
ECE 2036 Project
Dependencies: mbed wave_player 4DGL-uLCD-SE
wall.h
00001 #pragma once 00002 00003 // Forward declarations 00004 struct Physics; 00005 struct Entity; 00006 00007 /** 00008 * Structure to represent a Wall in the arena. 00009 * 00010 * This structure is a ArenaElement; its first data element is an integer (type) 00011 * whose value is always WALL. 00012 */ 00013 struct Wall { 00014 int type; 00015 int direction; 00016 int x, y; 00017 int length; 00018 float bounce; 00019 int should_draw; 00020 }; 00021 00022 // Directions 00023 #define HORIZONTAL 0 00024 #define VERTICAL 1 00025 00026 /** 00027 * Allocate and initialize a new wall object. 00028 * 00029 * @param[in] direction The direction of the wall (HORIZONTAL or VERTICAL) 00030 * @param[in] x The x location of the origin of the wall 00031 * @param[in] y The y location of the origin of the wall 00032 * @param[in] length The length of the wall. (HORIZONTAL = right, VERTICAL = down) 00033 * @param[in] bounce The coefficient of bounciness for the wall. Between 0 and 1. 00034 * @return The newly initialized wall. 00035 */ 00036 Wall* create_wall(int direction, int x, int y, int length, float bounce); 00037 00038 /** 00039 * Computes the physics update for a wall. 00040 * 00041 * @param[out] phys The result physics update. Assumed valid at function start. 00042 * @param[in] ball The ball to update 00043 * @param[in] wall The wall to bounce off of 00044 * @param[in] delta The elapsed time, in seconds, since the previous update 00045 */ 00046 void do_wall(Physics* next, const Physics* curr, Wall* wall, float delta); 00047 00048 /** 00049 * Draws a given wall to the screen. 00050 * 00051 * @param[in] wall The wall to draw 00052 */ 00053 void draw_wall(Wall* wall);
Generated on Fri Jul 15 2022 16:19:36 by
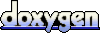