
Programme utilisé lors du premier laboratoire 247-436
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 //options de compilation 00003 //#define TEST 00004 00005 00006 #define TFT_SPDF5408 00007 00008 //intégration 00009 // représentation 00010 // G: gauche, D: droit, H: haut, B: bas 00011 // E: écran, T: touch 00012 // Ecran: 00013 // (XE_G,YE_H) ___ (XE_D,YE_H) 00014 // | | 00015 // | | 00016 // (XE_G,YE_B) |___| (XE_G,YE_B) 00017 // 00018 // représentation du touch 00019 // (XT_G,YT_H) ___ (XT_D,YT_H) 00020 // | | 00021 // | | 00022 // (XT_G,YT_B) |___| (XT_G,YT_B) 00023 00024 // équations pour conversion 00025 // XT: x touch lu, YT: y touch lu, XE: x ecran, YE: y ecran 00026 // XE = (XE_D - XE_G)/(XT_D - XT_G)*(XT - XT_G) + XE_G 00027 // YE = (YE_H - YE_B)/(YT_H - YT_B)*(YT - YT_G) + YE_G 00028 00029 // l'application présente 3 boutons et une surface de dessin 00030 // bouton noir: effacer 00031 // bouton rouge: dessiner en rouge 00032 // bouton blanc: dessiner en blanc 00033 00034 //touchscreen 00035 #define IDLE 1 00036 #define ACTIVE 0 00037 #define COMMAND 0 00038 #define DATA 1 00039 00040 #ifdef TFT_SPDF5408 00041 #define YP A3 00042 #define XM A2 00043 #define YM D9 00044 #define XP D8 00045 #endif 00046 00047 #ifdef MON_TFT 00048 #define YP A1 // must be an analog pin, use "An" notation! 00049 #define YM D7 // can be a digital pin 00050 #define XP D6 // can be a digital pin 00051 #define XM A2 // must be an analog pin, use "An" notation! 00052 #endif 00053 00054 #define TOUCH_SEUIL_HAUT 3000 00055 #define TOUCH_SEUIL_BAS 16 00056 00057 00058 //tftspfd5408 00059 #define TFTWIDTH 240 00060 #define TFTHEIGHT 320 00061 00062 #define LCD_CS A3 00063 #define LCD_CD A2 00064 #define LCD_WR A1 00065 #define LCD_RD A0 00066 #define LCD_RESET A4 00067 00068 #define TFT_NORTH SPFD5408_MADCTL_MY | SPFD5408_MADCTL_BGR 00069 00070 #if 0 00071 #define TFT_EAST {SPFD5408_MADCTL_MX | SPFD5408_MADCTL_MY | SPFD5408_MADCTL_MV | SPFD5408_MADCTL_BGR} 00072 #define TFT_SOUTH {SPFD5408_MADCTL_MX | SPFD5408_MADCTL_BGR} 00073 #define TFT_WEST {SPFD5408_MADCTL_MV | SPFD5408_MADCTL_BGR} 00074 #endif 00075 00076 #define BLACK 0x0000 00077 #define BLUE 0x001F 00078 #define RED 0xF800 00079 #define GREEN 0x07E0 00080 #define CYAN 0x07FF 00081 #define MAGENTA 0xF81F 00082 #define YELLOW 0xFFE0 00083 #define WHITE 0xFFFF 00084 00085 #define SPFD5408_SOFTRESET 0x01 00086 #define SPFD5408_SLEEPOUT 0x11 00087 00088 #if 0 00089 #define SPFD5408_SLEEPIN 0x10 00090 #define SPFD5408_NORMALDISP 0x13 00091 #define SPFD5408_INVERTOFF 0x20 00092 #define SPFD5408_INVERTON 0x21 00093 #define SPFD5408_GAMMASET 0x26 00094 #define SPFD5408_DISPLAYOFF 0x28 00095 #endif 00096 00097 #define SPFD5408_DISPLAYON 0x29 00098 #define SPFD5408_COLADDRSET 0x2A 00099 #define SPFD5408_PAGEADDRSET 0x2B 00100 #define SPFD5408_MEMORYWRITE 0x2C 00101 #define SPFD5408_PIXELFORMAT 0x3A 00102 #define SPFD5408_FRAMECONTROL 0xB1 00103 #if 0 00104 #define SPFD5408_DISPLAYFUNC 0xB6 00105 #define SPFD5408_ENTRYMODE 0xB7 00106 #define SPFD5408_POWERCONTROL1 0xC0 00107 #define SPFD5408_POWERCONTROL2 0xC1 00108 #define SPFD5408_VCOMCONTROL1 0xC5 00109 #define SPFD5408_VCOMCONTROL2 0xC7 00110 00111 #define SPFD5408_MADCTL 0x36 00112 #define SPFD5408_MADCTL_MX 0x40 00113 #define SPFD5408_MADCTL_MV 0x20 00114 #define SPFD5408_MADCTL_ML 0x10 00115 #define SPFD5408_MADCTL_RGB 0x00 00116 #endif 00117 #define SPFD5408_MEMCONTROL 0x36 00118 #define SPFD5408_MADCTL_MY 0x80 00119 #define SPFD5408_MADCTL_BGR 0x08 00120 #if 0 00121 #define SPFD5408_MADCTL_MH 0x04 00122 #endif 00123 00124 #define IDLE 1 00125 #define ACTIVE 0 00126 #define COMMAND 0 00127 #define DATA 1 00128 00129 #define TEMPS 40 00130 00131 00132 //integration 00133 00134 #define XE_G 0 00135 #define XE_D 240 00136 #define YE_H 0 00137 #define YE_B 320 00138 #define XT_G 3700 00139 #define XT_D 600 00140 #define YT_H 600 00141 #define YT_B 3700 00142 00143 //definitions de variables 00144 //touchscreen 00145 uint16_t x; 00146 uint16_t y; 00147 uint16_t z; 00148 00149 //tftspfd5408 00150 DigitalOut pinRD(LCD_RD); //PA_0; 00151 DigitalOut pinWR(LCD_WR); //PA_1; 00152 DigitalOut pinCD(LCD_CD); //PA_4; 00153 DigitalOut pinCS(LCD_CS); //PB_0; 00154 DigitalOut pinReset(LCD_RESET); //PC_1; 00155 00156 //integration 00157 Serial pc(SERIAL_TX, SERIAL_RX, 9600); 00158 DigitalOut myled(LED1); 00159 uint16_t couleurVariable; 00160 uint16_t tableDeCouleur[]= 00161 { 00162 WHITE, YELLOW, GREEN, BLUE, CYAN, MAGENTA, RED 00163 }; 00164 00165 00166 //declarations de fonctions 00167 //touchscreen 00168 void restoreXY(void); 00169 uint16_t readTouchX(void); 00170 uint16_t readTouchY(void); 00171 uint16_t detectTouch(void); 00172 00173 //tftspfd5408 00174 void WriteCommand(uint8_t c); 00175 void WriteData(uint8_t d); 00176 void begin(void); 00177 void setAddrWindow(int x1, int y1, int x2, int y2); 00178 void fillRect(uint16_t x1, uint16_t y1, uint16_t w, uint16_t h, uint16_t fillcolor); 00179 00180 //definitions de fonctions 00181 //touchscreen 00182 void restoreXY(void) 00183 { 00184 DigitalOut pinXP(XP); 00185 DigitalOut pinXM(XM); 00186 DigitalOut pinYP(YP); 00187 DigitalOut pinYM(YM); 00188 00189 pinXP = 1; 00190 pinXM = 1; 00191 pinYP = 1; 00192 pinYM = 1; 00193 wait_ms(1); 00194 } 00195 00196 uint16_t readTouchX(void) 00197 { 00198 uint16_t value; 00199 DigitalOut pinXP(XP); 00200 DigitalOut pinXM(XM); 00201 AnalogIn pinYP(YP); 00202 DigitalIn pinYM(YM); 00203 00204 pinXP = 0; 00205 pinXM = 1; 00206 pinYM.mode(OpenDrain); 00207 value = pinYP.read_u16() >> 4; 00208 restoreXY(); 00209 return value; 00210 } 00211 00212 uint16_t readTouchY(void) 00213 { 00214 uint16_t value; 00215 DigitalIn pinXP(XP); 00216 AnalogIn pinXM(XM); 00217 DigitalOut pinYP(YP); 00218 DigitalOut pinYM(YM); 00219 00220 pinYP = 1; 00221 pinYM = 0; 00222 pinXP.mode(OpenDrain); 00223 value = pinXM.read_u16() >> 4; 00224 restoreXY(); 00225 return value; 00226 } 00227 00228 uint16_t detectTouch(void) 00229 { 00230 uint16_t firstValue; 00231 uint16_t secondValue; 00232 DigitalOut pinXP(XP); 00233 DigitalOut pinXM(XM); 00234 AnalogIn pinYP(YP); 00235 DigitalIn pinYM(YM); 00236 00237 pinYM.mode(OpenDrain); 00238 pinXP = 1; 00239 pinXM = 0; 00240 firstValue = pinYP.read_u16() >> 4; 00241 00242 pinXP = 0; 00243 pinXM = 1; 00244 secondValue = pinYP.read_u16() >> 4; 00245 00246 restoreXY(); 00247 00248 if (secondValue > firstValue) 00249 { 00250 return firstValue; 00251 } 00252 return secondValue; 00253 } 00254 00255 //tftspfd5408 00256 void WriteCommand(uint8_t c) 00257 { 00258 BusInOut portTFT(D8, D9, D2, D3, D4, D5, D6, D7); 00259 portTFT.output(); 00260 pinCD = COMMAND; 00261 pinWR = ACTIVE; 00262 portTFT = c; 00263 pinWR = IDLE; 00264 } 00265 00266 void WriteData(uint8_t d) 00267 { 00268 BusInOut portTFT(D8, D9, D2, D3, D4, D5, D6, D7); 00269 portTFT.output(); 00270 pinCD = DATA; 00271 pinWR = ACTIVE; 00272 portTFT = d; 00273 pinWR = IDLE; 00274 } 00275 00276 void begin(void) 00277 { 00278 pinCS = IDLE; 00279 pinCD = DATA; 00280 pinWR = IDLE; 00281 pinRD = IDLE; 00282 00283 pinReset = ACTIVE; 00284 wait_ms(100); 00285 pinReset = IDLE; 00286 wait_ms(100); 00287 pinCS = ACTIVE; 00288 00289 WriteCommand(SPFD5408_SOFTRESET); 00290 WriteData(0); 00291 wait_ms(50); 00292 00293 WriteCommand(SPFD5408_MEMCONTROL); 00294 WriteData(TFT_NORTH); //(SPFD5408_MADCTL_MY | SPFD5408_MADCTL_BGR); 00295 00296 WriteCommand(SPFD5408_PIXELFORMAT); 00297 WriteData(0x55); 00298 00299 WriteCommand(SPFD5408_FRAMECONTROL); 00300 WriteData(0x00); 00301 WriteData(0x1B); 00302 00303 WriteCommand(SPFD5408_SLEEPOUT); 00304 WriteData(0); 00305 00306 WriteCommand(SPFD5408_DISPLAYON); 00307 WriteData(0); 00308 } 00309 00310 void setAddrWindow(int x1, int y1, int x2, int y2) { 00311 pinCS = ACTIVE; 00312 wait_us(TEMPS); 00313 WriteCommand(SPFD5408_COLADDRSET); 00314 WriteData(x1 >> 8); 00315 WriteData(x1); 00316 WriteData(x2 >> 8); 00317 WriteData(x2); 00318 wait_us(TEMPS); 00319 pinCS = IDLE; 00320 00321 pinCS = ACTIVE; 00322 wait_us(TEMPS); 00323 WriteCommand(SPFD5408_PAGEADDRSET); 00324 WriteData(y1 >> 8); 00325 WriteData(y1); 00326 WriteData(y2 >> 8); 00327 WriteData(y2); 00328 pinCS = IDLE; 00329 } 00330 00331 void fillRect(uint16_t x1, uint16_t y1, uint16_t w, uint16_t h, uint16_t fillcolor) 00332 { 00333 BusInOut portTFT(D8, D9, D2, D3, D4, D5, D6, D7); 00334 uint8_t hi, lo; 00335 uint16_t x2, y2; 00336 uint16_t i, j; 00337 00338 portTFT.output(); 00339 00340 x2 = x1 + w - 1; 00341 y2 = y1 + h - 1; 00342 setAddrWindow(x1, y1, x2, y2); 00343 00344 hi = fillcolor >> 8; 00345 lo = fillcolor; 00346 00347 pinCS = ACTIVE; 00348 00349 WriteCommand(SPFD5408_MEMORYWRITE); 00350 pinCD = DATA; 00351 for (i = h; i > 0; i--) 00352 { 00353 for (j = w; j > 0; j--) 00354 00355 { 00356 pinWR = ACTIVE; 00357 portTFT = hi; 00358 pinWR = IDLE; 00359 pinWR = ACTIVE; 00360 portTFT = lo; 00361 pinWR = IDLE; 00362 } 00363 } 00364 pinCS = IDLE; 00365 } 00366 00367 //intégration 00368 00369 int32_t conversion(int32_t x, int32_t x0, int32_t y0, int32_t x1, int32_t y1) 00370 { 00371 int32_t retour; 00372 int32_t limiteInferieure; 00373 int32_t limiteSuperieure; 00374 if (y0 < y1) 00375 { 00376 limiteInferieure = y0; 00377 limiteSuperieure = y1; 00378 } 00379 else 00380 { 00381 limiteInferieure = y1; 00382 limiteSuperieure = y0; 00383 } 00384 retour = (x - x0) * (y1 -y0) / (x1 -x0) + y0; 00385 00386 if (retour < limiteInferieure) 00387 { 00388 return limiteInferieure; 00389 } 00390 if (retour > limiteSuperieure) 00391 { 00392 return limiteSuperieure; 00393 } 00394 return retour; 00395 }; 00396 00397 uint16_t determineLaCouleur(void) 00398 { 00399 return tableDeCouleur[3]; 00400 } 00401 00402 int main() 00403 { 00404 uint16_t couleur; 00405 //initialisation du touchscreen 00406 restoreXY(); 00407 00408 //initialisation du tftspfd5408 00409 begin(); 00410 00411 //intégration 00412 couleur = RED; 00413 couleurVariable = BLUE; 00414 fillRect(0, 0, 240, 280, BLACK); //surface de dessin 00415 //bouton noir 00416 fillRect(0, 280, 80, 2, WHITE); // ligne horizontale haut 00417 fillRect(0, 282, 2, 38, WHITE); // ligne verticale gauche 00418 fillRect(79, 282, 1, 38, WHITE); // ligne verticale droite 00419 fillRect(2, 318, 77, 2, WHITE); //ligne horizontale bas 00420 fillRect(2, 282, 77, 36, BLACK); // centre noir 00421 //bouton rouge 00422 fillRect(80, 280, 80, 40, WHITE); // moins d'instructions mais plus long 00423 fillRect(81, 282, 78, 36, RED); // bouton rouge 00424 //bouton cyan 00425 fillRect(160, 280, 80, 40, WHITE); // moins d'instructions mais plus long 00426 fillRect(161, 282, 77, 36, BLUE); //bouton bleu 00427 //indicateur 00428 fillRect(81, 282, 10, 10, GREEN); //couleur rouge par défaut 00429 00430 while(1) { 00431 00432 //tâches du touchscreen 00433 myled = !myled; 00434 00435 x = readTouchX(); 00436 y = readTouchY(); 00437 z = detectTouch(); 00438 //tâches du tftspfd5408 00439 00440 00441 //tâches d'intégration 00442 couleurVariable = determineLaCouleur(); 00443 fillRect(228, 282, 10, 10, couleurVariable); //indicateur de couleur 00444 00445 if ((z > TOUCH_SEUIL_BAS)&&(z < TOUCH_SEUIL_HAUT)) 00446 { 00447 #ifdef TEST 00448 pc.printf("x lu %u\t ", x); 00449 pc.printf("y lu %u\t ", y); 00450 pc.printf("z lu %u\t ", z); 00451 #endif 00452 float u = conversion((float)x, XT_G, XE_G, XT_D, XE_D); 00453 float v = conversion((float)y, YT_B, YE_B, YT_H, YE_H); 00454 00455 #ifdef TEST 00456 pc.printf("x ecran %f\t ", u); 00457 pc.printf("y ecran %f\r\n", v); 00458 #endif 00459 if (v < 280) 00460 { 00461 fillRect(u, v, 1, 1, couleur); 00462 } 00463 else 00464 { 00465 if (u < 80) 00466 { 00467 fillRect(0, 0, 240, 280, BLACK); 00468 } 00469 else 00470 { 00471 if (u < 160) 00472 { 00473 //indicateur RED 00474 fillRect(81, 282, 10, 10, GREEN); //couleur rouge par défaut 00475 fillRect(161,282, 10, 10, couleurVariable); 00476 00477 couleur = RED; 00478 pc.printf("couleur: rouge\n\r"); 00479 } 00480 else 00481 { 00482 //indicateur AUTRE 00483 fillRect(161, 282, 77, 36, couleurVariable); 00484 fillRect(161, 282, 10, 10, GREEN); //bouton bleu pale 00485 fillRect(81, 282, 10, 10, RED); 00486 couleur = couleurVariable; 00487 pc.printf("couleur variable\n\r"); 00488 } 00489 } 00490 } 00491 } 00492 } 00493 }
Generated on Sat Jul 16 2022 22:15:48 by
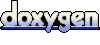