
This little program demonstrates how to use the SignalGenerator library.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "SignalGenerator.h" // It includes 'mbed.h' header 00002 00003 /* CPU Available indicator 00004 */ 00005 DigitalOut g_availableLed(LED1); //<! Led used to indicate the program is alive 00006 void AvailableLedIndicator(); //<! Ticker callback 00007 Ticker g_available; 00008 00009 /* SignalGenerator usage 00010 */ 00011 SignalGenerator g_signal(p18); 00012 void SynchronousUserInput(); //<! Ticker callback 00013 Ticker g_synchronousUserInput; 00014 Serial g_debug(USBTX, USBRX); //<! Needed to detect that the user pressed a key 00015 SignalGenerator::SignalGeneratorType DisplaySignalGeneratorTestMenuAndGetChoice(); //<! User menu to select a signal type 00016 00017 /* Program Entry Point 00018 */ 00019 int main() { 00020 volatile SignalGenerator::SignalGeneratorType _choice; //<! User selects the desired signal type 00021 volatile int frequency; //<! Signal frequency 00022 00023 wait(1); // Needed after startup 00024 00025 // Launch available indicator 00026 g_availableLed = 1; 00027 g_available.attach(&AvailableLedIndicator, 2.0); // Never detached 00028 00029 // Start infinite loop 00030 while(true) 00031 { 00032 // Acquire settings 00033 _choice = DisplaySignalGeneratorTestMenuAndGetChoice(); 00034 printf("\r\nEnter signal frequency (< 1MHz/# of samples): "); 00035 scanf("%d", &frequency); 00036 00037 // Prepare the signal 00038 g_signal.SetSignalFrequency(_choice, frequency); 00039 00040 // Launch execution 00041 if (g_signal.BeginRunAsync() == -1) { 00042 // Synchronous mode 00043 g_synchronousUserInput.attach(&SynchronousUserInput, 0.005); // 5ms 00044 g_signal.Run(); // Never stopped 00045 printf("\r\nSignal Generator terminated\r\n"); 00046 g_synchronousUserInput.detach(); 00047 } else { 00048 // Asynchronous mode 00049 printf("\r\n\r\nPress any key to terminate\r\n"); 00050 while (getchar() != 'q'); 00051 g_signal.EndRunAsync(); 00052 } 00053 } // End of 'while' statement 00054 } // End of main program 00055 00056 /** Callbak used by CPU availabe ticker to indicate the program is alive 00057 */ 00058 void AvailableLedIndicator() { 00059 g_availableLed != g_availableLed; 00060 } // End of function AvailableLedIndicator 00061 00062 /** Callbak used when SignalLibrary is used in synchronous mode (Run() method) 00063 */ 00064 void SynchronousUserInput() { 00065 if (g_debug.readable()) { 00066 g_signal.Stop(); 00067 getchar(); 00068 } 00069 } // End of function SynchronousUserInput 00070 00071 /** Display the user menu 00072 */ 00073 SignalGenerator::SignalGeneratorType DisplaySignalGeneratorTestMenuAndGetChoice() 00074 { 00075 do { 00076 printf("\r\n\r\nTest demo v0.1 - Generate signal\r\n"); 00077 printf("\tSquareSignal:\t0\r\n"); 00078 printf("\tTriangleSignal:\t1\r\n"); 00079 printf("\tSawtoothSignal:\t2\r\n"); 00080 printf("\tReverse sawtoothSignal:\t3\r\n"); 00081 printf("\tSinusSignal:\t4\r\n"); 00082 switch (getchar()) { 00083 case '0' : 00084 return SignalGenerator::SquareSignal; 00085 case '1' : 00086 return SignalGenerator::TriangleSignal; 00087 case '2' : 00088 return SignalGenerator::SawtoothSignal; 00089 case '3' : 00090 return SignalGenerator::ReverseSawtoothSignal; 00091 case '4' : 00092 return SignalGenerator::SinusSignal; 00093 } // End of 'switch' statement 00094 } while (true); 00095 } // End of function DisplaySignalGeneratorTestMenuAndGetChoice
Generated on Sun Jul 17 2022 07:45:23 by
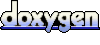