
手柄测试
Fork of nRF24L01P_Hello_World by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "nRF24L01P.h" 00003 00004 Serial pc(USBTX, USBRX, 115200); // tx, rx 00005 00006 // mosi, miso, sck, csn, ce, irq 00007 nRF24L01P my_nrf24l01p(D4, D5, D3, D7, D8, D6); 00008 00009 // The nRF24L01+ supports transfers from 1 to 32 bytes 00010 #define TRANSFER_SIZE 1 00011 00012 void initNrf24L01() 00013 { 00014 my_nrf24l01p.powerUp(); 00015 00016 // Display the (default) setup of the nRF24L01+ chip 00017 pc.printf( "nRF24L01+ Frequency : %d MHz\r\n", my_nrf24l01p.getRfFrequency() ); 00018 pc.printf( "nRF24L01+ Output power : %d dBm\r\n", my_nrf24l01p.getRfOutputPower() ); 00019 pc.printf( "nRF24L01+ Data Rate : %d kbps\r\n", my_nrf24l01p.getAirDataRate() ); 00020 pc.printf( "nRF24L01+ TX Address : 0x%010llX\r\n", my_nrf24l01p.getTxAddress() ); 00021 pc.printf( "nRF24L01+ RX Address : 0x%010llX\r\n", my_nrf24l01p.getRxAddress() ); 00022 00023 pc.printf( "Type keys to test transfers:\r\n (transfers are grouped into %d characters)\r\n", TRANSFER_SIZE ); 00024 00025 my_nrf24l01p.setTransferSize( TRANSFER_SIZE ); 00026 00027 my_nrf24l01p.setReceiveMode(); 00028 my_nrf24l01p.enable(); 00029 } 00030 int main() { 00031 char data[TRANSFER_SIZE]; 00032 initNrf24L01(); 00033 while (1) { 00034 // If we've received anything in the nRF24L01+... 00035 if ( my_nrf24l01p.readable() ) { 00036 // ...read the data into the receive buffer 00037 my_nrf24l01p.read( NRF24L01P_PIPE_P0, data, TRANSFER_SIZE); 00038 pc.putc(data[0]); 00039 } 00040 if ( pc.readable() ) { 00041 data[0] = pc.getc(); 00042 // Send the transmitbuffer via the nRF24L01+ 00043 my_nrf24l01p.write( NRF24L01P_PIPE_P0, data, TRANSFER_SIZE ); 00044 } 00045 } 00046 }
Generated on Tue Jul 19 2022 08:16:27 by
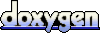