CMPS03 digital compass library.
Dependents: CMPS03_HelloWorld Final_Sonar xbeetx Compass ... more
CMPS03.h
00001 /** 00002 * @author Aaron Berk 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * CMPS03 digital compass module. 00029 * 00030 * Datasheet: 00031 * 00032 * http://www.robot-electronics.co.uk/htm/cmps3tech.htm 00033 */ 00034 00035 #ifndef CMPS03_H 00036 #define CMPS03_H 00037 00038 /** 00039 * Includes 00040 */ 00041 #include "mbed.h" 00042 00043 /** 00044 * Defines 00045 */ 00046 #define CMPS03_DEFAULT_I2C_ADDRESS 0xC0 00047 00048 //----------- 00049 // Registers 00050 //----------- 00051 #define SOFTWARE_REVISION_REG 0x0 00052 #define COMPASS_BEARING_WORD_REG 0x2 00053 00054 /** 00055 * CMPS03 digital compass module. 00056 */ 00057 class CMPS03 { 00058 00059 I2C* i2c; 00060 int i2cAddress; 00061 00062 public: 00063 00064 /** 00065 * Constructor. 00066 * 00067 * @param sda mbed pin to use for I2C SDA 00068 * @param scl mbed pin to use for I2C SCL 00069 * @param address I2C address of this device. 00070 */ 00071 CMPS03(PinName sda, PinName scl, int address); 00072 00073 /** 00074 * Reads the software revision register [register 0] on the device. 00075 * 00076 * @return The contents of the software revision register as a byte. 00077 */ 00078 char readSoftwareRevision(void); 00079 00080 /** 00081 * Reads the current bearing of the compass. 00082 * 00083 * @return The current bearing of the compass as a value between 0 and 3599, 00084 * representing 0 - 359.9 degrees. 00085 */ 00086 int readBearing(void); 00087 00088 }; 00089 00090 #endif /* CMPS03_H */
Generated on Tue Jul 12 2022 11:37:22 by
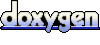