
内蔵USBメモリにキーデータとタイミングデータを書いたテキストを置いておくと1/60秒ごとに再生するプログラムです。詳しくはここ http://mbed.org/users/abanum/notebook/%E8%87%AA%E5%8B%95%E5%85%A5%E5%8A%9B%E8%A3%85%E7%BD%AE/
main.cpp
00001 #include "mbed.h" 00002 // button config for PSP 00003 BusOut Bout(p15, //X batu 00004 p16, // shikaku 00005 p17, // maru 00006 p18, // sankaku 00007 p19, // sita 00008 p20, // ue 00009 p21, // migi 00010 p22, // hidari 00011 p23, //L left 00012 p24 //R right 00013 ); 00014 00015 static unsigned int now_frame = 0; 00016 static unsigned int Bdata_n = 0; 00017 static unsigned int mode = 0; 00018 00019 struct { 00020 unsigned int frame; 00021 int key; 00022 } Bdata[2800]; 00023 00024 //syuuhen settei 00025 InterruptIn event(p8); 00026 DigitalIn start(p8); 00027 DigitalOut led1(LED1); 00028 DigitalOut led2(LED2); 00029 Serial pc(USBTX, USBRX); // tx, rx 00030 00031 00032 Ticker flipper; 00033 00034 void int_frame() { 00035 led2 = !led2; 00036 if (now_frame == Bdata[Bdata_n].frame ){ 00037 Bout = Bdata[Bdata_n].key; 00038 Bdata_n ++; 00039 if (Bdata[Bdata_n].frame == 0){ 00040 flipper.detach(); //frame == 0 is end 00041 wait(0.1); 00042 now_frame = 0; 00043 Bdata_n = 0; 00044 Bout = 0; 00045 mode = 0; 00046 } 00047 } 00048 now_frame ++; 00049 //pc.printf("now_frame=%d Bdata_n=%d\r\n",now_frame,Bdata_n); 00050 00051 } 00052 00053 void trigger() { 00054 wait(0.1); 00055 if (!start)return; //key filter 00056 00057 switch(mode){ 00058 case 0: 00059 flipper.attach(&int_frame, 1.0f/59.94f); // the address of the function to be attached (flip) and the interval (2 seconds) 00060 mode = 1; 00061 break; 00062 case 1: 00063 flipper.detach(); //frame == 0 is end 00064 wait(0.1); 00065 now_frame = 0; 00066 Bdata_n = 0; 00067 Bout = 0; 00068 mode = 0; 00069 break; 00070 } 00071 // pc.printf("triggered!\r\n"); 00072 } 00073 00074 int main() { 00075 event.mode (PullUp); //hard de pullup sinaito matomoni ugokanai 00076 event.rise(&trigger); 00077 00078 00079 // Bdata[0].key = 0; Bdata[0].frame = 1; 00080 // Bdata[1].key = 1; Bdata[1].frame = 2; 00081 /// Bdata[2].key = 0; Bdata[2].frame = 3; 00082 // Bdata[3].key = 2; Bdata[3].frame = 4; 00083 // Bdata[4].key = 4; Bdata[4].frame = 5; 00084 // Bdata[5].key = 0; Bdata[5].frame = 6; 00085 // Bdata[6].key = 0; Bdata[6].frame = 7; 00086 // Bdata[7].key = 8; Bdata[7].frame = 8; 00087 // Bdata[8].key = 0; Bdata[8].frame = 9; 00088 // Bdata[9].key = 16; Bdata[9].frame = 10; 00089 // Bdata[10].key = 0; Bdata[10].frame = 11; 00090 // Bdata[11].key = 32; Bdata[11].frame = 12; 00091 // Bdata[12].key = 0; Bdata[12].frame = 14; 00092 // Bdata[13].key = 0; Bdata[13].frame = 15; 00093 // Bdata[14].key = 0; Bdata[14].frame = 0; 00094 //datafile read & Analysis 00095 LocalFileSystem local( "local" ); 00096 char s[ 3 ]; 00097 char buf[ 100 ]; 00098 FILE *fp; 00099 00100 printf( "\r\nreading a data file.\r\n" ); 00101 00102 if ( NULL == (fp = fopen( "/local/data.txt", "r" )) ) { 00103 pc.printf( "\r\nError: The data file cannot be accessed\r\n" ); 00104 while(1) { 00105 led1 = !led1; 00106 wait(0.2); 00107 } 00108 // return -1; 00109 } 00110 //Analysis 00111 int onkey = 0,count = 0,file_stat,tempkey,i,k; 00112 unsigned int onframe = 0,offframe = 0,tempframe,onoffset,offoffset; 00113 Bdata[0].frame = 0; 00114 Bdata[0].key = 0; 00115 while (1) { 00116 //printf("file stat %d\n\r",file_stat); 00117 if ((int)fgets(buf, sizeof(buf), fp) == NULL)break; 00118 if ((buf[0] == '#') or (buf[0] == '/')){ 00119 printf(buf); 00120 // while(1){ 00121 // file_stat = fgetc(fp); 00122 // printf("%c",file_stat); 00123 // if (file_stat == '\n')break; 00124 // } 00125 continue; 00126 } 00127 file_stat = sscanf(buf,"%2s,%d,%d", s,&onframe,&offframe); 00128 if (file_stat != 3){ 00129 printf("para err\r\n"); 00130 while(1) { 00131 led1 = !led1; 00132 wait(0.2); 00133 } 00134 } 00135 00136 if (strcmp(s,"ba") == 0){onkey = 1;} 00137 if (strcmp(s,"si") == 0){onkey = 2;} 00138 if (strcmp(s,"ma") == 0){onkey = 4;} 00139 if (strcmp(s,"sa") == 0){onkey = 8;} 00140 if (strcmp(s,"dn") == 0){onkey = 16;} 00141 if (strcmp(s,"up") == 0){onkey = 32;} 00142 if (strcmp(s,"ri") == 0){onkey = 64;} 00143 if (strcmp(s,"le") == 0){onkey = 128;} 00144 if (strcmp(s,"lt") == 0){onkey = 256;} 00145 if (strcmp(s,"rt") == 0){onkey = 512;} 00146 00147 if (offframe == 0) offframe = onframe + 2; 00148 00149 // pc.printf("%d %s %d %x %d \r\n",file_stat, s,onframe,onkey,offframe); 00150 00151 if (count == 0){ 00152 Bdata[0].frame = onframe; 00153 Bdata[0].key = onkey; 00154 Bdata[1].frame = offframe; 00155 Bdata[1].key = 0; 00156 count = 2; 00157 } else { 00158 00159 Bdata[count].frame = onframe; 00160 Bdata[count].key = onkey; 00161 count ++; 00162 00163 Bdata[count].frame = offframe; 00164 // Bdata[count].key = offkey; 00165 Bdata[count].key = 0; 00166 count ++; 00167 //onframe irekae check 00168 for (i = count-2;i>=0;i--){ 00169 if (Bdata[i].frame < Bdata[i-1].frame){ 00170 tempframe = Bdata[i].frame; 00171 Bdata[i].frame = Bdata[i-1].frame; 00172 Bdata[i-1].frame = tempframe ; 00173 tempkey = Bdata[i].key; 00174 Bdata[i].key = Bdata[i-1].key; 00175 Bdata[i-1].key = tempkey ; 00176 printf("chg on index=%d\r\n",i); 00177 continue; 00178 } 00179 break; 00180 } 00181 onoffset = i; 00182 00183 //offframe irekae check 00184 for (i = count-1;i>=0;i--){ 00185 if (Bdata[i].frame < Bdata[i-1].frame){ 00186 tempframe = Bdata[i].frame; 00187 Bdata[i].frame = Bdata[i-1].frame; 00188 Bdata[i-1].frame = tempframe ; 00189 tempkey = Bdata[i].key; 00190 Bdata[i].key = Bdata[i-1].key; 00191 Bdata[i-1].key = tempkey ; 00192 printf("chg off index=%d\r\n",i); 00193 continue; 00194 } 00195 break; 00196 } 00197 offoffset = i; 00198 //printf("-----on %d off %d\r\n",onoffset,offoffset); 00199 // for(k=0;k<count;k++) pc.printf("%x %d \r\n\r\n",Bdata[k].key, Bdata[k].frame); 00200 00201 Bdata[onoffset].key = Bdata[onoffset-1].key; 00202 if (Bdata[onoffset].frame == Bdata[onoffset-1].frame){ 00203 for(i = onoffset;i<count;i++){ 00204 Bdata[i-1].frame = Bdata[i].frame; 00205 Bdata[i-1].key = Bdata[i].key; 00206 } 00207 --onoffset; 00208 --offoffset; 00209 --count; 00210 // for(k=0;k<count;k++) pc.printf("%x %d \r\n",Bdata[k].key, Bdata[k].frame); 00211 } 00212 //printf("onframe same %d\r\n",onoffset); 00213 //printf("-----on %d off %d c %d\r\n",onoffset,offoffset,count); 00214 Bdata[offoffset].key = Bdata[offoffset-1].key; 00215 if (Bdata[offoffset].frame == Bdata[offoffset-1].frame){ 00216 for(i = offoffset;i<count;i++){ 00217 Bdata[i-1].frame = Bdata[i].frame; 00218 Bdata[i-1].key = Bdata[i].key; 00219 printf("****\r\n"); 00220 } 00221 --offoffset; 00222 --count; 00223 // for(k=0;k<count;k++) pc.printf("%x %d \r\n",Bdata[k].key, Bdata[k].frame); 00224 printf("offframe same %d\r\n",offoffset); 00225 } 00226 for(i = count-1;i>=(long)onoffset;i--){ 00227 Bdata[i].key |= onkey; 00228 // printf("++++on c %d i %d\r\n",count,i); 00229 } 00230 00231 for(i = count-1;i>=(long)offoffset;i--){ 00232 Bdata[i].key &= ~onkey; 00233 // printf("++++off\r\n"); 00234 } 00235 00236 // for(k=0;k<count;k++) pc.printf("%x %d \r\n",Bdata[k].key, Bdata[k].frame); 00237 00238 } 00239 if (count >=2800) break; 00240 }; 00241 fclose( fp ); 00242 00243 Bdata[count].frame = 0; 00244 Bdata[count].key = 0; 00245 for(k=0;k<count+1;k++) pc.printf("%x %d \r\n",Bdata[k].key, Bdata[k].frame); 00246 00247 00248 led1 = 1; 00249 00250 00251 while(1) { 00252 } 00253 }
Generated on Sat Aug 6 2022 10:08:17 by
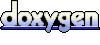