MODIFIED from mbed official WiflyInterface (interface for Roving Networks Wifly modules). Numerous performance and reliability improvements (see the detailed documentation). Also, tracking changes in mbed official version to retain functional parity.
Dependents: Smart-WiFly-WebServer PUB_WiflyInterface_Demo
Fork of WiflyInterface by
Socket.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "Socket.h" 00020 #include <cstring> 00021 00022 Socket::Socket() : _blocking(true), _timeout(1500) { 00023 wifi = Wifly::getInstance(); 00024 if (wifi == NULL) 00025 error("Socket constructor error: no wifly instance available!\r\n"); 00026 } 00027 00028 void Socket::set_blocking(bool blocking, unsigned int timeout) { 00029 _blocking = blocking; 00030 if (timeout < 1) 00031 _timeout = 1; 00032 else 00033 _timeout = timeout; 00034 } 00035 00036 int Socket::close() { 00037 return (wifi->close()) ? 0 : -1; 00038 } 00039 00040 Socket::~Socket() { 00041 close(); //Don't want to leak 00042 }
Generated on Tue Jul 12 2022 16:14:58 by
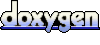