
Node code for sx1272 LoRa transciever
Dependencies: mbed BMP085 BufferedSerial DHT Sds021 Chainable_RGB_LED DigitDisplay LoRaWAN-lib SX1272Lib
Fork of LoRaWAN-demo-72-bootcamp by
SerialDisplay.cpp
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2015 Semtech 00008 00009 Description: VT100 serial display management 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #include "board.h" 00016 #include "vt100.h" 00017 #include "SerialDisplay.h" 00018 00019 //VT100 vt( USBTX, USBRX ); 00020 VT100 vt( PC_12, PD_2 ); 00021 00022 00023 void SerialPrintCheckBox( bool activated, uint8_t color ) 00024 { 00025 if( activated == true ) 00026 { 00027 vt.SetAttribute( VT100::ATTR_OFF, color, color ); 00028 } 00029 else 00030 { 00031 vt.SetAttribute( VT100::ATTR_OFF ); 00032 } 00033 vt.printf( " " ); 00034 vt.SetAttribute( VT100::ATTR_OFF ); 00035 } 00036 00037 void SerialDisplayUpdateActivationMode( bool otaa ) 00038 { 00039 vt.SetCursorPos( 4, 17 ); 00040 SerialPrintCheckBox( otaa, VT100::WHITE ); 00041 vt.SetCursorPos( 9, 17 ); 00042 SerialPrintCheckBox( !otaa, VT100::WHITE ); 00043 } 00044 00045 void SerialDisplayUpdateEui( uint8_t line, uint8_t *eui ) 00046 { 00047 vt.SetCursorPos( line, 27 ); 00048 for( uint8_t i = 0; i < 8; i++ ) 00049 { 00050 vt.printf( "%02X ", eui[i] ); 00051 } 00052 vt.SetCursorPos( line, 50 ); 00053 vt.printf( "]" ); 00054 } 00055 00056 void SerialDisplayUpdateKey( uint8_t line, uint8_t *key ) 00057 { 00058 vt.SetCursorPos( line, 27 ); 00059 for( uint8_t i = 0; i < 16; i++ ) 00060 { 00061 vt.printf( "%02X ", key[i] ); 00062 } 00063 vt.SetCursorPos( line, 74 ); 00064 vt.printf( "]" ); 00065 } 00066 00067 void SerialDisplayUpdateNwkId( uint8_t id ) 00068 { 00069 vt.SetCursorPos( 10, 27 ); 00070 vt.printf( "%03d", id ); 00071 } 00072 00073 void SerialDisplayUpdateDevAddr( uint32_t addr ) 00074 { 00075 vt.SetCursorPos( 11, 27 ); 00076 vt.printf( "%02X %02X %02X %02X", ( addr >> 24 ) & 0xFF, ( addr >> 16 ) & 0xFF, ( addr >> 8 ) & 0xFF, addr & 0xFF ); 00077 } 00078 00079 void SerialDisplayUpdateFrameType( bool confirmed ) 00080 { 00081 vt.SetCursorPos( 15, 17 ); 00082 SerialPrintCheckBox( confirmed, VT100::WHITE ); 00083 vt.SetCursorPos( 15, 32 ); 00084 SerialPrintCheckBox( !confirmed, VT100::WHITE ); 00085 } 00086 00087 void SerialDisplayUpdateAdr( bool adr ) 00088 { 00089 vt.SetCursorPos( 16, 27 ); 00090 if( adr == true ) 00091 { 00092 vt.printf( " ON" ); 00093 } 00094 else 00095 { 00096 vt.printf( "OFF" ); 00097 } 00098 } 00099 00100 void SerialDisplayUpdateDutyCycle( bool dutyCycle ) 00101 { 00102 vt.SetCursorPos( 17, 27 ); 00103 if( dutyCycle == true ) 00104 { 00105 vt.printf( " ON" ); 00106 } 00107 else 00108 { 00109 vt.printf( "OFF" ); 00110 } 00111 } 00112 00113 void SerialDisplayUpdatePublicNetwork( bool network ) 00114 { 00115 vt.SetCursorPos( 19, 17 ); 00116 SerialPrintCheckBox( network, VT100::WHITE ); 00117 vt.SetCursorPos( 19, 30 ); 00118 SerialPrintCheckBox( !network, VT100::WHITE ); 00119 } 00120 00121 void SerialDisplayUpdateNetworkIsJoined( bool state ) 00122 { 00123 vt.SetCursorPos( 20, 17 ); 00124 SerialPrintCheckBox( !state, VT100::RED ); 00125 vt.SetCursorPos( 20, 30 ); 00126 SerialPrintCheckBox( state, VT100::GREEN ); 00127 } 00128 00129 void SerialDisplayUpdateLedState( uint8_t id, uint8_t state ) 00130 { 00131 switch( id ) 00132 { 00133 case 1: 00134 vt.SetCursorPos( 22, 17 ); 00135 SerialPrintCheckBox( state, VT100::RED ); 00136 break; 00137 case 2: 00138 vt.SetCursorPos( 22, 31 ); 00139 SerialPrintCheckBox( state, VT100::GREEN ); 00140 break; 00141 case 3: 00142 vt.SetCursorPos( 22, 45 ); 00143 SerialPrintCheckBox( state, VT100::BLUE ); 00144 break; 00145 } 00146 } 00147 00148 void SerialDisplayUpdateData( uint8_t line, uint8_t *buffer, uint8_t size ) 00149 { 00150 if( size != 0 ) 00151 { 00152 vt.SetCursorPos( line, 27 ); 00153 for( uint8_t i = 0; i < size; i++ ) 00154 { 00155 vt.printf( "%02X ", buffer[i] ); 00156 if( ( ( i + 1 ) % 16 ) == 0 ) 00157 { 00158 line++; 00159 vt.SetCursorPos( line, 27 ); 00160 } 00161 } 00162 for( uint8_t i = size; i < 64; i++ ) 00163 { 00164 vt.printf( "__ " ); 00165 if( ( ( i + 1 ) % 16 ) == 0 ) 00166 { 00167 line++; 00168 vt.SetCursorPos( line, 27 ); 00169 } 00170 } 00171 vt.SetCursorPos( line - 1, 74 ); 00172 vt.printf( "]" ); 00173 } 00174 else 00175 { 00176 vt.SetCursorPos( line, 27 ); 00177 for( uint8_t i = 0; i < 64; i++ ) 00178 { 00179 vt.printf( "__ " ); 00180 if( ( ( i + 1 ) % 16 ) == 0 ) 00181 { 00182 line++; 00183 vt.SetCursorPos( line, 27 ); 00184 } 00185 } 00186 vt.SetCursorPos( line - 1, 74 ); 00187 vt.printf( "]" ); 00188 } 00189 } 00190 00191 void SerialDisplayUpdateUplinkAcked( bool state ) 00192 { 00193 vt.SetCursorPos( 24, 36 ); 00194 SerialPrintCheckBox( state, VT100::GREEN ); 00195 } 00196 00197 void SerialDisplayUpdateUplink( bool acked, uint8_t datarate, uint16_t counter, uint8_t port, uint8_t *buffer, uint8_t bufferSize ) 00198 { 00199 // Acked 00200 SerialDisplayUpdateUplinkAcked( acked ); 00201 // Datarate 00202 vt.SetCursorPos( 25, 33 ); 00203 vt.printf( "DR%d", datarate ); 00204 // Counter 00205 vt.SetCursorPos( 26, 27 ); 00206 vt.printf( "%10d", counter ); 00207 // Port 00208 vt.SetCursorPos( 27, 34 ); 00209 vt.printf( "%3d", port ); 00210 // Data 00211 SerialDisplayUpdateData( 28, buffer, bufferSize ); 00212 // Help message 00213 vt.SetCursorPos( 42, 1 ); 00214 vt.printf( "To refresh screen please hit 'r' key." ); 00215 } 00216 00217 void SerialDisplayUpdateDonwlinkRxData( bool state ) 00218 { 00219 vt.SetCursorPos( 34, 4 ); 00220 SerialPrintCheckBox( state, VT100::GREEN ); 00221 } 00222 00223 void SerialDisplayUpdateDownlink( bool rxData, int16_t rssi, int8_t snr, uint16_t counter, uint8_t port, uint8_t *buffer, uint8_t bufferSize ) 00224 { 00225 // Rx data 00226 SerialDisplayUpdateDonwlinkRxData( rxData ); 00227 // RSSI 00228 vt.SetCursorPos( 33, 32 ); 00229 vt.printf( "%5d", rssi ); 00230 // SNR 00231 vt.SetCursorPos( 34, 32 ); 00232 vt.printf( "%5d", snr ); 00233 // Counter 00234 vt.SetCursorPos( 35, 27 ); 00235 vt.printf( "%10d", counter ); 00236 if( rxData == true ) 00237 { 00238 // Port 00239 vt.SetCursorPos( 36, 34 ); 00240 vt.printf( "%3d", port ); 00241 // Data 00242 SerialDisplayUpdateData( 37, buffer, bufferSize ); 00243 } 00244 else 00245 { 00246 // Port 00247 vt.SetCursorPos( 36, 34 ); 00248 vt.printf( " " ); 00249 // Data 00250 SerialDisplayUpdateData( 37, NULL, 0 ); 00251 } 00252 } 00253 00254 void SerialDisplayDrawFirstLine( void ) 00255 { 00256 vt.PutBoxDrawingChar( 'l' ); 00257 for( int8_t i = 0; i <= 77; i++ ) 00258 { 00259 vt.PutBoxDrawingChar( 'q' ); 00260 } 00261 vt.PutBoxDrawingChar( 'k' ); 00262 vt.printf( "\r\n" ); 00263 } 00264 00265 void SerialDisplayDrawTitle( const char* title ) 00266 { 00267 vt.PutBoxDrawingChar( 'x' ); 00268 vt.printf( "%s", title ); 00269 vt.PutBoxDrawingChar( 'x' ); 00270 vt.printf( "\r\n" ); 00271 } 00272 void SerialDisplayDrawTopSeparator( void ) 00273 { 00274 vt.PutBoxDrawingChar( 't' ); 00275 for( int8_t i = 0; i <= 11; i++ ) 00276 { 00277 vt.PutBoxDrawingChar( 'q' ); 00278 } 00279 vt.PutBoxDrawingChar( 'w' ); 00280 for( int8_t i = 0; i <= 64; i++ ) 00281 { 00282 vt.PutBoxDrawingChar( 'q' ); 00283 } 00284 vt.PutBoxDrawingChar( 'u' ); 00285 vt.printf( "\r\n" ); 00286 } 00287 00288 void SerialDisplayDrawColSeparator( void ) 00289 { 00290 vt.PutBoxDrawingChar( 'x' ); 00291 for( int8_t i = 0; i <= 11; i++ ) 00292 { 00293 vt.PutBoxDrawingChar( ' ' ); 00294 } 00295 vt.PutBoxDrawingChar( 't' ); 00296 for( int8_t i = 0; i <= 64; i++ ) 00297 { 00298 vt.PutBoxDrawingChar( 'q' ); 00299 } 00300 vt.PutBoxDrawingChar( 'u' ); 00301 vt.printf( "\r\n" ); 00302 } 00303 00304 void SerialDisplayDrawSeparator( void ) 00305 { 00306 vt.PutBoxDrawingChar( 't' ); 00307 for( int8_t i = 0; i <= 11; i++ ) 00308 { 00309 vt.PutBoxDrawingChar( 'q' ); 00310 } 00311 vt.PutBoxDrawingChar( 'n' ); 00312 for( int8_t i = 0; i <= 64; i++ ) 00313 { 00314 vt.PutBoxDrawingChar( 'q' ); 00315 } 00316 vt.PutBoxDrawingChar( 'u' ); 00317 vt.printf( "\r\n" ); 00318 } 00319 00320 void SerialDisplayDrawLine( const char* firstCol, const char* secondCol ) 00321 { 00322 vt.PutBoxDrawingChar( 'x' ); 00323 vt.printf( "%s", firstCol ); 00324 vt.PutBoxDrawingChar( 'x' ); 00325 vt.printf( "%s", secondCol ); 00326 vt.PutBoxDrawingChar( 'x' ); 00327 vt.printf( "\r\n" ); 00328 } 00329 00330 void SerialDisplayDrawBottomLine( void ) 00331 { 00332 vt.PutBoxDrawingChar( 'm' ); 00333 for( int8_t i = 0; i <= 11; i++ ) 00334 { 00335 vt.PutBoxDrawingChar( 'q' ); 00336 } 00337 vt.PutBoxDrawingChar( 'v' ); 00338 for( int8_t i = 0; i <= 64; i++ ) 00339 { 00340 vt.PutBoxDrawingChar( 'q' ); 00341 } 00342 vt.PutBoxDrawingChar( 'j' ); 00343 vt.printf( "\r\n" ); 00344 } 00345 00346 void SerialDisplayInit( void ) 00347 { 00348 vt.ClearScreen( 2 ); 00349 vt.SetCursorMode( false ); 00350 vt.SetCursorPos( 0, 0 ); 00351 00352 // "+-----------------------------------------------------------------------------+" ); 00353 SerialDisplayDrawFirstLine( ); 00354 // "¦ LoRaWAN Demonstration Application ¦" ); 00355 SerialDisplayDrawTitle( " LoRaWAN Demonstration Application " ); 00356 // "+------------+----------------------------------------------------------------¦" ); 00357 SerialDisplayDrawTopSeparator( ); 00358 // "¦ Activation ¦ [ ]Over The Air ¦" ); 00359 SerialDisplayDrawLine( " Activation ", " [ ]Over The Air " ); 00360 // "¦ ¦ DevEui [__ __ __ __ __ __ __ __] ¦" ); 00361 SerialDisplayDrawLine( " ", " DevEui [__ __ __ __ __ __ __ __] " ); 00362 // "¦ ¦ AppEui [__ __ __ __ __ __ __ __] ¦" ); 00363 SerialDisplayDrawLine( " ", " AppEui [__ __ __ __ __ __ __ __] " ); 00364 // "¦ ¦ AppKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00365 SerialDisplayDrawLine( " ", " AppKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00366 // "¦ +----------------------------------------------------------------¦" ); 00367 SerialDisplayDrawColSeparator( ); 00368 // "¦ ¦ [x]Personalisation ¦" ); 00369 SerialDisplayDrawLine( " ", " [ ]Personalisation " ); 00370 // "¦ ¦ NwkId [___] ¦" ); 00371 SerialDisplayDrawLine( " ", " NwkId [___] " ); 00372 // "¦ ¦ DevAddr [__ __ __ __] ¦" ); 00373 SerialDisplayDrawLine( " ", " DevAddr [__ __ __ __] " ); 00374 // "¦ ¦ NwkSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00375 SerialDisplayDrawLine( " ", " NwkSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00376 // "¦ ¦ AppSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00377 SerialDisplayDrawLine( " ", " AppSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00378 // "+------------+----------------------------------------------------------------¦" ); 00379 SerialDisplayDrawSeparator( ); 00380 // "¦ MAC params ¦ [ ]Confirmed / [ ]Unconfirmed ¦" ); 00381 SerialDisplayDrawLine( " MAC params ", " [ ]Confirmed / [ ]Unconfirmed " ); 00382 // "¦ ¦ ADR [ ] ¦" ); 00383 SerialDisplayDrawLine( " ", " ADR [ ] " ); 00384 // "¦ ¦ Duty cycle[ ] ¦" ); 00385 SerialDisplayDrawLine( " ", " Duty cycle[ ] " ); 00386 // "+------------+----------------------------------------------------------------¦" ); 00387 SerialDisplayDrawSeparator( ); 00388 // "¦ Network ¦ [ ]Public / [ ]Private ¦" ); 00389 SerialDisplayDrawLine( " Network ", " [ ]Public / [ ]Private " ); 00390 // "¦ ¦ [ ]Joining / [ ]Joined ¦" ); 00391 SerialDisplayDrawLine( " ", " [ ]Joining / [ ]Joined " ); 00392 // "+------------+----------------------------------------------------------------¦" ); 00393 SerialDisplayDrawSeparator( ); 00394 // "¦ LED status ¦ [ ]LED1(Tx) / [ ]LED2(Rx) / [ ]LED3(App) ¦" ); 00395 SerialDisplayDrawLine( " LED status ", " [ ]LED1(Tx) / [ ]LED2(Rx) / [ ]LED3(App) " ); 00396 // "+------------+----------------------------------------------------------------¦" ); 00397 SerialDisplayDrawSeparator( ); 00398 // "¦ Uplink ¦ Acked [ ] ¦" ); 00399 SerialDisplayDrawLine( " Uplink ", " Acked [ ] " ); 00400 // "¦ ¦ Datarate [ ] ¦" ); 00401 SerialDisplayDrawLine( " ", " Datarate [ ] " ); 00402 // "¦ ¦ Counter [ ] ¦" ); 00403 SerialDisplayDrawLine( " ", " Counter [ ] " ); 00404 // "¦ ¦ Port [ ] ¦" ); 00405 SerialDisplayDrawLine( " ", " Port [ ] " ); 00406 // "¦ ¦ Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00407 SerialDisplayDrawLine( " ", " Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00408 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00409 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00410 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00411 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00412 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00413 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00414 // "+------------+----------------------------------------------------------------¦" ); 00415 SerialDisplayDrawSeparator( ); 00416 // "¦ Downlink ¦ RSSI [ ] dBm ¦" ); 00417 SerialDisplayDrawLine( " Downlink ", " RSSI [ ] dBm " ); 00418 // "¦ [ ]Data ¦ SNR [ ] dB ¦" ); 00419 SerialDisplayDrawLine( " [ ]Data ", " SNR [ ] dB " ); 00420 // "¦ ¦ Counter [ ] ¦" ); 00421 // "¦ ¦ Counter [ ] ¦" ); 00422 SerialDisplayDrawLine( " ", " Counter [ ] " ); 00423 // "¦ ¦ Port [ ] ¦" ); 00424 SerialDisplayDrawLine( " ", " Port [ ] " ); 00425 // "¦ ¦ Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00426 SerialDisplayDrawLine( " ", " Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00427 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00428 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00429 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00430 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00431 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00432 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00433 // "+------------+----------------------------------------------------------------+" ); 00434 SerialDisplayDrawBottomLine( ); 00435 vt.printf( "To refresh screen please hit 'r' key.\r\n" ); 00436 } 00437 00438 bool SerialDisplayReadable( void ) 00439 { 00440 return vt.Readable( ); 00441 } 00442 00443 uint8_t SerialDisplayGetChar( void ) 00444 { 00445 return vt.GetChar( ); 00446 }
Generated on Tue Jul 12 2022 21:57:19 by
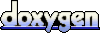