PingPong-ClickButtonToWork
Dependents: Lora_SX1272_Coragem-dev-shared Lora_SX1272_serial
SX1272.cpp
00001 /* 00002 * Library for LoRa 868 / 915MHz SX1272 LoRa module 00003 * 00004 * Copyright (C) Libelium Comunicaciones Distribuidas S.L. 00005 * http://www.libelium.com 00006 * 00007 * This program is free software: you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation, either version 3 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * This program is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program. If not, see http://www.gnu.org/licenses/. 00019 * 00020 * Version: 1.1 00021 * Design: David Gascón 00022 * Implementation: Covadonga Albiñana & Victor Boria 00023 */ 00024 00025 //**********************************************************************/ 00026 // Includes 00027 //**********************************************************************/ 00028 #include "mbed.h" 00029 #include "SX1272.h" 00030 #include <SPI.h> 00031 00032 /* CHANGE LOGS by C. Pham 00033 * June, 22th, 2017 00034 * - setPowerDBM(uint8_t dbm) calls setPower('X') when dbm is set to 20 00035 * Apr, 21th, 2017 00036 * - change the way timeout are detected: exitTime=millis()+(unsigned long)wait; then millis() < exitTime; 00037 * Mar, 26th, 2017 00038 * - insert delay(100) before setting radio module to sleep mode. Remove unstability issue 00039 * - (proposed by escyes - https://github.com/CongducPham/LowCostLoRaGw/issues/53#issuecomment-289237532) 00040 * Jan, 11th, 2017 00041 * - fix bug in getRSSIpacket() when SNR < 0 thanks to John Rohde from Aarhus University 00042 * Dec, 17th, 2016 00043 * - fix bug making -DPABOOST in radio.makefile inoperant 00044 * Dec, 1st, 2016 00045 * - add RSSI computation while performing CAD with doCAD() 00046 * - WARNING: the SX1272 lib for gateway (Raspberry) does not have this functionality 00047 * Nov, 26th, 2016 00048 * - add preliminary support for ToA limitation 00049 * - when in "production" mode, uncomment #define LIMIT_TOA 00050 * Nov, 16th, 2016 00051 * - provide better power management mechanisms 00052 * - manage PA_BOOST and dBm setting 00053 * Jan, 23rd, 2016 00054 * - the packet format at transmission does not use the original Libelium format anymore 00055 * * the retry field is removed therefore all operations using retry will probably not work well, not tested though 00056 * - therefore DO NOT use sendPacketTimeoutACKRetries() 00057 * - the reason is that we do not want to have a reserved byte after the payload 00058 * * the length field is removed because it is much better to get the packet length at the reception side 00059 * * after the dst field, we inserted a packet type field to better identify the packet type: DATA, ACK, encryption, app key,... 00060 * - the format is now dst(1B) ptype(1B) src(1B) seq(1B) payload(xB) 00061 * - ptype is decomposed in 2 parts type(4bits) flags(4bits) 00062 * - type can take current value of DATA=0001 and ACK=0010 00063 * - the flags are from left to right: ack_requested|encrypted|with_appkey|is_binary 00064 * - ptype can be set with setPacketType(), see constant defined in SX1272.h 00065 * - the header length is then 4 instead of 5 00066 * Jan, 16th, 2016 00067 * - add support for SX1276, automatic detect 00068 * - add LF/HF calibaration copied from LoRaMAC-Node. Don't know if it is really necessary though 00069 * - change various radio settings 00070 * Dec, 10th, 2015 00071 * - add SyncWord for test with simple LoRaWAN 00072 * - add mode 11 that have BW=125, CR=4/5, SF=7 on channel 868.1MHz 00073 * - use following in your code if (loraMode==11) { e = sx1272.setChannel(CH_18_868); } 00074 * Nov, 13th, 2015 00075 * - add CarrierSense() to perform some Listen Before Talk procedure 00076 * - add dynamic ACK suport 00077 * - compile with W_REQUESTED_ACK, retry field is used to indicate at the receiver 00078 * that an ACK should be sent 00079 * - receiveWithTimeout() has been modified to send an ACK if retry is 1 00080 * - at sender side, sendPacketTimeoutACK() has been modified to indicate 00081 * whether retry should be set to 1 or not in setPacket() 00082 * - receiver should always use receiveWithTimeout() while sender decides to use 00083 * sendPacketTimeout() or sendPacketTimeoutACK() 00084 * Jun, 2015 00085 * - Add time on air computation and CAD features 00086 */ 00087 00088 // Added by C. Pham 00089 // based on SIFS=3CAD 00090 uint8_t sx1272_SIFS_value[11]={0, 183, 94, 44, 47, 23, 24, 12, 12, 7, 4}; 00091 uint8_t sx1272_CAD_value[11]={0, 62, 31, 16, 16, 8, 9, 5, 3, 1, 1}; 00092 00093 //#define LIMIT_TOA 00094 // 0.1% for testing 00095 //#define MAX_DUTY_CYCLE_PER_HOUR 3600L 00096 // 1%, regular mode 00097 #define MAX_DUTY_CYCLE_PER_HOUR 36000L 00098 // normally 1 hour, set to smaller value for testing 00099 #define DUTYCYCLE_DURATION 3600000L 00100 // 4 min for testing 00101 //#define DUTYCYCLE_DURATION 240000L 00102 00103 // end 00104 00105 //ajoute par C.DUPATY 00106 //Serial pc(USBTX, USBRX); // tx, rx 00107 // config pour SX1272MB2xAS sur NUCLEO-L073RZ 00108 00109 00110 #define SX1272_debug_mode 0 00111 00112 00113 //Coragem pins 00114 SPI spi(P0_4,P0_6,P0_8);; // PA_7, PA_6, PA_5 00115 DigitalOut ss(P0_26); //(PB_6) 00116 DigitalOut rst(P1_15); 00117 00118 00119 //**********************************************************************/ 00120 // Public functions. 00121 //**********************************************************************/ 00122 00123 SX1272::SX1272() 00124 { 00125 //ajoute par C.DUPATY 00126 us_ticker_init(); 00127 srand(time(NULL)); 00128 // ajoute parC.Dupaty 00129 spi.format(8,0); // spi 8 bits mode 0 00130 spi.frequency(2000000); // spi clock 200KHz a l origine 00131 00132 // Initialize class variables 00133 _bandwidth = BW_125; 00134 _codingRate = CR_5; 00135 _spreadingFactor = SF_7; 00136 _channel = CH_07_900; 00137 _header = HEADER_ON; 00138 _CRC = CRC_OFF; 00139 _modem = FSK; 00140 _power = 15; 00141 _packetNumber = 0; 00142 _reception = CORRECT_PACKET; 00143 _retries = 0; 00144 // added by C. Pham 00145 _defaultSyncWord=0x12; 00146 _rawFormat=true;//____________________________________________________ 00147 _extendedIFS=true; 00148 _RSSIonSend=true; 00149 // disabled by default 00150 _enableCarrierSense=false; 00151 // DIFS by default 00152 _send_cad_number=9; 00153 #ifdef PABOOST 00154 _needPABOOST=true; 00155 #else 00156 _needPABOOST=false; 00157 #endif 00158 _limitToA=false; 00159 _startToAcycle=millis(); 00160 _remainingToA=MAX_DUTY_CYCLE_PER_HOUR; 00161 _endToAcycle=_startToAcycle+DUTYCYCLE_DURATION; 00162 #ifdef W_REQUESTED_ACK 00163 _requestACK = 0; 00164 #endif 00165 #ifdef W_NET_KEY 00166 _my_netkey[0] = net_key_0; 00167 _my_netkey[1] = net_key_1; 00168 #endif 00169 // end 00170 _maxRetries = 3; 00171 packet_sent.retry = _retries; 00172 }; 00173 00174 // added by C. Pham 00175 // copied from LoRaMAC-Node 00176 /*! 00177 * Performs the Rx chain calibration for LF and HF bands 00178 * \remark Must be called just after the reset so all registers are at their 00179 * default values 00180 */ 00181 void SX1272::RxChainCalibration () 00182 { 00183 if (_board==SX1276Chip) { 00184 00185 printf("SX1276 LF/HF calibration\r"); 00186 00187 // Cut the PA just in case, RFO output, power = -1 dBm 00188 writeRegister( REG_PA_CONFIG, 0x00 ); 00189 00190 // Launch Rx chain calibration for LF band 00191 writeRegister( REG_IMAGE_CAL, ( readRegister( REG_IMAGE_CAL ) & RF_IMAGECAL_IMAGECAL_MASK ) | RF_IMAGECAL_IMAGECAL_START ); 00192 while( ( readRegister( REG_IMAGE_CAL ) & RF_IMAGECAL_IMAGECAL_RUNNING ) == RF_IMAGECAL_IMAGECAL_RUNNING ) 00193 { 00194 } 00195 00196 // Sets a Frequency in HF band 00197 setChannel(CH_07_900); 00198 00199 // Launch Rx chain calibration for HF band 00200 writeRegister( REG_IMAGE_CAL, ( readRegister( REG_IMAGE_CAL ) & RF_IMAGECAL_IMAGECAL_MASK ) | RF_IMAGECAL_IMAGECAL_START ); 00201 while( ( readRegister( REG_IMAGE_CAL ) & RF_IMAGECAL_IMAGECAL_RUNNING ) == RF_IMAGECAL_IMAGECAL_RUNNING ) 00202 { 00203 } 00204 } 00205 } 00206 00207 00208 /* 00209 Function: Sets the module ON. 00210 Returns: uint8_t setLORA state 00211 */ 00212 //uint8_t SX1272::Read() 00213 //readRegister( 00214 uint8_t SX1272::ON() 00215 { 00216 uint8_t state = 2; 00217 00218 #if (SX1272_debug_mode > 1) 00219 printf("\n"); 00220 printf("Starting 'ON'\n"); 00221 #endif 00222 00223 00224 // Powering the module 00225 // pinMode(SX1272_SS,OUTPUT); 00226 // digitalWrite(SX1272_SS,HIGH); 00227 ss=1; 00228 wait_ms(100); 00229 00230 //#define USE_SPI_SETTINGS 00231 /* 00232 #ifdef USE_SPI_SETTINGS 00233 SPI.beginTransaction(SPISettings(2000000, MSBFIRST, SPI_MODE0)); 00234 #else 00235 //Configure the MISO, MOSI, CS, SPCR. 00236 SPI.begin(); 00237 //Set Most significant bit first 00238 SPI.setBitOrder(MSBFIRST); 00239 #ifdef _VARIANT_ARDUINO_DUE_X_ 00240 // for the DUE, set to 4MHz 00241 SPI.setClockDivider(42); 00242 #else 00243 // for the MEGA, set to 2MHz 00244 SPI.setClockDivider(SPI_CLOCK_DIV8); 00245 #endif 00246 //Set data mode 00247 SPI.setDataMode(SPI_MODE0); 00248 #endif 00249 */ 00250 wait_ms(100); 00251 00252 /* // added by C. Pham 00253 pinMode(SX1272_RST,OUTPUT); 00254 digitalWrite(SX1272_RST,HIGH); 00255 wait_ms(100); 00256 digitalWrite(SX1272_RST,LOW); 00257 wait_ms(100); 00258 */ 00259 00260 rst=1; 00261 wait_ms(100); 00262 rst=0; 00263 00264 // from single_chan_pkt_fwd by Thomas Telkamp 00265 00266 //__________________________lendo todos os registradores________________________ 00267 // for(char i=0;i<100;i++){ 00268 // int value =readRegister(i); 00269 //// printf("reg%d =%d ",i,value); 00270 // } 00271 readRegister(0);//evitar falsos positivos 00272 uint8_t version = readRegister(REG_VERSION); 00273 00274 if (version == 0x22) { //sx1272 ? 00275 // sx1272 00276 printf("\nSX1272 detected, starting\n"); 00277 _board = SX1272Chip; 00278 } 00279 else if (version ==0x12) {// sx1276? 00280 printf("SX1276 detected, starting\n"); 00281 _board = SX1276Chip; 00282 } 00283 else { 00284 // rst=0; 00285 // wait_ms(100); 00286 // rst=1; 00287 // wait_ms(100); 00288 00289 while(1){ 00290 printf("Unrecognized transceiver\n"); 00291 wait_ms(2000); 00292 } 00293 } 00294 00295 00296 // added by C. Pham 00297 RxChainCalibration (); 00298 00299 setMaxCurrent(0x1B); 00300 #if (SX1272_debug_mode > 1) 00301 printf("## Setting ON with maximum current supply ##"); 00302 printf("\n"); 00303 #endif 00304 00305 // set LoRa mode 00306 state = setLORA(); 00307 00308 // Added by C. Pham for ToA computation 00309 getPreambleLength(); 00310 #ifdef W_NET_KEY 00311 //#if (SX1272_debug_mode > 1) 00312 printf("## SX1272 layer has net key##"); 00313 //#endif 00314 #endif 00315 00316 #ifdef W_INITIALIZATION 00317 // CAUTION 00318 // doing initialization as proposed by Libelium seems not to work for the SX1276 00319 // so we decided to leave the default value of the SX127x, then configure the radio when 00320 // setting to LoRa mode 00321 00322 //Set initialization values 00323 writeRegister(0x0,0x0); 00324 // comment by C. Pham 00325 // still valid for SX1276 00326 writeRegister(0x1,0x81); 00327 // end 00328 writeRegister(0x2,0x1A); 00329 writeRegister(0x3,0xB); 00330 writeRegister(0x4,0x0); 00331 writeRegister(0x5,0x52); 00332 writeRegister(0x6,0xD8); 00333 writeRegister(0x7,0x99); 00334 writeRegister(0x8,0x99); 00335 // modified by C. Pham 00336 // added by C. Pham 00337 if (_board==SX1272Chip) 00338 // RFIO_pin RFU OutputPower 00339 // 0 000 0000 00340 writeRegister(0x9,0x8F);// was 0, 8F gives max outputpower 00341 else 00342 // RFO_pin MaxP OutputPower 00343 // 0 100 1111 00344 // set MaxPower to 0x4 and OutputPower to 0 00345 writeRegister(0x9,0x40); 00346 00347 writeRegister(0xA,0x9); 00348 writeRegister(0xB,0x3B); 00349 00350 // comment by C. Pham 00351 // still valid for SX1276 00352 writeRegister(0xC,0x23); 00353 00354 // REG_RX_CONFIG 00355 writeRegister(0xD,0x1); 00356 00357 writeRegister(0xE,0x80); 00358 writeRegister(0xF,0x0); 00359 writeRegister(0x10,0x0); 00360 writeRegister(0x11,0x0); 00361 writeRegister(0x12,0x0); 00362 writeRegister(0x13,0x0); 00363 writeRegister(0x14,0x0); 00364 writeRegister(0x15,0x0); 00365 writeRegister(0x16,0x0); 00366 writeRegister(0x17,0x0); 00367 writeRegister(0x18,0x10); 00368 writeRegister(0x19,0x0); 00369 writeRegister(0x1A,0x0); 00370 writeRegister(0x1B,0x0); 00371 writeRegister(0x1C,0x0); 00372 00373 // added by C. Pham 00374 if (_board==SX1272Chip) { 00375 // comment by C. Pham 00376 // 0x4A = 01 001 0 1 0 00377 // BW=250 CR=4/5 ImplicitH_off RxPayloadCrcOn_on LowDataRateOptimize_off 00378 writeRegister(0x1D,0x4A); 00379 // 1001 0 1 11 00380 // SF=9 TxContinuous_off AgcAutoOn SymbTimeOut 00381 writeRegister(0x1E,0x97); 00382 } 00383 else { 00384 // 1000 001 0 00385 // BW=250 CR=4/5 ImplicitH_off 00386 writeRegister(0x1D,0x82); 00387 // 1001 0 1 11 00388 // SF=9 TxContinuous_off RxPayloadCrcOn_on SymbTimeOut 00389 writeRegister(0x1E,0x97); 00390 } 00391 // end 00392 00393 writeRegister(0x1F,0xFF); 00394 writeRegister(0x20,0x0); 00395 writeRegister(0x21,0x8); 00396 writeRegister(0x22,0xFF); 00397 writeRegister(0x23,0xFF); 00398 writeRegister(0x24,0x0); 00399 writeRegister(0x25,0x0); 00400 00401 // added by C. Pham 00402 if (_board==SX1272Chip) 00403 writeRegister(0x26,0x0); 00404 else 00405 // 0000 0 1 00 00406 // reserved LowDataRateOptimize_off AgcAutoOn reserved 00407 writeRegister(0x26,0x04); 00408 00409 // REG_SYNC_CONFIG 00410 writeRegister(0x27,0x0); 00411 00412 writeRegister(0x28,0x0); 00413 writeRegister(0x29,0x0); 00414 writeRegister(0x2A,0x0); 00415 writeRegister(0x2B,0x0); 00416 writeRegister(0x2C,0x0); 00417 writeRegister(0x2D,0x50); 00418 writeRegister(0x2E,0x14); 00419 writeRegister(0x2F,0x40); 00420 writeRegister(0x30,0x0); 00421 writeRegister(0x31,0x3); 00422 writeRegister(0x32,0x5); 00423 writeRegister(0x33,0x27); 00424 writeRegister(0x34,0x1C); 00425 writeRegister(0x35,0xA); 00426 writeRegister(0x36,0x0); 00427 writeRegister(0x37,0xA); 00428 writeRegister(0x38,0x42); 00429 writeRegister(0x39,0x12); 00430 //writeRegister(0x3A,0x65); 00431 //writeRegister(0x3B,0x1D); 00432 //writeRegister(0x3C,0x1); 00433 //writeRegister(0x3D,0xA1); 00434 //writeRegister(0x3E,0x0); 00435 //writeRegister(0x3F,0x0); 00436 //writeRegister(0x40,0x0); 00437 //writeRegister(0x41,0x0); 00438 // commented by C. Pham 00439 // since now we handle also the SX1276 00440 //writeRegister(0x42,0x22); 00441 #endif 00442 // added by C. Pham 00443 // default sync word for non-LoRaWAN 00444 setSyncWord(_defaultSyncWord); 00445 getSyncWord(); 00446 _defaultSyncWord=_syncWord; 00447 00448 #ifdef LIMIT_TOA 00449 uint16_t remainingToA=limitToA(); 00450 printf("## Limit ToA ON ##"); 00451 printf("cycle begins at "); 00452 Serial.print(_startToAcycle); 00453 printf(" cycle ends at "); 00454 Serial.print(_endToAcycle); 00455 printf(" remaining ToA is "); 00456 Serial.print(remainingToA); 00457 printf("\n"); 00458 #endif 00459 //end 00460 00461 00462 // printf("reg1=%d \n",readRegister(1)); 00463 00464 return state; 00465 } 00466 00467 /* 00468 Function: Sets the module OFF. 00469 Returns: Nothing 00470 */ 00471 void SX1272::OFF() 00472 { 00473 #if (SX1272_debug_mode > 1) 00474 printf("\n"); 00475 printf("Starting 'OFF'\n"); 00476 #endif 00477 00478 // ajoute par C.Dupaty 00479 //SPI.end(); 00480 00481 // Powering the module 00482 // pinMode(SX1272_SS,OUTPUT); 00483 // digitalWrite(SX1272_SS,LOW); 00484 ss=0; // ????? !!!!!!!!!!!!!!!!! 00485 #if (SX1272_debug_mode > 1) 00486 printf("## Setting OFF ##"); 00487 printf("\n"); 00488 #endif 00489 } 00490 00491 /* 00492 Function: Reads the indicated register. 00493 Returns: The content of the register 00494 Parameters: 00495 address: address register to read from 00496 */ 00497 byte SX1272::readRegister(byte address) 00498 { 00499 byte value = 0x00; 00500 00501 //digitalWrite(SX1272_SS,LOW); 00502 ss=0; 00503 bitClear(address, 7); // Bit 7 cleared to write in registers 00504 // SPI.transfer(address); 00505 spi.write(address); 00506 //value = SPI.transfer(0x00); 00507 value = spi.write(0x00); 00508 //digitalWrite(SX1272_SS,HIGH); 00509 ss=1; 00510 00511 #if (SX1272_debug_mode > 1) 00512 printf("## Reading: "); 00513 printf("Register 0x%02X : 0x%02X\n",address,value); 00514 // Serial.print(address, HEX); 00515 // printf(": "); 00516 // Serial.print(value, HEX); 00517 // printf("\n"); 00518 #endif 00519 00520 return value; 00521 } 00522 00523 /* 00524 Function: Writes on the indicated register. 00525 Returns: Nothing 00526 Parameters: 00527 address: address register to write in 00528 data : value to write in the register 00529 */ 00530 void SX1272::writeRegister(byte address, byte data) 00531 { 00532 // printf("Samira - writeRegister ( ) - ENTER..\n"); 00533 00534 //digitalWrite(SX1272_SS,LOW); 00535 ss=0; 00536 bitSet(address, 7); // Bit 7 set to read from registers 00537 //SPI.transfer(address); 00538 //SPI.transfer(data); 00539 spi.write(address); 00540 spi.write(data); 00541 // digitalWrite(SX1272_SS,HIGH); 00542 ss=1; 00543 00544 #if (SX1272_debug_mode > 1) 00545 printf("## Writing: Register "); 00546 bitClear(address, 7); 00547 printf("0x%02X : 0x%02X\n",address,data); 00548 // Serial.print(address, HEX); 00549 // printf(": "); 00550 // Serial.print(data, HEX); 00551 // printf("\n"); 00552 #endif 00553 00554 } 00555 00556 /* 00557 Function: Clears the interruption flags 00558 Returns: Nothing 00559 */ 00560 void SX1272::clearFlags() 00561 { 00562 byte st0; 00563 00564 st0 = readRegister(REG_OP_MODE); // Save the previous status 00565 00566 if( _modem == LORA ) 00567 { // LoRa mode 00568 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Stdby mode to write in registers 00569 writeRegister(REG_IRQ_FLAGS, 0xFF); // LoRa mode flags register 00570 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 00571 #if (SX1272_debug_mode > 1) 00572 printf("## LoRa flags cleared ##\n"); 00573 #endif 00574 } 00575 else 00576 { // FSK mode 00577 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Stdby mode to write in registers 00578 writeRegister(REG_IRQ_FLAGS1, 0xFF); // FSK mode flags1 register 00579 writeRegister(REG_IRQ_FLAGS2, 0xFF); // FSK mode flags2 register 00580 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 00581 #if (SX1272_debug_mode > 1) 00582 printf("## FSK flags cleared ##"); 00583 #endif 00584 } 00585 } 00586 00587 /* 00588 Function: Sets the module in LoRa mode. 00589 Returns: Integer that determines if there has been any error 00590 state = 2 --> The command has not been executed 00591 state = 1 --> There has been an error while executing the command 00592 state = 0 --> The command has been executed with no errors 00593 */ 00594 uint8_t SX1272::setLORA() 00595 { 00596 uint8_t state = 2; 00597 byte st0; 00598 00599 #if (SX1272_debug_mode > 1) 00600 printf("\n"); 00601 printf("Starting 'setLORA'\n"); 00602 #endif 00603 00604 // modified by C. Pham 00605 uint8_t retry=0; 00606 00607 do { 00608 wait_ms(200); 00609 writeRegister(REG_OP_MODE, FSK_SLEEP_MODE); // Sleep mode (mandatory to set LoRa mode) 00610 writeRegister(REG_OP_MODE, LORA_SLEEP_MODE); // LoRa sleep mode 00611 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); 00612 wait_ms(50+retry*10); 00613 st0 = readRegister(REG_OP_MODE); 00614 printf("..."); 00615 00616 if ((retry % 2)==0) 00617 if (retry==20) 00618 retry=0; 00619 else 00620 retry++; 00621 /* 00622 if (st0!=LORA_STANDBY_MODE) { 00623 pinMode(SX1272_RST,OUTPUT); 00624 digitalWrite(SX1272_RST,HIGH); 00625 wait_ms(100); 00626 digitalWrite(SX1272_RST,LOW); 00627 } 00628 */ 00629 00630 } while (st0!=LORA_STANDBY_MODE); // LoRa standby mode 00631 00632 if( st0 == LORA_STANDBY_MODE) 00633 { // LoRa mode 00634 _modem = LORA; 00635 state = 0; 00636 #if (SX1272_debug_mode > 1) 00637 printf("## LoRa set with success ##"); 00638 printf("\n"); 00639 #endif 00640 } 00641 else 00642 { // FSK mode 00643 _modem = FSK; 00644 state = 1; 00645 #if (SX1272_debug_mode > 1) 00646 printf("** There has been an error while setting LoRa **"); 00647 printf("\n"); 00648 #endif 00649 } 00650 return state; 00651 } 00652 00653 /* 00654 Function: Sets the module in FSK mode. 00655 Returns: Integer that determines if there has been any error 00656 state = 2 --> The command has not been executed 00657 state = 1 --> There has been an error while executing the command 00658 state = 0 --> The command has been executed with no errors 00659 */ 00660 uint8_t SX1272::setFSK() 00661 { 00662 uint8_t state = 2; 00663 byte st0; 00664 byte config1; 00665 00666 if (_board==SX1276Chip) 00667 printf("Warning: FSK has not been tested on SX1276!"); 00668 00669 #if (SX1272_debug_mode > 1) 00670 printf("\n"); 00671 printf("Starting 'setFSK'\n"); 00672 #endif 00673 00674 writeRegister(REG_OP_MODE, FSK_SLEEP_MODE); // Sleep mode (mandatory to change mode) 00675 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // FSK standby mode 00676 config1 = readRegister(REG_PACKET_CONFIG1); 00677 config1 = config1 & 0B01111101; // clears bits 8 and 1 from REG_PACKET_CONFIG1 00678 config1 = config1 | 0B00000100; // sets bit 2 from REG_PACKET_CONFIG1 00679 writeRegister(REG_PACKET_CONFIG1,config1); // AddressFiltering = NodeAddress + BroadcastAddress 00680 writeRegister(REG_FIFO_THRESH, 0x80); // condition to start packet tx 00681 config1 = readRegister(REG_SYNC_CONFIG); 00682 config1 = config1 & 0B00111111; 00683 writeRegister(REG_SYNC_CONFIG,config1); 00684 00685 wait_ms(100); 00686 00687 st0 = readRegister(REG_OP_MODE); // Reading config mode 00688 if( st0 == FSK_STANDBY_MODE ) 00689 { // FSK mode 00690 _modem = FSK; 00691 state = 0; 00692 #if (SX1272_debug_mode > 1) 00693 printf("## FSK set with success ##"); 00694 printf("\n"); 00695 #endif 00696 } 00697 else 00698 { // LoRa mode 00699 _modem = LORA; 00700 state = 1; 00701 #if (SX1272_debug_mode > 1) 00702 printf("** There has been an error while setting FSK **"); 00703 printf("\n"); 00704 #endif 00705 } 00706 return state; 00707 } 00708 00709 /* 00710 Function: Gets the bandwidth, coding rate and spreading factor of the LoRa modulation. 00711 Returns: Integer that determines if there has been any error 00712 state = 2 --> The command has not been executed 00713 state = 1 --> There has been an error while executing the command 00714 state = 0 --> The command has been executed with no errors 00715 */ 00716 uint8_t SX1272::getMode() 00717 { 00718 byte st0; 00719 int8_t state = 2; 00720 byte value = 0x00; 00721 00722 #if (SX1272_debug_mode > 1) 00723 printf("\n"); 00724 printf("Starting 'getMode'\n"); 00725 #endif 00726 00727 st0 = readRegister(REG_OP_MODE); // Save the previous status 00728 if( _modem == FSK ) 00729 { 00730 setLORA(); // Setting LoRa mode 00731 } 00732 value = readRegister(REG_MODEM_CONFIG1); 00733 // added by C. Pham 00734 if (_board==SX1272Chip) { 00735 _bandwidth = (value >> 6); // Storing 2 MSB from REG_MODEM_CONFIG1 (=_bandwidth) 00736 // added by C. Pham 00737 // convert to common bandwidth values used by both SX1272 and SX1276 00738 _bandwidth += 7; 00739 } 00740 else 00741 _bandwidth = (value >> 4); // Storing 4 MSB from REG_MODEM_CONFIG1 (=_bandwidth) 00742 00743 if (_board==SX1272Chip) 00744 _codingRate = (value >> 3) & 0x07; // Storing third, forth and fifth bits from 00745 else 00746 _codingRate = (value >> 1) & 0x07; // Storing 3-1 bits REG_MODEM_CONFIG1 (=_codingRate) 00747 00748 value = readRegister(REG_MODEM_CONFIG2); 00749 _spreadingFactor = (value >> 4) & 0x0F; // Storing 4 MSB from REG_MODEM_CONFIG2 (=_spreadingFactor) 00750 state = 1; 00751 00752 if( isBW(_bandwidth) ) // Checking available values for: 00753 { // _bandwidth 00754 if( isCR(_codingRate) ) // _codingRate 00755 { // _spreadingFactor 00756 if( isSF(_spreadingFactor) ) 00757 { 00758 state = 0; 00759 } 00760 } 00761 } 00762 00763 #if (SX1272_debug_mode > 1) 00764 printf("## Parameters from configuration mode are:"); 00765 printf("Bandwidth: %X\n",_bandwidth); 00766 // Serial.print(_bandwidth, HEX); 00767 // printf("\n"); 00768 printf("\t Coding Rate: %X\n",_codingRate); 00769 // Serial.print(_codingRate, HEX); 00770 // printf("\n"); 00771 printf("\t Spreading Factor: %X\n",_spreadingFactor); 00772 // Serial.print(_spreadingFactor, HEX); 00773 printf(" ##"); 00774 printf("\n"); 00775 #endif 00776 00777 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 00778 wait_ms(100); 00779 return state; 00780 } 00781 00782 /* 00783 Function: Sets the bandwidth, coding rate and spreading factor of the LoRa modulation. 00784 Returns: Integer that determines if there has been any error 00785 state = 2 --> The command has not been executed 00786 state = 1 --> There has been an error while executing the command 00787 state = 0 --> The command has been executed with no errors 00788 state = -1 --> Forbidden command for this protocol 00789 Parameters: 00790 mode: mode number to set the required BW, SF and CR of LoRa modem. 00791 */ 00792 int8_t SX1272::setMode(uint8_t mode) 00793 { 00794 int8_t state = 2; 00795 byte st0; 00796 byte config1 = 0x00; 00797 byte config2 = 0x00; 00798 00799 #if (SX1272_debug_mode > 1) 00800 printf("\n"); 00801 printf("Starting 'setMode'\n"); 00802 #endif 00803 00804 st0 = readRegister(REG_OP_MODE); // Save the previous status 00805 00806 if( _modem == FSK ) 00807 { 00808 setLORA(); 00809 } 00810 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // LoRa standby mode 00811 00812 switch (mode) 00813 { 00814 // mode 1 (better reach, medium time on air) 00815 case 1: 00816 setCR(CR_5); // CR = 4/5 00817 setSF(SF_12); // SF = 12 00818 setBW(BW_125); // BW = 125 KHz 00819 break; 00820 00821 // mode 2 (medium reach, less time on air) 00822 case 2: 00823 setCR(CR_5); // CR = 4/5 00824 setSF(SF_12); // SF = 12 00825 setBW(BW_250); // BW = 250 KHz 00826 break; 00827 00828 // mode 3 (worst reach, less time on air) 00829 case 3: 00830 setCR(CR_5); // CR = 4/5 00831 setSF(SF_10); // SF = 10 00832 setBW(BW_125); // BW = 125 KHz 00833 break; 00834 00835 // mode 4 (better reach, low time on air) 00836 case 4: 00837 setCR(CR_5); // CR = 4/5 00838 setSF(SF_12); // SF = 12 00839 setBW(BW_500); // BW = 500 KHz 00840 break; 00841 00842 // mode 5 (better reach, medium time on air) 00843 case 5: 00844 setCR(CR_5); // CR = 4/5 00845 setSF(SF_10); // SF = 10 00846 setBW(BW_250); // BW = 250 KHz 00847 break; 00848 00849 // mode 6 (better reach, worst time-on-air) 00850 case 6: 00851 setCR(CR_5); // CR = 4/5 00852 setSF(SF_11); // SF = 11 00853 setBW(BW_500); // BW = 500 KHz 00854 break; 00855 00856 // mode 7 (medium-high reach, medium-low time-on-air) 00857 case 7: 00858 setCR(CR_5); // CR = 4/5 00859 setSF(SF_9); // SF = 9 00860 setBW(BW_250); // BW = 250 KHz 00861 break; 00862 00863 // mode 8 (medium reach, medium time-on-air) 00864 case 8: 00865 setCR(CR_5); // CR = 4/5 00866 setSF(SF_9); // SF = 9 00867 setBW(BW_500); // BW = 500 KHz 00868 break; 00869 00870 // mode 9 (medium-low reach, medium-high time-on-air) 00871 case 9: 00872 setCR(CR_5); // CR = 4/5 00873 setSF(SF_8); // SF = 8 00874 setBW(BW_500); // BW = 500 KHz 00875 break; 00876 00877 // mode 10 (worst reach, less time_on_air) 00878 case 10: 00879 setCR(CR_5); // CR = 4/5 00880 setSF(SF_7); // SF = 7 00881 setBW(BW_500); // BW = 500 KHz 00882 break; 00883 00884 // added by C. Pham 00885 // test for LoRaWAN channel 00886 case 11: 00887 setCR(CR_5); // CR = 4/5 00888 setSF(SF_12); // SF = 12 00889 setBW(BW_125); // BW = 125 KHz 00890 // set the sync word to the LoRaWAN sync word which is 0x34 00891 setSyncWord(0x34); 00892 printf("** Using sync word of 0x%X\n",_syncWord); 00893 // Serial.println(_syncWord, HEX); 00894 break; 00895 00896 default: state = -1; // The indicated mode doesn't exist 00897 00898 }; 00899 00900 if( state == -1 ) // if state = -1, don't change its value 00901 { 00902 #if (SX1272_debug_mode > 1) 00903 printf("** The indicated mode doesn't exist, "); 00904 printf("please select from 1 to 10 **"); 00905 #endif 00906 } 00907 else 00908 { 00909 state = 1; 00910 config1 = readRegister(REG_MODEM_CONFIG1); 00911 switch (mode) 00912 { // Different way to check for each mode: 00913 // (config1 >> 3) ---> take out bits 7-3 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 00914 // (config2 >> 4) ---> take out bits 7-4 from REG_MODEM_CONFIG2 (=_spreadingFactor) 00915 00916 // mode 1: BW = 125 KHz, CR = 4/5, SF = 12. 00917 case 1: 00918 00919 //modified by C. Pham 00920 if (_board==SX1272Chip) { 00921 //////////////////////////////////////////////possible pb sur config1 qui vaut 0 00922 if( (config1 >> 3) == 0x01 ) 00923 state=0; 00924 } 00925 else { 00926 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 00927 if( (config1 >> 1) == 0x39 ) 00928 state=0; 00929 } 00930 00931 if( state==0) { 00932 state = 1; 00933 config2 = readRegister(REG_MODEM_CONFIG2); 00934 00935 if( (config2 >> 4) == SF_12 ) 00936 { 00937 state = 0; 00938 } 00939 } 00940 break; 00941 00942 00943 // mode 2: BW = 250 KHz, CR = 4/5, SF = 12. 00944 case 2: 00945 00946 //modified by C. Pham 00947 if (_board==SX1272Chip) { 00948 if( (config1 >> 3) == 0x09 ) 00949 state=0; 00950 } 00951 else { 00952 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 00953 if( (config1 >> 1) == 0x41 ) 00954 state=0; 00955 } 00956 00957 if( state==0) { 00958 state = 1; 00959 config2 = readRegister(REG_MODEM_CONFIG2); 00960 00961 if( (config2 >> 4) == SF_12 ) 00962 { 00963 state = 0; 00964 } 00965 } 00966 break; 00967 00968 // mode 3: BW = 125 KHz, CR = 4/5, SF = 10. 00969 case 3: 00970 00971 //modified by C. Pham 00972 if (_board==SX1272Chip) { 00973 if( (config1 >> 3) == 0x01 ) 00974 state=0; 00975 } 00976 else { 00977 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 00978 if( (config1 >> 1) == 0x39 ) 00979 state=0; 00980 } 00981 00982 if( state==0) { 00983 state = 1; 00984 config2 = readRegister(REG_MODEM_CONFIG2); 00985 00986 if( (config2 >> 4) == SF_10 ) 00987 { 00988 state = 0; 00989 } 00990 } 00991 break; 00992 00993 // mode 4: BW = 500 KHz, CR = 4/5, SF = 12. 00994 case 4: 00995 00996 //modified by C. Pham 00997 if (_board==SX1272Chip) { 00998 if( (config1 >> 3) == 0x11 ) 00999 state=0; 01000 } 01001 else { 01002 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01003 if( (config1 >> 1) == 0x49 ) 01004 state=0; 01005 } 01006 01007 if( state==0) { 01008 state = 1; 01009 config2 = readRegister(REG_MODEM_CONFIG2); 01010 01011 if( (config2 >> 4) == SF_12 ) 01012 { 01013 state = 0; 01014 } 01015 } 01016 break; 01017 01018 // mode 5: BW = 250 KHz, CR = 4/5, SF = 10. 01019 case 5: 01020 01021 //modified by C. Pham 01022 if (_board==SX1272Chip) { 01023 if( (config1 >> 3) == 0x09 ) 01024 state=0; 01025 } 01026 else { 01027 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01028 if( (config1 >> 1) == 0x41 ) 01029 state=0; 01030 } 01031 01032 if( state==0) { 01033 state = 1; 01034 config2 = readRegister(REG_MODEM_CONFIG2); 01035 01036 if( (config2 >> 4) == SF_10 ) 01037 { 01038 state = 0; 01039 } 01040 } 01041 break; 01042 01043 // mode 6: BW = 500 KHz, CR = 4/5, SF = 11. 01044 case 6: 01045 01046 //modified by C. Pham 01047 if (_board==SX1272Chip) { 01048 if( (config1 >> 3) == 0x11 ) 01049 state=0; 01050 } 01051 else { 01052 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01053 if( (config1 >> 1) == 0x49 ) 01054 state=0; 01055 } 01056 01057 if( state==0) { 01058 state = 1; 01059 config2 = readRegister(REG_MODEM_CONFIG2); 01060 01061 if( (config2 >> 4) == SF_11 ) 01062 { 01063 state = 0; 01064 } 01065 } 01066 break; 01067 01068 // mode 7: BW = 250 KHz, CR = 4/5, SF = 9. 01069 case 7: 01070 01071 //modified by C. Pham 01072 if (_board==SX1272Chip) { 01073 if( (config1 >> 3) == 0x09 ) 01074 state=0; 01075 } 01076 else { 01077 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01078 if( (config1 >> 1) == 0x41 ) 01079 state=0; 01080 } 01081 01082 if( state==0) { 01083 state = 1; 01084 config2 = readRegister(REG_MODEM_CONFIG2); 01085 01086 if( (config2 >> 4) == SF_9 ) 01087 { 01088 state = 0; 01089 } 01090 } 01091 break; 01092 01093 // mode 8: BW = 500 KHz, CR = 4/5, SF = 9. 01094 case 8: 01095 01096 //modified by C. Pham 01097 if (_board==SX1272Chip) { 01098 if( (config1 >> 3) == 0x11 ) 01099 state=0; 01100 } 01101 else { 01102 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01103 if( (config1 >> 1) == 0x49 ) 01104 state=0; 01105 } 01106 01107 if( state==0) { 01108 state = 1; 01109 config2 = readRegister(REG_MODEM_CONFIG2); 01110 01111 if( (config2 >> 4) == SF_9 ) 01112 { 01113 state = 0; 01114 } 01115 } 01116 break; 01117 01118 // mode 9: BW = 500 KHz, CR = 4/5, SF = 8. 01119 case 9: 01120 01121 //modified by C. Pham 01122 if (_board==SX1272Chip) { 01123 if( (config1 >> 3) == 0x11 ) 01124 state=0; 01125 } 01126 else { 01127 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01128 if( (config1 >> 1) == 0x49 ) 01129 state=0; 01130 } 01131 01132 if( state==0) { 01133 state = 1; 01134 config2 = readRegister(REG_MODEM_CONFIG2); 01135 01136 if( (config2 >> 4) == SF_8 ) 01137 { 01138 state = 0; 01139 } 01140 } 01141 break; 01142 01143 // mode 10: BW = 500 KHz, CR = 4/5, SF = 7. 01144 case 10: 01145 01146 //modified by C. Pham 01147 if (_board==SX1272Chip) { 01148 if( (config1 >> 3) == 0x11 ) 01149 state=0; 01150 } 01151 else { 01152 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01153 if( (config1 >> 1) == 0x49 ) 01154 state=0; 01155 } 01156 01157 if( state==0) { 01158 state = 1; 01159 config2 = readRegister(REG_MODEM_CONFIG2); 01160 01161 if( (config2 >> 4) == SF_7 ) 01162 { 01163 state = 0; 01164 } 01165 } 01166 break; 01167 01168 // added by C. Pham 01169 // test of LoRaWAN channel 01170 // mode 11: BW = 125 KHz, CR = 4/5, SF = 12. 01171 case 11: 01172 01173 //modified by C. Pham 01174 if (_board==SX1272Chip) { 01175 if( (config1 >> 3) == 0x01 ) 01176 state=0; 01177 } 01178 else { 01179 // (config1 >> 1) ---> take out bits 7-1 from REG_MODEM_CONFIG1 (=_bandwidth & _codingRate together) 01180 if( (config1 >> 1) == 0x39 ) 01181 state=0; 01182 } 01183 01184 if( state==0) { 01185 state = 1; 01186 config2 = readRegister(REG_MODEM_CONFIG2); 01187 01188 if( (config2 >> 4) == SF_12 ) 01189 { 01190 state = 0; 01191 } 01192 } 01193 break; 01194 }// end switch 01195 01196 if (mode!=11) { 01197 setSyncWord(_defaultSyncWord); 01198 #if (SX1272_debug_mode > 1) 01199 printf("*** Using sync word of 0x%X\n",_defaultSyncWord); 01200 // Serial.println(_defaultSyncWord, HEX); 01201 #endif 01202 } 01203 } 01204 // added by C. Pham 01205 if (state == 0) 01206 _loraMode=mode; 01207 01208 #if (SX1272_debug_mode > 1) 01209 01210 if( state == 0 ) 01211 { 01212 printf("## Mode %d ",mode); 01213 // Serial.print(mode, DEC); 01214 printf(" configured with success ##"); 01215 } 01216 else 01217 { 01218 printf("** There has been an error while configuring mode %d ",mode); 01219 // Serial.print(mode, DEC); 01220 printf(". **\n"); 01221 } 01222 #endif 01223 01224 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 01225 wait_ms(100); 01226 return state; 01227 } 01228 01229 /* 01230 Function: Indicates if module is configured in implicit or explicit header mode. 01231 Returns: Integer that determines if there has been any error 01232 state = 2 --> The command has not been executed 01233 state = 1 --> There has been an error while executing the command 01234 state = 0 --> The command has been executed with no errors 01235 */ 01236 uint8_t SX1272::getHeader() 01237 { 01238 int8_t state = 2; 01239 01240 #if (SX1272_debug_mode > 1) 01241 printf("\n"); 01242 printf("Starting 'getHeader'\n"); 01243 #endif 01244 01245 // added by C. Pham 01246 uint8_t theHeaderBit; 01247 01248 if (_board==SX1272Chip) 01249 theHeaderBit=2; 01250 else 01251 theHeaderBit=0; 01252 01253 // take out bit 2 from REG_MODEM_CONFIG1 indicates ImplicitHeaderModeOn 01254 if( bitRead(REG_MODEM_CONFIG1, theHeaderBit) == 0 ) 01255 { // explicit header mode (ON) 01256 _header = HEADER_ON; 01257 state = 1; 01258 } 01259 else 01260 { // implicit header mode (OFF) 01261 _header = HEADER_OFF; 01262 state = 1; 01263 } 01264 01265 state = 0; 01266 01267 if( _modem == FSK ) 01268 { // header is not available in FSK mode 01269 #if (SX1272_debug_mode > 1) 01270 printf("## Notice that FSK mode packets hasn't header ##"); 01271 printf("\n"); 01272 #endif 01273 } 01274 else 01275 { // header in LoRa mode 01276 #if (SX1272_debug_mode > 1) 01277 printf("## Header is "); 01278 if( _header == HEADER_ON ) 01279 { 01280 printf("in explicit header mode ##"); 01281 } 01282 else 01283 { 01284 printf("in implicit header mode ##"); 01285 } 01286 printf("\n"); 01287 #endif 01288 } 01289 return state; 01290 } 01291 01292 /* 01293 Function: Sets the module in explicit header mode (header is sent). 01294 Returns: Integer that determines if there has been any error 01295 state = 2 --> The command has not been executed 01296 state = 1 --> There has been an error while executing the command 01297 state = 0 --> The command has been executed with no errors 01298 state = -1 --> Forbidden command for this protocol 01299 */ 01300 int8_t SX1272::setHeaderON() 01301 { 01302 int8_t state = 2; 01303 byte config1; 01304 01305 #if (SX1272_debug_mode > 1) 01306 printf("\n"); 01307 printf("Starting 'setHeaderON'\n"); 01308 #endif 01309 01310 if( _modem == FSK ) 01311 { 01312 state = -1; // header is not available in FSK mode 01313 #if (SX1272_debug_mode > 1) 01314 printf("## FSK mode packets hasn't header ##"); 01315 printf("\n"); 01316 #endif 01317 } 01318 else 01319 { 01320 config1 = readRegister(REG_MODEM_CONFIG1); // Save config1 to modify only the header bit 01321 if( _spreadingFactor == 6 ) 01322 { 01323 state = -1; // Mandatory headerOFF with SF = 6 01324 #if (SX1272_debug_mode > 1) 01325 printf("## Mandatory implicit header mode with spreading factor = 6 ##"); 01326 #endif 01327 } 01328 else 01329 { 01330 // added by C. Pham 01331 if (_board==SX1272Chip) 01332 config1 = config1 & 0B11111011; // clears bit 2 from config1 = headerON 01333 else 01334 config1 = config1 & 0B11111110; // clears bit 0 from config1 = headerON 01335 01336 writeRegister(REG_MODEM_CONFIG1,config1); // Update config1 01337 } 01338 01339 // added by C. Pham 01340 uint8_t theHeaderBit; 01341 01342 if (_board==SX1272Chip) 01343 theHeaderBit=2; 01344 else 01345 theHeaderBit=0; 01346 01347 if( _spreadingFactor != 6 ) 01348 { // checking headerON taking out bit 2 from REG_MODEM_CONFIG1 01349 config1 = readRegister(REG_MODEM_CONFIG1); 01350 // modified by C. Pham 01351 if( bitRead(config1, theHeaderBit) == HEADER_ON ) 01352 { 01353 state = 0; 01354 _header = HEADER_ON; 01355 #if (SX1272_debug_mode > 1) 01356 printf("## Header has been activated ##"); 01357 printf("\n"); 01358 #endif 01359 } 01360 else 01361 { 01362 state = 1; 01363 } 01364 } 01365 // modifie par C.Dupaty 01366 //return state; 01367 } 01368 return state; 01369 } 01370 01371 /* 01372 Function: Sets the module in implicit header mode (header is not sent). 01373 Returns: Integer that determines if there has been any error 01374 state = 2 --> The command has not been executed 01375 state = 1 --> There has been an error while executing the command 01376 state = 0 --> The command has been executed with no errors 01377 state = -1 --> Forbidden command for this protocol 01378 */ 01379 int8_t SX1272::setHeaderOFF() 01380 { 01381 int8_t state = 2; 01382 byte config1; 01383 01384 #if (SX1272_debug_mode > 1) 01385 printf("\n"); 01386 printf("Starting 'setHeaderOFF'\n"); 01387 #endif 01388 01389 if( _modem == FSK ) 01390 { // header is not available in FSK mode 01391 state = -1; 01392 #if (SX1272_debug_mode > 1) 01393 printf("## Notice that FSK mode packets hasn't header ##"); 01394 printf("\n"); 01395 #endif 01396 } 01397 else 01398 { 01399 config1 = readRegister(REG_MODEM_CONFIG1); // Save config1 to modify only the header bit 01400 01401 // modified by C. Pham 01402 if (_board==SX1272Chip) 01403 config1 = config1 | 0B00000100; // sets bit 2 from REG_MODEM_CONFIG1 = headerOFF 01404 else 01405 config1 = config1 | 0B00000001; // sets bit 0 from REG_MODEM_CONFIG1 = headerOFF 01406 01407 writeRegister(REG_MODEM_CONFIG1,config1); // Update config1 01408 01409 config1 = readRegister(REG_MODEM_CONFIG1); 01410 01411 // added by C. Pham 01412 uint8_t theHeaderBit; 01413 01414 if (_board==SX1272Chip) 01415 theHeaderBit=2; 01416 else 01417 theHeaderBit=0; 01418 01419 if( bitRead(config1, theHeaderBit) == HEADER_OFF ) 01420 { // checking headerOFF taking out bit 2 from REG_MODEM_CONFIG1 01421 state = 0; 01422 _header = HEADER_OFF; 01423 01424 #if (SX1272_debug_mode > 1) 01425 printf("## Header has been desactivated ##"); 01426 printf("\n"); 01427 #endif 01428 } 01429 else 01430 { 01431 state = 1; 01432 #if (SX1272_debug_mode > 1) 01433 printf("** Header hasn't been desactivated ##"); 01434 printf("\n"); 01435 #endif 01436 } 01437 } 01438 return state; 01439 } 01440 01441 /* 01442 Function: Indicates if module is configured with or without checking CRC. 01443 Returns: Integer that determines if there has been any error 01444 state = 2 --> The command has not been executed 01445 state = 1 --> There has been an error while executing the command 01446 state = 0 --> The command has been executed with no errors 01447 */ 01448 uint8_t SX1272::getCRC() 01449 { 01450 int8_t state = 2; 01451 byte value; 01452 01453 #if (SX1272_debug_mode > 1) 01454 printf("\n"); 01455 printf("Starting 'getCRC'\n"); 01456 #endif 01457 01458 if( _modem == LORA ) 01459 { // LoRa mode 01460 01461 // added by C. Pham 01462 uint8_t theRegister; 01463 uint8_t theCrcBit; 01464 01465 if (_board==SX1272Chip) { 01466 theRegister=REG_MODEM_CONFIG1; 01467 theCrcBit=1; 01468 } 01469 else { 01470 theRegister=REG_MODEM_CONFIG2; 01471 theCrcBit=2; 01472 } 01473 01474 // take out bit 1 from REG_MODEM_CONFIG1 indicates RxPayloadCrcOn 01475 value = readRegister(theRegister); 01476 if( bitRead(value, theCrcBit) == CRC_OFF ) 01477 { // CRCoff 01478 _CRC = CRC_OFF; 01479 #if (SX1272_debug_mode > 1) 01480 printf("## CRC is desactivated ##"); 01481 printf("\n"); 01482 #endif 01483 state = 0; 01484 } 01485 else 01486 { // CRCon 01487 _CRC = CRC_ON; 01488 #if (SX1272_debug_mode > 1) 01489 printf("## CRC is activated ##"); 01490 printf("\n"); 01491 #endif 01492 state = 0; 01493 } 01494 } 01495 else 01496 { // FSK mode 01497 01498 // take out bit 2 from REG_PACKET_CONFIG1 indicates CrcOn 01499 value = readRegister(REG_PACKET_CONFIG1); 01500 if( bitRead(value, 4) == CRC_OFF ) 01501 { // CRCoff 01502 _CRC = CRC_OFF; 01503 #if (SX1272_debug_mode > 1) 01504 printf("## CRC is desactivated ##"); 01505 printf("\n"); 01506 #endif 01507 state = 0; 01508 } 01509 else 01510 { // CRCon 01511 _CRC = CRC_ON; 01512 #if (SX1272_debug_mode > 1) 01513 printf("## CRC is activated ##"); 01514 printf("\n"); 01515 #endif 01516 state = 0; 01517 } 01518 } 01519 if( state != 0 ) 01520 { 01521 state = 1; 01522 #if (SX1272_debug_mode > 1) 01523 printf("** There has been an error while getting configured CRC **"); 01524 printf("\n"); 01525 #endif 01526 } 01527 return state; 01528 } 01529 01530 /* 01531 Function: Sets the module with CRC on. 01532 Returns: Integer that determines if there has been any error 01533 state = 2 --> The command has not been executed 01534 state = 1 --> There has been an error while executing the command 01535 state = 0 --> The command has been executed with no errors 01536 */ 01537 uint8_t SX1272::setCRC_ON() 01538 { 01539 uint8_t state = 2; 01540 byte config1; 01541 01542 #if (SX1272_debug_mode > 1) 01543 printf("\n"); 01544 printf("Starting 'setCRC_ON'\n"); 01545 #endif 01546 01547 if( _modem == LORA ) 01548 { // LORA mode 01549 01550 // added by C. Pham 01551 uint8_t theRegister; 01552 uint8_t theCrcBit; 01553 01554 if (_board==SX1272Chip) { 01555 theRegister=REG_MODEM_CONFIG1; 01556 theCrcBit=1; 01557 } 01558 else { 01559 theRegister=REG_MODEM_CONFIG2; 01560 theCrcBit=2; 01561 } 01562 01563 config1 = readRegister(theRegister); // Save config1 to modify only the CRC bit 01564 01565 if (_board==SX1272Chip) 01566 config1 = config1 | 0B00000010; // sets bit 1 from REG_MODEM_CONFIG1 = CRC_ON 01567 else 01568 config1 = config1 | 0B00000100; // sets bit 2 from REG_MODEM_CONFIG2 = CRC_ON 01569 01570 writeRegister(theRegister,config1); 01571 01572 state = 1; 01573 01574 config1 = readRegister(theRegister); 01575 01576 if( bitRead(config1, theCrcBit) == CRC_ON ) 01577 { // take out bit 1 from REG_MODEM_CONFIG1 indicates RxPayloadCrcOn 01578 state = 0; 01579 _CRC = CRC_ON; 01580 #if (SX1272_debug_mode > 1) 01581 printf("## CRC has been activated ##"); 01582 printf("\n"); 01583 #endif 01584 } 01585 } 01586 else 01587 { // FSK mode 01588 config1 = readRegister(REG_PACKET_CONFIG1); // Save config1 to modify only the CRC bit 01589 config1 = config1 | 0B00010000; // set bit 4 and 3 from REG_MODEM_CONFIG1 = CRC_ON 01590 writeRegister(REG_PACKET_CONFIG1,config1); 01591 01592 state = 1; 01593 01594 config1 = readRegister(REG_PACKET_CONFIG1); 01595 if( bitRead(config1, 4) == CRC_ON ) 01596 { // take out bit 4 from REG_PACKET_CONFIG1 indicates CrcOn 01597 state = 0; 01598 _CRC = CRC_ON; 01599 #if (SX1272_debug_mode > 1) 01600 printf("## CRC has been activated ##"); 01601 printf("\n"); 01602 #endif 01603 } 01604 } 01605 if( state != 0 ) 01606 { 01607 state = 1; 01608 #if (SX1272_debug_mode > 1) 01609 printf("** There has been an error while setting CRC ON **"); 01610 printf("\n"); 01611 #endif 01612 } 01613 return state; 01614 } 01615 01616 /* 01617 Function: Sets the module with CRC off. 01618 Returns: Integer that determines if there has been any error 01619 state = 2 --> The command has not been executed 01620 state = 1 --> There has been an error while executing the command 01621 state = 0 --> The command has been executed with no errors 01622 */ 01623 uint8_t SX1272::setCRC_OFF() 01624 { 01625 int8_t state = 2; 01626 byte config1; 01627 01628 #if (SX1272_debug_mode > 1) 01629 printf("\n"); 01630 printf("Starting 'setCRC_OFF'\n"); 01631 #endif 01632 01633 if( _modem == LORA ) 01634 { // LORA mode 01635 01636 // added by C. Pham 01637 uint8_t theRegister; 01638 uint8_t theCrcBit; 01639 01640 if (_board==SX1272Chip) { 01641 theRegister=REG_MODEM_CONFIG1; 01642 theCrcBit=1; 01643 } 01644 else { 01645 theRegister=REG_MODEM_CONFIG2; 01646 theCrcBit=2; 01647 } 01648 01649 config1 = readRegister(theRegister); // Save config1 to modify only the CRC bit 01650 if (_board==SX1272Chip) 01651 config1 = config1 & 0B11111101; // clears bit 1 from config1 = CRC_OFF 01652 else 01653 config1 = config1 & 0B11111011; // clears bit 2 from config1 = CRC_OFF 01654 01655 writeRegister(theRegister,config1); 01656 01657 config1 = readRegister(theRegister); 01658 if( (bitRead(config1, theCrcBit)) == CRC_OFF ) 01659 { // take out bit 1 from REG_MODEM_CONFIG1 indicates RxPayloadCrcOn 01660 state = 0; 01661 _CRC = CRC_OFF; 01662 #if (SX1272_debug_mode > 1) 01663 printf("## CRC has been desactivated ##"); 01664 printf("\n"); 01665 #endif 01666 } 01667 } 01668 else 01669 { // FSK mode 01670 config1 = readRegister(REG_PACKET_CONFIG1); // Save config1 to modify only the CRC bit 01671 config1 = config1 & 0B11101111; // clears bit 4 from config1 = CRC_OFF 01672 writeRegister(REG_PACKET_CONFIG1,config1); 01673 01674 config1 = readRegister(REG_PACKET_CONFIG1); 01675 if( bitRead(config1, 4) == CRC_OFF ) 01676 { // take out bit 4 from REG_PACKET_CONFIG1 indicates RxPayloadCrcOn 01677 state = 0; 01678 _CRC = CRC_OFF; 01679 #if (SX1272_debug_mode > 1) 01680 printf("## CRC has been desactivated ##"); 01681 printf("\n"); 01682 #endif 01683 } 01684 } 01685 if( state != 0 ) 01686 { 01687 state = 1; 01688 #if (SX1272_debug_mode > 1) 01689 printf("** There has been an error while setting CRC OFF **"); 01690 printf("\n"); 01691 #endif 01692 } 01693 return state; 01694 } 01695 01696 /* 01697 Function: Checks if SF is a valid value. 01698 Returns: Boolean that's 'true' if the SF value exists and 01699 it's 'false' if the SF value does not exist. 01700 Parameters: 01701 spr: spreading factor value to check. 01702 */ 01703 boolean SX1272::isSF(uint8_t spr) 01704 { 01705 #if (SX1272_debug_mode > 1) 01706 printf("\n"); 01707 printf("Starting 'isSF'\n"); 01708 #endif 01709 01710 // Checking available values for _spreadingFactor 01711 switch(spr) 01712 { 01713 case SF_6: 01714 case SF_7: 01715 case SF_8: 01716 case SF_9: 01717 case SF_10: 01718 case SF_11: 01719 case SF_12: 01720 return true; 01721 //break; 01722 01723 default: 01724 return false; 01725 } 01726 #if (SX1272_debug_mode > 1) 01727 printf("## Finished 'isSF' ##"); 01728 printf("\n"); 01729 #endif 01730 } 01731 01732 /* 01733 Function: Gets the SF within the module is configured. 01734 Returns: Integer that determines if there has been any error 01735 state = 2 --> The command has not been executed 01736 state = 1 --> There has been an error while executing the command 01737 state = 0 --> The command has been executed with no errors 01738 state = -1 --> Forbidden command for this protocol 01739 */ 01740 int8_t SX1272::getSF() 01741 { 01742 int8_t state = 2; 01743 byte config2; 01744 01745 #if (SX1272_debug_mode > 1) 01746 printf("\n"); 01747 printf("Starting 'getSF'\n"); 01748 #endif 01749 01750 if( _modem == FSK ) 01751 { 01752 state = -1; // SF is not available in FSK mode 01753 #if (SX1272_debug_mode > 1) 01754 printf("** FSK mode hasn't spreading factor **"); 01755 printf("\n"); 01756 #endif 01757 } 01758 else 01759 { 01760 // take out bits 7-4 from REG_MODEM_CONFIG2 indicates _spreadingFactor 01761 config2 = (readRegister(REG_MODEM_CONFIG2)) >> 4; 01762 _spreadingFactor = config2; 01763 state = 1; 01764 01765 if( (config2 == _spreadingFactor) && isSF(_spreadingFactor) ) 01766 { 01767 state = 0; 01768 #if (SX1272_debug_mode > 1) 01769 printf("## Spreading factor is %X",_spreadingFactor); 01770 // Serial.print(_spreadingFactor,HEX); 01771 printf(" ##"); 01772 printf("\n"); 01773 #endif 01774 } 01775 } 01776 return state; 01777 } 01778 01779 /* 01780 Function: Sets the indicated SF in the module. 01781 Returns: Integer that determines if there has been any error 01782 state = 2 --> The command has not been executed 01783 state = 1 --> There has been an error while executing the command 01784 state = 0 --> The command has been executed with no errors 01785 Parameters: 01786 spr: spreading factor value to set in LoRa modem configuration. 01787 */ 01788 uint8_t SX1272::setSF(uint8_t spr) 01789 { 01790 byte st0; 01791 int8_t state = 2; 01792 byte config1; 01793 byte config2; 01794 01795 #if (SX1272_debug_mode > 1) 01796 printf("\n"); 01797 printf("Starting 'setSF'\n"); 01798 #endif 01799 01800 st0 = readRegister(REG_OP_MODE); // Save the previous status 01801 01802 if( _modem == FSK ) 01803 { 01804 #if (SX1272_debug_mode > 1) 01805 printf("## Notice that FSK hasn't Spreading Factor parameter, "); 01806 printf("so you are configuring it in LoRa mode ##"); 01807 #endif 01808 state = setLORA(); // Setting LoRa mode 01809 } 01810 else 01811 { // LoRa mode 01812 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // LoRa standby mode 01813 config2 = (readRegister(REG_MODEM_CONFIG2)); // Save config2 to modify SF value (bits 7-4) 01814 switch(spr) 01815 { 01816 case SF_6: config2 = config2 & 0B01101111; // clears bits 7 & 4 from REG_MODEM_CONFIG2 01817 config2 = config2 | 0B01100000; // sets bits 6 & 5 from REG_MODEM_CONFIG2 01818 setHeaderOFF(); // Mandatory headerOFF with SF = 6 01819 break; 01820 case SF_7: config2 = config2 & 0B01111111; // clears bits 7 from REG_MODEM_CONFIG2 01821 config2 = config2 | 0B01110000; // sets bits 6, 5 & 4 01822 break; 01823 case SF_8: config2 = config2 & 0B10001111; // clears bits 6, 5 & 4 from REG_MODEM_CONFIG2 01824 config2 = config2 | 0B10000000; // sets bit 7 from REG_MODEM_CONFIG2 01825 break; 01826 case SF_9: config2 = config2 & 0B10011111; // clears bits 6, 5 & 4 from REG_MODEM_CONFIG2 01827 config2 = config2 | 0B10010000; // sets bits 7 & 4 from REG_MODEM_CONFIG2 01828 break; 01829 case SF_10: config2 = config2 & 0B10101111; // clears bits 6 & 4 from REG_MODEM_CONFIG2 01830 config2 = config2 | 0B10100000; // sets bits 7 & 5 from REG_MODEM_CONFIG2 01831 break; 01832 case SF_11: config2 = config2 & 0B10111111; // clears bit 6 from REG_MODEM_CONFIG2 01833 config2 = config2 | 0B10110000; // sets bits 7, 5 & 4 from REG_MODEM_CONFIG2 01834 getBW(); 01835 01836 // modified by C. Pham 01837 if( _bandwidth == BW_125) 01838 { // LowDataRateOptimize (Mandatory with SF_11 if BW_125) 01839 if (_board==SX1272Chip) { 01840 config1 = (readRegister(REG_MODEM_CONFIG1)); // Save config1 to modify only the LowDataRateOptimize 01841 config1 = config1 | 0B00000001; 01842 writeRegister(REG_MODEM_CONFIG1,config1); 01843 } 01844 else { 01845 byte config3=readRegister(REG_MODEM_CONFIG3); 01846 config3 = config3 | 0B00001000; 01847 writeRegister(REG_MODEM_CONFIG3,config3); 01848 } 01849 } 01850 break; 01851 case SF_12: config2 = config2 & 0B11001111; // clears bits 5 & 4 from REG_MODEM_CONFIG2 01852 config2 = config2 | 0B11000000; // sets bits 7 & 6 from REG_MODEM_CONFIG2 01853 if( _bandwidth == BW_125) 01854 { // LowDataRateOptimize (Mandatory with SF_12 if BW_125) 01855 // modified by C. Pham 01856 if (_board==SX1272Chip) { 01857 config1 = (readRegister(REG_MODEM_CONFIG1)); // Save config1 to modify only the LowDataRateOptimize 01858 config1 = config1 | 0B00000001; 01859 writeRegister(REG_MODEM_CONFIG1,config1); 01860 } 01861 else { 01862 byte config3=readRegister(REG_MODEM_CONFIG3); 01863 config3 = config3 | 0B00001000; 01864 writeRegister(REG_MODEM_CONFIG3,config3); 01865 } 01866 } 01867 break; 01868 } 01869 01870 // Check if it is neccesary to set special settings for SF=6 01871 if( spr == SF_6 ) 01872 { 01873 // Mandatory headerOFF with SF = 6 (Implicit mode) 01874 setHeaderOFF(); 01875 01876 // Set the bit field DetectionOptimize of 01877 // register RegLoRaDetectOptimize to value "0b101". 01878 writeRegister(REG_DETECT_OPTIMIZE, 0x05); 01879 01880 // Write 0x0C in the register RegDetectionThreshold. 01881 writeRegister(REG_DETECTION_THRESHOLD, 0x0C); 01882 } 01883 else 01884 { 01885 // added by C. Pham 01886 setHeaderON(); 01887 01888 // LoRa detection Optimize: 0x03 --> SF7 to SF12 01889 writeRegister(REG_DETECT_OPTIMIZE, 0x03); 01890 01891 // LoRa detection threshold: 0x0A --> SF7 to SF12 01892 writeRegister(REG_DETECTION_THRESHOLD, 0x0A); 01893 } 01894 01895 // added by C. Pham 01896 if (_board==SX1272Chip) { 01897 // comment by C. Pham 01898 // bit 9:8 of SymbTimeout are then 11 01899 // single_chan_pkt_fwd uses 00 and then 00001000 01900 // why? 01901 // sets bit 2-0 (AgcAutoOn and SymbTimout) for any SF value 01902 //config2 = config2 | 0B00000111; 01903 // modified by C. Pham 01904 config2 = config2 | 0B00000100; 01905 writeRegister(REG_MODEM_CONFIG1, config1); // Update config1 01906 } 01907 else { 01908 // set the AgcAutoOn in bit 2 of REG_MODEM_CONFIG3 01909 uint8_t config3 = (readRegister(REG_MODEM_CONFIG3)); 01910 config3=config3 | 0B00000100; 01911 writeRegister(REG_MODEM_CONFIG3, config3); 01912 } 01913 01914 // here we write the new SF 01915 writeRegister(REG_MODEM_CONFIG2, config2); // Update config2 01916 01917 wait_ms(100); 01918 01919 // added by C. Pham 01920 byte configAgc; 01921 uint8_t theLDRBit; 01922 01923 if (_board==SX1272Chip) { 01924 config1 = (readRegister(REG_MODEM_CONFIG1)); // Save config1 to check update 01925 config2 = (readRegister(REG_MODEM_CONFIG2)); // Save config2 to check update 01926 // comment by C. Pham 01927 // (config2 >> 4) ---> take out bits 7-4 from REG_MODEM_CONFIG2 (=_spreadingFactor) 01928 // bitRead(config1, 0) ---> take out bits 1 from config1 (=LowDataRateOptimize) 01929 // config2 is only for the AgcAutoOn 01930 configAgc=config2; 01931 theLDRBit=0; 01932 } 01933 else { 01934 config1 = (readRegister(REG_MODEM_CONFIG3)); // Save config1 to check update 01935 config2 = (readRegister(REG_MODEM_CONFIG2)); 01936 // LowDataRateOptimize is in REG_MODEM_CONFIG3 01937 // AgcAutoOn is in REG_MODEM_CONFIG3 01938 configAgc=config1; 01939 theLDRBit=3; 01940 } 01941 01942 01943 switch(spr) 01944 { 01945 case SF_6: if( ((config2 >> 4) == spr) 01946 && (bitRead(configAgc, 2) == 1) 01947 && (_header == HEADER_OFF)) 01948 { 01949 state = 0; 01950 } 01951 break; 01952 case SF_7: if( ((config2 >> 4) == 0x07) 01953 && (bitRead(configAgc, 2) == 1)) 01954 { 01955 state = 0; 01956 } 01957 break; 01958 case SF_8: if( ((config2 >> 4) == 0x08) 01959 && (bitRead(configAgc, 2) == 1)) 01960 { 01961 state = 0; 01962 } 01963 break; 01964 case SF_9: if( ((config2 >> 4) == 0x09) 01965 && (bitRead(configAgc, 2) == 1)) 01966 { 01967 state = 0; 01968 } 01969 break; 01970 case SF_10: if( ((config2 >> 4) == 0x0A) 01971 && (bitRead(configAgc, 2) == 1)) 01972 { 01973 state = 0; 01974 } 01975 break; 01976 case SF_11: if( ((config2 >> 4) == 0x0B) 01977 && (bitRead(configAgc, 2) == 1) 01978 && (bitRead(config1, theLDRBit) == 1)) 01979 { 01980 state = 0; 01981 } 01982 break; 01983 case SF_12: if( ((config2 >> 4) == 0x0C) 01984 && (bitRead(configAgc, 2) == 1) 01985 && (bitRead(config1, theLDRBit) == 1)) 01986 { 01987 state = 0; 01988 } 01989 break; 01990 default: state = 1; 01991 } 01992 } 01993 01994 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 01995 wait_ms(100); 01996 01997 if( isSF(spr) ) 01998 { // Checking available value for _spreadingFactor 01999 state = 0; 02000 _spreadingFactor = spr; 02001 #if (SX1272_debug_mode > 1) 02002 printf("## Spreading factor %d ",_spreadingFactor); 02003 // Serial.print(_spreadingFactor, DEC); 02004 printf(" has been successfully set ##"); 02005 printf("\n"); 02006 #endif 02007 } 02008 else 02009 { 02010 if( state != 0 ) 02011 { 02012 #if (SX1272_debug_mode > 1) 02013 printf("** There has been an error while setting the spreading factor **"); 02014 printf("\n"); 02015 #endif 02016 } 02017 } 02018 return state; 02019 } 02020 02021 /* 02022 Function: Checks if BW is a valid value. 02023 Returns: Boolean that's 'true' if the BW value exists and 02024 it's 'false' if the BW value does not exist. 02025 Parameters: 02026 band: bandwidth value to check. 02027 */ 02028 boolean SX1272::isBW(uint16_t band) 02029 { 02030 #if (SX1272_debug_mode > 1) 02031 printf("\n"); 02032 printf("Starting 'isBW'\n"); 02033 #endif 02034 02035 // Checking available values for _bandwidth 02036 // added by C. Pham 02037 if (_board==SX1272Chip) { 02038 switch(band) 02039 { 02040 case BW_125: 02041 case BW_250: 02042 case BW_500: 02043 return true; 02044 //break; 02045 02046 default: 02047 return false; 02048 } 02049 } 02050 else { 02051 switch(band) 02052 { 02053 case BW_7_8: 02054 case BW_10_4: 02055 case BW_15_6: 02056 case BW_20_8: 02057 case BW_31_25: 02058 case BW_41_7: 02059 case BW_62_5: 02060 case BW_125: 02061 case BW_250: 02062 case BW_500: 02063 return true; 02064 //break; 02065 02066 default: 02067 return false; 02068 } 02069 } 02070 02071 #if (SX1272_debug_mode > 1) 02072 printf("## Finished 'isBW' ##"); 02073 printf("\n"); 02074 #endif 02075 } 02076 02077 /* 02078 Function: Gets the BW within the module is configured. 02079 Returns: Integer that determines if there has been any error 02080 state = 2 --> The command has not been executed 02081 state = 1 --> There has been an error while executing the command 02082 state = 0 --> The command has been executed with no errors 02083 state = -1 --> Forbidden command for this protocol 02084 */ 02085 int8_t SX1272::getBW() 02086 { 02087 int8_t state = 2; 02088 byte config1; 02089 02090 #if (SX1272_debug_mode > 1) 02091 printf("\n"); 02092 printf("Starting 'getBW'\n"); 02093 #endif 02094 02095 if( _modem == FSK ) 02096 { 02097 state = -1; // BW is not available in FSK mode 02098 #if (SX1272_debug_mode > 1) 02099 printf("** FSK mode hasn't bandwidth **"); 02100 printf("\n"); 02101 #endif 02102 } 02103 else 02104 { 02105 // added by C. Pham 02106 if (_board==SX1272Chip) { 02107 // take out bits 7-6 from REG_MODEM_CONFIG1 indicates _bandwidth 02108 config1 = (readRegister(REG_MODEM_CONFIG1)) >> 6; 02109 } 02110 else { 02111 // take out bits 7-4 from REG_MODEM_CONFIG1 indicates _bandwidth 02112 config1 = (readRegister(REG_MODEM_CONFIG1)) >> 4; 02113 } 02114 02115 _bandwidth = config1; 02116 02117 if( (config1 == _bandwidth) && isBW(_bandwidth) ) 02118 { 02119 state = 0; 02120 #if (SX1272_debug_mode > 1) 02121 printf("## Bandwidth is %X ",_bandwidth); 02122 // Serial.print(_bandwidth,HEX); 02123 printf(" ##"); 02124 printf("\n"); 02125 #endif 02126 } 02127 else 02128 { 02129 state = 1; 02130 #if (SX1272_debug_mode > 1) 02131 printf("** There has been an error while getting bandwidth **"); 02132 printf("\n"); 02133 #endif 02134 } 02135 } 02136 return state; 02137 } 02138 02139 /* 02140 Function: Sets the indicated BW in the module. 02141 Returns: Integer that determines if there has been any error 02142 state = 2 --> The command has not been executed 02143 state = 1 --> There has been an error while executing the command 02144 state = 0 --> The command has been executed with no errors 02145 Parameters: 02146 band: bandwith value to set in LoRa modem configuration. 02147 */ 02148 int8_t SX1272::setBW(uint16_t band) 02149 { 02150 byte st0; 02151 int8_t state = 2; 02152 byte config1; 02153 02154 #if (SX1272_debug_mode > 1) 02155 printf("\n"); 02156 printf("Starting 'setBW'\n"); 02157 #endif 02158 02159 if(!isBW(band) ) 02160 { 02161 state = 1; 02162 #if (SX1272_debug_mode > 1) 02163 printf("** Bandwidth %X ",band); 02164 // Serial.print(band, HEX); 02165 printf(" is not a correct value **"); 02166 printf("\n"); 02167 #endif 02168 return state; 02169 } 02170 02171 st0 = readRegister(REG_OP_MODE); // Save the previous status 02172 02173 if( _modem == FSK ) 02174 { 02175 #if (SX1272_debug_mode > 1) 02176 printf("## Notice that FSK hasn't Bandwidth parameter, "); 02177 printf("so you are configuring it in LoRa mode ##"); 02178 #endif 02179 state = setLORA(); 02180 } 02181 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // LoRa standby mode 02182 config1 = (readRegister(REG_MODEM_CONFIG1)); // Save config1 to modify only the BW 02183 02184 // added by C. Pham for SX1276 02185 if (_board==SX1272Chip) { 02186 switch(band) 02187 { 02188 case BW_125: config1 = config1 & 0B00111111; // clears bits 7 & 6 from REG_MODEM_CONFIG1 02189 getSF(); 02190 if( _spreadingFactor == 11 ) 02191 { // LowDataRateOptimize (Mandatory with BW_125 if SF_11) 02192 config1 = config1 | 0B00000001; 02193 } 02194 if( _spreadingFactor == 12 ) 02195 { // LowDataRateOptimize (Mandatory with BW_125 if SF_12) 02196 config1 = config1 | 0B00000001; 02197 } 02198 break; 02199 case BW_250: config1 = config1 & 0B01111111; // clears bit 7 from REG_MODEM_CONFIG1 02200 config1 = config1 | 0B01000000; // sets bit 6 from REG_MODEM_CONFIG1 02201 break; 02202 case BW_500: config1 = config1 & 0B10111111; //clears bit 6 from REG_MODEM_CONFIG1 02203 config1 = config1 | 0B10000000; //sets bit 7 from REG_MODEM_CONFIG1 02204 break; 02205 } 02206 } 02207 else { 02208 // SX1276 02209 config1 = config1 & 0B00001111; // clears bits 7 - 4 from REG_MODEM_CONFIG1 02210 switch(band) 02211 { 02212 case BW_125: 02213 // 0111 02214 config1 = config1 | 0B01110000; 02215 getSF(); 02216 if( _spreadingFactor == 11 || _spreadingFactor == 12) 02217 { // LowDataRateOptimize (Mandatory with BW_125 if SF_11 or SF_12) 02218 byte config3=readRegister(REG_MODEM_CONFIG3); 02219 config3 = config3 | 0B00001000; 02220 writeRegister(REG_MODEM_CONFIG3,config3); 02221 } 02222 break; 02223 case BW_250: 02224 // 1000 02225 config1 = config1 | 0B10000000; 02226 break; 02227 case BW_500: 02228 // 1001 02229 config1 = config1 | 0B10010000; 02230 break; 02231 } 02232 } 02233 // end 02234 02235 writeRegister(REG_MODEM_CONFIG1,config1); // Update config1 02236 02237 wait_ms(100); 02238 02239 config1 = (readRegister(REG_MODEM_CONFIG1)); 02240 02241 // added by C. Pham 02242 if (_board==SX1272Chip) { 02243 // (config1 >> 6) ---> take out bits 7-6 from REG_MODEM_CONFIG1 (=_bandwidth) 02244 switch(band) 02245 { 02246 case BW_125: if( (config1 >> 6) == SX1272_BW_125 ) 02247 { 02248 state = 0; 02249 if( _spreadingFactor == 11 ) 02250 { 02251 if( bitRead(config1, 0) == 1 ) 02252 { // LowDataRateOptimize 02253 state = 0; 02254 } 02255 else 02256 { 02257 state = 1; 02258 } 02259 } 02260 if( _spreadingFactor == 12 ) 02261 { 02262 if( bitRead(config1, 0) == 1 ) 02263 { // LowDataRateOptimize 02264 state = 0; 02265 } 02266 else 02267 { 02268 state = 1; 02269 } 02270 } 02271 } 02272 break; 02273 case BW_250: if( (config1 >> 6) == SX1272_BW_250 ) 02274 { 02275 state = 0; 02276 } 02277 break; 02278 case BW_500: if( (config1 >> 6) == SX1272_BW_500 ) 02279 { 02280 state = 0; 02281 } 02282 break; 02283 } 02284 } 02285 else { 02286 // (config1 >> 4) ---> take out bits 7-4 from REG_MODEM_CONFIG1 (=_bandwidth) 02287 switch(band) 02288 { 02289 case BW_125: if( (config1 >> 4) == BW_125 ) 02290 { 02291 state = 0; 02292 02293 byte config3 = (readRegister(REG_MODEM_CONFIG3)); 02294 02295 if( _spreadingFactor == 11 ) 02296 { 02297 if( bitRead(config3, 3) == 1 ) 02298 { // LowDataRateOptimize 02299 state = 0; 02300 } 02301 else 02302 { 02303 state = 1; 02304 } 02305 } 02306 if( _spreadingFactor == 12 ) 02307 { 02308 if( bitRead(config3, 3) == 1 ) 02309 { // LowDataRateOptimize 02310 state = 0; 02311 } 02312 else 02313 { 02314 state = 1; 02315 } 02316 } 02317 } 02318 break; 02319 case BW_250: if( (config1 >> 4) == BW_250 ) 02320 { 02321 state = 0; 02322 } 02323 break; 02324 case BW_500: if( (config1 >> 4) == BW_500 ) 02325 { 02326 state = 0; 02327 } 02328 break; 02329 } 02330 } 02331 02332 if(state==0) 02333 { 02334 _bandwidth = band; 02335 #if (SX1272_debug_mode > 1) 02336 printf("## Bandwidth %X ",band); 02337 // Serial.print(band, HEX); 02338 printf(" has been successfully set ##"); 02339 printf("\n"); 02340 #endif 02341 } 02342 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 02343 wait_ms(100); 02344 return state; 02345 } 02346 02347 /* 02348 Function: Checks if CR is a valid value. 02349 Returns: Boolean that's 'true' if the CR value exists and 02350 it's 'false' if the CR value does not exist. 02351 Parameters: 02352 cod: coding rate value to check. 02353 */ 02354 boolean SX1272::isCR(uint8_t cod) 02355 { 02356 #if (SX1272_debug_mode > 1) 02357 printf("\n"); 02358 printf("Starting 'isCR'\n"); 02359 #endif 02360 02361 // Checking available values for _codingRate 02362 switch(cod) 02363 { 02364 case CR_5: 02365 case CR_6: 02366 case CR_7: 02367 case CR_8: 02368 return true; 02369 //break; 02370 02371 default: 02372 return false; 02373 } 02374 #if (SX1272_debug_mode > 1) 02375 printf("## Finished 'isCR' ##"); 02376 printf("\n"); 02377 #endif 02378 } 02379 02380 /* 02381 Function: Indicates the CR within the module is configured. 02382 Returns: Integer that determines if there has been any error 02383 state = 2 --> The command has not been executed 02384 state = 1 --> There has been an error while executing the command 02385 state = 0 --> The command has been executed with no errors 02386 state = -1 --> Forbidden command for this protocol 02387 */ 02388 int8_t SX1272::getCR() 02389 { 02390 int8_t state = 2; 02391 byte config1; 02392 02393 #if (SX1272_debug_mode > 1) 02394 printf("\n"); 02395 printf("Starting 'getCR'\n"); 02396 #endif 02397 02398 if( _modem == FSK ) 02399 { 02400 state = -1; // CR is not available in FSK mode 02401 #if (SX1272_debug_mode > 1) 02402 printf("** FSK mode hasn't coding rate **"); 02403 printf("\n"); 02404 #endif 02405 } 02406 else 02407 { 02408 // added by C. Pham 02409 if (_board==SX1272Chip) { 02410 // take out bits 7-3 from REG_MODEM_CONFIG1 indicates _bandwidth & _codingRate 02411 config1 = (readRegister(REG_MODEM_CONFIG1)) >> 3; 02412 config1 = config1 & 0B00000111; // clears bits 7-3 ---> clears _bandwidth 02413 } 02414 else { 02415 // take out bits 7-1 from REG_MODEM_CONFIG1 indicates _bandwidth & _codingRate 02416 config1 = (readRegister(REG_MODEM_CONFIG1)) >> 1; 02417 config1 = config1 & 0B00000111; // clears bits 7-3 ---> clears _bandwidth 02418 } 02419 02420 _codingRate = config1; 02421 state = 1; 02422 02423 if( (config1 == _codingRate) && isCR(_codingRate) ) 02424 { 02425 state = 0; 02426 #if (SX1272_debug_mode > 1) 02427 printf("## Coding rate is %X ",_codingRate); 02428 // Serial.print(_codingRate, HEX); 02429 printf(" ##"); 02430 printf("\n"); 02431 #endif 02432 } 02433 } 02434 return state; 02435 } 02436 02437 /* 02438 Function: Sets the indicated CR in the module. 02439 Returns: Integer that determines if there has been any error 02440 state = 2 --> The command has not been executed 02441 state = 1 --> There has been an error while executing the command 02442 state = 0 --> The command has been executed with no errors 02443 state = -1 --> Forbidden command for this protocol 02444 Parameters: 02445 cod: coding rate value to set in LoRa modem configuration. 02446 */ 02447 int8_t SX1272::setCR(uint8_t cod) 02448 { 02449 byte st0; 02450 int8_t state = 2; 02451 byte config1; 02452 02453 #if (SX1272_debug_mode > 1) 02454 printf("\n"); 02455 printf("Starting 'setCR'\n"); 02456 #endif 02457 02458 st0 = readRegister(REG_OP_MODE); // Save the previous status 02459 02460 if( _modem == FSK ) 02461 { 02462 #if (SX1272_debug_mode > 1) 02463 printf("## Notice that FSK hasn't Coding Rate parameter, "); 02464 printf("so you are configuring it in LoRa mode ##"); 02465 #endif 02466 state = setLORA(); 02467 } 02468 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Set Standby mode to write in registers 02469 02470 config1 = readRegister(REG_MODEM_CONFIG1); // Save config1 to modify only the CR 02471 02472 // added by C. Pham 02473 if (_board==SX1272Chip) { 02474 switch(cod) 02475 { 02476 case CR_5: config1 = config1 & 0B11001111; // clears bits 5 & 4 from REG_MODEM_CONFIG1 02477 config1 = config1 | 0B00001000; // sets bit 3 from REG_MODEM_CONFIG1 02478 break; 02479 case CR_6: config1 = config1 & 0B11010111; // clears bits 5 & 3 from REG_MODEM_CONFIG1 02480 config1 = config1 | 0B00010000; // sets bit 4 from REG_MODEM_CONFIG1 02481 break; 02482 case CR_7: config1 = config1 & 0B11011111; // clears bit 5 from REG_MODEM_CONFIG1 02483 config1 = config1 | 0B00011000; // sets bits 4 & 3 from REG_MODEM_CONFIG1 02484 break; 02485 case CR_8: config1 = config1 & 0B11100111; // clears bits 4 & 3 from REG_MODEM_CONFIG1 02486 config1 = config1 | 0B00100000; // sets bit 5 from REG_MODEM_CONFIG1 02487 break; 02488 } 02489 } 02490 else { 02491 // SX1276 02492 config1 = config1 & 0B11110001; // clears bits 3 - 1 from REG_MODEM_CONFIG1 02493 switch(cod) 02494 { 02495 case CR_5: 02496 config1 = config1 | 0B00000010; 02497 break; 02498 case CR_6: 02499 config1 = config1 | 0B00000100; 02500 break; 02501 case CR_7: 02502 config1 = config1 | 0B00000110; 02503 break; 02504 case CR_8: 02505 config1 = config1 | 0B00001000; 02506 break; 02507 } 02508 } 02509 writeRegister(REG_MODEM_CONFIG1, config1); // Update config1 02510 02511 wait_ms(100); 02512 02513 config1 = readRegister(REG_MODEM_CONFIG1); 02514 02515 // added by C. Pham 02516 uint8_t nshift=3; 02517 02518 // only 1 right shift for SX1276 02519 if (_board==SX1276Chip) 02520 nshift=1; 02521 02522 // ((config1 >> 3) & 0B0000111) ---> take out bits 5-3 from REG_MODEM_CONFIG1 (=_codingRate) 02523 switch(cod) 02524 { 02525 case CR_5: if( ((config1 >> nshift) & 0B0000111) == 0x01 ) 02526 { 02527 state = 0; 02528 } 02529 break; 02530 case CR_6: if( ((config1 >> nshift) & 0B0000111) == 0x02 ) 02531 { 02532 state = 0; 02533 } 02534 break; 02535 case CR_7: if( ((config1 >> nshift) & 0B0000111) == 0x03 ) 02536 { 02537 state = 0; 02538 } 02539 break; 02540 case CR_8: if( ((config1 >> nshift) & 0B0000111) == 0x04 ) 02541 { 02542 state = 0; 02543 } 02544 break; 02545 } 02546 02547 02548 if( isCR(cod) ) 02549 { 02550 _codingRate = cod; 02551 #if (SX1272_debug_mode > 1) 02552 printf("## Coding Rate %X ",cod); 02553 // Serial.print(cod, HEX); 02554 printf(" has been successfully set ##"); 02555 printf("\n"); 02556 #endif 02557 } 02558 else 02559 { 02560 state = 1; 02561 #if (SX1272_debug_mode > 1) 02562 printf("** There has been an error while configuring Coding Rate parameter **"); 02563 printf("\n"); 02564 #endif 02565 } 02566 writeRegister(REG_OP_MODE,st0); // Getting back to previous status 02567 wait_ms(100); 02568 return state; 02569 } 02570 02571 /* 02572 Function: Checks if channel is a valid value. 02573 Returns: Boolean that's 'true' if the CR value exists and 02574 it's 'false' if the CR value does not exist. 02575 Parameters: 02576 ch: frequency channel value to check. 02577 */ 02578 boolean SX1272::isChannel(uint32_t ch) 02579 { 02580 #if (SX1272_debug_mode > 1) 02581 printf("\n"); 02582 printf("Starting 'isChannel'\n"); 02583 #endif 02584 02585 // Checking available values for _channel 02586 switch(ch) 02587 { 02588 //added by C. Pham 02589 case CH_04_868: 02590 case CH_05_868: 02591 case CH_06_868: 02592 case CH_07_868: 02593 case CH_08_868: 02594 case CH_09_868: 02595 //end 02596 case CH_10_868: 02597 case CH_11_868: 02598 case CH_12_868: 02599 case CH_13_868: 02600 case CH_14_868: 02601 case CH_15_868: 02602 case CH_16_868: 02603 case CH_17_868: 02604 //added by C. Pham 02605 case CH_18_868: 02606 //end 02607 case CH_00_900: 02608 case CH_01_900: 02609 case CH_02_900: 02610 case CH_03_900: 02611 case CH_04_900: 02612 case CH_05_900: 02613 case CH_06_900: 02614 case CH_07_900: 02615 case CH_08_900: 02616 case CH_09_900: 02617 case CH_10_900: 02618 case CH_11_900: 02619 //added by C. Pham 02620 case CH_12_900: 02621 case CH_00_433: 02622 case CH_01_433: 02623 case CH_02_433: 02624 case CH_03_433: 02625 //end 02626 return true; 02627 //break; 02628 02629 default: 02630 return false; 02631 } 02632 #if (SX1272_debug_mode > 1) 02633 printf("## Finished 'isChannel' ##"); 02634 printf("\n"); 02635 #endif 02636 } 02637 02638 /* 02639 Function: Indicates the frequency channel within the module is configured. 02640 Returns: Integer that determines if there has been any error 02641 state = 2 --> The command has not been executed 02642 state = 1 --> There has been an error while executing the command 02643 state = 0 --> The command has been executed with no errors 02644 */ 02645 uint8_t SX1272::getChannel() 02646 { 02647 uint8_t state = 2; 02648 uint32_t ch; 02649 uint8_t freq3; 02650 uint8_t freq2; 02651 uint8_t freq1; 02652 02653 #if (SX1272_debug_mode > 1) 02654 printf("\n"); 02655 printf("Starting 'getChannel'\n"); 02656 #endif 02657 02658 freq3 = readRegister(REG_FRF_MSB); // frequency channel MSB 02659 freq2 = readRegister(REG_FRF_MID); // frequency channel MID 02660 freq1 = readRegister(REG_FRF_LSB); // frequency channel LSB 02661 ch = ((uint32_t)freq3 << 16) + ((uint32_t)freq2 << 8) + (uint32_t)freq1; 02662 _channel = ch; // frequency channel 02663 02664 if( (_channel == ch) && isChannel(_channel) ) 02665 { 02666 state = 0; 02667 #if (SX1272_debug_mode > 1) 02668 printf("## Frequency channel is %d ", _channel); 02669 // Serial.print(_channel, HEX); 02670 printf(" ##"); 02671 printf("\n"); 02672 #endif 02673 } 02674 else 02675 { 02676 state = 1; 02677 } 02678 return state; 02679 } 02680 02681 /* 02682 Function: Sets the indicated channel in the module. 02683 Returns: Integer that determines if there has been any error 02684 state = 2 --> The command has not been executed 02685 state = 1 --> There has been an error while executing the command 02686 state = 0 --> The command has been executed with no errors 02687 state = -1 --> Forbidden command for this protocol 02688 Parameters: 02689 ch: frequency channel value to set in configuration. 02690 */ 02691 int8_t SX1272::setChannel(uint32_t ch) 02692 { 02693 byte st0; 02694 int8_t state = 2; 02695 unsigned int freq3; 02696 unsigned int freq2; 02697 uint8_t freq1; 02698 uint32_t freq; 02699 02700 #if (SX1272_debug_mode > 1) 02701 printf("\n"); 02702 printf("Starting 'setChannel'\n"); 02703 #endif 02704 02705 // added by C. Pham 02706 _starttime=millis(); 02707 02708 st0 = readRegister(REG_OP_MODE); // Save the previous status 02709 if( _modem == LORA ) 02710 { 02711 // LoRa Stdby mode in order to write in registers 02712 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); 02713 } 02714 else 02715 { 02716 // FSK Stdby mode in order to write in registers 02717 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); 02718 } 02719 02720 freq3 = ((ch >> 16) & 0x0FF); // frequency channel MSB 02721 freq2 = ((ch >> 8) & 0x0FF); // frequency channel MIB 02722 freq1 = (ch & 0xFF); // frequency channel LSB 02723 02724 writeRegister(REG_FRF_MSB, freq3); 02725 writeRegister(REG_FRF_MID, freq2); 02726 writeRegister(REG_FRF_LSB, freq1); 02727 02728 // added by C. Pham 02729 _stoptime=millis(); 02730 02731 wait_ms(100); 02732 02733 // storing MSB in freq channel value 02734 freq3 = (readRegister(REG_FRF_MSB)); 02735 freq = (freq3 << 8) & 0xFFFFFF; 02736 02737 // storing MID in freq channel value 02738 freq2 = (readRegister(REG_FRF_MID)); 02739 freq = (freq << 8) + ((freq2 << 8) & 0xFFFFFF); 02740 02741 // storing LSB in freq channel value 02742 freq = freq + ((readRegister(REG_FRF_LSB)) & 0xFFFFFF); 02743 02744 if( freq == ch ) 02745 { 02746 state = 0; 02747 _channel = ch; 02748 #if (SX1272_debug_mode > 1) 02749 printf("## Frequency channel %X ",ch); 02750 // Serial.print(ch, HEX); 02751 printf(" has been successfully set ##"); 02752 printf("\n"); 02753 #endif 02754 } 02755 else 02756 { 02757 state = 1; 02758 } 02759 02760 // commented by C. Pham to avoid adding new channel each time 02761 // besides, the test above is sufficient 02762 /* 02763 if(!isChannel(ch) ) 02764 { 02765 state = -1; 02766 #if (SX1272_debug_mode > 1) 02767 printf("** Frequency channel "); 02768 Serial.print(ch, HEX); 02769 printf("is not a correct value **"); 02770 printf("\n"); 02771 #endif 02772 } 02773 */ 02774 02775 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 02776 wait_ms(100); 02777 return state; 02778 } 02779 02780 /* 02781 Function: Gets the signal power within the module is configured. 02782 Returns: Integer that determines if there has been any error 02783 state = 2 --> The command has not been executed 02784 state = 1 --> There has been an error while executing the command 02785 state = 0 --> The command has been executed with no errors 02786 */ 02787 uint8_t SX1272::getPower() 02788 { 02789 uint8_t state = 2; 02790 int8_t value = 0x00; // modifie par C.Dupaty type byte a l'origine 02791 02792 #if (SX1272_debug_mode > 1) 02793 printf("\n"); 02794 printf("Starting 'getPower'\n"); 02795 #endif 02796 02797 value = readRegister(REG_PA_CONFIG); 02798 state = 1; 02799 02800 // modified by C. Pham 02801 // get only the OutputPower 02802 _power = value & 0B00001111; 02803 02804 if( (value > -1) & (value < 16) ) 02805 { 02806 state = 0; 02807 #if (SX1272_debug_mode > 1) 02808 printf("## Output power is %X ",_power); 02809 // Serial.print(_power, HEX); 02810 printf(" ##"); 02811 printf("\n"); 02812 #endif 02813 } 02814 02815 return state; 02816 } 02817 02818 /* 02819 Function: Sets the signal power indicated in the module. 02820 Returns: Integer that determines if there has been any error 02821 state = 2 --> The command has not been executed 02822 state = 1 --> There has been an error while executing the command 02823 state = 0 --> The command has been executed with no errors 02824 state = -1 --> Forbidden command for this protocol 02825 Parameters: 02826 p: power option to set in configuration. 02827 */ 02828 int8_t SX1272::setPower(char p) 02829 { 02830 byte st0; 02831 int8_t state = 2; 02832 byte value = 0x00; 02833 02834 byte RegPaDacReg=(_board==SX1272Chip)?0x5A:0x4D; 02835 02836 #if (SX1272_debug_mode > 1) 02837 printf("\n"); 02838 printf("Starting 'setPower'\n"); 02839 #endif 02840 02841 st0 = readRegister(REG_OP_MODE); // Save the previous status 02842 if( _modem == LORA ) 02843 { // LoRa Stdby mode to write in registers 02844 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); 02845 } 02846 else 02847 { // FSK Stdby mode to write in registers 02848 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); 02849 } 02850 02851 switch (p) 02852 { 02853 // L = Low. On SX1272/76: PA0 on RFO setting 02854 // H = High. On SX1272/76: PA0 on RFO setting 02855 // M = MAX. On SX1272/76: PA0 on RFO setting 02856 02857 // x = extreme; added by C. Pham. On SX1272/76: PA1&PA2 PA_BOOST setting 02858 // X = eXtreme; added by C. Pham. On SX1272/76: PA1&PA2 PA_BOOST setting + 20dBm settings 02859 02860 // added by C. Pham 02861 // 02862 case 'x': 02863 case 'X': 02864 case 'M': value = 0x0F; 02865 // SX1272/76: 14dBm 02866 break; 02867 02868 // modified by C. Pham, set to 0x03 instead of 0x00 02869 case 'L': value = 0x03; 02870 // SX1272/76: 2dBm 02871 break; 02872 02873 case 'H': value = 0x07; 02874 // SX1272/76: 6dBm 02875 break; 02876 02877 default: state = -1; 02878 break; 02879 } 02880 02881 // 100mA 02882 setMaxCurrent(0x0B); 02883 02884 if (p=='x') { 02885 // we set only the PA_BOOST pin 02886 // limit to 14dBm 02887 value = 0x0C; 02888 value = value & 0B10000000; 02889 // set RegOcp for OcpOn and OcpTrim 02890 // 130mA 02891 setMaxCurrent(0x10); 02892 } 02893 02894 if (p=='X') { 02895 // normally value = 0x0F; 02896 // we set the PA_BOOST pin 02897 value = value & 0B10000000; 02898 // and then set the high output power config with register REG_PA_DAC 02899 writeRegister(RegPaDacReg, 0x87); 02900 // set RegOcp for OcpOn and OcpTrim 02901 // 150mA 02902 setMaxCurrent(0x12); 02903 } 02904 else { 02905 // disable high power output in all other cases 02906 writeRegister(RegPaDacReg, 0x84); 02907 } 02908 02909 // added by C. Pham 02910 if (_board==SX1272Chip) { 02911 // Pout = -1 + _power[3:0] on RFO 02912 // Pout = 2 + _power[3:0] on PA_BOOST 02913 // so: L=2dBm; H=6dBm, M=14dBm, x=14dBm (PA), X=20dBm(PA+PADAC) 02914 writeRegister(REG_PA_CONFIG, value); // Setting output power value 02915 } 02916 else { 02917 // for the SX1276 02918 02919 // set MaxPower to 7 -> Pmax=10.8+0.6*MaxPower [dBm] = 15 02920 value = value & 0B01110000; 02921 02922 // then Pout = Pmax-(15-_power[3:0]) if PaSelect=0 (RFO pin for +14dBm) 02923 // so L=3dBm; H=7dBm; M=15dBm (but should be limited to 14dBm by RFO pin) 02924 02925 // and Pout = 17-(15-_power[3:0]) if PaSelect=1 (PA_BOOST pin for +14dBm) 02926 // so x= 14dBm (PA); 02927 // when p=='X' for 20dBm, value is 0x0F and RegPaDacReg=0x87 so 20dBm is enabled 02928 02929 writeRegister(REG_PA_CONFIG, value); 02930 } 02931 02932 _power=value; 02933 02934 value = readRegister(REG_PA_CONFIG); 02935 02936 if( value == _power ) 02937 { 02938 state = 0; 02939 #if (SX1272_debug_mode > 1) 02940 printf("## Output power has been successfully set ##"); 02941 printf("\n"); 02942 #endif 02943 } 02944 else 02945 { 02946 state = 1; 02947 } 02948 02949 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 02950 wait_ms(100); 02951 return state; 02952 } 02953 02954 /* 02955 Function: Sets the signal power indicated in the module. 02956 Returns: Integer that determines if there has been any error 02957 state = 2 --> The command has not been executed 02958 state = 1 --> There has been an error while executing the command 02959 state = 0 --> The command has been executed with no errors 02960 state = -1 --> Forbidden command for this protocol 02961 Parameters: 02962 p: power option to set in configuration. 02963 */ 02964 int8_t SX1272::setPowerNum(uint8_t pow) 02965 { 02966 byte st0; 02967 int8_t state = 2; 02968 byte value = 0x00; 02969 02970 #if (SX1272_debug_mode > 1) 02971 printf("\n"); 02972 printf("Starting 'setPower'\n"); 02973 #endif 02974 02975 st0 = readRegister(REG_OP_MODE); // Save the previous status 02976 if( _modem == LORA ) 02977 { // LoRa Stdby mode to write in registers 02978 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); 02979 } 02980 else 02981 { // FSK Stdby mode to write in registers 02982 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); 02983 } 02984 02985 // Modifie par C.Dupaty 02986 // if ( (pow >= 0) & (pow < 15) ) 02987 if (pow < 15) 02988 { 02989 _power = pow; 02990 } 02991 else 02992 { 02993 state = -1; 02994 #if (SX1272_debug_mode > 1) 02995 printf("## Power value is not valid ##"); 02996 printf("\n"); 02997 #endif 02998 } 02999 03000 // added by C. Pham 03001 if (_board==SX1276Chip) { 03002 value=readRegister(REG_PA_CONFIG); 03003 // clear OutputPower, but keep current value of PaSelect and MaxPower 03004 value=value & 0B11110000; 03005 value=value + _power; 03006 _power=value; 03007 } 03008 writeRegister(REG_PA_CONFIG, _power); // Setting output power value 03009 value = readRegister(REG_PA_CONFIG); 03010 03011 if( value == _power ) 03012 { 03013 state = 0; 03014 #if (SX1272_debug_mode > 1) 03015 printf("## Output power has been successfully set ##"); 03016 printf("\n"); 03017 #endif 03018 } 03019 else 03020 { 03021 state = 1; 03022 } 03023 03024 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 03025 wait_ms(100); 03026 return state; 03027 } 03028 03029 03030 /* 03031 Function: Gets the preamble length from the module. 03032 Returns: Integer that determines if there has been any error 03033 state = 2 --> The command has not been executed 03034 state = 1 --> There has been an error while executing the command 03035 state = 0 --> The command has been executed with no errors 03036 */ 03037 uint8_t SX1272::getPreambleLength() 03038 { 03039 int8_t state = 2; 03040 uint8_t p_length; 03041 03042 #if (SX1272_debug_mode > 1) 03043 printf("\n"); 03044 printf("Starting 'getPreambleLength'\n"); 03045 #endif 03046 03047 state = 1; 03048 if( _modem == LORA ) 03049 { // LORA mode 03050 p_length = readRegister(REG_PREAMBLE_MSB_LORA); 03051 // Saving MSB preamble length in LoRa mode 03052 _preamblelength = (p_length << 8) & 0xFFFF; 03053 p_length = readRegister(REG_PREAMBLE_LSB_LORA); 03054 // Saving LSB preamble length in LoRa mode 03055 _preamblelength = _preamblelength + (p_length & 0xFFFF); 03056 #if (SX1272_debug_mode > 1) 03057 printf("## Preamble length configured is %X ",_preamblelength); 03058 // Serial.print(_preamblelength, HEX); 03059 printf(" ##"); 03060 printf("\n"); 03061 #endif 03062 } 03063 else 03064 { // FSK mode 03065 p_length = readRegister(REG_PREAMBLE_MSB_FSK); 03066 // Saving MSB preamble length in FSK mode 03067 _preamblelength = (p_length << 8) & 0xFFFF; 03068 p_length = readRegister(REG_PREAMBLE_LSB_FSK); 03069 // Saving LSB preamble length in FSK mode 03070 _preamblelength = _preamblelength + (p_length & 0xFFFF); 03071 #if (SX1272_debug_mode > 1) 03072 printf("## Preamble length configured is %X ",_preamblelength); 03073 // Serial.print(_preamblelength, HEX); 03074 printf(" ##"); 03075 printf("\n"); 03076 #endif 03077 } 03078 state = 0; 03079 return state; 03080 } 03081 03082 /* 03083 Function: Sets the preamble length in the module 03084 Returns: Integer that determines if there has been any error 03085 state = 2 --> The command has not been executed 03086 state = 1 --> There has been an error while executing the command 03087 state = 0 --> The command has been executed with no errors 03088 Parameters: 03089 l: length value to set as preamble length. 03090 */ 03091 uint8_t SX1272::setPreambleLength(uint16_t l) 03092 { 03093 byte st0; 03094 uint8_t p_length; 03095 int8_t state = 2; 03096 03097 #if (SX1272_debug_mode > 1) 03098 printf("\n"); 03099 printf("Starting 'setPreambleLength'\n"); 03100 #endif 03101 03102 st0 = readRegister(REG_OP_MODE); // Save the previous status 03103 state = 1; 03104 if( _modem == LORA ) 03105 { // LoRa mode 03106 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Set Standby mode to write in registers 03107 p_length = ((l >> 8) & 0x0FF); 03108 // Storing MSB preamble length in LoRa mode 03109 writeRegister(REG_PREAMBLE_MSB_LORA, p_length); 03110 p_length = (l & 0x0FF); 03111 // Storing LSB preamble length in LoRa mode 03112 writeRegister(REG_PREAMBLE_LSB_LORA, p_length); 03113 } 03114 else 03115 { // FSK mode 03116 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Set Standby mode to write in registers 03117 p_length = ((l >> 8) & 0x0FF); 03118 // Storing MSB preamble length in FSK mode 03119 writeRegister(REG_PREAMBLE_MSB_FSK, p_length); 03120 p_length = (l & 0x0FF); 03121 // Storing LSB preamble length in FSK mode 03122 writeRegister(REG_PREAMBLE_LSB_FSK, p_length); 03123 } 03124 03125 state = 0; 03126 #if (SX1272_debug_mode > 1) 03127 printf("## Preamble length %X ",l); 03128 // Serial.print(l, HEX); 03129 printf(" has been successfully set ##"); 03130 printf("\n"); 03131 #endif 03132 03133 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 03134 wait_ms(100); 03135 return state; 03136 } 03137 03138 /* 03139 Function: Gets the payload length from the module. 03140 Returns: Integer that determines if there has been any error 03141 state = 2 --> The command has not been executed 03142 state = 1 --> There has been an error while executing the command 03143 state = 0 --> The command has been executed with no errors 03144 */ 03145 uint8_t SX1272::getPayloadLength() 03146 { 03147 uint8_t state = 2; 03148 03149 #if (SX1272_debug_mode > 1) 03150 printf("\n"); 03151 printf("Starting 'getPayloadLength'\n"); 03152 #endif 03153 03154 if( _modem == LORA ) 03155 { // LORA mode 03156 // Saving payload length in LoRa mode 03157 _payloadlength = readRegister(REG_PAYLOAD_LENGTH_LORA); 03158 state = 1; 03159 } 03160 else 03161 { // FSK mode 03162 // Saving payload length in FSK mode 03163 _payloadlength = readRegister(REG_PAYLOAD_LENGTH_FSK); 03164 state = 1; 03165 } 03166 03167 #if (SX1272_debug_mode > 1) 03168 printf("## Payload length configured is %X ",_payloadlength); 03169 // Serial.print(_payloadlength, HEX); 03170 printf(" ##"); 03171 printf("\n"); 03172 #endif 03173 03174 state = 0; 03175 return state; 03176 } 03177 03178 /* 03179 Function: Sets the packet length in the module. 03180 Returns: Integer that determines if there has been any error 03181 state = 2 --> The command has not been executed 03182 state = 1 --> There has been an error while executing the command 03183 state = 0 --> The command has been executed with no errors 03184 state = -1 --> Forbidden command for this protocol 03185 */ 03186 int8_t SX1272::setPacketLength() 03187 { 03188 uint16_t length; 03189 03190 // added by C. Pham 03191 // if gateway is in rawFormat mode for packet reception, it will also send in rawFormat 03192 // unless we switch it back to normal format just for transmission, e.g. for downlink transmission 03193 if (_rawFormat) 03194 length = _payloadlength; 03195 else 03196 length = _payloadlength + OFFSET_PAYLOADLENGTH; 03197 03198 return setPacketLength(length); 03199 } 03200 03201 /* 03202 Function: Sets the packet length in the module. 03203 Returns: Integer that determines if there has been any error 03204 state = 2 --> The command has not been executed 03205 state = 1 --> There has been an error while executing the command 03206 state = 0 --> The command has been executed with no errors 03207 state = -1 --> Forbidden command for this protocol 03208 Parameters: 03209 l: length value to set as payload length. 03210 */ 03211 int8_t SX1272::setPacketLength(uint8_t l) 03212 { 03213 byte st0; 03214 byte value = 0x00; 03215 int8_t state = 2; 03216 03217 #if (SX1272_debug_mode > 1) 03218 printf("\n"); 03219 printf("Starting 'setPacketLength'\n"); 03220 #endif 03221 03222 st0 = readRegister(REG_OP_MODE); // Save the previous status 03223 packet_sent.length = l; 03224 03225 if( _modem == LORA ) 03226 { // LORA mode 03227 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Set LoRa Standby mode to write in registers 03228 writeRegister(REG_PAYLOAD_LENGTH_LORA, packet_sent.length); 03229 // Storing payload length in LoRa mode 03230 value = readRegister(REG_PAYLOAD_LENGTH_LORA); 03231 } 03232 else 03233 { // FSK mode 03234 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Set FSK Standby mode to write in registers 03235 writeRegister(REG_PAYLOAD_LENGTH_FSK, packet_sent.length); 03236 // Storing payload length in FSK mode 03237 value = readRegister(REG_PAYLOAD_LENGTH_FSK); 03238 } 03239 03240 if( packet_sent.length == value ) 03241 { 03242 state = 0; 03243 #if (SX1272_debug_mode > 1) 03244 printf("## Packet length %d ",packet_sent.length); 03245 // Serial.print(packet_sent.length, DEC); 03246 printf(" has been successfully set ##"); 03247 printf("\n"); 03248 #endif 03249 } 03250 else 03251 { 03252 state = 1; 03253 } 03254 03255 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 03256 // comment by C. Pham 03257 // this delay is included in the send delay overhead 03258 // TODO: do we really need this delay? 03259 wait_ms(250); 03260 return state; 03261 } 03262 03263 /* 03264 Function: Gets the node address in the module. 03265 Returns: Integer that determines if there has been any error 03266 state = 2 --> The command has not been executed 03267 state = 1 --> There has been an error while executing the command 03268 state = 0 --> The command has been executed with no errors 03269 */ 03270 uint8_t SX1272::getNodeAddress() 03271 { 03272 byte st0 = 0; 03273 uint8_t state = 2; 03274 03275 #if (SX1272_debug_mode > 1) 03276 printf("\n"); 03277 printf("Starting 'getNodeAddress'\n"); 03278 #endif 03279 03280 if( _modem == LORA ) 03281 { // LoRa mode 03282 st0 = readRegister(REG_OP_MODE); // Save the previous status 03283 // Allowing access to FSK registers while in LoRa standby mode 03284 writeRegister(REG_OP_MODE, LORA_STANDBY_FSK_REGS_MODE); 03285 } 03286 03287 // Saving node address 03288 _nodeAddress = readRegister(REG_NODE_ADRS); 03289 state = 1; 03290 03291 if( _modem == LORA ) 03292 { 03293 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 03294 } 03295 03296 state = 0; 03297 #if (SX1272_debug_mode > 1) 03298 printf("## Node address configured is %d ", _nodeAddress); 03299 // Serial.print(_nodeAddress); 03300 printf(" ##"); 03301 printf("\n"); 03302 #endif 03303 return state; 03304 } 03305 03306 /* 03307 Function: Sets the node address in the module. 03308 Returns: Integer that determines if there has been any error 03309 state = 2 --> The command has not been executed 03310 state = 1 --> There has been an error while executing the command 03311 state = 0 --> The command has been executed with no errors 03312 state = -1 --> Forbidden command for this protocol 03313 Parameters: 03314 addr: address value to set as node address. 03315 */ 03316 int8_t SX1272::setNodeAddress(uint8_t addr) 03317 { 03318 byte st0; 03319 uint8_t value; // type byte a l origine. Modifie par C.Dupaty 03320 int8_t state = 2; // type uint_8 a l origine 03321 03322 #if (SX1272_debug_mode > 1) 03323 printf("\n"); 03324 printf("Starting 'setNodeAddress'\n"); 03325 #endif 03326 03327 if( addr > 255 ) 03328 { 03329 state = -1; 03330 #if (SX1272_debug_mode > 1) 03331 printf("** Node address must be less than 255 **"); 03332 printf("\n"); 03333 #endif 03334 } 03335 else 03336 { 03337 // Saving node address 03338 _nodeAddress = addr; 03339 st0 = readRegister(REG_OP_MODE); // Save the previous status 03340 03341 if( _modem == LORA ) 03342 { // Allowing access to FSK registers while in LoRa standby mode 03343 writeRegister(REG_OP_MODE, LORA_STANDBY_FSK_REGS_MODE); 03344 } 03345 else 03346 { //Set FSK Standby mode to write in registers 03347 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); 03348 } 03349 03350 // Storing node and broadcast address 03351 writeRegister(REG_NODE_ADRS, addr); 03352 writeRegister(REG_BROADCAST_ADRS, BROADCAST_0); 03353 03354 value = readRegister(REG_NODE_ADRS); 03355 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 03356 03357 if( value == _nodeAddress ) 03358 { 03359 state = 0; 03360 #if (SX1272_debug_mode > 1) 03361 printf("## Node address %d ",addr); 03362 // Serial.print(addr); 03363 printf(" has been successfully set ##"); 03364 printf("\n"); 03365 #endif 03366 } 03367 else 03368 { 03369 state = 1; 03370 #if (SX1272_debug_mode > 1) 03371 printf("** There has been an error while setting address ##"); 03372 printf("\n"); 03373 #endif 03374 } 03375 } 03376 return state; 03377 } 03378 03379 /* 03380 Function: Gets the SNR value in LoRa mode. 03381 Returns: Integer that determines if there has been any error 03382 state = 2 --> The command has not been executed 03383 state = 1 --> There has been an error while executing the command 03384 state = 0 --> The command has been executed with no errors 03385 state = -1 --> Forbidden command for this protocol 03386 */ 03387 int8_t SX1272::getSNR() 03388 { // getSNR exists only in LoRa mode 03389 int8_t state = 2; 03390 byte value; 03391 03392 #if (SX1272_debug_mode > 1) 03393 printf("\n"); 03394 printf("Starting 'getSNR'\n"); 03395 #endif 03396 03397 if( _modem == LORA ) 03398 { // LoRa mode 03399 state = 1; 03400 value = readRegister(REG_PKT_SNR_VALUE); 03401 _rawSNR = value; 03402 03403 if( value & 0x80 ) // The SNR sign bit is 1 03404 { 03405 // Invert and divide by 4 03406 value = ( ( ~value + 1 ) & 0xFF ) >> 2; 03407 _SNR = -value; 03408 } 03409 else 03410 { 03411 // Divide by 4 03412 _SNR = ( value & 0xFF ) >> 2; 03413 } 03414 state = 0; 03415 #if (SX1272_debug_mode > 0) 03416 printf("## SNR value is %d\n",_SNR); 03417 // Serial.print(_SNR, DEC); 03418 printf(" ##"); 03419 printf("\n"); 03420 #endif 03421 } 03422 else 03423 { // forbidden command if FSK mode 03424 state = -1; 03425 #if (SX1272_debug_mode > 0) 03426 printf("** SNR does not exist in FSK mode **"); 03427 printf("\n"); 03428 #endif 03429 } 03430 return state; 03431 } 03432 03433 /* 03434 Function: Gets the current value of RSSI. 03435 Returns: Integer that determines if there has been any error 03436 state = 2 --> The command has not been executed 03437 state = 1 --> There has been an error while executing the command 03438 state = 0 --> The command has been executed with no errors 03439 */ 03440 uint8_t SX1272::getRSSI() 03441 { 03442 uint8_t state = 2; 03443 int rssi_mean = 0; 03444 int total = 5; 03445 03446 #if (SX1272_debug_mode > 1) 03447 printf("\n"); 03448 printf("Starting 'getRSSI'\n"); 03449 #endif 03450 03451 if( _modem == LORA ) 03452 { 03453 /// LoRa mode 03454 // get mean value of RSSI 03455 for(int i = 0; i < total; i++) 03456 { 03457 // modified by C. Pham 03458 // with SX1276 we have to add 18 to OFFSET_RSSI to obtain -157 03459 _RSSI = -(OFFSET_RSSI+(_board==SX1276Chip?18:0)) + readRegister(REG_RSSI_VALUE_LORA); 03460 rssi_mean += _RSSI; 03461 } 03462 03463 rssi_mean = rssi_mean / total; 03464 _RSSI = rssi_mean; 03465 03466 state = 0; 03467 #if (SX1272_debug_mode > 0) 03468 printf("## RSSI value is %d",_RSSI); 03469 // Serial.print(_RSSI, DEC); 03470 printf(" ##"); 03471 printf("\n"); 03472 #endif 03473 } 03474 else 03475 { 03476 /// FSK mode 03477 // get mean value of RSSI 03478 for(int i = 0; i < total; i++) 03479 { 03480 _RSSI = (readRegister(REG_RSSI_VALUE_FSK) >> 1); 03481 rssi_mean += _RSSI; 03482 } 03483 rssi_mean = rssi_mean / total; 03484 _RSSI = rssi_mean; 03485 03486 state = 0; 03487 03488 #if (SX1272_debug_mode > 0) 03489 printf("## RSSI value is %d ",_RSSI); 03490 //Serial.print(_RSSI); 03491 printf(" ##"); 03492 printf("\n"); 03493 #endif 03494 } 03495 return state; 03496 } 03497 03498 /* 03499 Function: Gets the RSSI of the last packet received in LoRa mode. 03500 Returns: Integer that determines if there has been any error 03501 state = 2 --> The command has not been executed 03502 state = 1 --> There has been an error while executing the command 03503 state = 0 --> The command has been executed with no errors 03504 state = -1 --> Forbidden command for this protocol 03505 */ 03506 int16_t SX1272::getRSSIpacket() 03507 { // RSSIpacket only exists in LoRa 03508 int8_t state = 2; 03509 03510 #if (SX1272_debug_mode > 1) 03511 printf("\n"); 03512 printf("Starting 'getRSSIpacket'\n"); 03513 #endif 03514 03515 state = 1; 03516 if( _modem == LORA ) 03517 { // LoRa mode 03518 state = getSNR(); 03519 if( state == 0 ) 03520 { 03521 // added by C. Pham 03522 _RSSIpacket = readRegister(REG_PKT_RSSI_VALUE); 03523 03524 if( _SNR < 0 ) 03525 { 03526 // commented by C. Pham 03527 //_RSSIpacket = -NOISE_ABSOLUTE_ZERO + 10.0 * SignalBwLog[_bandwidth] + NOISE_FIGURE + ( double )_SNR; 03528 03529 // added by C. Pham, using Semtech SX1272 rev3 March 2015 03530 // for SX1272 we use -139, for SX1276, we use -157 03531 // then for SX1276 when using low-frequency (i.e. 433MHz) then we use -164 03532 _RSSIpacket = -(OFFSET_RSSI+(_board==SX1276Chip?18:0)+(_channel<CH_04_868?7:0)) + (double)_RSSIpacket + (double)_rawSNR*0.25; 03533 state = 0; 03534 } 03535 else 03536 { 03537 // commented by C. Pham 03538 //_RSSIpacket = readRegister(REG_PKT_RSSI_VALUE); 03539 _RSSIpacket = -(OFFSET_RSSI+(_board==SX1276Chip?18:0)+(_channel<CH_04_868?7:0)) + (double)_RSSIpacket; 03540 //end 03541 state = 0; 03542 } 03543 #if (SX1272_debug_mode > 0) 03544 printf("## RSSI packet value is %d",_RSSIpacket); 03545 // Serial.print(_RSSIpacket, DEC); 03546 printf(" ##"); 03547 printf("\n"); 03548 #endif 03549 } 03550 } 03551 else 03552 { // RSSI packet doesn't exist in FSK mode 03553 state = -1; 03554 #if (SX1272_debug_mode > 0) 03555 printf("** RSSI packet does not exist in FSK mode **"); 03556 printf("\n"); 03557 #endif 03558 } 03559 return state; 03560 } 03561 03562 /* 03563 Function: It sets the maximum number of retries. 03564 Returns: Integer that determines if there has been any error 03565 state = 2 --> The command has not been executed 03566 state = 1 --> There has been an error while executing the command 03567 state = 0 --> The command has been executed with no errors 03568 state = -1 --> 03569 */ 03570 uint8_t SX1272::setRetries(uint8_t ret) 03571 { 03572 int8_t state = 2; // uint8_t a l origine 03573 03574 #if (SX1272_debug_mode > 1) 03575 printf("\n"); 03576 printf("Starting 'setRetries'\n"); 03577 #endif 03578 03579 state = 1; 03580 if( ret > MAX_RETRIES ) 03581 { 03582 state = -1; 03583 #if (SX1272_debug_mode > 1) 03584 printf("** Retries value can't be greater than %d ",MAX_RETRIES ); 03585 // Serial.print(MAX_RETRIES, DEC); 03586 printf(" **"); 03587 printf("\n"); 03588 #endif 03589 } 03590 else 03591 { 03592 _maxRetries = ret; 03593 state = 0; 03594 #if (SX1272_debug_mode > 1) 03595 printf("## Maximum retries value = %d ",_maxRetries); 03596 // Serial.print(_maxRetries, DEC); 03597 printf(" ##"); 03598 printf("\n"); 03599 #endif 03600 } 03601 return state; 03602 } 03603 03604 /* 03605 Function: Gets the current supply limit of the power amplifier, protecting battery chemistries. 03606 Returns: Integer that determines if there has been any error 03607 state = 2 --> The command has not been executed 03608 state = 1 --> There has been an error while executing the command 03609 state = 0 --> The command has been executed with no errors 03610 Parameters: 03611 rate: value to compute the maximum current supply. Maximum current is 45+5*'rate' [mA] 03612 */ 03613 uint8_t SX1272::getMaxCurrent() 03614 { 03615 int8_t state = 2; 03616 byte value; 03617 03618 #if (SX1272_debug_mode > 1) 03619 printf("\n"); 03620 printf("Starting 'getMaxCurrent'\n"); 03621 #endif 03622 03623 state = 1; 03624 _maxCurrent = readRegister(REG_OCP); 03625 03626 // extract only the OcpTrim value from the OCP register 03627 _maxCurrent &= 0B00011111; 03628 03629 if( _maxCurrent <= 15 ) 03630 { 03631 value = (45 + (5 * _maxCurrent)); 03632 } 03633 else if( _maxCurrent <= 27 ) 03634 { 03635 value = (-30 + (10 * _maxCurrent)); 03636 } 03637 else 03638 { 03639 value = 240; 03640 } 03641 03642 _maxCurrent = value; 03643 #if (SX1272_debug_mode > 1) 03644 printf("## Maximum current supply configured is %d ",value); 03645 // Serial.print(value, DEC); 03646 printf(" mA ##"); 03647 printf("\n"); 03648 #endif 03649 state = 0; 03650 return state; 03651 } 03652 03653 /* 03654 Function: Limits the current supply of the power amplifier, protecting battery chemistries. 03655 Returns: Integer that determines if there has been any error 03656 state = 2 --> The command has not been executed 03657 state = 1 --> There has been an error while executing the command 03658 state = 0 --> The command has been executed with no errors 03659 state = -1 --> Forbidden parameter value for this function 03660 Parameters: 03661 rate: value to compute the maximum current supply. Maximum current is 45+5*'rate' [mA] 03662 */ 03663 int8_t SX1272::setMaxCurrent(uint8_t rate) 03664 { 03665 int8_t state = 2; 03666 byte st0; 03667 03668 #if (SX1272_debug_mode > 1) 03669 printf("\n"); 03670 printf("Starting 'setMaxCurrent'\n"); 03671 #endif 03672 03673 // Maximum rate value = 0x1B, because maximum current supply = 240 mA 03674 if (rate > 0x1B) 03675 { 03676 state = -1; 03677 #if (SX1272_debug_mode > 1) 03678 printf("** Maximum current supply is 240 mA, "); 03679 printf("so maximum parameter value must be 27 (DEC) or 0x1B (HEX) **"); 03680 printf("\n"); 03681 #endif 03682 } 03683 else 03684 { 03685 // Enable Over Current Protection 03686 rate |= 0B00100000; 03687 03688 state = 1; 03689 st0 = readRegister(REG_OP_MODE); // Save the previous status 03690 if( _modem == LORA ) 03691 { // LoRa mode 03692 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Set LoRa Standby mode to write in registers 03693 } 03694 else 03695 { // FSK mode 03696 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Set FSK Standby mode to write in registers 03697 } 03698 writeRegister(REG_OCP, rate); // Modifying maximum current supply 03699 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 03700 state = 0; 03701 } 03702 return state; 03703 } 03704 03705 /* 03706 Function: Gets the content of different registers. 03707 Returns: Integer that determines if there has been any error 03708 state = 2 --> The command has not been executed 03709 state = 1 --> There has been an error while executing the command 03710 state = 0 --> The command has been executed with no errors 03711 */ 03712 uint8_t SX1272::getRegs() 03713 { 03714 int8_t state = 2; 03715 uint8_t state_f = 2; 03716 03717 #if (SX1272_debug_mode > 1) 03718 printf("\n"); 03719 printf("Starting 'getRegs'\n"); 03720 #endif 03721 03722 state_f = 1; 03723 state = getMode(); // Stores the BW, CR and SF. 03724 if( state == 0 ) 03725 { 03726 state = getPower(); // Stores the power. 03727 } 03728 else 03729 { 03730 state_f = 1; 03731 #if (SX1272_debug_mode > 1) 03732 printf("** Error getting mode **"); 03733 #endif 03734 } 03735 if( state == 0 ) 03736 { 03737 state = getChannel(); // Stores the channel. 03738 } 03739 else 03740 { 03741 state_f = 1; 03742 #if (SX1272_debug_mode > 1) 03743 printf("** Error getting power **"); 03744 #endif 03745 } 03746 if( state == 0 ) 03747 { 03748 state = getCRC(); // Stores the CRC configuration. 03749 } 03750 else 03751 { 03752 state_f = 1; 03753 #if (SX1272_debug_mode > 1) 03754 printf("** Error getting channel **"); 03755 #endif 03756 } 03757 if( state == 0 ) 03758 { 03759 state = getHeader(); // Stores the header configuration. 03760 } 03761 else 03762 { 03763 state_f = 1; 03764 #if (SX1272_debug_mode > 1) 03765 printf("** Error getting CRC **"); 03766 #endif 03767 } 03768 if( state == 0 ) 03769 { 03770 state = getPreambleLength(); // Stores the preamble length. 03771 } 03772 else 03773 { 03774 state_f = 1; 03775 #if (SX1272_debug_mode > 1) 03776 printf("** Error getting header **"); 03777 #endif 03778 } 03779 if( state == 0 ) 03780 { 03781 state = getPayloadLength(); // Stores the payload length. 03782 } 03783 else 03784 { 03785 state_f = 1; 03786 #if (SX1272_debug_mode > 1) 03787 printf("** Error getting preamble length **"); 03788 #endif 03789 } 03790 if( state == 0 ) 03791 { 03792 state = getNodeAddress(); // Stores the node address. 03793 } 03794 else 03795 { 03796 state_f = 1; 03797 #if (SX1272_debug_mode > 1) 03798 printf("** Error getting payload length **"); 03799 #endif 03800 } 03801 if( state == 0 ) 03802 { 03803 state = getMaxCurrent(); // Stores the maximum current supply. 03804 } 03805 else 03806 { 03807 state_f = 1; 03808 #if (SX1272_debug_mode > 1) 03809 printf("** Error getting node address **"); 03810 #endif 03811 } 03812 if( state == 0 ) 03813 { 03814 state_f = getTemp(); // Stores the module temperature. 03815 } 03816 else 03817 { 03818 state_f = 1; 03819 #if (SX1272_debug_mode > 1) 03820 printf("** Error getting maximum current supply **"); 03821 #endif 03822 } 03823 if( state_f != 0 ) 03824 { 03825 #if (SX1272_debug_mode > 1) 03826 printf("** Error getting temperature **"); 03827 printf("\n"); 03828 #endif 03829 } 03830 return state_f; 03831 } 03832 03833 /* 03834 Function: It truncs the payload length if it is greater than 0xFF. 03835 Returns: Integer that determines if there has been any error 03836 state = 2 --> The command has not been executed 03837 state = 1 --> There has been an error while executing the command 03838 state = 0 --> The command has been executed with no errors 03839 */ 03840 uint8_t SX1272::truncPayload(uint16_t length16) 03841 { 03842 uint8_t state = 2; 03843 03844 state = 1; 03845 03846 #if (SX1272_debug_mode > 1) 03847 printf("\n"); 03848 printf("Starting 'truncPayload'\n"); 03849 #endif 03850 03851 if( length16 > MAX_PAYLOAD ) 03852 { 03853 printf("XXXXXXXXXXXXXXXXXXXXX MAX_PAYLOAD XXXXXXXXXXXXXXXX\n"); 03854 _payloadlength = MAX_PAYLOAD; 03855 } 03856 else 03857 { 03858 _payloadlength = (length16 & 0xFF); 03859 } 03860 state = 0; 03861 03862 return state; 03863 } 03864 03865 /* 03866 Function: It sets an ACK in FIFO in order to send it. 03867 Returns: Integer that determines if there has been any error 03868 state = 2 --> The command has not been executed 03869 state = 1 --> There has been an error while executing the command 03870 state = 0 --> The command has been executed with no errors 03871 */ 03872 uint8_t SX1272::setACK() 03873 { 03874 uint8_t state = 2; 03875 03876 #if (SX1272_debug_mode > 1) 03877 printf("\n"); 03878 printf("Starting 'setACK'\n"); 03879 #endif 03880 03881 // added by C. Pham 03882 // check for enough remaining ToA 03883 // when operating under duty-cycle mode 03884 if (_limitToA) { 03885 if (getRemainingToA() - getToA(ACK_LENGTH) < 0) { 03886 printf("## not enough ToA for ACK at %d",millis()); 03887 // Serial.println(millis()); 03888 return SX1272_ERROR_TOA; 03889 } 03890 } 03891 03892 // delay(1000); 03893 03894 clearFlags(); // Initializing flags 03895 03896 if( _modem == LORA ) 03897 { // LoRa mode 03898 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Stdby LoRa mode to write in FIFO 03899 } 03900 else 03901 { // FSK mode 03902 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Stdby FSK mode to write in FIFO 03903 } 03904 03905 // Setting ACK length in order to send it 03906 state = setPacketLength(ACK_LENGTH); 03907 if( state == 0 ) 03908 { 03909 // Setting ACK 03910 ACK.dst = packet_received.src; // ACK destination is packet source 03911 ACK.type = PKT_TYPE_ACK; 03912 ACK.src = packet_received.dst; // ACK source is packet destination 03913 ACK.packnum = packet_received.packnum; // packet number that has been correctly received 03914 ACK.length = 2; 03915 ACK.data[0] = _reception; // CRC of the received packet 03916 // added by C. Pham 03917 // store the SNR 03918 ACK.data[1]= readRegister(REG_PKT_SNR_VALUE); 03919 03920 // Setting address pointer in FIFO data buffer 03921 writeRegister(REG_FIFO_ADDR_PTR, 0x80); 03922 03923 state = 1; 03924 03925 // Writing ACK to send in FIFO 03926 writeRegister(REG_FIFO, ACK.dst); // Writing the destination in FIFO 03927 writeRegister(REG_FIFO, ACK.type); 03928 writeRegister(REG_FIFO, ACK.src); // Writing the source in FIFO 03929 writeRegister(REG_FIFO, ACK.packnum); // Writing the packet number in FIFO 03930 writeRegister(REG_FIFO, ACK.length); // Writing the packet length in FIFO 03931 writeRegister(REG_FIFO, ACK.data[0]); // Writing the ACK in FIFO 03932 writeRegister(REG_FIFO, ACK.data[1]); // Writing the ACK in FIFO 03933 03934 //#if (SX1272_debug_mode > 0) 03935 printf("## ACK set and written in FIFO ##\n"); 03936 // Print the complete ACK if debug_mode 03937 printf("## ACK to send:\n"); 03938 printf("Destination: %d\n",ACK.dst); 03939 // Serial.println(ACK.dst); // Printing destination 03940 printf("Source: %d\n",ACK.src); 03941 // Serial.println(ACK.src); // Printing source 03942 printf("ACK number: %d\n",ACK.packnum); 03943 // Serial.println(ACK.packnum); // Printing ACK number 03944 printf("ACK length: %d\n",ACK.length); 03945 // Serial.println(ACK.length); // Printing ACK length 03946 printf("ACK payload: %d\n",ACK.data[0]); 03947 // Serial.println(ACK.data[0]); // Printing ACK payload 03948 printf("ACK SNR last rcv pkt: %d\n",_SNR); 03949 // Serial.println(_SNR); 03950 printf("##\n"); 03951 printf("\n"); 03952 //#endif 03953 03954 state = 0; 03955 _reception = CORRECT_PACKET; // Updating value to next packet 03956 03957 // comment by C. Pham 03958 // TODO: do we really need this delay? 03959 wait_ms(500); 03960 } 03961 return state; 03962 } 03963 03964 /* 03965 Function: Configures the module to receive information. 03966 Returns: Integer that determines if there has been any error 03967 state = 2 --> The command has not been executed 03968 state = 1 --> There has been an error while executing the command 03969 state = 0 --> The command has been executed with no errors 03970 */ 03971 uint8_t SX1272::receive() 03972 { 03973 uint8_t state = 1; 03974 03975 #if (SX1272_debug_mode > 1) 03976 printf("\n"); 03977 printf("Starting 'receive'\n"); 03978 #endif 03979 03980 // Initializing packet_received struct 03981 memset( &packet_received, 0x00, sizeof(packet_received) ); 03982 03983 // Setting Testmode 03984 // commented by C. Pham 03985 //writeRegister(0x31,0x43); 03986 03987 // Set LowPnTxPllOff 03988 // modified by C. Pham from 0x09 to 0x08 03989 writeRegister(REG_PA_RAMP, 0x08); 03990 03991 //writeRegister(REG_LNA, 0x23); // Important in reception 03992 // modified by C. Pham 03993 writeRegister(REG_LNA, LNA_MAX_GAIN); 03994 writeRegister(REG_FIFO_ADDR_PTR, 0x00); // Setting address pointer in FIFO data buffer 03995 // change RegSymbTimeoutLsb 03996 // comment by C. Pham 03997 // single_chan_pkt_fwd uses 00 00001000 03998 // why here we have 11 11111111 03999 // change RegSymbTimeoutLsb 04000 //writeRegister(REG_SYMB_TIMEOUT_LSB, 0xFF); 04001 04002 // modified by C. Pham 04003 if (_spreadingFactor == SF_10 || _spreadingFactor == SF_11 || _spreadingFactor == SF_12) { 04004 writeRegister(REG_SYMB_TIMEOUT_LSB,0x05); 04005 } else { 04006 writeRegister(REG_SYMB_TIMEOUT_LSB,0x08); 04007 } 04008 //end 04009 04010 writeRegister(REG_FIFO_RX_BYTE_ADDR, 0x00); // Setting current value of reception buffer pointer 04011 //clearFlags(); // Initializing flags 04012 //state = 1; 04013 if( _modem == LORA ) 04014 { // LoRa mode 04015 state = setPacketLength(MAX_LENGTH); // With MAX_LENGTH gets all packets with length < MAX_LENGTH 04016 writeRegister(REG_OP_MODE, LORA_RX_MODE); // LORA mode - Rx 04017 #if (SX1272_debug_mode > 1) 04018 printf("## Receiving LoRa mode activated with success ##"); 04019 printf("\n"); 04020 #endif 04021 } 04022 else 04023 { // FSK mode 04024 state = setPacketLength(); 04025 writeRegister(REG_OP_MODE, FSK_RX_MODE); // FSK mode - Rx 04026 #if (SX1272_debug_mode > 1) 04027 printf("## Receiving FSK mode activated with success ##"); 04028 printf("\n"); 04029 #endif 04030 } 04031 return state; 04032 } 04033 04034 /* 04035 Function: Configures the module to receive information. 04036 Returns: Integer that determines if there has been any error 04037 state = 2 --> The command has not been executed 04038 state = 1 --> There has been an error while executing the command 04039 state = 0 --> The command has been executed with no errors 04040 */ 04041 uint8_t SX1272::receivePacketMAXTimeout() 04042 { 04043 return receivePacketTimeout(MAX_TIMEOUT); 04044 } 04045 04046 /* 04047 Function: Configures the module to receive information. 04048 Returns: Integer that determines if there has been any error 04049 state = 2 --> The command has not been executed 04050 state = 1 --> There has been an error while executing the command 04051 state = 0 --> The command has been executed with no errors 04052 */ 04053 uint8_t SX1272::receivePacketTimeout() 04054 { 04055 setTimeout(); 04056 return receivePacketTimeout(_sendTime); 04057 } 04058 04059 /* 04060 Function: Configures the module to receive information. 04061 Returns: Integer that determines if there has been any error 04062 state = 2 --> The command has not been executed 04063 state = 1 --> There has been an error while executing the command 04064 state = 0 --> The command has been executed with no errors 04065 */ 04066 #ifdef W_REQUESTED_ACK 04067 04068 // added by C. Pham 04069 // receiver always use receivePacketTimeout() 04070 // sender should either use sendPacketTimeout() or sendPacketTimeoutACK() 04071 04072 uint8_t SX1272::receivePacketTimeout(uint16_t wait) 04073 { 04074 uint8_t state = 2; 04075 uint8_t state_f = 2; 04076 04077 04078 #if (SX1272_debug_mode > 1) 04079 printf("\n"); 04080 printf("Starting 'receivePacketTimeout'\n"); 04081 #endif 04082 04083 state = receive(); 04084 if( state == 0 ) 04085 { 04086 if( availableData(wait) ) 04087 { 04088 state = getPacket(); 04089 } 04090 else 04091 { 04092 state = 1; 04093 state_f = 3; // There is no packet received 04094 } 04095 } 04096 else 04097 { 04098 state = 1; 04099 state_f = 1; // There has been an error with the 'receive' function 04100 } 04101 04102 if( (state == 0) || (state == 3) || (state == 5) ) 04103 { 04104 if( _reception == INCORRECT_PACKET ) 04105 { 04106 state_f = 4; // The packet has been incorrectly received 04107 } 04108 else 04109 { 04110 state_f = 0; // The packet has been correctly received 04111 // added by C. Pham 04112 // we get the SNR and RSSI of the received packet for future usage 04113 getSNR(); 04114 getRSSIpacket(); 04115 } 04116 04117 // need to send an ACK 04118 if ( state == 5 && state_f == 0) { 04119 04120 state = setACK(); 04121 04122 if( state == 0 ) 04123 { 04124 state = sendWithTimeout(); 04125 if( state == 0 ) 04126 { 04127 state_f = 0; 04128 #if (SX1272_debug_mode > 1) 04129 printf("This last packet was an ACK, so ..."); 04130 printf("ACK successfully sent"); 04131 printf("\n"); 04132 #endif 04133 } 04134 else 04135 { 04136 state_f = 1; // There has been an error with the 'sendWithTimeout' function 04137 } 04138 } 04139 else 04140 { 04141 state_f = 1; // There has been an error with the 'setACK' function 04142 } 04143 } 04144 } 04145 else 04146 { 04147 // we need to conserve state_f=3 to indicate that no packet has been received after timeout 04148 //state_f = 1; 04149 } 04150 return state_f; 04151 } 04152 #else 04153 04154 uint8_t SX1272::receivePacketTimeout(uint16_t wait) 04155 { 04156 uint8_t state = 2; 04157 uint8_t state_f = 2; 04158 04159 #if (SX1272_debug_mode > 1) 04160 printf("\n"); 04161 printf("Starting 'receivePacketTimeout'\n"); 04162 #endif 04163 04164 state = receive(); 04165 if( state == 0 ) 04166 { 04167 if( availableData(wait) ) 04168 { 04169 // If packet received, getPacket 04170 state_f = getPacket(); 04171 } 04172 else 04173 { 04174 state_f = 1; 04175 } 04176 } 04177 else 04178 { 04179 state_f = state; 04180 } 04181 return state_f; 04182 } 04183 #endif 04184 04185 /* 04186 Function: Configures the module to receive information and send an ACK. 04187 Returns: Integer that determines if there has been any error 04188 state = 2 --> The command has not been executed 04189 state = 1 --> There has been an error while executing the command 04190 state = 0 --> The command has been executed with no errors 04191 */ 04192 uint8_t SX1272::receivePacketMAXTimeoutACK() 04193 { 04194 return receivePacketTimeoutACK(MAX_TIMEOUT); 04195 } 04196 04197 /* 04198 Function: Configures the module to receive information and send an ACK. 04199 Returns: Integer that determines if there has been any error 04200 state = 2 --> The command has not been executed 04201 state = 1 --> There has been an error while executing the command 04202 state = 0 --> The command has been executed with no errors 04203 */ 04204 uint8_t SX1272::receivePacketTimeoutACK() 04205 { 04206 setTimeout(); 04207 return receivePacketTimeoutACK(_sendTime); 04208 } 04209 04210 /* 04211 Function: Configures the module to receive information and send an ACK. 04212 Returns: Integer that determines if there has been any error 04213 state = 4 --> The command has been executed but the packet received is incorrect 04214 state = 3 --> The command has been executed but there is no packet received 04215 state = 2 --> The command has not been executed 04216 state = 1 --> There has been an error while executing the command 04217 state = 0 --> The command has been executed with no errors 04218 */ 04219 uint8_t SX1272::receivePacketTimeoutACK(uint16_t wait) 04220 { 04221 // commented by C. Pham because not used 04222 /* 04223 uint8_t state = 2; 04224 uint8_t state_f = 2; 04225 04226 04227 #if (SX1272_debug_mode > 1) 04228 printf("\n"); 04229 printf("Starting 'receivePacketTimeoutACK'"); 04230 #endif 04231 04232 state = receive(); 04233 if( state == 0 ) 04234 { 04235 if( availableData(wait) ) 04236 { 04237 state = getPacket(); 04238 } 04239 else 04240 { 04241 state = 1; 04242 state_f = 3; // There is no packet received 04243 } 04244 } 04245 else 04246 { 04247 state = 1; 04248 state_f = 1; // There has been an error with the 'receive' function 04249 } 04250 if( (state == 0) || (state == 3) ) 04251 { 04252 if( _reception == INCORRECT_PACKET ) 04253 { 04254 state_f = 4; // The packet has been incorrectly received 04255 } 04256 else 04257 { 04258 state_f = 1; // The packet has been correctly received 04259 } 04260 state = setACK(); 04261 if( state == 0 ) 04262 { 04263 state = sendWithTimeout(); 04264 if( state == 0 ) 04265 { 04266 state_f = 0; 04267 #if (SX1272_debug_mode > 1) 04268 printf("This last packet was an ACK, so ..."); 04269 printf("ACK successfully sent"); 04270 printf("\n"); 04271 #endif 04272 } 04273 else 04274 { 04275 state_f = 1; // There has been an error with the 'sendWithTimeout' function 04276 } 04277 } 04278 else 04279 { 04280 state_f = 1; // There has been an error with the 'setACK' function 04281 } 04282 } 04283 else 04284 { 04285 state_f = 1; 04286 } 04287 return state_f; 04288 */ 04289 // ajoute par C.Dupaty 04290 return 0; 04291 } 04292 04293 /* 04294 Function: Configures the module to receive all the information on air, before MAX_TIMEOUT expires. 04295 Returns: Integer that determines if there has been any error 04296 state = 2 --> The command has not been executed 04297 state = 1 --> There has been an error while executing the command 04298 state = 0 --> The command has been executed with no errors 04299 */ 04300 uint8_t SX1272::receiveAll() 04301 { 04302 return receiveAll(MAX_TIMEOUT); 04303 } 04304 04305 /* 04306 Function: Configures the module to receive all the information on air. 04307 Returns: Integer that determines if there has been any error 04308 state = 2 --> The command has not been executed 04309 state = 1 --> There has been an error while executing the command 04310 state = 0 --> The command has been executed with no errors 04311 */ 04312 uint8_t SX1272::receiveAll(uint16_t wait) 04313 { 04314 uint8_t state = 2; 04315 byte config1; 04316 04317 #if (SX1272_debug_mode > 1) 04318 printf("\n"); 04319 printf("Starting 'receiveAll'\n"); 04320 #endif 04321 04322 if( _modem == FSK ) 04323 { // FSK mode 04324 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Setting standby FSK mode 04325 config1 = readRegister(REG_PACKET_CONFIG1); 04326 config1 = config1 & 0B11111001; // clears bits 2-1 from REG_PACKET_CONFIG1 04327 writeRegister(REG_PACKET_CONFIG1, config1); // AddressFiltering = None 04328 } 04329 #if (SX1272_debug_mode > 1) 04330 printf("## Address filtering desactivated ##"); 04331 printf("\n"); 04332 #endif 04333 state = receive(); // Setting Rx mode 04334 if( state == 0 ) 04335 { 04336 state = getPacket(wait); // Getting all packets received in wait 04337 } 04338 return state; 04339 } 04340 04341 /* 04342 Function: If a packet is received, checks its destination. 04343 Returns: Boolean that's 'true' if the packet is for the module and 04344 it's 'false' if the packet is not for the module. 04345 */ 04346 boolean SX1272::availableData() 04347 { 04348 return availableData(MAX_TIMEOUT); 04349 } 04350 04351 /* 04352 Function: If a packet is received, checks its destination. 04353 Returns: Boolean that's 'true' if the packet is for the module and 04354 it's 'false' if the packet is not for the module. 04355 Parameters: 04356 wait: time to wait while there is no a valid header received. 04357 */ 04358 boolean SX1272::availableData(uint16_t wait) 04359 { 04360 byte value; 04361 byte header = 0; 04362 boolean forme = false; 04363 boolean _hreceived = false; 04364 //unsigned long previous; 04365 unsigned long exitTime; 04366 04367 04368 #if (SX1272_debug_mode > 0) 04369 printf("\n"); 04370 printf("Starting 'availableData'\n"); 04371 #endif 04372 04373 exitTime=millis()+(unsigned long)wait; 04374 04375 //previous = millis(); 04376 if( _modem == LORA ) 04377 { // LoRa mode 04378 value = readRegister(REG_IRQ_FLAGS); 04379 // Wait to ValidHeader interrupt 04380 //while( (bitRead(value, 4) == 0) && (millis() - previous < (unsigned long)wait) ) 04381 while( (bitRead(value, 4) == 0) && (millis() < exitTime) ) 04382 { 04383 value = readRegister(REG_IRQ_FLAGS); 04384 // Condition to avoid an overflow (DO NOT REMOVE) 04385 //if( millis() < previous ) 04386 //{ 04387 // previous = millis(); 04388 //} 04389 } // end while (millis) 04390 04391 if( bitRead(value, 4) == 1 ) 04392 { // header received 04393 #if (SX1272_debug_mode > 0) 04394 printf("## Valid Header received in LoRa mode ##"); 04395 #endif 04396 _hreceived = true; 04397 04398 #ifdef W_NET_KEY 04399 // actually, need to wait until 3 bytes have been received 04400 //while( (header < 3) && (millis() - previous < (unsigned long)wait) ) 04401 while( (header < 3) && (millis() < exitTime) ) 04402 #else 04403 //while( (header == 0) && (millis() - previous < (unsigned long)wait) ) 04404 while( (header == 0) && (millis() < exitTime) ) 04405 #endif 04406 { // Waiting to read first payload bytes from packet 04407 header = readRegister(REG_FIFO_RX_BYTE_ADDR); 04408 // Condition to avoid an overflow (DO NOT REMOVE) 04409 //if( millis() < previous ) 04410 //{ 04411 // previous = millis(); 04412 //} 04413 } 04414 04415 if( header != 0 ) 04416 { // Reading first byte of the received packet 04417 #ifdef W_NET_KEY 04418 // added by C. Pham 04419 // if we actually wait for an ACK, there is no net key before ACK data 04420 if (_requestACK==0) { 04421 _the_net_key_0 = readRegister(REG_FIFO); 04422 _the_net_key_1 = readRegister(REG_FIFO); 04423 } 04424 #endif 04425 _destination = readRegister(REG_FIFO); 04426 } 04427 } 04428 else 04429 { 04430 forme = false; 04431 _hreceived = false; 04432 #if (SX1272_debug_mode > 0) 04433 printf("** The timeout has expired **"); 04434 printf("\n"); 04435 #endif 04436 } 04437 } 04438 else 04439 { // FSK mode 04440 value = readRegister(REG_IRQ_FLAGS2); 04441 // Wait to Payload Ready interrupt 04442 //while( (bitRead(value, 2) == 0) && (millis() - previous < wait) ) 04443 while( (bitRead(value, 2) == 0) && (millis() < exitTime) ) 04444 { 04445 value = readRegister(REG_IRQ_FLAGS2); 04446 // Condition to avoid an overflow (DO NOT REMOVE) 04447 //if( millis() < previous ) 04448 //{ 04449 // previous = millis(); 04450 //} 04451 }// end while (millis) 04452 04453 if( bitRead(value, 2) == 1 ) // something received 04454 { 04455 _hreceived = true; 04456 #if (SX1272_debug_mode > 0) 04457 printf("## Valid Preamble detected in FSK mode ##"); 04458 #endif 04459 // Reading first byte of the received packet 04460 _destination = readRegister(REG_FIFO); 04461 } 04462 else 04463 { 04464 forme = false; 04465 _hreceived = false; 04466 #if (SX1272_debug_mode > 0) 04467 printf("** The timeout has expired **"); 04468 printf("\n"); 04469 #endif 04470 } 04471 } 04472 // We use _hreceived because we need to ensure that _destination value is correctly 04473 // updated and is not the _destination value from the previously packet 04474 if( _hreceived == true ) 04475 { // Checking destination 04476 #if (SX1272_debug_mode > 0) 04477 printf("## Checking destination ##"); 04478 #endif 04479 04480 // added by C. Pham 04481 #ifdef W_NET_KEY 04482 forme=true; 04483 04484 // if we wait for an ACK, then we do not check for net key 04485 if (_requestACK==0) 04486 if (_the_net_key_0!=_my_netkey[0] || _the_net_key_1!=_my_netkey[1]) { 04487 //#if (SX1272_debug_mode > 0) 04488 printf("## Wrong net key ##"); 04489 //#endif 04490 forme=false; 04491 } 04492 else 04493 { 04494 //#if (SX1272_debug_mode > 0) 04495 printf("## Good net key ##"); 04496 //#endif 04497 } 04498 04499 04500 if( forme && ((_destination == _nodeAddress) || (_destination == BROADCAST_0)) ) 04501 #else 04502 // modified by C. Pham 04503 // if _rawFormat, accept all 04504 if( (_destination == _nodeAddress) || (_destination == BROADCAST_0) || _rawFormat) 04505 #endif 04506 { // LoRa or FSK mode 04507 forme = true; 04508 #if (SX1272_debug_mode > 0) 04509 printf("## Packet received is for me ##"); 04510 #endif 04511 } 04512 else 04513 { 04514 forme = false; 04515 #if (SX1272_debug_mode > 0) 04516 printf("## Packet received is not for me ##"); 04517 printf("\n"); 04518 #endif 04519 if( _modem == LORA ) // STANDBY PARA MINIMIZAR EL CONSUMO 04520 { // LoRa mode 04521 //writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Setting standby LoRa mode 04522 } 04523 else 04524 { // FSK mode 04525 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Setting standby FSK mode 04526 } 04527 } 04528 } 04529 //----else 04530 // { 04531 // } 04532 return forme; 04533 } 04534 04535 /* 04536 Function: It gets and stores a packet if it is received before MAX_TIMEOUT expires. 04537 Returns: Integer that determines if there has been any error 04538 state = 2 --> The command has not been executed 04539 state = 1 --> There has been an error while executing the command 04540 state = 0 --> The command has been executed with no errors 04541 */ 04542 uint8_t SX1272::getPacketMAXTimeout() 04543 { 04544 return getPacket(MAX_TIMEOUT); 04545 } 04546 04547 /* 04548 Function: It gets and stores a packet if it is received. 04549 Returns: Integer that determines if there has been any error 04550 state = 2 --> The command has not been executed 04551 state = 1 --> There has been an error while executing the command 04552 state = 0 --> The command has been executed with no errors 04553 */ 04554 int8_t SX1272::getPacket() 04555 { 04556 return getPacket(MAX_TIMEOUT); 04557 } 04558 04559 /* 04560 Function: It gets and stores a packet if it is received before ending 'wait' time. 04561 Returns: Integer that determines if there has been any error 04562 // added by C. Pham 04563 state = 5 --> The command has been executed with no errors and an ACK is requested 04564 state = 3 --> The command has been executed but packet has been incorrectly received 04565 state = 2 --> The command has not been executed 04566 state = 1 --> There has been an error while executing the command 04567 state = 0 --> The command has been executed with no errors 04568 state = -1 --> Forbidden parameter value for this function 04569 Parameters: 04570 wait: time to wait while there is no a valid header received. 04571 */ 04572 int8_t SX1272::getPacket(uint16_t wait) 04573 { 04574 int8_t state = 2; // uint8_t a l origine 04575 byte value = 0x00; 04576 //unsigned long previous; 04577 unsigned long exitTime; 04578 boolean p_received = false; 04579 04580 #if (SX1272_debug_mode > 0) 04581 printf("\n"); 04582 printf("Starting 'getPacket'\n"); 04583 #endif 04584 04585 //previous = millis(); 04586 exitTime = millis() + (unsigned long)wait; 04587 if( _modem == LORA ) 04588 { // LoRa mode 04589 value = readRegister(REG_IRQ_FLAGS); 04590 // Wait until the packet is received (RxDone flag) or the timeout expires 04591 //while( (bitRead(value, 6) == 0) && (millis() - previous < (unsigned long)wait) ) 04592 while( (bitRead(value, 6) == 0) && (millis() < exitTime) ) 04593 { 04594 value = readRegister(REG_IRQ_FLAGS); 04595 // Condition to avoid an overflow (DO NOT REMOVE) 04596 //if( millis() < previous ) 04597 //{ 04598 // previous = millis(); 04599 //} 04600 } // end while (millis) 04601 04602 if( (bitRead(value, 6) == 1) && (bitRead(value, 5) == 0) ) 04603 { // packet received & CRC correct 04604 p_received = true; // packet correctly received 04605 _reception = CORRECT_PACKET; 04606 #if (SX1272_debug_mode > 0) 04607 printf("## Packet correctly received in LoRa mode ##"); 04608 #endif 04609 } 04610 else 04611 { 04612 if( bitRead(value, 5) != 0 ) 04613 { // CRC incorrect 04614 _reception = INCORRECT_PACKET; 04615 state = 3; 04616 #if (SX1272_debug_mode > 0) 04617 printf("** The CRC is incorrect **"); 04618 printf("\n"); 04619 #endif 04620 } 04621 } 04622 //writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Setting standby LoRa mode 04623 } 04624 else 04625 { // FSK mode 04626 value = readRegister(REG_IRQ_FLAGS2); 04627 //while( (bitRead(value, 2) == 0) && (millis() - previous < wait) ) 04628 while( (bitRead(value, 2) == 0) && (millis() < exitTime) ) 04629 { 04630 value = readRegister(REG_IRQ_FLAGS2); 04631 // Condition to avoid an overflow (DO NOT REMOVE) 04632 //if( millis() < previous ) 04633 //{ 04634 // previous = millis(); 04635 //} 04636 } // end while (millis) 04637 04638 if( bitRead(value, 2) == 1 ) 04639 { // packet received 04640 if( bitRead(value, 1) == 1 ) 04641 { // CRC correct 04642 _reception = CORRECT_PACKET; 04643 p_received = true; 04644 #if (SX1272_debug_mode > 0) 04645 printf("## Packet correctly received in FSK mode ##"); 04646 #endif 04647 } 04648 else 04649 { // CRC incorrect 04650 _reception = INCORRECT_PACKET; 04651 state = 3; 04652 p_received = false; 04653 #if (SX1272_debug_mode > 0) 04654 printf("## Packet incorrectly received in FSK mode ##"); 04655 #endif 04656 } 04657 } 04658 else 04659 { 04660 #if (SX1272_debug_mode > 0) 04661 printf("** The timeout has expired **"); 04662 printf("\n"); 04663 #endif 04664 } 04665 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Setting standby FSK mode 04666 } 04667 if( p_received == true ) 04668 { 04669 // Store the packet 04670 if( _modem == LORA ) 04671 { 04672 // comment by C. Pham 04673 // set the FIFO addr to 0 to read again all the bytes 04674 writeRegister(REG_FIFO_ADDR_PTR, 0x00); // Setting address pointer in FIFO data buffer 04675 04676 #ifdef W_NET_KEY 04677 // added by C. Pham 04678 packet_received.netkey[0]=readRegister(REG_FIFO); 04679 packet_received.netkey[1]=readRegister(REG_FIFO); 04680 #endif 04681 //modified by C. Pham 04682 if (!_rawFormat) 04683 packet_received.dst = readRegister(REG_FIFO); // Storing first byte of the received packet 04684 else 04685 packet_received.dst = 0; 04686 } 04687 else 04688 { 04689 value = readRegister(REG_PACKET_CONFIG1); 04690 if( (bitRead(value, 2) == 0) && (bitRead(value, 1) == 0) ) 04691 { 04692 packet_received.dst = readRegister(REG_FIFO); // Storing first byte of the received packet 04693 } 04694 else 04695 { 04696 packet_received.dst = _destination; // Storing first byte of the received packet 04697 } 04698 } 04699 04700 // modified by C. Pham 04701 if (!_rawFormat) { 04702 packet_received.type = readRegister(REG_FIFO); // Reading second byte of the received packet 04703 packet_received.src = readRegister(REG_FIFO); // Reading second byte of the received packet 04704 packet_received.packnum = readRegister(REG_FIFO); // Reading third byte of the received packet 04705 //packet_received.length = readRegister(REG_FIFO); // Reading fourth byte of the received packet 04706 } 04707 else { 04708 packet_received.type = 0; 04709 packet_received.src = 0; 04710 packet_received.packnum = 0; 04711 } 04712 04713 packet_received.length = readRegister(REG_RX_NB_BYTES); 04714 04715 if( _modem == LORA ) 04716 { 04717 if (_rawFormat) { 04718 _payloadlength=packet_received.length; 04719 } 04720 else 04721 _payloadlength = packet_received.length - OFFSET_PAYLOADLENGTH; 04722 } 04723 if( packet_received.length > (MAX_LENGTH + 1) ) 04724 { 04725 #if (SX1272_debug_mode > 0) 04726 printf("Corrupted packet, length must be less than 256"); 04727 #endif 04728 } 04729 else 04730 { 04731 for(unsigned int i = 0; i < _payloadlength; i++) 04732 { 04733 packet_received.data[i] = readRegister(REG_FIFO); // Storing payload 04734 } 04735 04736 // commented by C. Pham 04737 //packet_received.retry = readRegister(REG_FIFO); 04738 04739 // Print the packet if debug_mode 04740 #if (SX1272_debug_mode > 0) 04741 printf("## Packet received:\n"); 04742 printf("Destination: %d\n",packet_received.dst); 04743 // Serial.println(packet_received.dst); // Printing destination 04744 printf("Type: %d\n",packet_received.type); 04745 // Serial.println(packet_received.type); // Printing source 04746 printf("Source: %d\n",packet_received.src); 04747 // Serial.println(packet_received.src); // Printing source 04748 printf("Packet number: %d\n",packet_received.packnum); 04749 // Serial.println(packet_received.packnum); // Printing packet number 04750 //printf("Packet length: "); 04751 //Serial.println(packet_received.length); // Printing packet length 04752 printf("Data: "); 04753 for(unsigned int i = 0; i < _payloadlength; i++) 04754 { 04755 //Serial.print((char)packet_received.data[i]); // Printing payload 04756 printf("%c",packet_received.data[i]); 04757 } 04758 printf("\n"); 04759 //printf("Retry number: "); 04760 //Serial.println(packet_received.retry); // Printing number retry 04761 printf("##"); 04762 printf("\n"); 04763 #endif 04764 state = 0; 04765 04766 #ifdef W_REQUESTED_ACK 04767 // added by C. Pham 04768 // need to send an ACK 04769 if (packet_received.type & PKT_FLAG_ACK_REQ) { 04770 state = 5; 04771 _requestACK_indicator=1; 04772 } 04773 else 04774 _requestACK_indicator=0; 04775 #endif 04776 } 04777 } 04778 else 04779 { 04780 state = 1; 04781 if( (_reception == INCORRECT_PACKET) && (_retries < _maxRetries) ) 04782 { 04783 // comment by C. Pham 04784 // what is the purpose of incrementing retries here? 04785 // bug? not needed? 04786 _retries++; 04787 #if (SX1272_debug_mode > 0) 04788 printf("## Retrying to send the last packet ##"); 04789 printf("\n"); 04790 #endif 04791 } 04792 } 04793 if( _modem == LORA ) 04794 { 04795 writeRegister(REG_FIFO_ADDR_PTR, 0x00); // Setting address pointer in FIFO data buffer 04796 } 04797 clearFlags(); // Initializing flags 04798 if( wait > MAX_WAIT ) 04799 { 04800 state = -1; 04801 #if (SX1272_debug_mode > 0) 04802 printf("** The timeout must be smaller than 12.5 seconds **"); 04803 printf("\n"); 04804 #endif 04805 } 04806 04807 return state; 04808 } 04809 04810 /* 04811 Function: It sets the packet destination. 04812 Returns: Integer that determines if there has been any error 04813 state = 2 --> The command has not been executed 04814 state = 1 --> There has been an error while executing the command 04815 state = 0 --> The command has been executed with no errors 04816 Parameters: 04817 dest: destination value of the packet sent. 04818 */ 04819 int8_t SX1272::setDestination(uint8_t dest) 04820 { 04821 int8_t state = 2; 04822 04823 #if (SX1272_debug_mode > 1) 04824 printf("\n"); 04825 printf("Starting 'setDestination'\n"); 04826 #endif 04827 04828 state = 1; 04829 _destination = dest; // Storing destination in a global variable 04830 packet_sent.dst = dest; // Setting destination in packet structure 04831 packet_sent.src = _nodeAddress; // Setting source in packet structure 04832 packet_sent.packnum = _packetNumber; // Setting packet number in packet structure 04833 _packetNumber++; 04834 state = 0; 04835 04836 #if (SX1272_debug_mode > 1) 04837 printf("## Destination %X\n",_destination); 04838 // Serial.print(_destination, HEX); 04839 printf(" successfully set ##"); 04840 printf("## Source %d\n",packet_sent.src); 04841 // Serial.print(packet_sent.src, DEC); 04842 printf(" successfully set ##"); 04843 printf("## Packet number %d\n",packet_sent.packnum); 04844 // Serial.print(packet_sent.packnum, DEC); 04845 printf(" successfully set ##"); 04846 printf("\n"); 04847 #endif 04848 return state; 04849 } 04850 04851 /* 04852 Function: It sets the timeout according to the configured mode. 04853 Returns: Integer that determines if there has been any error 04854 state = 2 --> The command has not been executed 04855 state = 1 --> There has been an error while executing the command 04856 state = 0 --> The command has been executed with no errors 04857 */ 04858 uint8_t SX1272::setTimeout() 04859 { 04860 uint8_t state = 2; 04861 // retire par C.Dupaty 04862 // uint16_t delay; 04863 04864 #if (SX1272_debug_mode > 1) 04865 printf("\n"); 04866 printf("Starting 'setTimeout'\n"); 04867 #endif 04868 04869 state = 1; 04870 04871 // changed by C. Pham 04872 // we always use MAX_TIMEOUT 04873 _sendTime = MAX_TIMEOUT; 04874 04875 /* 04876 if( _modem == LORA ) 04877 { 04878 switch(_spreadingFactor) 04879 { // Choosing Spreading Factor 04880 case SF_6: switch(_bandwidth) 04881 { // Choosing bandwidth 04882 case BW_125: 04883 switch(_codingRate) 04884 { // Choosing coding rate 04885 case CR_5: _sendTime = 335; 04886 break; 04887 case CR_6: _sendTime = 352; 04888 break; 04889 case CR_7: _sendTime = 368; 04890 break; 04891 case CR_8: _sendTime = 386; 04892 break; 04893 } 04894 break; 04895 case BW_250: 04896 switch(_codingRate) 04897 { // Choosing coding rate 04898 case CR_5: _sendTime = 287; 04899 break; 04900 case CR_6: _sendTime = 296; 04901 break; 04902 case CR_7: _sendTime = 305; 04903 break; 04904 case CR_8: _sendTime = 312; 04905 break; 04906 } 04907 break; 04908 case BW_500: 04909 switch(_codingRate) 04910 { // Choosing coding rate 04911 case CR_5: _sendTime = 242; 04912 break; 04913 case CR_6: _sendTime = 267; 04914 break; 04915 case CR_7: _sendTime = 272; 04916 break; 04917 case CR_8: _sendTime = 276; 04918 break; 04919 } 04920 break; 04921 } 04922 break; 04923 04924 case SF_7: switch(_bandwidth) 04925 { // Choosing bandwidth 04926 case BW_125: 04927 switch(_codingRate) 04928 { // Choosing coding rate 04929 case CR_5: _sendTime = 408; 04930 break; 04931 case CR_6: _sendTime = 438; 04932 break; 04933 case CR_7: _sendTime = 468; 04934 break; 04935 case CR_8: _sendTime = 497; 04936 break; 04937 } 04938 break; 04939 case BW_250: 04940 switch(_codingRate) 04941 { // Choosing coding rate 04942 case CR_5: _sendTime = 325; 04943 break; 04944 case CR_6: _sendTime = 339; 04945 break; 04946 case CR_7: _sendTime = 355; 04947 break; 04948 case CR_8: _sendTime = 368; 04949 break; 04950 } 04951 break; 04952 case BW_500: 04953 switch(_codingRate) 04954 { // Choosing coding rate 04955 case CR_5: _sendTime = 282; 04956 break; 04957 case CR_6: _sendTime = 290; 04958 break; 04959 case CR_7: _sendTime = 296; 04960 break; 04961 case CR_8: _sendTime = 305; 04962 break; 04963 } 04964 break; 04965 } 04966 break; 04967 04968 case SF_8: switch(_bandwidth) 04969 { // Choosing bandwidth 04970 case BW_125: 04971 switch(_codingRate) 04972 { // Choosing coding rate 04973 case CR_5: _sendTime = 537; 04974 break; 04975 case CR_6: _sendTime = 588; 04976 break; 04977 case CR_7: _sendTime = 640; 04978 break; 04979 case CR_8: _sendTime = 691; 04980 break; 04981 } 04982 break; 04983 case BW_250: 04984 switch(_codingRate) 04985 { // Choosing coding rate 04986 case CR_5: _sendTime = 388; 04987 break; 04988 case CR_6: _sendTime = 415; 04989 break; 04990 case CR_7: _sendTime = 440; 04991 break; 04992 case CR_8: _sendTime = 466; 04993 break; 04994 } 04995 break; 04996 case BW_500: 04997 switch(_codingRate) 04998 { // Choosing coding rate 04999 case CR_5: _sendTime = 315; 05000 break; 05001 case CR_6: _sendTime = 326; 05002 break; 05003 case CR_7: _sendTime = 340; 05004 break; 05005 case CR_8: _sendTime = 352; 05006 break; 05007 } 05008 break; 05009 } 05010 break; 05011 05012 case SF_9: switch(_bandwidth) 05013 { // Choosing bandwidth 05014 case BW_125: 05015 switch(_codingRate) 05016 { // Choosing coding rate 05017 case CR_5: _sendTime = 774; 05018 break; 05019 case CR_6: _sendTime = 864; 05020 break; 05021 case CR_7: _sendTime = 954; 05022 break; 05023 case CR_8: _sendTime = 1044; 05024 break; 05025 } 05026 break; 05027 case BW_250: 05028 switch(_codingRate) 05029 { // Choosing coding rate 05030 case CR_5: _sendTime = 506; 05031 break; 05032 case CR_6: _sendTime = 552; 05033 break; 05034 case CR_7: _sendTime = 596; 05035 break; 05036 case CR_8: _sendTime = 642; 05037 break; 05038 } 05039 break; 05040 case BW_500: 05041 switch(_codingRate) 05042 { // Choosing coding rate 05043 case CR_5: _sendTime = 374; 05044 break; 05045 case CR_6: _sendTime = 396; 05046 break; 05047 case CR_7: _sendTime = 418; 05048 break; 05049 case CR_8: _sendTime = 441; 05050 break; 05051 } 05052 break; 05053 } 05054 break; 05055 05056 case SF_10: switch(_bandwidth) 05057 { // Choosing bandwidth 05058 case BW_125: 05059 switch(_codingRate) 05060 { // Choosing coding rate 05061 case CR_5: _sendTime = 1226; 05062 break; 05063 case CR_6: _sendTime = 1388; 05064 break; 05065 case CR_7: _sendTime = 1552; 05066 break; 05067 case CR_8: _sendTime = 1716; 05068 break; 05069 } 05070 break; 05071 case BW_250: 05072 switch(_codingRate) 05073 { // Choosing coding rate 05074 case CR_5: _sendTime = 732; 05075 break; 05076 case CR_6: _sendTime = 815; 05077 break; 05078 case CR_7: _sendTime = 896; 05079 break; 05080 case CR_8: _sendTime = 977; 05081 break; 05082 } 05083 break; 05084 case BW_500: 05085 switch(_codingRate) 05086 { // Choosing coding rate 05087 case CR_5: _sendTime = 486; 05088 break; 05089 case CR_6: _sendTime = 527; 05090 break; 05091 case CR_7: _sendTime = 567; 05092 break; 05093 case CR_8: _sendTime = 608; 05094 break; 05095 } 05096 break; 05097 } 05098 break; 05099 05100 case SF_11: switch(_bandwidth) 05101 { // Choosing bandwidth 05102 case BW_125: 05103 switch(_codingRate) 05104 { // Choosing coding rate 05105 case CR_5: _sendTime = 2375; 05106 break; 05107 case CR_6: _sendTime = 2735; 05108 break; 05109 case CR_7: _sendTime = 3095; 05110 break; 05111 case CR_8: _sendTime = 3456; 05112 break; 05113 } 05114 break; 05115 case BW_250: 05116 switch(_codingRate) 05117 { // Choosing coding rate 05118 case CR_5: _sendTime = 1144; 05119 break; 05120 case CR_6: _sendTime = 1291; 05121 break; 05122 case CR_7: _sendTime = 1437; 05123 break; 05124 case CR_8: _sendTime = 1586; 05125 break; 05126 } 05127 break; 05128 case BW_500: 05129 switch(_codingRate) 05130 { // Choosing coding rate 05131 case CR_5: _sendTime = 691; 05132 break; 05133 case CR_6: _sendTime = 766; 05134 break; 05135 case CR_7: _sendTime = 838; 05136 break; 05137 case CR_8: _sendTime = 912; 05138 break; 05139 } 05140 break; 05141 } 05142 break; 05143 05144 case SF_12: switch(_bandwidth) 05145 { // Choosing bandwidth 05146 case BW_125: 05147 switch(_codingRate) 05148 { // Choosing coding rate 05149 case CR_5: _sendTime = 4180; 05150 break; 05151 case CR_6: _sendTime = 4836; 05152 break; 05153 case CR_7: _sendTime = 5491; 05154 break; 05155 case CR_8: _sendTime = 6146; 05156 break; 05157 } 05158 break; 05159 case BW_250: 05160 switch(_codingRate) 05161 { // Choosing coding rate 05162 case CR_5: _sendTime = 1965; 05163 break; 05164 case CR_6: _sendTime = 2244; 05165 break; 05166 case CR_7: _sendTime = 2521; 05167 break; 05168 case CR_8: _sendTime = 2800; 05169 break; 05170 } 05171 break; 05172 case BW_500: 05173 switch(_codingRate) 05174 { // Choosing coding rate 05175 case CR_5: _sendTime = 1102; 05176 break; 05177 case CR_6: _sendTime = 1241; 05178 break; 05179 case CR_7: _sendTime = 1381; 05180 break; 05181 case CR_8: _sendTime = 1520; 05182 break; 05183 } 05184 break; 05185 } 05186 break; 05187 default: _sendTime = MAX_TIMEOUT; 05188 } 05189 } 05190 else 05191 { 05192 _sendTime = MAX_TIMEOUT; 05193 } 05194 delay = ((0.1*_sendTime) + 1); 05195 _sendTime = (uint16_t) ((_sendTime * 1.2) + (rand()%delay)); 05196 05197 */ 05198 #if (SX1272_debug_mode > 1) 05199 printf("Timeout to send/receive is: %d\n",_sendTime); 05200 // Serial.println(_sendTime, DEC); 05201 #endif 05202 state = 0; 05203 return state; 05204 } 05205 05206 /* 05207 Function: It sets a char array payload packet in a packet struct. 05208 Returns: Integer that determines if there has been any error 05209 state = 2 --> The command has not been executed 05210 state = 1 --> There has been an error while executing the command 05211 state = 0 --> The command has been executed with no errors 05212 */ 05213 uint8_t SX1272::setPayload(char *payload) 05214 { 05215 uint8_t state = 2; 05216 uint8_t state_f = 2; 05217 uint16_t length16; 05218 05219 #if (SX1272_debug_mode > 1) 05220 printf("\n"); 05221 printf("Starting 'setPayload'\n"); 05222 #endif 05223 05224 state = 1; 05225 length16 = (uint16_t)strlen(payload); 05226 state = truncPayload(length16); 05227 if( state == 0 ) 05228 { 05229 // fill data field until the end of the string 05230 for(unsigned int i = 0; i < _payloadlength; i++) 05231 { 05232 packet_sent.data[i] = payload[i]; 05233 } 05234 } 05235 else 05236 { 05237 state_f = state; 05238 } 05239 if( ( _modem == FSK ) && ( _payloadlength > MAX_PAYLOAD_FSK ) ) 05240 { 05241 _payloadlength = MAX_PAYLOAD_FSK; 05242 state = 1; 05243 #if (SX1272_debug_mode > 1) 05244 printf("In FSK, payload length must be less than 60 bytes."); 05245 printf("\n"); 05246 #endif 05247 } 05248 // set length with the actual counter value 05249 state_f = setPacketLength(); // Setting packet length in packet structure 05250 return state_f; 05251 } 05252 05253 /* 05254 Function: It sets an uint8_t array payload packet in a packet struct. 05255 Returns: Integer that determines if there has been any error 05256 state = 2 --> The command has not been executed 05257 state = 1 --> There has been an error while executing the command 05258 state = 0 --> The command has been executed with no errors 05259 */ 05260 uint8_t SX1272::setPayload(uint8_t *payload) 05261 { 05262 uint8_t state = 2; 05263 05264 #if (SX1272_debug_mode > 1) 05265 printf("\n"); 05266 printf("Starting 'setPayload'\n"); 05267 #endif 05268 05269 state = 1; 05270 if( ( _modem == FSK ) && ( _payloadlength > MAX_PAYLOAD_FSK ) ) 05271 { 05272 _payloadlength = MAX_PAYLOAD_FSK; 05273 state = 1; 05274 #if (SX1272_debug_mode > 1) 05275 printf("In FSK, payload length must be less than 60 bytes."); 05276 printf("\n"); 05277 #endif 05278 } 05279 for(unsigned int i = 0; i < _payloadlength; i++) 05280 { 05281 packet_sent.data[i] = payload[i]; // Storing payload in packet structure 05282 } 05283 // set length with the actual counter value 05284 state = setPacketLength(); // Setting packet length in packet structure 05285 return state; 05286 } 05287 05288 /* 05289 Function: It sets a packet struct in FIFO in order to send it. 05290 Returns: Integer that determines if there has been any error 05291 state = 2 --> The command has not been executed 05292 state = 1 --> There has been an error while executing the command 05293 state = 0 --> The command has been executed with no errors 05294 */ 05295 uint8_t SX1272::setPacket(uint8_t dest, char *payload) 05296 { 05297 int8_t state = 2; 05298 05299 #if (SX1272_debug_mode > 1) 05300 printf("\n"); 05301 printf("Starting 'setPacket'\n"); 05302 #endif 05303 05304 // printf("dentro do setPacket payload = %s\n",payload); 05305 // added by C. Pham 05306 // check for enough remaining ToA 05307 // when operating under duty-cycle mode 05308 if (_limitToA) { 05309 uint16_t length16 = (uint16_t)strlen(payload); 05310 05311 if (!_rawFormat) 05312 length16 = length16 + OFFSET_PAYLOADLENGTH; 05313 05314 if (getRemainingToA() - getToA(length16) < 0) { 05315 printf("## not enough ToA at %d\n",millis()); 05316 // Serial.println(millis()); 05317 return SX1272_ERROR_TOA; 05318 } 05319 } 05320 05321 clearFlags(); // Initializing flags 05322 printf("setPacket1____________________\n"); 05323 if( _modem == LORA ) 05324 { // LoRa mode 05325 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Stdby LoRa mode to write in FIFO 05326 printf("_modem == Lora\n"); 05327 } 05328 else 05329 { // FSK mode 05330 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Stdby FSK mode to write in FIFO 05331 printf("_modem == FSK\n"); 05332 } 05333 05334 _reception = CORRECT_PACKET; // Updating incorrect value 05335 if( _retries == 0 ) 05336 { // Updating this values only if is not going to re-send the last packet 05337 state = setDestination(dest); // Setting destination in packet structure 05338 packet_sent.retry = _retries; 05339 if( state == 0 ) 05340 { 05341 state = setPayload(payload); 05342 } 05343 } 05344 else 05345 { 05346 // comment by C. Pham 05347 // why to increase the length here? 05348 // bug? 05349 if( _retries == 1 ) 05350 { 05351 packet_sent.length++; 05352 } 05353 state = setPacketLength(); 05354 packet_sent.retry = _retries; 05355 #if (SX1272_debug_mode > 0) 05356 printf("** Retrying to send last packet %d\n",_retries); 05357 // Serial.print(_retries, DEC); 05358 printf(" time **"); 05359 #endif 05360 } 05361 05362 // added by C. Pham 05363 // set the type to be a data packet 05364 packet_sent.type |= PKT_TYPE_DATA; 05365 05366 #ifdef W_REQUESTED_ACK 05367 // added by C. Pham 05368 // indicate that an ACK should be sent by the receiver 05369 if (_requestACK) 05370 packet_sent.type |= PKT_FLAG_ACK_REQ; 05371 #endif 05372 05373 writeRegister(REG_FIFO_ADDR_PTR, 0x80); // Setting address pointer in FIFO data buffer 05374 if( state == 0 ) 05375 { 05376 state = 1; 05377 // Writing packet to send in FIFO 05378 #ifdef W_NET_KEY 05379 // added by C. Pham 05380 packet_sent.netkey[0]=_my_netkey[0]; 05381 packet_sent.netkey[1]=_my_netkey[1]; 05382 //#if (SX1272_debug_mode > 0) 05383 // printf("## Setting net key ##"); 05384 //#endif 05385 writeRegister(REG_FIFO, packet_sent.netkey[0]); 05386 writeRegister(REG_FIFO, packet_sent.netkey[1]); 05387 #endif 05388 // added by C. Pham 05389 // we can skip the header for instance when we want to generate 05390 // at a higher layer a LoRaWAN packet 05391 writeRegister(0x01,129);//standby mode 05392 if (!_rawFormat) { 05393 writeRegister(REG_FIFO, packet_sent.dst); // Writing the destination in FIFO 05394 // // added by C. Pham 05395 writeRegister(REG_FIFO, packet_sent.type); // Writing the packet type in FIFO 05396 writeRegister(REG_FIFO, packet_sent.src); // Writing the source in FIFO 05397 writeRegister(REG_FIFO, packet_sent.packnum); // Writing the packet number in FIFO 05398 } 05399 // commented by C. Pham 05400 //writeRegister(REG_FIFO, packet_sent.length); // Writing the packet length in FIFO 05401 for(unsigned int i = 0; i < _payloadlength; i++) 05402 { 05403 writeRegister(REG_FIFO, packet_sent.data[i]); // Writing the payload in FIFO 05404 } 05405 // commented by C. Pham 05406 //writeRegister(REG_FIFO, packet_sent.retry); // Writing the number retry in FIFO 05407 // for (int i=0 ; i<255 ; i++) 05408 // printf("%d ",readRegister(REG_FIFO)); 05409 // printf("/n"); 05410 state = 0; 05411 #if (SX1272_debug_mode > 0) 05412 printf("in FIFO ##"); 05413 // Print the complete packet if debug_mode 05414 printf("## Packet to send: \n"); 05415 printf("Destination: %d\n",packet_sent.dst); 05416 // Serial.println(packet_sent.dst); // Printing destination 05417 printf("Packet type: %d\n",packet_sent.type); 05418 // Serial.println(packet_sent.type); // Printing packet type 05419 printf("Source: %d\n",packet_sent.src); 05420 // Serial.println(packet_sent.src); // Printing source 05421 printf("Packet number: %d\n",packet_sent.packnum); 05422 // Serial.println(packet_sent.packnum); // Printing packet number 05423 printf("Packet length: %d\n",packet_sent.length); 05424 // Serial.println(packet_sent.length); // Printing packet length 05425 printf("Data: "); 05426 for(unsigned int i = 0; i < _payloadlength; i++) 05427 { 05428 // Serial.print((char)packet_sent.data[i]); // Printing payload 05429 printf("%c",packet_sent.data[i]); 05430 } 05431 printf("\n"); 05432 //printf("Retry number: "); 05433 //Serial.println(packet_sent.retry); // Printing retry number 05434 printf("##"); 05435 #endif 05436 } 05437 05438 return state; 05439 } 05440 05441 /* 05442 Function: It sets a packet struct in FIFO in order to sent it. 05443 Returns: Integer that determines if there has been any error 05444 state = 2 --> The command has not been executed 05445 state = 1 --> There has been an error while executing the command 05446 state = 0 --> The command has been executed with no errors 05447 */ 05448 uint8_t SX1272::setPacket(uint8_t dest, uint8_t *payload) 05449 { 05450 int8_t state = 2; 05451 byte st0; 05452 05453 // sx1272.writeRegister(0x01,129); //standby mode 05454 #if (SX1272_debug_mode > 1) 05455 printf("\n"); 05456 printf("Starting 'setPacket'\n"); 05457 #endif 05458 05459 // added by C. Pham 05460 // check for enough remaining ToA 05461 // when operating under duty-cycle mode 05462 if (_limitToA) { 05463 // here truncPayload() should have been called before in 05464 // sendPacketTimeout(uint8_t dest, uint8_t *payload, uint16_t length16) 05465 uint16_t length16 = _payloadlength; 05466 05467 if (!_rawFormat) 05468 length16 = length16 + OFFSET_PAYLOADLENGTH; 05469 05470 if (getRemainingToA() - getToA(length16) < 0) { 05471 printf("## not enough ToA at %d\n",millis()); 05472 // Serial.println(millis()); 05473 return SX1272_ERROR_TOA; 05474 } 05475 } 05476 05477 st0 = readRegister(REG_OP_MODE); // Save the previous status 05478 clearFlags(); // Initializing flags 05479 // printf("setPacket_2_____________________\n"); 05480 // printf("mode =%d\n",readRegister(REG_OP_MODE)&3); 05481 if( _modem == LORA ) 05482 { // LoRa mode 05483 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Stdby LoRa mode to write in FIFO 05484 // printf("setpacket_2 LORA\n"); 05485 } 05486 else 05487 { // FSK mode 05488 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Stdby FSK mode to write in FIFO 05489 printf("setpacket_2 FSK\n"); 05490 } 05491 // printf("mode =%d\n",readRegister(REG_OP_MODE)&3); 05492 _reception = CORRECT_PACKET; // Updating incorrect value to send a packet (old or new) 05493 if( _retries == 0 ) 05494 { // Sending new packet 05495 state = setDestination(dest); // Setting destination in packet structure 05496 packet_sent.retry = _retries; 05497 if( state == 0 ) 05498 { 05499 state = setPayload(payload); 05500 } 05501 } 05502 else 05503 { 05504 // comment by C. Pham 05505 // why to increase the length here? 05506 // bug? 05507 if( _retries == 1 ) 05508 { 05509 packet_sent.length++; 05510 } 05511 state = setPacketLength(); 05512 packet_sent.retry = _retries; 05513 #if (SX1272_debug_mode > 0) 05514 printf("** Retrying to send last packet %d\n",_retries); 05515 // Serial.print(_retries, DEC); 05516 printf(" time **"); 05517 #endif 05518 } 05519 05520 // added by C. Pham 05521 // set the type to be a data packet 05522 packet_sent.type |= PKT_TYPE_DATA; 05523 05524 #ifdef W_REQUESTED_ACK 05525 // added by C. Pham 05526 // indicate that an ACK should be sent by the receiver 05527 if (_requestACK) 05528 packet_sent.type |= PKT_FLAG_ACK_REQ; 05529 #endif 05530 writeRegister(REG_FIFO_ADDR_PTR, 0x80); // Setting address pointer in FIFO data buffer 05531 if( state == 0 ) 05532 { 05533 state = 1; 05534 // Writing packet to send in FIFO 05535 #ifdef W_NET_KEY 05536 // added by C. Pham 05537 05538 packet_sent.netkey[0]=_my_netkey[0]; 05539 packet_sent.netkey[1]=_my_netkey[1]; 05540 //#if (SX1272_debug_mode > 0) 05541 printf("## Setting net key ##"); 05542 //#endif 05543 writeRegister(REG_FIFO, packet_sent.netkey[0]); 05544 writeRegister(REG_FIFO, packet_sent.netkey[1]); 05545 #endif 05546 // added by C. Pham 05547 // we can skip the header for instance when we want to generate 05548 // at a higher layer a LoRaWAN packet 05549 05550 if (!_rawFormat) { 05551 writeRegister(REG_FIFO, packet_sent.dst); // Writing the destination in FIFO 05552 // added by C. Pham 05553 writeRegister(REG_FIFO, packet_sent.type); // Writing the packet type in FIFO 05554 writeRegister(REG_FIFO, packet_sent.src); // Writing the source in FIFO 05555 writeRegister(REG_FIFO, packet_sent.packnum); // Writing the packet number in FIFO 05556 } 05557 this->packet_sent.packnum++; 05558 // commented by C. Pham 05559 //writeRegister(REG_FIFO, packet_sent.length); // Writing the packet length in FIFO 05560 for(unsigned int i = 0; i < _payloadlength; i++) 05561 { 05562 writeRegister(REG_FIFO, packet_sent.data[i]); // Writing the payload in FIFO 05563 } 05564 // printf("FIFO depois da escrita\n"); 05565 // for(int i=0; i!= 255 ;i++) 05566 // printf("%c",readRegister(REG_FIFO)); 05567 // printf("\n"); 05568 // commented by C. Pham 05569 //writeRegister(REG_FIFO, packet_sent.retry); // Writing the number retry in FIFO 05570 state = 0; 05571 #if (SX1272_debug_mode > 0) 05572 printf("## Packet set and written in FIFO ##"); 05573 // Print the complete packet if debug_mode 05574 printf("## Packet to send: "); 05575 printf("Destination: %d\n",packet_sent.dst); 05576 // Serial.println(packet_sent.dst); // Printing destination 05577 printf("Packet type: %d\n",packet_sent.type); 05578 // Serial.println(packet_sent.type); // Printing packet type 05579 printf("Source: %d\n",packet_sent.src); 05580 // Serial.println(packet_sent.src); // Printing source 05581 printf("Packet number: %d\n",packet_sent.packnum); 05582 // Serial.println(packet_sent.packnum); // Printing packet number 05583 printf("Packet length: %d\n",packet_sent.length); 05584 // Serial.println(packet_sent.length); // Printing packet length 05585 printf("Data: "); 05586 for(unsigned int i = 0; i < _payloadlength; i++) 05587 { 05588 //Serial.print((char)packet_sent.data[i]); // Printing payload 05589 printf("%c",packet_sent.data[i]); 05590 } 05591 printf("\n"); 05592 //printf("Retry number: "); 05593 //Serial.println(packet_sent.retry); // Printing retry number 05594 printf("##"); 05595 #endif 05596 } 05597 //writeRegister(REG_OP_MODE, st0); // Getting back to previous status 05598 return state; 05599 } 05600 05601 /* 05602 Function: Configures the module to transmit information. 05603 Returns: Integer that determines if there has been any error 05604 state = 2 --> The command has not been executed 05605 state = 1 --> There has been an error while executing the command 05606 state = 0 --> The command has been executed with no errors 05607 */ 05608 uint8_t SX1272::sendWithMAXTimeout() 05609 { 05610 return sendWithTimeout(MAX_TIMEOUT); 05611 } 05612 05613 /* 05614 Function: Configures the module to transmit information. 05615 Returns: Integer that determines if there has been any error 05616 state = 2 --> The command has not been executed 05617 state = 1 --> There has been an error while executing the command 05618 state = 0 --> The command has been executed with no errors 05619 */ 05620 uint8_t SX1272::sendWithTimeout() 05621 { 05622 setTimeout(); 05623 return sendWithTimeout(_sendTime); 05624 } 05625 05626 /* 05627 Function: Configures the module to transmit information. 05628 Returns: Integer that determines if there has been any error 05629 state = 2 --> The command has not been executed 05630 state = 1 --> There has been an error while executing the command 05631 state = 0 --> The command has been executed with no errors 05632 */ 05633 uint8_t SX1272::sendWithTimeout(uint16_t wait) 05634 { 05635 uint8_t state = 2; 05636 byte value = 0x00; 05637 //unsigned long previous; 05638 unsigned long exitTime; 05639 05640 #if (SX1272_debug_mode > 1) 05641 printf("\n"); 05642 printf("Starting 'sendWithTimeout'\n"); 05643 #endif 05644 05645 // clearFlags(); // Initializing flags 05646 05647 // wait to TxDone flag 05648 //previous = millis(); 05649 exitTime = millis() + (unsigned long)wait; 05650 if( _modem == LORA ) 05651 { // LoRa mode 05652 clearFlags(); // Initializing flags 05653 05654 writeRegister(REG_OP_MODE, LORA_TX_MODE); // LORA mode - Tx 05655 05656 #if (SX1272_debug_mode > 1) 05657 value = readRegister(REG_OP_MODE); 05658 05659 if (value & LORA_TX_MODE == LORA_TX_MODE) 05660 printf("OK"); 05661 else 05662 printf("ERROR"); 05663 #endif 05664 value = readRegister(REG_IRQ_FLAGS); 05665 // Wait until the packet is sent (TX Done flag) or the timeout expires 05666 //while ((bitRead(value, 3) == 0) && (millis() - previous < wait)) 05667 while ((bitRead(value, 3) == 0) && (millis() < exitTime)) 05668 { 05669 value = readRegister(REG_IRQ_FLAGS); 05670 // Condition to avoid an overflow (DO NOT REMOVE) 05671 //if( millis() < previous ) 05672 //{ 05673 // previous = millis(); 05674 //} 05675 } 05676 state = 1; 05677 } 05678 else 05679 { // FSK mode 05680 writeRegister(REG_OP_MODE, FSK_TX_MODE); // FSK mode - Tx 05681 05682 value = readRegister(REG_IRQ_FLAGS2); 05683 // Wait until the packet is sent (Packet Sent flag) or the timeout expires 05684 //while ((bitRead(value, 3) == 0) && (millis() - previous < wait)) 05685 while ((bitRead(value, 3) == 0) && (millis() < exitTime)) 05686 { 05687 value = readRegister(REG_IRQ_FLAGS2); 05688 // Condition to avoid an overflow (DO NOT REMOVE) 05689 //if( millis() < previous ) 05690 //{ 05691 // previous = millis(); 05692 //} 05693 } 05694 state = 1; 05695 } 05696 if( bitRead(value, 3) == 1 ) 05697 { 05698 state = 0; // Packet successfully sent 05699 #if (SX1272_debug_mode > 1) 05700 printf("## Packet successfully sent ##"); 05701 printf("\n"); 05702 #endif 05703 // added by C. Pham 05704 // normally there should be enough remaing ToA as the test has been done earlier 05705 if (_limitToA) 05706 removeToA(_currentToA); 05707 } 05708 else 05709 { 05710 if( state == 1 ) 05711 { 05712 #if (SX1272_debug_mode > 1) 05713 printf("** Timeout has expired **"); 05714 printf("\n"); 05715 #endif 05716 } 05717 else 05718 { 05719 #if (SX1272_debug_mode > 1) 05720 printf("** There has been an error and packet has not been sent **"); 05721 printf("\n"); 05722 #endif 05723 } 05724 } 05725 05726 clearFlags(); // Initializing flags 05727 return state; 05728 } 05729 05730 /* 05731 Function: Configures the module to transmit information. 05732 Returns: Integer that determines if there has been any error 05733 state = 2 --> The command has not been executed 05734 state = 1 --> There has been an error while executing the command 05735 state = 0 --> The command has been executed with no errors 05736 */ 05737 uint8_t SX1272::sendPacketMAXTimeout(uint8_t dest, char *payload) 05738 { 05739 return sendPacketTimeout(dest, payload, MAX_TIMEOUT); 05740 } 05741 05742 /* 05743 Function: Configures the module to transmit information. 05744 Returns: Integer that determines if there has been any error 05745 state = 2 --> The command has not been executed 05746 state = 1 --> There has been an error while executing the command 05747 state = 0 --> The command has been executed with no errors 05748 */ 05749 uint8_t SX1272::sendPacketMAXTimeout(uint8_t dest, uint8_t *payload, uint16_t length16) 05750 { 05751 return sendPacketTimeout(dest, payload, length16, MAX_TIMEOUT); 05752 } 05753 05754 /* 05755 Function: Configures the module to transmit information. 05756 Returns: Integer that determines if there has been any error 05757 state = 2 --> The command has not been executed 05758 state = 1 --> There has been an error while executing the command 05759 state = 0 --> The command has been executed with no errors 05760 */ 05761 uint8_t SX1272::sendPacketTimeout(uint8_t dest, char *payload) 05762 { 05763 uint8_t state = 2; 05764 05765 #if (SX1272_debug_mode > 1) 05766 printf("\n"); 05767 printf("Starting sendPacketTimeout\n"); 05768 #endif 05769 05770 state = setPacket(dest, payload); // Setting a packet with 'dest' destination 05771 printf("sendPacketTimeout saio da fifo\n"); 05772 if (state == 0) // and writing it in FIFO. 05773 { 05774 printf("sendPacketTimeout vai enviar\n"); 05775 state = sendWithTimeout(); // Sending the packet 05776 printf("sendPacketTimeout enviou\n"); 05777 } 05778 return state; 05779 } 05780 05781 /* 05782 Function: Configures the module to transmit information. 05783 Returns: Integer that determines if there has been any error 05784 state = 2 --> The command has not been executed 05785 state = 1 --> There has been an error while executing the command 05786 state = 0 --> The command has been executed with no errors 05787 */ 05788 uint8_t SX1272::sendPacketTimeout(uint8_t dest, uint8_t *payload, uint16_t length16) 05789 { 05790 uint8_t state = 2; 05791 uint8_t state_f = 2; 05792 // printf("chegou no sendPacketTimeout\n"); 05793 #if (SX1272_debug_mode > 1) 05794 printf("\n"); 05795 printf("Starting 'sendPacketTimeout'\n"); 05796 #endif 05797 state = truncPayload(length16); 05798 if( state == 0 ) 05799 { 05800 // printf("vai pro setpacket\n"); 05801 state_f = setPacket(dest, payload); // Setting a packet with 'dest' destination 05802 } // and writing it in FIFO. 05803 else 05804 { 05805 state_f = state; 05806 } 05807 if( state_f == 0 ) 05808 { 05809 state_f = sendWithTimeout(); // Sending the packet 05810 } 05811 return state_f; 05812 } 05813 05814 /* 05815 Function: Configures the module to transmit information. 05816 Returns: Integer that determines if there has been any error 05817 state = 2 --> The command has not been executed 05818 state = 1 --> There has been an error while executing the command 05819 state = 0 --> The command has been executed with no errors 05820 */ 05821 uint8_t SX1272::sendPacketTimeout(uint8_t dest, char *payload, uint16_t wait) 05822 { 05823 uint8_t state = 2; 05824 05825 #if (SX1272_debug_mode > 1) 05826 printf("\n"); 05827 printf("Starting 'sendPacketTimeout'\n"); 05828 #endif 05829 05830 state = setPacket(dest, payload); // Setting a packet with 'dest' destination 05831 if (state == 0) // and writing it in FIFO. 05832 { 05833 state = sendWithTimeout(wait); // Sending the packet 05834 } 05835 return state; 05836 } 05837 05838 /* 05839 Function: Configures the module to transmit information. 05840 Returns: Integer that determines if there has been any error 05841 state = 2 --> The command has not been executed 05842 state = 1 --> There has been an error while executing the command 05843 state = 0 --> The command has been executed with no errors 05844 */ 05845 uint8_t SX1272::sendPacketTimeout(uint8_t dest, uint8_t *payload, uint16_t length16, uint16_t wait) 05846 { 05847 uint8_t state = 2; 05848 uint8_t state_f = 2; 05849 05850 #if (SX1272_debug_mode > 1) 05851 printf("\n"); 05852 printf("Starting 'sendPacketTimeout'\n"); 05853 #endif 05854 05855 state = truncPayload(length16); 05856 if( state == 0 ) 05857 { 05858 state_f = setPacket(dest, payload); // Setting a packet with 'dest' destination 05859 } 05860 else 05861 { 05862 state_f = state; 05863 } 05864 if( state_f == 0 ) // and writing it in FIFO. 05865 { 05866 state_f = sendWithTimeout(wait); // Sending the packet 05867 } 05868 return state_f; 05869 } 05870 05871 /* 05872 Function: Configures the module to transmit information. 05873 Returns: Integer that determines if there has been any error 05874 state = 2 --> The command has not been executed 05875 state = 1 --> There has been an error while executing the command 05876 state = 0 --> The command has been executed with no errors 05877 */ 05878 uint8_t SX1272::sendPacketMAXTimeoutACK(uint8_t dest, char *payload) 05879 { 05880 return sendPacketTimeoutACK(dest, payload, MAX_TIMEOUT); 05881 } 05882 05883 /* 05884 Function: Configures the module to transmit information and receive an ACK. 05885 Returns: Integer that determines if there has been any error 05886 state = 2 --> The command has not been executed 05887 state = 1 --> There has been an error while executing the command 05888 state = 0 --> The command has been executed with no errors 05889 */ 05890 uint8_t SX1272::sendPacketMAXTimeoutACK(uint8_t dest, uint8_t *payload, uint16_t length16) 05891 { 05892 return sendPacketTimeoutACK(dest, payload, length16, MAX_TIMEOUT); 05893 } 05894 05895 /* 05896 Function: Configures the module to transmit information and receive an ACK. 05897 Returns: Integer that determines if there has been any error 05898 state = 3 --> Packet has been sent but ACK has not been received 05899 state = 2 --> The command has not been executed 05900 state = 1 --> There has been an error while executing the command 05901 state = 0 --> The command has been executed with no errors 05902 */ 05903 uint8_t SX1272::sendPacketTimeoutACK(uint8_t dest, char *payload) 05904 { 05905 uint8_t state = 2; 05906 uint8_t state_f = 2; 05907 05908 #if (SX1272_debug_mode > 1) 05909 printf("\n"); 05910 printf("Starting 'sendPacketTimeoutACK'\n"); 05911 #endif 05912 05913 #ifdef W_REQUESTED_ACK 05914 _requestACK = 1; 05915 #endif 05916 state = sendPacketTimeout(dest, payload); // Sending packet to 'dest' destination 05917 05918 if( state == 0 ) 05919 { 05920 state = receive(); // Setting Rx mode to wait an ACK 05921 } 05922 else 05923 { 05924 state_f = state; 05925 } 05926 if( state == 0 ) 05927 { 05928 // added by C. Pham 05929 printf("wait for ACK"); 05930 05931 if( availableData() ) 05932 { 05933 state_f = getACK(); // Getting ACK 05934 } 05935 else 05936 { 05937 state_f = SX1272_ERROR_ACK; 05938 // added by C. Pham 05939 printf("no ACK"); 05940 } 05941 } 05942 else 05943 { 05944 state_f = state; 05945 } 05946 05947 #ifdef W_REQUESTED_ACK 05948 _requestACK = 0; 05949 #endif 05950 return state_f; 05951 } 05952 05953 /* 05954 Function: Configures the module to transmit information and receive an ACK. 05955 Returns: Integer that determines if there has been any error 05956 state = 3 --> Packet has been sent but ACK has not been received 05957 state = 2 --> The command has not been executed 05958 state = 1 --> There has been an error while executing the command 05959 state = 0 --> The command has been executed with no errors 05960 */ 05961 uint8_t SX1272::sendPacketTimeoutACK(uint8_t dest, uint8_t *payload, uint16_t length16) 05962 { 05963 uint8_t state = 2; 05964 uint8_t state_f = 2; 05965 05966 #if (SX1272_debug_mode > 1) 05967 printf("\n"); 05968 printf("Starting 'sendPacketTimeoutACK'\n"); 05969 #endif 05970 05971 #ifdef W_REQUESTED_ACK 05972 _requestACK = 1; 05973 #endif 05974 // Sending packet to 'dest' destination 05975 state = sendPacketTimeout(dest, payload, length16); 05976 05977 // Trying to receive the ACK 05978 if( state == 0 ) 05979 { 05980 state = receive(); // Setting Rx mode to wait an ACK 05981 } 05982 else 05983 { 05984 state_f = state; 05985 } 05986 if( state == 0 ) 05987 { 05988 // added by C. Pham 05989 printf("wait for ACK"); 05990 05991 if( availableData() ) 05992 { 05993 state_f = getACK(); // Getting ACK 05994 } 05995 else 05996 { 05997 state_f = SX1272_ERROR_ACK; 05998 // added by C. Pham 05999 printf("no ACK"); 06000 } 06001 } 06002 else 06003 { 06004 state_f = state; 06005 } 06006 06007 #ifdef W_REQUESTED_ACK 06008 _requestACK = 0; 06009 #endif 06010 return state_f; 06011 } 06012 06013 /* 06014 Function: Configures the module to transmit information and receive an ACK. 06015 Returns: Integer that determines if there has been any error 06016 state = 3 --> Packet has been sent but ACK has not been received 06017 state = 2 --> The command has not been executed 06018 state = 1 --> There has been an error while executing the command 06019 state = 0 --> The command has been executed with no errors 06020 */ 06021 uint8_t SX1272::sendPacketTimeoutACK(uint8_t dest, char *payload, uint16_t wait) 06022 { 06023 uint8_t state = 2; 06024 uint8_t state_f = 2; 06025 06026 #if (SX1272_debug_mode > 1) 06027 printf("\n"); 06028 printf("Starting 'sendPacketTimeoutACK'\n"); 06029 #endif 06030 06031 #ifdef W_REQUESTED_ACK 06032 _requestACK = 1; 06033 #endif 06034 state = sendPacketTimeout(dest, payload, wait); // Sending packet to 'dest' destination 06035 06036 if( state == 0 ) 06037 { 06038 state = receive(); // Setting Rx mode to wait an ACK 06039 } 06040 else 06041 { 06042 state_f = 1; 06043 } 06044 if( state == 0 ) 06045 { 06046 // added by C. Pham 06047 printf("wait for ACK"); 06048 06049 if( availableData() ) 06050 { 06051 state_f = getACK(); // Getting ACK 06052 } 06053 else 06054 { 06055 state_f = SX1272_ERROR_ACK; 06056 // added by C. Pham 06057 printf("no ACK"); 06058 } 06059 } 06060 else 06061 { 06062 state_f = 1; 06063 } 06064 06065 #ifdef W_REQUESTED_ACK 06066 _requestACK = 0; 06067 #endif 06068 return state_f; 06069 } 06070 06071 /* 06072 Function: Configures the module to transmit information and receive an ACK. 06073 Returns: Integer that determines if there has been any error 06074 state = 3 --> Packet has been sent but ACK has not been received 06075 state = 2 --> The command has not been executed 06076 state = 1 --> There has been an error while executing the command 06077 state = 0 --> The command has been executed with no errors 06078 */ 06079 uint8_t SX1272::sendPacketTimeoutACK(uint8_t dest, uint8_t *payload, uint16_t length16, uint16_t wait) 06080 { 06081 uint8_t state = 2; 06082 uint8_t state_f = 2; 06083 06084 #if (SX1272_debug_mode > 1) 06085 printf("\n"); 06086 printf("Starting 'sendPacketTimeoutACK'\n"); 06087 #endif 06088 06089 #ifdef W_REQUESTED_ACK 06090 _requestACK = 1; 06091 #endif 06092 state = sendPacketTimeout(dest, payload, length16, wait); // Sending packet to 'dest' destination 06093 06094 if( state == 0 ) 06095 { 06096 state = receive(); // Setting Rx mode to wait an ACK 06097 } 06098 else 06099 { 06100 state_f = 1; 06101 } 06102 if( state == 0 ) 06103 { 06104 // added by C. Pham 06105 printf("wait for ACK"); 06106 06107 if( availableData() ) 06108 { 06109 state_f = getACK(); // Getting ACK 06110 } 06111 else 06112 { 06113 state_f = SX1272_ERROR_ACK; 06114 // added by C. Pham 06115 printf("no ACK"); 06116 } 06117 } 06118 else 06119 { 06120 state_f = 1; 06121 } 06122 06123 #ifdef W_REQUESTED_ACK 06124 _requestACK = 0; 06125 #endif 06126 return state_f; 06127 } 06128 06129 /* 06130 Function: It gets and stores an ACK if it is received. 06131 Returns: 06132 */ 06133 uint8_t SX1272::getACK() 06134 { 06135 return getACK(MAX_TIMEOUT); 06136 } 06137 06138 /* 06139 Function: It gets and stores an ACK if it is received, before ending 'wait' time. 06140 Returns: Integer that determines if there has been any error 06141 state = 2 --> The ACK has not been received 06142 state = 1 --> The N-ACK has been received with no errors 06143 state = 0 --> The ACK has been received with no errors 06144 Parameters: 06145 wait: time to wait while there is no a valid header received. 06146 */ 06147 uint8_t SX1272::getACK(uint16_t wait) 06148 { 06149 uint8_t state = 2; 06150 byte value = 0x00; 06151 //unsigned long previous; 06152 unsigned long exitTime; 06153 boolean a_received = false; 06154 06155 //#if (SX1272_debug_mode > 1) 06156 printf("\n"); 06157 printf("Starting 'getACK'\n"); 06158 //#endif 06159 06160 //previous = millis(); 06161 exitTime = millis()+(unsigned long)wait; 06162 if( _modem == LORA ) 06163 { // LoRa mode 06164 value = readRegister(REG_IRQ_FLAGS); 06165 // Wait until the ACK is received (RxDone flag) or the timeout expires 06166 //while ((bitRead(value, 6) == 0) && (millis() - previous < wait)) 06167 while ((bitRead(value, 6) == 0) && (millis() < exitTime)) 06168 { 06169 value = readRegister(REG_IRQ_FLAGS); 06170 //if( millis() < previous ) 06171 //{ 06172 // previous = millis(); 06173 //} 06174 } 06175 if( bitRead(value, 6) == 1 ) 06176 { // ACK received 06177 // comment by C. Pham 06178 // not really safe because the received packet may not be an ACK 06179 // probability is low if using unicast to gateway, but if broadcast 06180 // can get a packet from another node!! 06181 a_received = true; 06182 } 06183 // Standby para minimizar el consumo 06184 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Setting standby LoRa mode 06185 } 06186 else 06187 { // FSK mode 06188 value = readRegister(REG_IRQ_FLAGS2); 06189 // Wait until the packet is received (RxDone flag) or the timeout expires 06190 //while ((bitRead(value, 2) == 0) && (millis() - previous < wait)) 06191 while ((bitRead(value, 2) == 0) && (millis() < exitTime)) 06192 { 06193 value = readRegister(REG_IRQ_FLAGS2); 06194 //if( millis() < previous ) 06195 //{ 06196 // previous = millis(); 06197 //} 06198 } 06199 if( bitRead(value, 2) == 1 ) 06200 { // ACK received 06201 a_received = true; 06202 } 06203 // Standby para minimizar el consumo 06204 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); // Setting standby FSK mode 06205 } 06206 06207 // comment by C. Pham 06208 // not safe because the received packet may not be an ACK! 06209 if( a_received ) 06210 { 06211 // Storing the received ACK 06212 ACK.dst = _destination; 06213 ACK.type = readRegister(REG_FIFO); 06214 ACK.src = readRegister(REG_FIFO); 06215 ACK.packnum = readRegister(REG_FIFO); 06216 ACK.length = readRegister(REG_FIFO); 06217 ACK.data[0] = readRegister(REG_FIFO); 06218 ACK.data[1] = readRegister(REG_FIFO); 06219 06220 if (ACK.type == PKT_TYPE_ACK) { 06221 06222 // Checking the received ACK 06223 if( ACK.dst == packet_sent.src ) 06224 { 06225 if( ACK.src == packet_sent.dst ) 06226 { 06227 if( ACK.packnum == packet_sent.packnum ) 06228 { 06229 if( ACK.length == 2 ) 06230 { 06231 if( ACK.data[0] == CORRECT_PACKET ) 06232 { 06233 state = 0; 06234 //#if (SX1272_debug_mode > 0) 06235 // Printing the received ACK 06236 printf("## ACK received:"); 06237 printf("Destination: %d\n",ACK.dst); 06238 // Serial.println(ACK.dst); // Printing destination 06239 printf("Source: %d\n",ACK.src); 06240 // Serial.println(ACK.src); // Printing source 06241 printf("ACK number: %d\n",ACK.packnum); 06242 // Serial.println(ACK.packnum); // Printing ACK number 06243 printf("ACK length: %d\n",ACK.length); 06244 // Serial.println(ACK.length); // Printing ACK length 06245 printf("ACK payload: %d\n",ACK.data[0]); 06246 // Serial.println(ACK.data[0]); // Printing ACK payload 06247 printf("ACK SNR of rcv pkt at gw: "); 06248 06249 value = ACK.data[1]; 06250 06251 if( value & 0x80 ) // The SNR sign bit is 1 06252 { 06253 // Invert and divide by 4 06254 value = ( ( ~value + 1 ) & 0xFF ) >> 2; 06255 _rcv_snr_in_ack = -value; 06256 } 06257 else 06258 { 06259 // Divide by 4 06260 _rcv_snr_in_ack = ( value & 0xFF ) >> 2; 06261 } 06262 06263 //Serial.println(_rcv_snr_in_ack); 06264 printf("%d\n",_rcv_snr_in_ack); 06265 printf("##"); 06266 printf("\n"); 06267 //#endif 06268 } 06269 else 06270 { 06271 state = 1; 06272 //#if (SX1272_debug_mode > 0) 06273 printf("** N-ACK received **"); 06274 printf("\n"); 06275 //#endif 06276 } 06277 } 06278 else 06279 { 06280 state = 1; 06281 //#if (SX1272_debug_mode > 0) 06282 printf("** ACK length incorrectly received **"); 06283 printf("\n"); 06284 //#endif 06285 } 06286 } 06287 else 06288 { 06289 state = 1; 06290 //#if (SX1272_debug_mode > 0) 06291 printf("** ACK number incorrectly received **"); 06292 printf("\n"); 06293 //#endif 06294 } 06295 } 06296 else 06297 { 06298 state = 1; 06299 //#if (SX1272_debug_mode > 0) 06300 printf("** ACK source incorrectly received **"); 06301 printf("\n"); 06302 //#endif 06303 } 06304 } 06305 } 06306 else 06307 { 06308 state = 1; 06309 //#if (SX1272_debug_mode > 0) 06310 printf("** ACK destination incorrectly received **"); 06311 printf("\n"); 06312 //#endif 06313 } 06314 } 06315 else 06316 { 06317 state = 1; 06318 //#if (SX1272_debug_mode > 0) 06319 printf("** ACK lost **"); 06320 printf("\n"); 06321 //#endif 06322 } 06323 clearFlags(); // Initializing flags 06324 return state; 06325 } 06326 06327 /* 06328 Function: Configures the module to transmit information with retries in case of error. 06329 Returns: Integer that determines if there has been any error 06330 state = 2 --> The command has not been executed 06331 state = 1 --> There has been an error while executing the command 06332 state = 0 --> The command has been executed with no errors 06333 */ 06334 uint8_t SX1272::sendPacketMAXTimeoutACKRetries(uint8_t dest, char *payload) 06335 { 06336 return sendPacketTimeoutACKRetries(dest, payload, MAX_TIMEOUT); 06337 } 06338 06339 /* 06340 Function: Configures the module to transmit information with retries in case of error. 06341 Returns: Integer that determines if there has been any error 06342 state = 2 --> The command has not been executed 06343 state = 1 --> There has been an error while executing the command 06344 state = 0 --> The command has been executed with no errors 06345 */ 06346 uint8_t SX1272::sendPacketMAXTimeoutACKRetries(uint8_t dest, uint8_t *payload, uint16_t length16) 06347 { 06348 return sendPacketTimeoutACKRetries(dest, payload, length16, MAX_TIMEOUT); 06349 } 06350 06351 /* 06352 Function: Configures the module to transmit information with retries in case of error. 06353 Returns: Integer that determines if there has been any error 06354 state = 2 --> The command has not been executed 06355 state = 1 --> There has been an error while executing the command 06356 state = 0 --> The command has been executed with no errors 06357 */ 06358 uint8_t SX1272::sendPacketTimeoutACKRetries(uint8_t dest, char *payload) 06359 { 06360 uint8_t state = 2; 06361 06362 #if (SX1272_debug_mode > 1) 06363 printf("\n"); 06364 printf("Starting 'sendPacketTimeoutACKRetries'\n"); 06365 #endif 06366 06367 // Sending packet to 'dest' destination and waiting an ACK response. 06368 state = 1; 06369 while( (state != 0) && (_retries <= _maxRetries) ) 06370 { 06371 state = sendPacketTimeoutACK(dest, payload); 06372 _retries++; 06373 } 06374 _retries = 0; 06375 06376 return state; 06377 } 06378 06379 /* 06380 Function: Configures the module to transmit information with retries in case of error. 06381 Returns: Integer that determines if there has been any error 06382 state = 2 --> The command has not been executed 06383 state = 1 --> There has been an error while executing the command 06384 state = 0 --> The command has been executed with no errors 06385 */ 06386 uint8_t SX1272::sendPacketTimeoutACKRetries(uint8_t dest, uint8_t *payload, uint16_t length16) 06387 { 06388 uint8_t state = 2; 06389 06390 #if (SX1272_debug_mode > 1) 06391 printf("\n"); 06392 printf("Starting 'sendPacketTimeoutACKRetries'\n"); 06393 #endif 06394 06395 // Sending packet to 'dest' destination and waiting an ACK response. 06396 state = 1; 06397 while((state != 0) && (_retries <= _maxRetries)) 06398 { 06399 state = sendPacketTimeoutACK(dest, payload, length16); 06400 _retries++; 06401 06402 } 06403 _retries = 0; 06404 06405 return state; 06406 } 06407 06408 /* 06409 Function: Configures the module to transmit information with retries in case of error. 06410 Returns: Integer that determines if there has been any error 06411 state = 2 --> The command has not been executed 06412 state = 1 --> There has been an error while executing the command 06413 state = 0 --> The command has been executed with no errors 06414 */ 06415 uint8_t SX1272::sendPacketTimeoutACKRetries(uint8_t dest, char *payload, uint16_t wait) 06416 { 06417 uint8_t state = 2; 06418 06419 #if (SX1272_debug_mode > 1) 06420 printf("\n"); 06421 printf("Starting 'sendPacketTimeoutACKRetries'\n"); 06422 #endif 06423 06424 // Sending packet to 'dest' destination and waiting an ACK response. 06425 state = 1; 06426 while((state != 0) && (_retries <= _maxRetries)) 06427 { 06428 state = sendPacketTimeoutACK(dest, payload, wait); 06429 _retries++; 06430 } 06431 _retries = 0; 06432 06433 return state; 06434 } 06435 06436 /* 06437 Function: Configures the module to transmit information with retries in case of error. 06438 Returns: Integer that determines if there has been any error 06439 state = 2 --> The command has not been executed 06440 state = 1 --> There has been an error while executing the command 06441 state = 0 --> The command has been executed with no errors 06442 */ 06443 uint8_t SX1272::sendPacketTimeoutACKRetries(uint8_t dest, uint8_t *payload, uint16_t length16, uint16_t wait) 06444 { 06445 uint8_t state = 2; 06446 06447 #if (SX1272_debug_mode > 1) 06448 printf("\n"); 06449 printf("Starting 'sendPacketTimeoutACKRetries'\n"); 06450 #endif 06451 06452 // Sending packet to 'dest' destination and waiting an ACK response. 06453 state = 1; 06454 while((state != 0) && (_retries <= _maxRetries)) 06455 { 06456 state = sendPacketTimeoutACK(dest, payload, length16, wait); 06457 _retries++; 06458 } 06459 _retries = 0; 06460 06461 return state; 06462 } 06463 06464 /* 06465 Function: It gets the temperature from the measurement block module. 06466 Returns: Integer that determines if there has been any error 06467 state = 2 --> The command has not been executed 06468 state = 1 --> There has been an error while executing the command 06469 state = 0 --> The command has been executed with no errors 06470 */ 06471 uint8_t SX1272::getTemp() 06472 { 06473 byte st0; 06474 uint8_t state = 2; 06475 06476 #if (SX1272_debug_mode > 1) 06477 printf("\n"); 06478 printf("Starting 'getTemp'\n"); 06479 #endif 06480 06481 st0 = readRegister(REG_OP_MODE); // Save the previous status 06482 06483 if( _modem == LORA ) 06484 { // Allowing access to FSK registers while in LoRa standby mode 06485 writeRegister(REG_OP_MODE, LORA_STANDBY_FSK_REGS_MODE); 06486 } 06487 06488 state = 1; 06489 // Saving temperature value 06490 _temp = readRegister(REG_TEMP); 06491 if( _temp & 0x80 ) // The SNR sign bit is 1 06492 { 06493 // Invert and divide by 4 06494 _temp = ( ( ~_temp + 1 ) & 0xFF ); 06495 } 06496 else 06497 { 06498 // Divide by 4 06499 _temp = ( _temp & 0xFF ); 06500 } 06501 06502 06503 #if (SX1272_debug_mode > 1) 06504 printf("## Temperature is: %d ",_temp); 06505 Serial.print(_temp); 06506 printf(" ##"); 06507 printf("\n"); 06508 #endif 06509 06510 if( _modem == LORA ) 06511 { 06512 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 06513 } 06514 06515 state = 0; 06516 return state; 06517 } 06518 06519 //**********************************************************************/ 06520 // Added by C. Pham 06521 //**********************************************************************/ 06522 06523 void SX1272::setPacketType(uint8_t type) 06524 { 06525 packet_sent.type=type; 06526 06527 if (type & PKT_FLAG_ACK_REQ) 06528 _requestACK=1; 06529 } 06530 06531 /* 06532 Function: Configures the module to perform CAD. 06533 Returns: Integer that determines if the number of requested CAD have been successfull 06534 state = 2 --> The command has not been executed 06535 state = 1 --> There has been an error while executing the command 06536 state = 0 --> The command has been executed with no errors 06537 */ 06538 uint8_t SX1272::doCAD(uint8_t counter) 06539 { 06540 uint8_t state = 2; 06541 byte value = 0x00; 06542 unsigned long startCAD, endCAD, startDoCad, endDoCad; 06543 //unsigned long previous; 06544 unsigned long exitTime; 06545 uint16_t wait = 100; 06546 bool failedCAD=false; 06547 uint8_t retryCAD = 3; 06548 uint8_t save_counter; 06549 byte st0; 06550 int rssi_count=0; 06551 int rssi_mean=0; 06552 double bw=0.0; 06553 bool hasRSSI=false; 06554 unsigned long startRSSI=0; 06555 06556 bw=(_bandwidth==BW_125)?125e3:((_bandwidth==BW_250)?250e3:500e3); 06557 // Symbol rate : time for one symbol (usecs) 06558 double rs = bw / ( 1 << _spreadingFactor); 06559 double ts = 1 / rs; 06560 ts = ts * 1000000.0; 06561 06562 st0 = readRegister(REG_OP_MODE); // Save the previous status 06563 06564 #ifdef DEBUG_CAD 06565 printf("SX1272::Starting 'doCAD'\n"); 06566 #endif 06567 06568 save_counter = counter; 06569 06570 startDoCad=millis(); 06571 06572 if( _modem == LORA ) { // LoRa mode 06573 06574 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); 06575 06576 do { 06577 06578 hasRSSI=false; 06579 06580 clearFlags(); // Initializing flags 06581 06582 // wait to CadDone flag 06583 // previous = millis(); 06584 startCAD = millis(); 06585 exitTime = millis()+(unsigned long)wait; 06586 06587 writeRegister(REG_OP_MODE, LORA_CAD_MODE); // LORA mode - Cad 06588 06589 startRSSI=micros(); 06590 06591 value = readRegister(REG_IRQ_FLAGS); 06592 // Wait until CAD ends (CAD Done flag) or the timeout expires 06593 //while ((bitRead(value, 2) == 0) && (millis() - previous < wait)) 06594 while ((bitRead(value, 2) == 0) && (millis() < exitTime)) 06595 { 06596 // only one reading per CAD 06597 if (micros()-startRSSI > ts+240 && !hasRSSI) { 06598 _RSSI = -(OFFSET_RSSI+(_board==SX1276Chip?18:0)) + readRegister(REG_RSSI_VALUE_LORA); 06599 rssi_mean += _RSSI; 06600 rssi_count++; 06601 hasRSSI=true; 06602 } 06603 06604 value = readRegister(REG_IRQ_FLAGS); 06605 // Condition to avoid an overflow (DO NOT REMOVE) 06606 //if( millis() < previous ) 06607 //{ 06608 // previous = millis(); 06609 //} 06610 } 06611 state = 1; 06612 06613 endCAD = millis(); 06614 06615 if( bitRead(value, 2) == 1 ) 06616 { 06617 state = 0; // CAD successfully performed 06618 #ifdef DEBUG_CAD 06619 printf("SX1272::CAD duration %X\n",endCAD-startCAD); 06620 06621 // Serial.println(endCAD-startCAD); 06622 printf("SX1272::CAD successfully performed"); 06623 #endif 06624 06625 value = readRegister(REG_IRQ_FLAGS); 06626 06627 // look for the CAD detected bit 06628 if( bitRead(value, 0) == 1 ) 06629 { 06630 // we detected activity 06631 failedCAD=true; 06632 #ifdef DEBUG_CAD 06633 printf("SX1272::CAD exits after %d\n",save_counter-counter); 06634 // Serial.println(save_counter-counter); 06635 #endif 06636 } 06637 06638 counter--; 06639 } 06640 else 06641 { 06642 #ifdef DEBUG_CAD 06643 printf("SX1272::CAD duration %d\n",endCAD-startCAD); 06644 // Serial.println(endCAD-startCAD); 06645 #endif 06646 if( state == 1 ) 06647 { 06648 #ifdef DEBUG_CAD 06649 printf("SX1272::Timeout has expired"); 06650 #endif 06651 } 06652 else 06653 { 06654 #ifdef DEBUG_CAD 06655 printf("SX1272::Error and CAD has not been performed"); 06656 #endif 06657 } 06658 06659 retryCAD--; 06660 06661 // to many errors, so exit by indicating that channel is not free 06662 if (!retryCAD) 06663 failedCAD=true; 06664 } 06665 06666 } while (counter && !failedCAD); 06667 06668 rssi_mean = rssi_mean / rssi_count; 06669 _RSSI = rssi_mean; 06670 } 06671 06672 writeRegister(REG_OP_MODE, st0); 06673 06674 endDoCad=millis(); 06675 06676 clearFlags(); // Initializing flags 06677 06678 #ifdef DEBUG_CAD 06679 printf("SX1272::doCAD duration %d\n",endDoCad-startDoCad); 06680 // Serial.println(endDoCad-startDoCad); 06681 #endif 06682 06683 if (failedCAD) 06684 return 2; 06685 06686 return state; 06687 } 06688 06689 //#define DEBUG_GETTOA 06690 06691 #ifdef DEBUG_GETTOA 06692 06693 void printDouble( double val, byte precision){ 06694 // prints val with number of decimal places determine by precision 06695 // precision is a number from 0 to 6 indicating the desired decimial places 06696 // example: lcdPrintDouble( 3.1415, 2); // prints 3.14 (two decimal places) 06697 06698 if(val < 0.0){ 06699 Serial.print('-'); 06700 val = -val; 06701 } 06702 06703 Serial.print (int(val)); //prints the int part 06704 if( precision > 0) { 06705 Serial.print("."); // print the decimal point 06706 unsigned long frac; 06707 unsigned long mult = 1; 06708 byte padding = precision -1; 06709 while(precision--) 06710 mult *=10; 06711 06712 if(val >= 0) 06713 frac = (val - int(val)) * mult; 06714 else 06715 frac = (int(val)- val ) * mult; 06716 unsigned long frac1 = frac; 06717 while( frac1 /= 10 ) 06718 padding--; 06719 while( padding--) 06720 Serial.print("0"); 06721 printfrac,DEC) ; 06722 } 06723 } 06724 06725 #endif 06726 06727 uint16_t SX1272::getToA(uint8_t pl) { 06728 06729 uint8_t DE = 0; 06730 uint32_t airTime = 0; 06731 06732 double bw=0.0; 06733 06734 bw=(_bandwidth==BW_125)?125e3:((_bandwidth==BW_250)?250e3:500e3); 06735 06736 #ifdef DEBUG_GETTOA 06737 printf("SX1272::bw is "); 06738 Serial.println(bw); 06739 06740 printf("SX1272::SF is "); 06741 Serial.println(_spreadingFactor); 06742 #endif 06743 06744 //double ts=pow(2,_spreadingFactor)/bw; 06745 06746 ////// from LoRaMAC SX1272GetTimeOnAir() 06747 06748 // Symbol rate : time for one symbol (secs) 06749 double rs = bw / ( 1 << _spreadingFactor); 06750 double ts = 1 / rs; 06751 06752 // must add 4 to the programmed preamble length to get the effective preamble length 06753 double tPreamble=((_preamblelength+4)+4.25)*ts; 06754 06755 #ifdef DEBUG_GETTOA 06756 printf("SX1272::ts is "); 06757 printDouble(ts,6); 06758 printf("\n"); 06759 printf("SX1272::tPreamble is "); 06760 printDouble(tPreamble,6); 06761 printf("\n"); 06762 #endif 06763 06764 // for low data rate optimization 06765 if ((_bandwidth == BW_125) && _spreadingFactor == 12) 06766 DE = 1; 06767 06768 // Symbol length of payload and time 06769 double tmp = (8*pl - 4*_spreadingFactor + 28 + 16 - 20*_header) / 06770 (double)(4*(_spreadingFactor-2*DE) ); 06771 06772 #ifdef DEBUG_GETTOA 06773 printf("SX1272::tmp is "); 06774 printDouble(tmp,6); 06775 printf("\n"); 06776 #endif 06777 06778 tmp = ceil(tmp)*(_codingRate + 4); 06779 06780 double nPayload = 8 + ( ( tmp > 0 ) ? tmp : 0 ); 06781 06782 #ifdef DEBUG_GETTOA 06783 printf("SX1272::nPayload is "); 06784 Serial.println(nPayload); 06785 #endif 06786 06787 double tPayload = nPayload * ts; 06788 // Time on air 06789 double tOnAir = tPreamble + tPayload; 06790 // in us secs 06791 airTime = floor( tOnAir * 1e6 + 0.999 ); 06792 06793 ////// 06794 06795 #ifdef DEBUG_GETTOA 06796 printf("SX1272::airTime is "); 06797 Serial.println(airTime); 06798 #endif 06799 // return in ms 06800 // Modifie par C.Dupaty A VERIFIER !!!!!!!!!!!!!!!!!!!!!!!!! 06801 // _currentToA=ceil(airTime/1000)+1; 06802 _currentToA=(airTime/1000)+1; 06803 return _currentToA; 06804 } 06805 06806 // need to set _send_cad_number to a value > 0 06807 // we advise using _send_cad_number=3 for a SIFS and _send_cad_number=9 for a DIFS 06808 // prior to send any data 06809 // TODO: have a maximum number of trials 06810 void SX1272::CarrierSense() { 06811 06812 int e; 06813 bool carrierSenseRetry=false; 06814 06815 if (_send_cad_number && _enableCarrierSense) { 06816 do { 06817 do { 06818 06819 // check for free channel (SIFS/DIFS) 06820 _startDoCad=millis(); 06821 e = doCAD(_send_cad_number); 06822 _endDoCad=millis(); 06823 06824 // printf("--> CAD duration %d\n",_endDoCad-_startDoCad); 06825 // Serial.print(_endDoCad-_startDoCad); 06826 // printf("\n"); 06827 06828 if (!e) { 06829 // printf("OK1\n"); 06830 06831 if (_extendedIFS) { 06832 // wait for random number of CAD 06833 // uint8_t w = random(1,8); 06834 // ajoute par C.Dupaty 06835 uint8_t w = rand()%8+1; 06836 // printf("--> waiting for %d\n",w); 06837 // Serial.print(w); 06838 // printf(" CAD = %d\n",sx1272_CAD_value[_loraMode]*w); 06839 // Serial.print(sx1272_CAD_value[_loraMode]*w); 06840 printf("\n"); 06841 06842 wait_ms(sx1272_CAD_value[_loraMode]*w); 06843 06844 // check for free channel (SIFS/DIFS) once again 06845 _startDoCad=millis(); 06846 e = doCAD(_send_cad_number); 06847 _endDoCad=millis(); 06848 06849 // printf("--> CAD duration %d\n",_endDoCad-_startDoCad); 06850 // Serial.print(_endDoCad-_startDoCad); 06851 // printf("\n"); 06852 06853 // if (!e) 06854 //// printf("OK2"); 06855 // else 06856 // printf("###2"); 06857 // 06858 // printf("\n"); 06859 } 06860 } 06861 else { 06862 printf("###1\n"); 06863 06864 // wait for random number of DIFS 06865 uint8_t w = rand()%8+1; 06866 06867 // printf("--> waiting for %d\n",w); 06868 // Serial.print(w); 06869 printf(" DIFS (DIFS=3SIFS) = %d\n",sx1272_SIFS_value[_loraMode]*3*w); 06870 // Serial.print(sx1272_SIFS_value[_loraMode]*3*w); 06871 printf("\n"); 06872 06873 wait_ms(sx1272_SIFS_value[_loraMode]*3*w); 06874 06875 printf("--> retry\n"); 06876 } 06877 06878 } while (e); 06879 06880 // CAD is OK, but need to check RSSI 06881 if (_RSSIonSend) { 06882 06883 e=getRSSI(); 06884 06885 uint8_t rssi_retry_count=10; 06886 06887 if (!e) { 06888 06889 printf("--> RSSI %d\n",_RSSI); 06890 //Serial.print(_RSSI); 06891 printf("\n"); 06892 06893 while (_RSSI > -90 && rssi_retry_count) { 06894 06895 wait_ms(1); 06896 getRSSI(); 06897 printf("--> RSSI %d\n",_RSSI); 06898 //Serial.print(_RSSI); 06899 printf("\n"); 06900 rssi_retry_count--; 06901 } 06902 } 06903 else 06904 printf("--> RSSI error\n"); 06905 06906 if (!rssi_retry_count) 06907 carrierSenseRetry=true; 06908 else 06909 carrierSenseRetry=false; 06910 } 06911 06912 } while (carrierSenseRetry); 06913 } 06914 } 06915 06916 /* 06917 Function: Indicates the CR within the module is configured. 06918 Returns: Integer that determines if there has been any error 06919 state = 2 --> The command has not been executed 06920 state = 1 --> There has been an error while executing the command 06921 state = 0 --> The command has been executed with no errors 06922 state = -1 --> Forbidden command for this protocol 06923 */ 06924 int8_t SX1272::getSyncWord() 06925 { 06926 int8_t state = 2; 06927 06928 #if (SX1272_debug_mode > 1) 06929 printf("\n"); 06930 printf("Starting 'getSyncWord'\n"); 06931 #endif 06932 06933 if( _modem == FSK ) 06934 { 06935 state = -1; // sync word is not available in FSK mode 06936 #if (SX1272_debug_mode > 1) 06937 printf("** FSK mode hasn't sync word **"); 06938 printf("\n"); 06939 #endif 06940 } 06941 else 06942 { 06943 _syncWord = readRegister(REG_SYNC_WORD); 06944 06945 state = 0; 06946 06947 #if (SX1272_debug_mode > 1) 06948 printf("## Sync word is %X ",_syncWord); 06949 // Serial.print(_syncWord, HEX); 06950 printf(" ##"); 06951 printf("\n"); 06952 #endif 06953 } 06954 return state; 06955 } 06956 06957 /* 06958 Function: Sets the sync word in the module. 06959 Returns: Integer that determines if there has been any error 06960 state = 2 --> The command has not been executed 06961 state = 1 --> There has been an error while executing the command 06962 state = 0 --> The command has been executed with no errors 06963 state = -1 --> Forbidden command for this protocol 06964 Parameters: 06965 cod: sw is sync word value to set in LoRa modem configuration. 06966 */ 06967 int8_t SX1272::setSyncWord(uint8_t sw) 06968 { 06969 byte st0; 06970 int8_t state = 2; 06971 byte config1; 06972 06973 #if (SX1272_debug_mode > 1) 06974 printf("\n"); 06975 printf("Starting 'setSyncWord'\n"); 06976 #endif 06977 06978 st0 = readRegister(REG_OP_MODE); // Save the previous status 06979 06980 if( _modem == FSK ) 06981 { 06982 #if (SX1272_debug_mode > 1) 06983 printf("## Notice that FSK hasn't sync word parameter, "); 06984 printf("so you are configuring it in LoRa mode ##"); 06985 #endif 06986 state = setLORA(); 06987 } 06988 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); // Set Standby mode to write in registers 06989 06990 writeRegister(REG_SYNC_WORD, sw); 06991 06992 wait_ms(100); 06993 06994 config1 = readRegister(REG_SYNC_WORD); 06995 06996 if (config1==sw) { 06997 state=0; 06998 _syncWord = sw; 06999 #if (SX1272_debug_mode > 1) 07000 printf("## Sync Word %X ",sw); 07001 // Serial.print(sw, HEX); 07002 printf(" has been successfully set ##"); 07003 printf("\n"); 07004 #endif 07005 } 07006 else { 07007 state=1; 07008 #if (SX1272_debug_mode > 1) 07009 printf("** There has been an error while configuring Sync Word parameter **"); 07010 printf("\n"); 07011 #endif 07012 } 07013 07014 writeRegister(REG_OP_MODE,st0); // Getting back to previous status 07015 wait_ms(100); 07016 return state; 07017 } 07018 07019 07020 int8_t SX1272::setSleepMode() { 07021 07022 int8_t state = 2; 07023 byte value; 07024 07025 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); 07026 // proposed by escyes 07027 // https://github.com/CongducPham/LowCostLoRaGw/issues/53#issuecomment-289237532 07028 // 07029 // inserted to avoid REG_OP_MODE stay = 0x40 (no sleep mode) 07030 wait_ms(100); 07031 writeRegister(REG_OP_MODE, LORA_SLEEP_MODE); // LoRa sleep mode 07032 07033 //delay(50); 07034 07035 value = readRegister(REG_OP_MODE); 07036 07037 //printf("## REG_OP_MODE 0x"); 07038 //Serial.println(value, HEX); 07039 07040 if (value == LORA_SLEEP_MODE) 07041 state=0; 07042 else 07043 state=1; 07044 07045 return state; 07046 } 07047 07048 int8_t SX1272::setPowerDBM(uint8_t dbm) { 07049 byte st0; 07050 int8_t state = 2; 07051 byte value = 0x00; 07052 07053 byte RegPaDacReg=(_board==SX1272Chip)?0x5A:0x4D; 07054 07055 #if (SX1272_debug_mode > 1) 07056 printf("\n"); 07057 printf("Starting 'setPowerDBM'\n"); 07058 #endif 07059 07060 st0 = readRegister(REG_OP_MODE); // Save the previous status 07061 if( _modem == LORA ) 07062 { // LoRa Stdby mode to write in registers 07063 writeRegister(REG_OP_MODE, LORA_STANDBY_MODE); 07064 } 07065 else 07066 { // FSK Stdby mode to write in registers 07067 writeRegister(REG_OP_MODE, FSK_STANDBY_MODE); 07068 } 07069 07070 if (dbm == 20) { 07071 return setPower('X'); 07072 } 07073 07074 if (dbm > 14) 07075 return state; 07076 07077 // disable high power output in all other cases 07078 writeRegister(RegPaDacReg, 0x84); 07079 07080 if (dbm > 10) 07081 // set RegOcp for OcpOn and OcpTrim 07082 // 130mA 07083 setMaxCurrent(0x10); 07084 else 07085 // 100mA 07086 setMaxCurrent(0x0B); 07087 07088 if (_board==SX1272Chip) { 07089 // Pout = -1 + _power[3:0] on RFO 07090 // Pout = 2 + _power[3:0] on PA_BOOST 07091 if (_needPABOOST) { 07092 value = dbm - 2; 07093 // we set the PA_BOOST pin 07094 value = value & 0B10000000; 07095 } 07096 else 07097 value = dbm + 1; 07098 07099 writeRegister(REG_PA_CONFIG, value); // Setting output power value 07100 } 07101 else { 07102 // for the SX1276 07103 uint8_t pmax=15; 07104 07105 // then Pout = Pmax-(15-_power[3:0]) if PaSelect=0 (RFO pin for +14dBm) 07106 // so L=3dBm; H=7dBm; M=15dBm (but should be limited to 14dBm by RFO pin) 07107 07108 // and Pout = 17-(15-_power[3:0]) if PaSelect=1 (PA_BOOST pin for +14dBm) 07109 // so x= 14dBm (PA); 07110 // when p=='X' for 20dBm, value is 0x0F and RegPaDacReg=0x87 so 20dBm is enabled 07111 07112 if (_needPABOOST) { 07113 value = dbm - 17 + 15; 07114 // we set the PA_BOOST pin 07115 value = value & 0B10000000; 07116 } 07117 else 07118 value = dbm - pmax + 15; 07119 07120 // set MaxPower to 7 -> Pmax=10.8+0.6*MaxPower [dBm] = 15 07121 value = value & 0B01110000; 07122 07123 writeRegister(REG_PA_CONFIG, value); 07124 } 07125 07126 _power=value; 07127 07128 value = readRegister(REG_PA_CONFIG); 07129 07130 if( value == _power ) 07131 { 07132 state = 0; 07133 #if (SX1272_debug_mode > 1) 07134 printf("## Output power has been successfully set ##"); 07135 printf("\n"); 07136 #endif 07137 } 07138 else 07139 { 07140 state = 1; 07141 } 07142 07143 writeRegister(REG_OP_MODE, st0); // Getting back to previous status 07144 wait_ms(100); 07145 return state; 07146 } 07147 07148 long SX1272::limitToA() { 07149 07150 // first time we set limitToA? 07151 // in this design, once you set _limitToA to true 07152 // it is not possible to set it back to false 07153 if (_limitToA==false) { 07154 _startToAcycle=millis(); 07155 _remainingToA=MAX_DUTY_CYCLE_PER_HOUR; 07156 // we are handling millis() rollover by calculating the end of cycle time 07157 _endToAcycle=_startToAcycle+DUTYCYCLE_DURATION; 07158 } 07159 07160 _limitToA=true; 07161 return getRemainingToA(); 07162 } 07163 07164 long SX1272::getRemainingToA() { 07165 07166 if (_limitToA==false) 07167 return MAX_DUTY_CYCLE_PER_HOUR; 07168 07169 // we compare to the end of cycle so that millis() rollover is taken into account 07170 // using unsigned long modulo operation 07171 if ( (millis() > _endToAcycle ) ) { 07172 _startToAcycle=_endToAcycle; 07173 _remainingToA=MAX_DUTY_CYCLE_PER_HOUR; 07174 _endToAcycle=_startToAcycle+DUTYCYCLE_DURATION; 07175 07176 printf("## new cycle for ToA ##"); 07177 printf("cycle begins at %d\n",_startToAcycle); 07178 // Serial.print(_startToAcycle); 07179 printf(" cycle ends at %d\n",_endToAcycle); 07180 // Serial.print(_endToAcycle); 07181 printf(" remaining ToA is %d\n",_remainingToA); 07182 // Serial.print(_remainingToA); 07183 printf("\n"); 07184 } 07185 07186 return _remainingToA; 07187 } 07188 07189 long SX1272::removeToA(uint16_t toa) { 07190 07191 // first, update _remainingToA 07192 getRemainingToA(); 07193 07194 if (_limitToA) { 07195 _remainingToA-=toa; 07196 } 07197 07198 return _remainingToA; 07199 } 07200 07201 SX1272 sx1272 = SX1272();
Generated on Fri Jul 15 2022 12:44:35 by
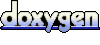