t SF_11 = 0x0B; 00299 const uint8_t SF_12 = 0x0C; 00300 00301 //LORA MODES: 00302 const uint8_t LORA_SLEEP_MODE = 0x80; 00303 const uint8_t LORA_STANDBY_MODE = 0x81; 00304 const uint8_t LORA_TX_MODE = 0x83; 00305 const uint8_t LORA_RX_MODE = 0x85; 00306 00307 // added by C. Pham 00308 const uint8_t LORA_CAD_MODE = 0x87; 00309 #define LNA_MAX_GAIN 0x23 00310 #define LNA_OFF_GAIN 0x00 00311 #define LNA_LOW_GAIN 0x20 00312 // end 00313 00314 const uint8_t LORA_STANDBY_FSK_REGS_MODE = 0xC1; 00315 00316 //FSK MODES: 00317 const uint8_t FSK_SLEEP_MODE = 0x00; 00318 const uint8_t FSK_STANDBY_MODE = 0x01; 00319 const uint8_t FSK_TX_MODE = 0x03; 00320 const uint8_t FSK_RX_MODE = 0x05; 00321 00322 //OTHER CONSTANTS: 00323 00324 const uint8_t HEADER_ON = 0; 00325 const uint8_t HEADER_OFF = 1; 00326 const uint8_t CRC_ON = 1; 00327 const uint8_t CRC_OFF = 0; 00328 const uint8_t LORA = 1; 00329 const uint8_t FSK = 0; 00330 const uint8_t BROADCAST_0 = 0x00; 00331 const uint8_t MAX_LENGTH = 255; 00332 const uint8_t MAX_PAYLOAD = 251; 00333 const uint8_t MAX_LENGTH_FSK = 64; 00334 const uint8_t MAX_PAYLOAD_FSK = 60; 00335 //modified by C. Pham, 7 instead of 5 because we added a type field which should be PKT_TYPE_ACK and the SNR 00336 const uint8_t ACK_LENGTH = 7; 00337 // added by C. Pham 00338 #ifdef W_NET_KEY 00339 const uint8_t NET_KEY_LENGTH=2; 00340 const uint8_t OFFSET_PAYLOADLENGTH = 4+NET_KEY_LENGTH; 00341 const uint8_t net_key_0 = 0x12; 00342 const uint8_t net_key_1 = 0x34; 00343 #else 00344 // modified by C. Pham to remove the retry field and the length field 00345 // which will be replaced by packet type field 00346 const uint8_t OFFSET_PAYLOADLENGTH = 4; 00347 #endif 00348 const uint8_t OFFSET_RSSI = 139; 00349 const uint8_t NOISE_FIGURE = 6.0; 00350 const uint8_t NOISE_ABSOLUTE_ZERO = 174.0; 00351 const uint16_t MAX_TIMEOUT = 1500; //10000 msec = 10.0 sec 00352 const uint16_t MAX_WAIT = 12000; //12000 msec = 12.0 sec 00353 const uint8_t MAX_RETRIES = 5; 00354 const uint8_t CORRECT_PACKET = 0; 00355 const uint8_t INCORRECT_PACKET = 1; 00356 00357 // added by C. Pham 00358 // Packet type definition 00359 00360 #define PKT_TYPE_MASK 0xF0 00361 #define PKT_FLAG_MASK 0x0F 00362 00363 #define PKT_TYPE_DATA 0x10 00364 #define PKT_TYPE_ACK 0x20 00365 00366 #define PKT_FLAG_ACK_REQ 0x08 00367 #define PKT_FLAG_DATA_ENCRYPTED 0x04 00368 #define PKT_FLAG_DATA_WAPPKEY 0x02 00369 #define PKT_FLAG_DATA_ISBINARY 0x01 00370 00371 #define SX1272_ERROR_ACK 3 00372 #define SX1272_ERROR_TOA 4 00373 00374 //! Structure : 00375 /*! 00376 */ 00377 struct pack 00378 { 00379 // added by C. Pham 00380 #ifdef W_NET_KEY 00381 uint8_t netkey[NET_KEY_LENGTH]; 00382 #endif 00383 //! Structure Variable : Packet destination 00384 /*! 00385 */ 00386 uint8_t dst; 00387 00388 // added by C. Pham 00389 //! Structure Variable : Packet type 00390 /*! 00391 */ 00392 uint8_t type; 00393 00394 //! Structure Variable : Packet source 00395 /*! 00396 */ 00397 uint8_t src; 00398 00399 //! Structure Variable : Packet number 00400 /*! 00401 */ 00402 uint8_t packnum; 00403 00404 // modified by C. Pham 00405 // will not be used in the transmitted packet 00406 //! Structure Variable : Packet length 00407 /*! 00408 */ 00409 uint8_t length; 00410 00411 //! Structure Variable : Packet payload 00412 /*! 00413 */ 00414 uint8_t data[MAX_PAYLOAD]; 00415 00416 // modified by C. Pham 00417 // will not be used in the transmitted packet 00418 //! Structure Variable : Retry number 00419 /*! 00420 */ 00421 uint8_t retry; 00422 }; 00423 00424 /****************************************************************************** 00425 * Class 00426 ******************************************************************************/ 00427 00428 //! SX1272 Class 00429 /*! 00430 SX1272 Class defines all the variables and functions used to manage 00431 SX1272 modules. 00432 */ 00433 class SX1272 00434 { 00435 00436 public: 00437 00438 //! class constructor 00439 /*! 00440 It does nothing 00441 \param void 00442 \return void 00443 */ 00444 SX1272(); 00445 00446 //! It puts the module ON 00447 /*! 00448 \param void 00449 \return uint8_t setLORA state 00450 */ 00451 uint8_t ON(); 00452 00453 //! It puts the module OFF 00454 /*! 00455 \param void 00456 \return void 00457 */ 00458 void OFF(); 00459 00460 //! It reads an internal module register. 00461 /*! 00462 \param byte address : address register to read from. 00463 \return the content of the register. 00464 */ 00465 byte readRegister(byte address); 00466 00467 //! It writes in an internal module register. 00468 /*! 00469 \param byte address : address register to write in. 00470 \param byte data : value to write in the register. 00471 */ 00472 void writeRegister(byte address, byte data); 00473 00474 //! It clears the interruption flags. 00475 /*! 00476 \param void 00477 \return void 00478 */ 00479 void clearFlags(); 00480 00481 //! It sets the LoRa mode on. 00482 /*! 00483 It stores in global '_LORA' variable '1' when success 00484 \return '0' on success, '1' otherwise 00485 */ 00486 uint8_t setLORA(); 00487 00488 //! It sets the FSK mode on. 00489 /*! 00490 It stores in global '_FSK' variable '1' when success 00491 \return '0' on success, '1' otherwise 00492 */ 00493 uint8_t setFSK(); 00494 00495 //! It gets the BW, SF and CR of the module. 00496 /*! 00497 It stores in global '_bandwidth' variable the BW 00498 It stores in global '_codingRate' variable the CR 00499 It stores in global '_spreadingFactor' variable the SF 00500 \return '0' on success, '1' otherwise 00501 */ 00502 uint8_t getMode(); 00503 00504 //! It sets the BW, SF and CR of the module. 00505 /*! 00506 It stores in global '_bandwidth' variable the BW 00507 It stores in global '_codingRate' variable the CR 00508 It stores in global '_spreadingFactor' variable the SF 00509 \param uint8_t mode : there is a mode number to different values of 00510 the configured parameters with this function. 00511 \return '0' on success, '1' otherwise 00512 */ 00513 int8_t setMode(uint8_t mode); 00514 00515 //! It gets the header mode configured. 00516 /*! 00517 It stores in global '_header' variable '0' when header is sent 00518 (explicit header mode) or '1' when is not sent (implicit header 00519 mode). 00520 \return '0' on success, '1' otherwise 00521 */ 00522 uint8_t getHeader(); 00523 00524 //! It sets explicit header mode. 00525 /*! 00526 It stores in global '_header' variable '1' when success 00527 \return '0' on success, '1' otherwise 00528 */ 00529 int8_t setHeaderON(); 00530 00531 //! It sets implicit header mode. 00532 /*! 00533 It stores in global '_header' variable '0' when success 00534 \return '0' on success, '1' otherwise 00535 */ 00536 int8_t setHeaderOFF(); 00537 00538 //! It gets the CRC configured. 00539 /*! 00540 It stores in global '_CRC' variable '1' enabling CRC generation on 00541 payload, or '0' disabling the CRC. 00542 \return '0' on success, '1' otherwise 00543 */ 00544 uint8_t getCRC(); 00545 00546 //! It sets CRC on. 00547 /*! 00548 It stores in global '_CRC' variable '1' when success 00549 \return '0' on success, '1' otherwise 00550 */ 00551 uint8_t setCRC_ON(); 00552 00553 //! It sets CRC off. 00554 /*! 00555 It stores in global '_CRC' variable '0' when success 00556 \return '0' on success, '1' otherwise 00557 */ 00558 uint8_t setCRC_OFF(); 00559 00560 //! It is true if the SF selected exists. 00561 /*! 00562 \param uint8_t spr : spreading factor value to check. 00563 \return 'true' on success, 'false' otherwise 00564 */ 00565 boolean isSF(uint8_t spr); 00566 00567 //! It gets the SF configured. 00568 /*! 00569 It stores in global '_spreadingFactor' variable the current value of SF 00570 \return '0' on success, '1' otherwise 00571 */ 00572 int8_t getSF(); 00573 00574 //! It sets the SF. 00575 /*! 00576 It stores in global '_spreadingFactor' variable the current value of SF 00577 \param uint8_t spr : spreading factor value to set in the configuration. 00578 \return '0' on success, '1' otherwise 00579 */ 00580 uint8_t setSF(uint8_t spr); 00581 00582 //! It is true if the BW selected exists. 00583 /*! 00584 \param uint16_t band : bandwidth value to check. 00585 \return 'true' on success, 'false' otherwise 00586 */ 00587 boolean isBW(uint16_t band); 00588 00589 //! It gets the BW configured. 00590 /*! 00591 It stores in global '_bandwidth' variable the BW selected 00592 in the configuration 00593 \return '0' on success, '1' otherwise 00594 */ 00595 int8_t getBW(); 00596 00597 //! It sets the BW. 00598 /*! 00599 It stores in global '_bandwidth' variable the BW selected 00600 in the configuration 00601 \param uint16_t band : bandwidth value to set in the configuration. 00602 \return '0' on success, '1' otherwise 00603 */ 00604 int8_t setBW(uint16_t band); 00605 00606 //! It is true if the CR selected exists. 00607 /*! 00608 \param uint8_t cod : the coding rate value to check. 00609 \return 'true' on success, 'false' otherwise 00610 */ 00611 boolean isCR(uint8_t cod); 00612 00613 //! It gets the CR configured. 00614 /*! 00615 It stores in global '_codingRate' variable the CR selected 00616 in the configuration 00617 \return '0' on success, '1' otherwise 00618 */ 00619 int8_t getCR(); 00620 00621 //! It sets the CR. 00622 /*! 00623 It stores in global '_codingRate' variable the CR selected 00624 in the configuration 00625 \param uint8_t cod : coding rate value to set in the configuration. 00626 \return '0' on success, '1' otherwise 00627 */ 00628 int8_t setCR(uint8_t cod); 00629 00630 00631 //! It is true if the channel selected exists. 00632 /*! 00633 \param uint32_t ch : frequency channel value to check. 00634 \return 'true' on success, 'false' otherwise 00635 */ 00636 boolean isChannel(uint32_t ch); 00637 00638 //! It gets frequency channel the module is using. 00639 /*! 00640 It stores in global '_channel' variable the frequency channel 00641 \return '0' on success, '1' otherwise 00642 */ 00643 uint8_t getChannel(); 00644 00645 //! It sets frequency channel the module is using. 00646 /*! 00647 It stores in global '_channel' variable the frequency channel 00648 \param uint32_t ch : frequency channel value to set in the configuration. 00649 \return '0' on success, '1' otherwise 00650 */ 00651 int8_t setChannel(uint32_t ch); 00652 00653 //! It gets the output power of the signal. 00654 /*! 00655 It stores in global '_power' variable the output power of the signal 00656 \return '0' on success, '1' otherwise 00657 */ 00658 uint8_t getPower(); 00659 00660 //! It sets the output power of the signal. 00661 /*! 00662 It stores in global '_power' variable the output power of the signal 00663 \param char p : 'M', 'H' or 'L' if you want Maximum, High or Low 00664 output power signal. 00665 \return '0' on success, '1' otherwise 00666 */ 00667 int8_t setPower(char p); 00668 00669 //! It sets the output power of the signal. 00670 /*! 00671 It stores in global '_power' variable the output power of the signal 00672 \param uint8_t pow : value to set as output power. 00673 \return '0' on success, '1' otherwise 00674 */ 00675 int8_t setPowerNum(uint8_t pow); 00676 00677 //! It gets the preamble length configured. 00678 /*! 00679 It stores in global '_preamblelength' variable the preamble length 00680 \return '0' on success, '1' otherwise 00681 */ 00682 uint8_t getPreambleLength(); 00683 00684 //! It sets the preamble length. 00685 /*! 00686 It stores in global '_preamblelength' variable the preamble length 00687 \param uint16_t l : preamble length to set in the configuration. 00688 \return '0' on success, '1' otherwise 00689 */ 00690 uint8_t setPreambleLength(uint16_t l); 00691 00692 //! It gets the payload length of the last packet to send/receive. 00693 /*! 00694 It stores in global '_payloadlength' variable the payload length of 00695 the last packet to send/receive. 00696 \return '0' on success, '1' otherwise 00697 */ 00698 uint8_t getPayloadLength(); 00699 00700 //! It sets the packet length to send/receive. 00701 /*! 00702 It stores in global '_payloadlength' variable the payload length of 00703 the last packet to send/receive. 00704 \return '0' on success, '1' otherwise 00705 */ 00706 int8_t setPacketLength(); 00707 00708 //! It sets the packet length to send/receive. 00709 /*! 00710 It stores in global '_payloadlength' variable the payload length of 00711 the last packet to send/receive. 00712 \param uint8_t l : payload length to set in the configuration. 00713 \return '0' on success, '1' otherwise 00714 */ 00715 int8_t setPacketLength(uint8_t l); 00716 00717 //! It gets the node address of the mote. 00718 /*! 00719 It stores in global '_nodeAddress' variable the node address 00720 \return '0' on success, '1' otherwise 00721 */ 00722 uint8_t getNodeAddress(); 00723 00724 //! It sets the node address of the mote. 00725 /*! 00726 It stores in global '_nodeAddress' variable the node address 00727 \param uint8_t addr : address value to set as node address. 00728 \return '0' on success, '1' otherwise 00729 */ 00730 int8_t setNodeAddress(uint8_t addr); 00731 00732 //! It gets the SNR of the latest received packet. 00733 /*! 00734 It stores in global '_SNR' variable the SNR 00735 \return '0' on success, '1' otherwise 00736 */ 00737 int8_t getSNR(); 00738 00739 //! It gets the current value of RSSI. 00740 /*! 00741 It stores in global '_RSSI' variable the current value of RSSI 00742 \return '0' on success, '1' otherwise 00743 */ 00744 uint8_t getRSSI(); 00745 00746 //! It gets the RSSI of the latest received packet. 00747 /*! 00748 It stores in global '_RSSIpacket' variable the RSSI of the latest 00749 packet received. 00750 \return '0' on success, '1' otherwise 00751 */ 00752 int16_t getRSSIpacket(); 00753 00754 //! It sets the total of retries when a packet is not correctly received. 00755 /*! 00756 It stores in global '_maxRetries' variable the number of retries. 00757 \param uint8_t ret : number of retries. 00758 \return '0' on success, '1' otherwise 00759 */ 00760 uint8_t setRetries(uint8_t ret); 00761 00762 //! It gets the maximum current supply by the module. 00763 /*! 00764 * 00765 \return '0' on success, '1' otherwise 00766 */ 00767 uint8_t getMaxCurrent(); 00768 00769 //! It sets the maximum current supply by the module. 00770 /*! 00771 It stores in global '_maxCurrent' variable the maximum current supply. 00772 \param uint8_t rate : maximum current supply. 00773 \return '0' on success, '1' otherwise 00774 */ 00775 int8_t setMaxCurrent(uint8_t rate); 00776 00777 //! It gets the content of the main configuration registers. 00778 /*! 00779 It stores in global '_bandwidth' variable the BW. 00780 It stores in global '_codingRate' variable the CR. 00781 It stores in global '_spreadingFactor' variable the SF. 00782 It stores in global '_power' variable the output power of the signal. 00783 It stores in global '_channel' variable the frequency channel. 00784 It stores in global '_CRC' variable '1' enabling CRC generation on 00785 payload, or '0' disabling the CRC. 00786 It stores in global '_header' variable '0' when header is sent 00787 (explicit header mode) or '1' when is not sent (implicit header 00788 mode). 00789 It stores in global '_preamblelength' variable the preamble length. 00790 It stores in global '_payloadlength' variable the payload length of 00791 the last packet to send/receive. 00792 It stores in global '_nodeAddress' variable the node address. 00793 It stores in global '_temp' variable the module temperature. 00794 \return '0' on success, '1' otherwise 00795 */ 00796 uint8_t getRegs(); 00797 00798 //! It sets the maximum number of bytes from a frame that fit in a packet structure. 00799 /*! 00800 It stores in global '_payloadlength' variable the maximum number of bytes. 00801 \param uint16_t length16 : total frame length. 00802 \return '0' on success, '1' otherwise 00803 */ 00804 uint8_t truncPayload(uint16_t length16); 00805 00806 //! It writes an ACK in FIFO to send it. 00807 /*! 00808 * 00809 \return '0' on success, '1' otherwise 00810 */ 00811 uint8_t setACK(); 00812 00813 //! It puts the module in reception mode. 00814 /*! 00815 * 00816 \return '0' on success, '1' otherwise 00817 */ 00818 uint8_t receive(); 00819 00820 //! It receives a packet before MAX_TIMEOUT. 00821 /*! 00822 * 00823 \return '0' on success, '1' otherwise 00824 */ 00825 uint8_t receivePacketMAXTimeout(); 00826 00827 //! It receives a packet before a timeout. 00828 /*! 00829 * 00830 \return '0' on success, '1' otherwise 00831 */ 00832 uint8_t receivePacketTimeout(); 00833 00834 //! It receives a packet before a timeout. 00835 /*! 00836 \param uint16_t wait : time to wait to receive something. 00837 \return '0' on success, '1' otherwise 00838 */ 00839 uint8_t receivePacketTimeout(uint16_t wait); 00840 00841 //! It receives a packet before MAX_TIMEOUT and reply with an ACK. 00842 /*! 00843 * 00844 \return '0' on success, '1' otherwise 00845 */ 00846 uint8_t receivePacketMAXTimeoutACK(); 00847 00848 //! It receives a packet before a timeout and reply with an ACK. 00849 /*! 00850 * 00851 \return '0' on success, '1' otherwise 00852 */ 00853 uint8_t receivePacketTimeoutACK(); 00854 00855 //! It receives a packet before a timeout and reply with an ACK. 00856 /*! 00857 \param uint16_t wait : time to wait to receive something. 00858 \return '0' on success, '1' otherwise 00859 */ 00860 uint8_t receivePacketTimeoutACK(uint16_t wait); 00861 00862 //! It puts the module in 'promiscuous' reception mode. 00863 /*! 00864 * 00865 \return '0' on success, '1' otherwise 00866 */ 00867 uint8_t receiveAll(); 00868 00869 //! It puts the module in 'promiscuous' reception mode with a timeout. 00870 /*! 00871 \param uint16_t wait : time to wait to receive something. 00872 \return '0' on success, '1' otherwise 00873 */ 00874 uint8_t receiveAll(uint16_t wait); 00875 00876 //! It checks if there is an available packet and its destination. 00877 /*! 00878 * 00879 \return 'true' on success, 'false' otherwise 00880 */ 00881 boolean availableData(); 00882 00883 //! It checks if there is an available packet and its destination before a timeout. 00884 /*! 00885 * 00886 \param uint16_t wait : time to wait while there is no a valid header received. 00887 \return 'true' on success, 'false' otherwise 00888 */ 00889 boolean availableData(uint16_t wait); 00890 00891 //! It writes a packet in FIFO in order to send it. 00892 /*! 00893 \param uint8_t dest : packet destination. 00894 \param char *payload : packet payload. 00895 \return '0' on success, '1' otherwise 00896 */ 00897 uint8_t setPacket(uint8_t dest, char *payload); 00898 00899 //! It writes a packet in FIFO in order to send it. 00900 /*! 00901 \param uint8_t dest : packet destination. 00902 \param uint8_t *payload: packet payload. 00903 \return '0' on success, '1' otherwise 00904 */ 00905 uint8_t setPacket(uint8_t dest, uint8_t *payload); 00906 00907 //! It reads a received packet from the FIFO, if it arrives before ending MAX_TIMEOUT time. 00908 /*! 00909 * 00910 \return '0' on success, '1' otherwise 00911 */ 00912 uint8_t getPacketMAXTimeout(); 00913 00914 //! It reads a received packet from the FIFO, if it arrives before ending '_sendTime' time. 00915 /*! 00916 * 00917 \return '0' on success, '1' otherwise 00918 */ 00919 int8_t getPacket(); 00920 00921 //! It receives and gets a packet from FIFO, if it arrives before ending 'wait' time. 00922 /*! 00923 * 00924 \param uint16_t wait : time to wait while there is no a complete packet received. 00925 \return '0' on success, '1' otherwise 00926 */ 00927 int8_t getPacket(uint16_t wait); 00928 00929 //! It sends the packet stored in FIFO before ending MAX_TIMEOUT. 00930 /*! 00931 * 00932 \return '0' on success, '1' otherwise 00933 */ 00934 uint8_t sendWithMAXTimeout(); 00935 00936 //! It sends the packet stored in FIFO before ending _sendTime time. 00937 /*! 00938 * 00939 \return '0' on success, '1' otherwise 00940 */ 00941 uint8_t sendWithTimeout(); 00942 00943 //! It tries to send the packet stored in FIFO before ending 'wait' time. 00944 /*! 00945 \param uint16_t wait : time to wait to send the packet. 00946 \return '0' on success, '1' otherwise 00947 */ 00948 uint8_t sendWithTimeout(uint16_t wait); 00949 00950 //! It tries to send the packet wich payload is a parameter before ending MAX_TIMEOUT. 00951 /*! 00952 \param uint8_t dest : packet destination. 00953 \param char *payload : packet payload. 00954 \return '0' on success, '1' otherwise 00955 */ 00956 uint8_t sendPacketMAXTimeout(uint8_t dest, char *payload); 00957 00958 //! It tries to send the packet wich payload is a parameter before ending MAX_TIMEOUT. 00959 /*! 00960 \param uint8_t dest : packet destination. 00961 \param uint8_t *payload : packet payload. 00962 \param uint16_t length : payload buffer length. 00963 \return '0' on success, '1' otherwise 00964 */ 00965 uint8_t sendPacketMAXTimeout(uint8_t dest, uint8_t *payload, uint16_t length); 00966 00967 00968 //! It sends the packet wich payload is a parameter before ending MAX_TIMEOUT. 00969 /*! 00970 \param uint8_t dest : packet destination. 00971 \param char *payload : packet payload. 00972 \return '0' on success, '1' otherwise 00973 */ 00974 uint8_t sendPacketTimeout(uint8_t dest, char *payload); 00975 00976 //! It sends the packet wich payload is a parameter before ending MAX_TIMEOUT. 00977 /*! 00978 \param uint8_t dest : packet destination. 00979 \param uint8_t *payload: packet payload. 00980 \param uint16_t length : payload buffer length. 00981 \return '0' on success, '1' otherwise 00982 */ 00983 uint8_t sendPacketTimeout(uint8_t dest, uint8_t *payload, uint16_t length); 00984 00985 //! It sends the packet wich payload is a parameter before ending 'wait' time. 00986 /*! 00987 \param uint8_t dest : packet destination. 00988 \param char *payload : packet payload. 00989 \param uint16_t wait : time to wait. 00990 \return '0' on success, '1' otherwise 00991 */ 00992 uint8_t sendPacketTimeout(uint8_t dest, char *payload, uint16_t wait); 00993 00994 //! It sends the packet wich payload is a parameter before ending 'wait' time. 00995 /*! 00996 \param uint8_t dest : packet destination. 00997 \param uint8_t *payload : packet payload. 00998 \param uint16_t length : payload buffer length. 00999 \param uint16_t wait : time to wait. 01000 \return '0' on success, '1' otherwise 01001 */ 01002 uint8_t sendPacketTimeout(uint8_t dest, uint8_t *payload, uint16_t length, uint16_t wait); 01003 01004 //! It sends the packet wich payload is a parameter before MAX_TIMEOUT, and replies with ACK. 01005 /*! 01006 \param uint8_t dest : packet destination. 01007 \param char *payload : packet payload. 01008 \return '0' on success, '1' otherwise 01009 */ 01010 uint8_t sendPacketMAXTimeoutACK(uint8_t dest, char *payload); 01011 01012 //! It sends the packet wich payload is a parameter before MAX_TIMEOUT, and replies with ACK. 01013 /*! 01014 \param uint8_t dest : packet destination. 01015 \param uint8_t payload: packet payload. 01016 \param uint16_t length : payload buffer length. 01017 \return '0' on success, '1' otherwise 01018 */ 01019 uint8_t sendPacketMAXTimeoutACK(uint8_t dest, uint8_t *payload, uint16_t length); 01020 01021 //! It sends the packet wich payload is a parameter before a timeout, and replies with ACK. 01022 /*! 01023 \param uint8_t dest : packet destination. 01024 \param char *payload : packet payload. 01025 \return '0' on success, '1' otherwise 01026 */ 01027 uint8_t sendPacketTimeoutACK(uint8_t dest, char *payload); 01028 01029 //! It sends the packet wich payload is a parameter before a timeout, and replies with ACK. 01030 /*! 01031 \param uint8_t dest : packet destination. 01032 \param uint8_t payload: packet payload. 01033 \param uint16_t length : payload buffer length. 01034 \return '0' on success, '1' otherwise 01035 */ 01036 uint8_t sendPacketTimeoutACK(uint8_t dest, uint8_t *payload, uint16_t length); 01037 01038 //! It sends the packet wich payload is a parameter before 'wait' time, and replies with ACK. 01039 /*! 01040 \param uint8_t dest : packet destination. 01041 \param char *payload : packet payload. 01042 \param uint16_t wait : time to wait to send the packet. 01043 \return '0' on success, '1' otherwise 01044 */ 01045 uint8_t sendPacketTimeoutACK(uint8_t dest, char *payload, uint16_t wait); 01046 01047 //! It sends the packet wich payload is a parameter before 'wait' time, and replies with ACK. 01048 /*! 01049 \param uint8_t dest : packet destination. 01050 \param uint8_t payload: packet payload. 01051 \param uint16_t length : payload buffer length. 01052 \param uint16_t wait : time to wait to send the packet. 01053 \return '0' on success, '1' otherwise 01054 */ 01055 uint8_t sendPacketTimeoutACK(uint8_t dest, uint8_t *payload, uint16_t length, uint16_t wait); 01056 01057 //! It sets the destination of a packet. 01058 /*! 01059 \param uint8_t dest : value to set as destination address. 01060 \return '0' on success, '1' otherwise 01061 */ 01062 int8_t setDestination(uint8_t dest); 01063 01064 //! It sets the waiting time to send a packet. 01065 /*! 01066 It stores in global '_sendTime' variable the time for each mode. 01067 \return '0' on success, '1' otherwise 01068 */ 01069 uint8_t setTimeout(); 01070 01071 //! It sets the payload of the packet that is going to be sent. 01072 /*! 01073 \param char *payload : packet payload. 01074 \return '0' on success, '1' otherwise 01075 */ 01076 uint8_t setPayload(char *payload); 01077 01078 //! It sets the payload of the packet that is going to be sent. 01079 /*! 01080 \param uint8_t payload: packet payload. 01081 \return '0' on success, '1' otherwise 01082 */ 01083 uint8_t setPayload(uint8_t *payload); 01084 01085 //! If an ACK is received, it gets it and checks its content. 01086 /*! 01087 * 01088 \return '0' on success, '1' otherwise 01089 */ 01090 uint8_t getACK(); 01091 01092 //! It receives and gets an ACK from FIFO, if it arrives before ending 'wait' time. 01093 /*! 01094 * 01095 \param uint16_t wait : time to wait while there is no an ACK received. 01096 \return '0' on success, '1' otherwise 01097 */ 01098 uint8_t getACK(uint16_t wait); 01099 01100 //! It sends a packet, waits to receive an ACK and updates the _retries value, before ending MAX_TIMEOUT time. 01101 /*! 01102 \param uint8_t dest : packet destination. 01103 \param char *payload : packet payload. 01104 \return '0' on success, '1' otherwise 01105 */ 01106 uint8_t sendPacketMAXTimeoutACKRetries(uint8_t dest, char *payload); 01107 01108 //! It sends a packet, waits to receive an ACK and updates the _retries value, before ending MAX_TIMEOUT time. 01109 /*! 01110 \param uint8_t dest : packet destination. 01111 \param uint8_t *payload : packet payload. 01112 \param uint16_t length : payload buffer length. 01113 \return '0' on success, '1' otherwise 01114 */ 01115 uint8_t sendPacketMAXTimeoutACKRetries(uint8_t dest, uint8_t *payload, uint16_t length); 01116 01117 //! It sends a packet, waits to receive an ACK and updates the _retries value. 01118 /*! 01119 \param uint8_t dest : packet destination. 01120 \param char *payload : packet payload. 01121 \return '0' on success, '1' otherwise 01122 */ 01123 uint8_t sendPacketTimeoutACKRetries(uint8_t dest, char *payload); 01124 01125 //! It sends a packet, waits to receive an ACK and updates the _retries value. 01126 /*! 01127 \param uint8_t dest : packet destination. 01128 \param uint8_t *payload : packet payload. 01129 \param uint16_t length : payload buffer length. 01130 \return '0' on success, '1' otherwise 01131 */ 01132 uint8_t sendPacketTimeoutACKRetries(uint8_t dest, uint8_t *payload, uint16_t length); 01133 01134 //! It sends a packet, waits to receive an ACK and updates the _retries value, before ending 'wait' time. 01135 /*! 01136 \param uint8_t dest : packet destination. 01137 \param char *payload : packet payload. 01138 \param uint16_t wait : time to wait while trying to send the packet. 01139 \return '0' on success, '1' otherwise 01140 */ 01141 uint8_t sendPacketTimeoutACKRetries(uint8_t dest, char *payload, uint16_t wait); 01142 01143 //! It sends a packet, waits to receive an ACK and updates the _retries value, before ending 'wait' time. 01144 /*! 01145 \param uint8_t dest : packet destination. 01146 \param uint8_t *payload : packet payload. 01147 \param uint16_t length : payload buffer length. 01148 \param uint16_t wait : time to wait while trying to send the packet. 01149 \return '0' on success, '1' otherwise 01150 */ 01151 uint8_t sendPacketTimeoutACKRetries(uint8_t dest, uint8_t *payload, uint16_t length, uint16_t wait); 01152 01153 //! It gets the internal temperature of the module. 01154 /*! 01155 It stores in global '_temp' variable the module temperature. 01156 \return '0' on success, '1' otherwise 01157 */ 01158 uint8_t getTemp(); 01159 01160 // added by C. Pham 01161 void setPacketType(uint8_t type); 01162 void RxChainCalibration (); 01163 uint8_t doCAD(uint8_t counter); 01164 uint16_t getToA(uint8_t pl); 01165 void CarrierSense(); 01166 int8_t setSyncWord(uint8_t sw); 01167 int8_t getSyncWord(); 01168 int8_t setSleepMode(); 01169 int8_t setPowerDBM(uint8_t dbm); 01170 long limitToA(); 01171 long getRemainingToA(); 01172 long removeToA(uint16_t toa); 01173 01174 // SX1272 or SX1276? 01175 uint8_t _board; 01176 uint8_t _syncWord; 01177 uint8_t _defaultSyncWord; 01178 unsigned long _starttime; 01179 unsigned long _stoptime; 01180 unsigned long _startDoCad; 01181 unsigned long _endDoCad; 01182 uint8_t _loraMode; 01183 uint8_t _send_cad_number; 01184 bool _extendedIFS; 01185 bool _RSSIonSend; 01186 bool _enableCarrierSense; 01187 bool _rawFormat; 01188 int8_t _rcv_snr_in_ack; 01189 bool _needPABOOST; 01190 uint8_t _rawSNR; 01191 01192 #ifdef W_REQUESTED_ACK 01193 uint8_t _requestACK; 01194 uint8_t _requestACK_indicator; 01195 #endif 01196 01197 #ifdef W_NET_KEY 01198 uint8_t _my_netkey[NET_KEY_LENGTH]; 01199 uint8_t _the_net_key_0; 01200 uint8_t _the_net_key_1; 01201 #endif 01202 // end 01203 01204 /// Variables ///////////////////////////////////////////////////////////// 01205 01206 //! Variable : bandwidth configured in LoRa mode. 01207 //! bandwidth = 00 --> BW = 125KHz 01208 //! bandwidth = 01 --> BW = 250KHz 01209 //! bandwidth = 10 --> BW = 500KHz 01210 /*! 01211 */ 01212 uint8_t _bandwidth; 01213 01214 //! Variable : coding rate configured in LoRa mode. 01215 //! codingRate = 001 --> CR = 4/5 01216 //! codingRate = 010 --> CR = 4/6 01217 //! codingRate = 011 --> CR = 4/7 01218 //! codingRate = 100 --> CR = 4/8 01219 /*! 01220 */ 01221 uint8_t _codingRate; 01222 01223 //! Variable : spreading factor configured in LoRa mode. 01224 //! spreadingFactor = 6 --> SF = 6, 64 chips/symbol 01225 //! spreadingFactor = 7 --> SF = 7, 128 chips/symbol 01226 //! spreadingFactor = 8 --> SF = 8, 256 chips/symbol 01227 //! spreadingFactor = 9 --> SF = 9, 512 chips/symbol 01228 //! spreadingFactor = 10 --> SF = 10, 1024 chips/symbol 01229 //! spreadingFactor = 11 --> SF = 11, 2048 chips/symbol 01230 //! spreadingFactor = 12 --> SF = 12, 4096 chips/symbol 01231 /*! 01232 */ 01233 uint8_t _spreadingFactor; 01234 01235 //! Variable : frequency channel. 01236 //! channel = 0xD84CCC --> CH = 10_868, 865.20MHz 01237 //! channel = 0xD86000 --> CH = 11_868, 865.50MHz 01238 //! channel = 0xD87333 --> CH = 12_868, 865.80MHz 01239 //! channel = 0xD88666 --> CH = 13_868, 866.10MHz 01240 //! channel = 0xD89999 --> CH = 14_868, 866.40MHz 01241 //! channel = 0xD8ACCC --> CH = 15_868, 866.70MHz 01242 //! channel = 0xD8C000 --> CH = 16_868, 867.00MHz 01243 //! channel = 0xE1C51E --> CH = 00_900, 903.08MHz 01244 //! channel = 0xE24F5C --> CH = 01_900, 905.24MHz 01245 //! channel = 0xE2D999 --> CH = 02_900, 907.40MHz 01246 //! channel = 0xE363D7 --> CH = 03_900, 909.56MHz 01247 //! channel = 0xE3EE14 --> CH = 04_900, 911.72MHz 01248 //! channel = 0xE47851 --> CH = 05_900, 913.88MHz 01249 //! channel = 0xE5028F --> CH = 06_900, 916.04MHz 01250 //! channel = 0xE58CCC --> CH = 07_900, 918.20MHz 01251 //! channel = 0xE6170A --> CH = 08_900, 920.36MHz 01252 //! channel = 0xE6A147 --> CH = 09_900, 922.52MHz 01253 //! channel = 0xE72B85 --> CH = 10_900, 924.68MHz 01254 //! channel = 0xE7B5C2 --> CH = 11_900, 926.84MHz 01255 /*! 01256 */ 01257 uint32_t _channel; 01258 01259 //! Variable : output power. 01260 //! 01261 /*! 01262 */ 01263 uint8_t _power; 01264 01265 //! Variable : SNR from the last packet received in LoRa mode. 01266 //! 01267 /*! 01268 */ 01269 int8_t _SNR; 01270 01271 //! Variable : RSSI current value. 01272 //! 01273 /*! 01274 */ 01275 int8_t _RSSI; 01276 01277 //! Variable : RSSI from the last packet received in LoRa mode. 01278 //! 01279 /*! 01280 */ 01281 int16_t _RSSIpacket; 01282 01283 //! Variable : preamble length sent/received. 01284 //! 01285 /*! 01286 */ 01287 uint16_t _preamblelength; 01288 01289 //! Variable : payload length sent/received. 01290 //! 01291 /*! 01292 */ 01293 uint16_t _payloadlength; 01294 01295 //! Variable : node address. 01296 //! 01297 /*! 01298 */ 01299 uint8_t _nodeAddress; 01300 01301 //! Variable : implicit or explicit header in LoRa mode. 01302 //! 01303 /*! 01304 */ 01305 uint8_t _header; 01306 01307 //! Variable : header received while waiting a packet to arrive. 01308 //! 01309 /*! 01310 */ 01311 uint8_t _hreceived; 01312 01313 //! Variable : presence or absence of CRC calculation. 01314 //! 01315 /*! 01316 */ 01317 uint8_t _CRC; 01318 01319 //! Variable : packet destination. 01320 //! 01321 /*! 01322 */ 01323 uint8_t _destination; 01324 01325 //! Variable : packet number. 01326 //! 01327 /*! 01328 */ 01329 uint8_t _packetNumber; 01330 01331 //! Variable : indicates if received packet is correct or incorrect. 01332 //! 01333 /*! 01334 */ 01335 uint8_t _reception; 01336 01337 //! Variable : number of current retry. 01338 //! 01339 /*! 01340 */ 01341 uint8_t _retries; 01342 01343 //! Variable : maximum number of retries. 01344 //! 01345 /*! 01346 */ 01347 uint8_t _maxRetries; 01348 01349 //! Variable : maximum current supply. 01350 //! 01351 /*! 01352 */ 01353 uint8_t _maxCurrent; 01354 01355 //! Variable : indicates FSK or LoRa 'modem'. 01356 //! 01357 /*! 01358 */ 01359 uint8_t _modem; 01360 01361 //! Variable : array with all the information about a sent packet. 01362 //! 01363 /*! 01364 */ 01365 pack packet_sent; 01366 01367 //! Variable : array with all the information about a received packet. 01368 //! 01369 /*! 01370 */ 01371 pack packet_received; 01372 01373 //! Variable : array with all the information about a sent/received ack. 01374 //! 01375 /*! 01376 */ 01377 pack ACK; 01378 01379 //! Variable : temperature module. 01380 //! 01381 /*! 01382 */ 01383 int _temp; 01384 01385 //! Variable : current timeout to send a packet. 01386 //! 01387 /*! 01388 */ 01389 uint16_t _sendTime; 01390 01391 // added by C. Pham for ToA management 01392 // 01393 private: 01394 01395 bool _limitToA; 01396 long _remainingToA; 01397 unsigned long _startToAcycle; 01398 unsigned long _endToAcycle; 01399 uint16_t _currentToA; 01400 }; 01401 01402 extern SX1272 sx1272; 01403 01404 #endif
Generated on Fri Jul 15 2022 12:44:35 by
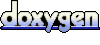