This library contains a simple device driver for the X10 CM17a module. It also contains a simple X10Server, which listens for network commands to be forwarded to the module.
X10.h
00001 00002 00003 #ifndef X10_H 00004 #define X10_H 00005 #include "mbed.h" 00006 #include "rtos.h" 00007 00008 /// X10Interface is an interface to a CM17a module. This interface 00009 /// accepts various commands and translates and forwards those 00010 /// to a connected CM17a (Firecracker) device. 00011 /// 00012 /// The system architecture for which this is relevant: 00013 /// @code 00014 /// +---------+ +---------+ +=========+ +---------+ 00015 /// | Web |- Internet-| Web |- Network -| X10 | | CM17a | 00016 /// | Browser | | Server | | | Server |-- RS232 --| Module | 00017 /// +---------+ +---------+ .../ +=========+ +---------+ 00018 /// / 00019 /// ~|+|~~~~~~~ AC Line ~~~|+|~~~~~~~~~~~~~~~~~~~|+|~~~~~~~~~~~~~~~~|+|~ /_ 00020 /// ~|+|~~~~~~~~~~~~~~~~~~~|+|~~~~~~~~~~~~~~~~~~~|+|~~~~~~~~~~~~~~~~|+|~ / 00021 /// | | | | / 00022 /// +---------+ +---------+ +---------+ +---------+ 00023 /// | X10 | | X10 | | X10 | | TM751 | 00024 /// | Module | | Module | | Module | | Module | 00025 /// +---------+ +---------+ +---------+ +---------+ 00026 /// | | | | 00027 /// +---------+ +---------+ +---------+ +---------+ 00028 /// |Appliance| |Appliance| |Appliance| |Appliance| 00029 /// | | | | | | | | 00030 /// +---------+ +---------+ +---------+ +---------+ 00031 /// @endcode 00032 /// 00033 /// Other PCs in the house also had the SW component needed to send a message to 00034 /// the X10 Server, and in the corner of one of them is a scheduled task (e.g. 00035 /// cron job) to perform some automated control. This is suggested by the '...' 00036 /// in the diagram above. 00037 /// 00038 /// The problem with this configuration is that the Web Server and the X10 Server 00039 /// were PCs; consuming PC class power. For a while, they were one in the same PC, 00040 /// but over time the network evolved and they split. The X10 Server was a bit more 00041 /// power hungry than I would like, and needs to change. This X10Interface is a key 00042 /// component in that change. 00043 /// 00044 /// The Software Architecture for the Network connected X10 Server and CM17a module: 00045 /// @code 00046 /// __ mbed module ____________________ 00047 /// / \ 00048 /// +---------+ +---------+ +---------+ +---------+ 00049 /// | Network |- Network -| Socket |-----------|X10 | | CM17a | 00050 /// | Device | | Listener| |Interface|-- DB-9 ---| Module | 00051 /// +---------+ +---------+ +---------+ +---------+ 00052 /// \__________________________________/ 00053 /// @endcode 00054 /// 00055 class X10Interface 00056 { 00057 public: 00058 typedef enum SIGNAL { 00059 RESET = 0, 00060 HIGH = 1, 00061 LOW = 2, 00062 STANDBY = 3 00063 } signal_t; 00064 00065 /// return codes used by all features 00066 typedef enum 00067 { 00068 ERR_SUCCESS, ///< Command was process successfully. 00069 ERR_BADARGS, ///< Bad arguments were supplied, could not process. 00070 ERR_NODESPEC, ///< Invalid house or unit code was supplied. 00071 ERR_COMERR, ///< Could not properly queue commands for transmit (buffer full?). 00072 ERR_BADSTEP, ///< Brighten and Dim commands only accept the range '1' to '6' 00073 ERR_BADCMD ///< Parsing could not extract the command. 00074 } ERR_EXIT; 00075 00076 /// Constructor for the X10 interface 00077 /// 00078 /// This instantiates the X10 Interface object. When a command is received 00079 /// it sends this to the connected CM17a module for transmitting. 00080 /// 00081 /// @code 00082 /// 00083 /// GND DTR TxD RxD DCD DB-9 Standard Pinout 00084 /// 5 4 3 2 1 used by CM17a module 00085 /// 9 8 7 6 00086 /// RI CTS RTS DSR 00087 /// 00088 /// DB-9 LowPower | Standby | '1' | Wait | '0' | Wait | '1' | Wait... 00089 /// ___ _______ ___________________ ________ 00090 /// DTR(4) _________| // |_____| |_____| 00091 /// ___ ____________________ _____________________ 00092 /// RTS(7) _________| // |_____| 00093 /// 00094 /// | bit period | = 1/BaudRate 00095 /// GND(5) 00096 /// @endcode 00097 /// 00098 /// @param[in] RTS is the mbed pin that the CM17a RTS pin is connected to. 00099 /// @param[in] DTR is the mbed pin that the CM17a DTR pin is connected to. 00100 /// @param[in] MultiMessageDelay_s is the delay in seconds between messages 00101 /// when more than one message is queued at once. Default is 2. 00102 /// @param[in] BaudRate is the desired baudrate for the communications 00103 /// to the CM17a module. A 'bit' in BaudRate terms is the period 00104 /// of an asserted 1 (or 0) followed by one of the 'wait' periods. 00105 /// While there is not a known reason to change it, values in the 00106 /// range 200 to 10000 have been tested successfully. 00107 /// The default value is 2000. 00108 /// 00109 X10Interface(PinName RTS, PinName DTR, uint8_t MultiMessageDelay_s = 1, uint32_t BaudRate = 10000); 00110 00111 /// Parse a command for the X10 Communications. 00112 /// 00113 /// This method accepts several different types and formats for commands. 00114 /// 00115 /// @note This method will modify the provided message as it parses it. 00116 /// 00117 /// @verbatim 00118 /// <House><Unit> <cmd> [<House><Unit> <cmd> [...]] 00119 /// House is 'a' - 'p' 00120 /// Unit is '1' - '16' 00121 /// cmd is 1=on, 0=off, +1 to +6 to brighten, -1 to -6 to dim. 00122 /// /XXXX sends the hex value XXXX to the module. 00123 /// ##### sets the baudrate to the value ##### 00124 /// @endverbatim 00125 /// 00126 /// @param[in] message is the pointer to the buffer to process. The 00127 /// buffer is modified, but there is no information sent back. 00128 /// @returns X10Interface::ERR_EXIT code. 00129 /// 00130 ERR_EXIT ParseCommand(char * message); 00131 00132 /// Set the communication baudrate to the CM17a module. 00133 /// 00134 /// @param[in] baudrate is the new baudrate. While any value is accepted, 00135 /// the module may not operate under all possible settings. 00136 /// @returns X10Interface::ERR_EXIT code. 00137 /// 00138 ERR_EXIT baud(uint32_t baudrate); 00139 00140 /// Set the delay between messages when multiple messages are processed from 00141 /// one command. 00142 /// 00143 /// @param[in] MultiMessageDelay_s is the delay in seconds that is applied 00144 /// between commands to the CM17a. If this delay is not long enough, 00145 /// commands might not be delivered to the receivers. 00146 /// @returns X10Interface::ERR_EXIT code. 00147 /// 00148 ERR_EXIT delay(uint8_t MultiMessageDelay_s); 00149 00150 private: 00151 ERR_EXIT cm17a_Header(void); 00152 ERR_EXIT enqueueData(uint16_t word); 00153 ERR_EXIT cm17a_Footer(void); 00154 ERR_EXIT DoCommand(const char* szDevice, const char* szOp); 00155 uint16_t cm17a_CmdLookup(int iHouse, int iUnit, enum command_index command); 00156 ERR_EXIT cm17a_CmdSend(int iHouse, int iUnit, enum command_index command); 00157 ERR_EXIT cm17a_CmdSend(uint16_t command); 00158 ERR_EXIT write_stream(const unsigned char* data, size_t byte_count); 00159 ERR_EXIT parse_device(const char* szDevice, int* iHouse, int* iUnit); 00160 00161 Ticker ticker; // used to manage the bitstream timing 00162 Timer timer; // used for delays between messages 00163 void x10stream(void); 00164 BusOut * cm17Pins; 00165 char commandBuffer[100]; // for parsing command streams 00166 int mIndex; 00167 00168 // This is the queue of stuff to send to the unit. It must be 00169 // able to hold multiple messages, the equivalent of: 00170 // a1 1 a2 0 a3 +1 a15 0 ... 00171 // All commands for the CM17a are held in the lower 8 bits 00172 // If the upper 8-bits is non-zero, it is a special command 00173 // (typically to introduce delays between messages). 00174 uint16_t bitBuffer[20]; 00175 int bitEnqueue; 00176 int bitDequeue; 00177 00178 // Used when processing the queue to the port pins 00179 int mask; 00180 uint32_t bitperiod_us; 00181 uint8_t multiMsgDelay_s; 00182 }; 00183 00184 00185 #endif // X10_H
Generated on Mon Jul 25 2022 09:04:25 by
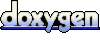