This matrix will be use to control the position and direction of a mobile robot in a 2D enviroment. See header file for more info
Dependents: Kinematics Matrix_class
TrackVector2D Class Reference
This class keeps track of a "vector" on a 2D plane. More...
#include <TrackVector2D.h>
Public Member Functions | |
TrackVector2D () | |
default Constructor. | |
TrackVector2D (const TrackVector2D &orig) | |
Copies all elements. | |
const Matrix | moveto (float x, float y) |
Move the "vector" to position x, y in the plane. | |
void | rotate (float degrees) |
Changes the direction of the "vector" to point to a new direction. | |
const Matrix | GetRotM () |
Returns 3x3 Matrix Rotation. | |
const Matrix | GetTrasfM () |
Retirns 4x4 Full Transformation Matrix. | |
const Matrix | GetLastMove () |
Returns 1x2 Matrix last [ X ][ Y ] coordenates. | |
const Matrix | GetMove (int index) |
Retruns nx2 Matrix with all n-Moves. | |
float | getX () |
Cuerrent X position of Robot. | |
float | getY () |
Current Y position of Robot. | |
float | getRad () |
Current direction with respect to "X" axis. | |
float | getDeg () |
Current direction with respect to "X" axis. | |
Static Public Member Functions | |
static float | rad2deg (float angle) |
Transform from RADIANS to DEGREES. | |
static float | deg2rad (float angle) |
Transform from DEGREES to RADIANS. | |
static const Matrix | Rect2Polar (const Matrix &Coord) |
Transforms from Rectangular to Polar coordenates. | |
static const Matrix | Polar2Rect (const Matrix &Coord) |
Transforms from Polar to Rectangular coordenates. |
Detailed Description
This class keeps track of a "vector" on a 2D plane.
Vector is defined as any point on an XY plane, with a determine direction. Could be a mobile robot for instance. Distance is unit-less depends on the use. Angles are internally measured in radiands but the API uses degrees.
Static Functions are provided for Angle and Coordenates transformations.
Definition at line 29 of file TrackVector2D.h.
Constructor & Destructor Documentation
TrackVector2D | ( | ) |
default Constructor.
Initializes Matrices to default Values
Definition at line 20 of file TrackVector2D.cpp.
TrackVector2D | ( | const TrackVector2D & | orig ) |
Copies all elements.
Copy Constructor.
- Parameters:
-
orig Original "Vector"
Definition at line 31 of file TrackVector2D.cpp.
Member Function Documentation
float deg2rad | ( | float | angle ) | [static] |
Transform from DEGREES to RADIANS.
- Parameters:
-
angle in degrees
- Returns:
- angle in radians
Definition at line 156 of file TrackVector2D.cpp.
float getDeg | ( | ) |
Current direction with respect to "X" axis.
- Returns:
- Angle in Degrees
Definition at line 142 of file TrackVector2D.cpp.
const Matrix GetLastMove | ( | ) |
Returns 1x2 Matrix last [ X ][ Y ] coordenates.
- Returns:
- 1x2 with last only Cordenates.
Definition at line 114 of file TrackVector2D.cpp.
const Matrix GetMove | ( | int | index ) |
Retruns nx2 Matrix with all n-Moves.
- Returns:
- Large nx2 Matrix with all moves done by "vector"
Definition at line 122 of file TrackVector2D.cpp.
float getRad | ( | ) |
Current direction with respect to "X" axis.
- Returns:
- Angle in radians
Definition at line 144 of file TrackVector2D.cpp.
const Matrix GetRotM | ( | ) |
Returns 3x3 Matrix Rotation.
- Returns:
- 3x3 Matrix Rotation only
Definition at line 108 of file TrackVector2D.cpp.
const Matrix GetTrasfM | ( | ) |
Retirns 4x4 Full Transformation Matrix.
- Returns:
- 4x4 Full Transfrmation Matrix.
Definition at line 111 of file TrackVector2D.cpp.
float getX | ( | ) |
Cuerrent X position of Robot.
- Returns:
- X position in Coordenate Sistem.
Definition at line 132 of file TrackVector2D.cpp.
float getY | ( | ) |
Current Y position of Robot.
- Returns:
- Y position in Coordenate Sistem
Definition at line 137 of file TrackVector2D.cpp.
const Matrix moveto | ( | float | x, |
float | y | ||
) |
Move the "vector" to position x, y in the plane.
Calculates the angle the vector should rotate, and the distance it should travel. Distance depeneds on metric units of coordenates.
- Parameters:
-
x Coordenate in "X" axis. y Coordenate in "Y" axis.
- Returns:
- 1x2 Matrix, [1][1] Distance to travel -no units. [1][2] Rotation Angle in degrees
Definition at line 46 of file TrackVector2D.cpp.
const Matrix Polar2Rect | ( | const Matrix & | Coord ) | [static] |
Transforms from Polar to Rectangular coordenates.
- Parameters:
-
1x2 Matrix [Magnitude][Direction] in Degrees!
- Returns:
- 1x2 Matrix [x][y]
Definition at line 205 of file TrackVector2D.cpp.
float rad2deg | ( | float | angle ) | [static] |
Transform from RADIANS to DEGREES.
- Parameters:
-
angle in radians
- Returns:
- angle in degrees
Definition at line 147 of file TrackVector2D.cpp.
const Matrix Rect2Polar | ( | const Matrix & | Coord ) | [static] |
Transforms from Rectangular to Polar coordenates.
- Parameters:
-
1x2 Matrix [x][y]
- Returns:
- 1x2 Matrix [Magnitude][Direction] in Degrees!
Definition at line 165 of file TrackVector2D.cpp.
void rotate | ( | float | degrees ) |
Changes the direction of the "vector" to point to a new direction.
Keeps track of ratation. Doesn't return anything because does not travels any distance, and rotation is already known.
- Parameters:
-
degrees Angle in degrees to Rotate
Definition at line 102 of file TrackVector2D.cpp.
Generated on Fri Jul 15 2022 05:44:54 by
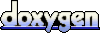