Simple library of a few functions.
Embed:
(wiki syntax)
Show/hide line numbers
Utilities.h
00001 /// Utilities.h is an adapter to support easier transition from 00002 /// the mbed cloud compiler to Visual Studio 00003 /// 00004 #ifndef UTILITIES_H 00005 #define UTILITIES_H 00006 #include <string.h> 00007 00008 // Helper functions follow. These functions may exist in some environments and 00009 // not in other combinations of libraries and compilers, so private versions 00010 // are here to ensure consistent behavior. 00011 00012 /// Access to intentionally reset the mbed 00013 /// 00014 extern "C" void mbed_reset(); 00015 00016 #if 0 00017 /// mytolower exists because not all compiler libraries have this function 00018 /// 00019 /// This takes a character and if it is upper-case, it converts it to 00020 /// lower-case and returns it. 00021 /// 00022 /// @param a is the character to convert 00023 /// @returns the lower case equivalent to a 00024 /// 00025 char mytolower(char a); 00026 00027 00028 /// mystrnicmp exists because not all compiler libraries have this function. 00029 /// 00030 /// Some have strnicmp, others _strnicmp, and others have C++ methods, which 00031 /// is outside the scope of this C-portable set of functions. 00032 /// 00033 /// @param l is a pointer to the string on the left 00034 /// @param r is a pointer to the string on the right 00035 /// @param n is the number of characters to compare 00036 /// @returns -1 if l < r 00037 /// @returns 0 if l == r 00038 /// @returns +1 if l > r 00039 /// 00040 int mystrnicmp(const char *l, const char *r, size_t n); 00041 #endif 00042 00043 /// mystrcat exists because not all compiler libraries have this function 00044 /// 00045 /// This function concatinates one string onto another. It is generally 00046 /// considered unsafe, because of the potential for buffer overflow. 00047 /// Some libraries offer a strcat_s as the safe version, and others may have 00048 /// _strcat. Because this is needed only internal to the CommandProcessor, 00049 /// this version was created. 00050 /// 00051 /// @param dst is a pointer to the destination string 00052 /// @param src is a pointer to the source string 00053 /// @returns nothing 00054 /// 00055 void mystrcat(char *dst, char *src); 00056 00057 /// myisprint exists because not all compiler libraries have this function 00058 /// 00059 /// This function tests a character to see if it is printable (a member 00060 /// of the standard ASCII set). 00061 /// 00062 /// @param c is the character to test 00063 /// @returns TRUE if the character is printable 00064 /// @returns FALSE if the character is not printable 00065 /// 00066 int myisprint(int c); 00067 00068 00069 /// hextoi converts a hex string to an unsigned integer 00070 /// 00071 /// This functions converts text to an unsigned integer, as long as 00072 /// the stream contains hex-ASCII characters 00073 /// 00074 /// @param p is a pointer to the string 00075 /// @returns unsigned long integer value 00076 /// 00077 unsigned long hextoul(char *p); 00078 00079 00080 #ifdef WIN32 00081 //####################################################################### 00082 //####################################################################### 00083 //####################################################################### 00084 // 00085 // Everything from here forward is intended to "satisfy" the Visual 00086 // Studio 2010 compiler. It isn't intended to necessarily run, since 00087 // we're faking out hardware for the most part. 00088 // 00089 00090 #include <memory.h> 00091 #include <stdio.h> 00092 #include <stdlib.h> 00093 #include <string.h> 00094 #include <math.h> 00095 #include <conio.h> 00096 00097 #define __disable_irq() 00098 #define __enable_irq() 00099 00100 enum { 00101 CANData, 00102 CANRemote 00103 }; 00104 00105 enum { 00106 CANStandard, 00107 CANExtended 00108 }; 00109 00110 enum { 00111 PullNone 00112 }; 00113 00114 typedef unsigned int uint32_t; 00115 //typedef unsigned int PinName; 00116 typedef enum { 00117 NC, 00118 USBTX, 00119 USBRX, 00120 LED1, 00121 LED2, 00122 LED3, 00123 LED4, 00124 p1, p2, p3, p4, p5, p6, p7, p8, p9, p10, 00125 p11, p12, p13, p14, p15, p16, p17, p18, p19, p20, 00126 p21, p22, p23, p24, p25, p26, p27, p28, p29, p30 00127 } PinName; 00128 00129 00130 #define SystemCoreClock 48000000 00131 00132 typedef struct { 00133 uint32_t WDMOD; 00134 uint32_t WDCLKSEL; 00135 uint32_t WDTC; 00136 uint32_t WDFEED; 00137 } LPC_WDT_BASE; 00138 00139 extern LPC_WDT_BASE * LPC_WDT; 00140 00141 typedef struct { 00142 uint32_t MOD; 00143 uint32_t GSR; 00144 uint32_t ICR; 00145 uint32_t IER; 00146 uint32_t BTR; 00147 uint32_t EWL; 00148 uint32_t SR; 00149 uint32_t RFS; 00150 uint32_t RID; 00151 uint32_t RDA; 00152 uint32_t RDB; 00153 uint32_t TFI1; 00154 uint32_t TID1; 00155 uint32_t TDA1; 00156 uint32_t TDB1; 00157 uint32_t TFI2; 00158 uint32_t TID2; 00159 uint32_t TDA2; 00160 uint32_t TDB2; 00161 uint32_t TFI3; 00162 uint32_t TID3; 00163 uint32_t TDA3; 00164 uint32_t TDB3; 00165 } LPC_CAN_BASE; 00166 00167 extern LPC_CAN_BASE * LPC_CAN1; 00168 extern LPC_CAN_BASE * LPC_CAN2; 00169 00170 00171 class CANMessage { 00172 public: 00173 CANMessage(); 00174 CANMessage(uint32_t _id, char * _pdata, int _len, int _type, int _format); 00175 ~CANMessage(); 00176 uint32_t id; 00177 int dir; 00178 char data[8]; 00179 int len; 00180 int format; 00181 int type; 00182 }; 00183 00184 class CAN { 00185 public: 00186 CAN(PinName r, PinName t); 00187 ~CAN(); 00188 int write(CANMessage msg); 00189 int read(CANMessage &msg); 00190 void attach(void(*fp)(void)); 00191 void monitor(bool t); 00192 bool frequency(uint32_t f); 00193 uint32_t tderror(); 00194 uint32_t rderror(); 00195 void reset(); 00196 }; 00197 00198 class PwmOut { 00199 public: 00200 PwmOut(PinName p); 00201 ~PwmOut(); 00202 double &PwmOut::operator=(double p); 00203 private: 00204 double p; 00205 }; 00206 00207 class Timeout { 00208 public: 00209 Timeout(); 00210 ~Timeout(); 00211 template <typename T> void attach(T * tptr, void (T::*mptr)(void), float t) { 00212 (void)tptr; 00213 (void)mptr; 00214 (void)t; 00215 } 00216 }; 00217 00218 class DigitalInOut { 00219 public: 00220 DigitalInOut(PinName p); 00221 ~DigitalInOut(); 00222 void output(); 00223 void input(); 00224 void write(bool v); 00225 void mode(int m); 00226 }; 00227 00228 class Serial { 00229 public: 00230 Serial(PinName t, PinName r); 00231 ~Serial(); 00232 int printf(char *fmt, ...); 00233 int readable(); 00234 int getc(); 00235 int putc(int c); 00236 void baud(uint32_t freq); 00237 }; 00238 00239 class Timer { 00240 public: 00241 Timer(); 00242 ~Timer(); 00243 void start(); 00244 uint32_t read_ms(); 00245 uint32_t read_us(); 00246 }; 00247 00248 void wait(float t); 00249 00250 #endif // WIN32 00251 00252 #endif // UTILITIES_H 00253 /// Utilities.h is an adapter to support easier transition from 00254 /// the mbed cloud compiler to Visual Studio 00255 /// 00256 #ifndef UTILITIES_H 00257 #define UTILITIES_H 00258 #include <string.h> 00259 00260 // Helper functions follow. These functions may exist in some environments and 00261 // not in other combinations of libraries and compilers, so private versions 00262 // are here to ensure consistent behavior. 00263 00264 /// Access to intentionally reset the mbed 00265 /// 00266 extern "C" void mbed_reset(); 00267 00268 00269 /// mytolower exists because not all compiler libraries have this function 00270 /// 00271 /// This takes a character and if it is upper-case, it converts it to 00272 /// lower-case and returns it. 00273 /// 00274 /// @param a is the character to convert 00275 /// @returns the lower case equivalent to a 00276 /// 00277 char mytolower(char a); 00278 00279 /// mystrnicmp exists because not all compiler libraries have this function. 00280 /// 00281 /// Some have strnicmp, others _strnicmp, and others have C++ methods, which 00282 /// is outside the scope of this C-portable set of functions. 00283 /// 00284 /// @param l is a pointer to the string on the left 00285 /// @param r is a pointer to the string on the right 00286 /// @param n is the number of characters to compare 00287 /// @returns -1 if l < r 00288 /// @returns 0 if l == r 00289 /// @returns +1 if l > r 00290 /// 00291 int mystrnicmp(const char *l, const char *r, size_t n); 00292 00293 /// mystrcat exists because not all compiler libraries have this function 00294 /// 00295 /// This function concatinates one string onto another. It is generally 00296 /// considered unsafe, because of the potential for buffer overflow. 00297 /// Some libraries offer a strcat_s as the safe version, and others may have 00298 /// _strcat. Because this is needed only internal to the CommandProcessor, 00299 /// this version was created. 00300 /// 00301 /// @param dst is a pointer to the destination string 00302 /// @param src is a pointer to the source string 00303 /// @returns nothing 00304 /// 00305 void mystrcat(char *dst, char *src); 00306 00307 /// myisprint exists because not all compiler libraries have this function 00308 /// 00309 /// This function tests a character to see if it is printable (a member 00310 /// of the standard ASCII set). 00311 /// 00312 /// @param c is the character to test 00313 /// @returns TRUE if the character is printable 00314 /// @returns FALSE if the character is not printable 00315 /// 00316 int myisprint(int c); 00317 00318 00319 /// hextoi converts a hex string to an unsigned integer 00320 /// 00321 /// This functions converts text to an unsigned integer, as long as 00322 /// the stream contains hex-ASCII characters 00323 /// 00324 /// @param p is a pointer to the string 00325 /// @returns unsigned long integer value 00326 /// 00327 unsigned long hextoul(char *p); 00328 00329 00330 #ifdef WIN32 00331 //####################################################################### 00332 //####################################################################### 00333 //####################################################################### 00334 // 00335 // Everything from here forward is intended to "satisfy" the Visual 00336 // Studio 2010 compiler. It isn't intended to necessarily run, since 00337 // we're faking out hardware for the most part. 00338 // 00339 00340 #include <memory.h> 00341 #include <stdio.h> 00342 #include <stdlib.h> 00343 #include <string.h> 00344 #include <math.h> 00345 #include <conio.h> 00346 00347 #define __disable_irq() 00348 #define __enable_irq() 00349 00350 enum { 00351 CANData, 00352 CANRemote 00353 }; 00354 00355 enum { 00356 CANStandard, 00357 CANExtended 00358 }; 00359 00360 enum { 00361 PullNone 00362 }; 00363 00364 typedef unsigned int uint32_t; 00365 //typedef unsigned int PinName; 00366 typedef enum { 00367 NC, 00368 USBTX, 00369 USBRX, 00370 LED1, 00371 LED2, 00372 LED3, 00373 LED4, 00374 p1, p2, p3, p4, p5, p6, p7, p8, p9, p10, 00375 p11, p12, p13, p14, p15, p16, p17, p18, p19, p20, 00376 p21, p22, p23, p24, p25, p26, p27, p28, p29, p30 00377 } PinName; 00378 00379 00380 #define SystemCoreClock 48000000 00381 00382 typedef struct { 00383 uint32_t WDMOD; 00384 uint32_t WDCLKSEL; 00385 uint32_t WDTC; 00386 uint32_t WDFEED; 00387 } LPC_WDT_BASE; 00388 00389 extern LPC_WDT_BASE * LPC_WDT; 00390 00391 typedef struct { 00392 uint32_t MOD; 00393 uint32_t GSR; 00394 uint32_t ICR; 00395 uint32_t IER; 00396 uint32_t BTR; 00397 uint32_t EWL; 00398 uint32_t SR; 00399 uint32_t RFS; 00400 uint32_t RID; 00401 uint32_t RDA; 00402 uint32_t RDB; 00403 uint32_t TFI1; 00404 uint32_t TID1; 00405 uint32_t TDA1; 00406 uint32_t TDB1; 00407 uint32_t TFI2; 00408 uint32_t TID2; 00409 uint32_t TDA2; 00410 uint32_t TDB2; 00411 uint32_t TFI3; 00412 uint32_t TID3; 00413 uint32_t TDA3; 00414 uint32_t TDB3; 00415 } LPC_CAN_BASE; 00416 00417 extern LPC_CAN_BASE * LPC_CAN1; 00418 extern LPC_CAN_BASE * LPC_CAN2; 00419 00420 00421 class CANMessage { 00422 public: 00423 CANMessage(); 00424 CANMessage(uint32_t _id, char * _pdata, int _len, int _type, int _format); 00425 ~CANMessage(); 00426 uint32_t id; 00427 int dir; 00428 char data[8]; 00429 int len; 00430 int format; 00431 int type; 00432 }; 00433 00434 class CAN { 00435 public: 00436 CAN(PinName r, PinName t); 00437 ~CAN(); 00438 int write(CANMessage msg); 00439 int read(CANMessage &msg); 00440 void attach(void(*fp)(void)); 00441 void monitor(bool t); 00442 bool frequency(uint32_t f); 00443 uint32_t tderror(); 00444 uint32_t rderror(); 00445 void reset(); 00446 }; 00447 00448 class PwmOut { 00449 public: 00450 PwmOut(PinName p); 00451 ~PwmOut(); 00452 double &PwmOut::operator=(double p); 00453 private: 00454 double p; 00455 }; 00456 00457 class Timeout { 00458 public: 00459 Timeout(); 00460 ~Timeout(); 00461 template <typename T> void attach(T * tptr, void (T::*mptr)(void), float t) { 00462 (void)tptr; 00463 (void)mptr; 00464 (void)t; 00465 } 00466 }; 00467 00468 class DigitalInOut { 00469 public: 00470 DigitalInOut(PinName p); 00471 ~DigitalInOut(); 00472 void output(); 00473 void input(); 00474 void write(bool v); 00475 void mode(int m); 00476 }; 00477 00478 class Serial { 00479 public: 00480 Serial(PinName t, PinName r); 00481 ~Serial(); 00482 int printf(char *fmt, ...); 00483 int readable(); 00484 int getc(); 00485 int putc(int c); 00486 void baud(uint32_t freq); 00487 }; 00488 00489 class Timer { 00490 public: 00491 Timer(); 00492 ~Timer(); 00493 void start(); 00494 uint32_t read_ms(); 00495 uint32_t read_us(); 00496 }; 00497 00498 void wait(float t); 00499 00500 #endif // WIN32 00501 00502 #endif // UTILITIES_H
Generated on Thu Jul 14 2022 04:09:08 by
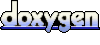