A driver for the MAX8U GPS by uBlox. Provides core functionality. Communicates through I2C.
Embed:
(wiki syntax)
Show/hide line numbers
Example.cpp
00001 //Example Usage of MAX8U Driver 00002 00003 00004 #include "MAX8U.h" 00005 00006 int main(){ 00007 MAX8U gps(&pc, PIN_I2C_SDA, PIN_I2C_SCL, p25); 00008 bool booted = gps.begin(); 00009 00010 if(!booted){ 00011 //handle error 00012 } 00013 booted = gps.configure(); 00014 if(!booted){ 00015 //handle error 00016 } 00017 00018 while(true){ 00019 bool newMessageRcvd = gps.update(); 00020 pc.printf(">Position: %.06f %c, %.06f %c, Height %.02f m\r\n", 00021 std::abs(gps.latitude), gps.latitude > 0 ? 'N' : 'S', 00022 std::abs(gps.longitude), gps.longitude > 0 ? 'E' : 'W', 00023 gps.height); 00024 00025 // velocity 00026 pc.printf(">Velocity: %.02f m/s N, %.02f m/s E, %.02f m/s Down\r\n", 00027 gps.northVel * .02f, 00028 gps.eastVel * .02f, 00029 gps.downVel * .02f); 00030 00031 // accuracy 00032 pc.printf(">Fix: Quality: %" PRIu8 ", Num Satellites: %" PRIu8 ", Position Accuracy: %.02f m\r\n", 00033 static_cast<uint8_t>(gps.fixQuality), gps.numSatellites, gps.posAccuracy); 00034 00035 // time 00036 pc.printf(">Time: %" PRIu8 "/%" PRIu8"/%" PRIu16" %" PRIu8":%" PRIu8 ":%" PRIu8 "\r\n", 00037 gps.month, 00038 gps.day, 00039 gps.year, 00040 gps.hour, 00041 gps.minute, 00042 gps.second); 00043 } 00044 }
Generated on Thu Jul 21 2022 02:08:23 by
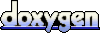