Simple library of a few functions.
Embed:
(wiki syntax)
Show/hide line numbers
Utilities.cpp
00001 /// A collection of utilities that ease the migration to/from Visual Studio and mbed 00002 /// cloud compiler. 00003 00004 #include "Utilities.h" // mbed <-> Visual Studio 00005 00006 00007 #include "string.h" 00008 00009 #ifndef TRUE 00010 #define TRUE 1 00011 #define FALSE 0 00012 #endif 00013 00014 // Helper functions follow. These functions may exist in some environments and 00015 // not in other combinations of libraries and compilers, so private versions 00016 // are here to ensure consistent behavior. 00017 00018 #if 0 00019 /// mytolower exists because not all compiler libraries have this function 00020 /// 00021 /// This takes a character and if it is upper-case, it converts it to 00022 /// lower-case and returns it. 00023 /// 00024 /// @param a is the character to convert 00025 /// @returns the lower case equivalent to a 00026 /// 00027 char mytolower(char a) { 00028 if (a >= 'A' && a <= 'Z') 00029 return (a - 'A' + 'a'); 00030 else 00031 return a; 00032 } 00033 00034 00035 /// mystrnicmp exists because not all compiler libraries have this function. 00036 /// 00037 /// Some have strnicmp, others _strnicmp, and others have C++ methods, which 00038 /// is outside the scope of this C-portable set of functions. 00039 /// 00040 /// @param l is a pointer to the string on the left 00041 /// @param r is a pointer to the string on the right 00042 /// @param n is the number of characters to compare 00043 /// @returns -1 if l < r 00044 /// @returns 0 if l == r 00045 /// @returns +1 if l > r 00046 /// 00047 int mystrnicmp(const char *l, const char *r, size_t n) { 00048 int result = 0; 00049 00050 if (n != 0) { 00051 do { 00052 result = mytolower(*l++) - mytolower(*r++); 00053 } while ((result == 0) && (*l != '\0') && (--n > 0)); 00054 } 00055 if (result < -1) 00056 result = -1; 00057 else if (result > 1) 00058 result = 1; 00059 return result; 00060 } 00061 #endif 00062 00063 /// mystrcat exists because not all compiler libraries have this function 00064 /// 00065 /// This function concatinates one string onto another. It is generally 00066 /// considered unsafe, because of the potential for buffer overflow. 00067 /// Some libraries offer a strcat_s as the safe version, and others may have 00068 /// _strcat. Because this is needed only internal to the CommandProcessor, 00069 /// this version was created. 00070 /// 00071 /// @param dst is a pointer to the destination string 00072 /// @param src is a pointer to the source string 00073 /// @returns nothing 00074 /// 00075 void mystrcat(char *dst, char *src) { 00076 while (*dst) 00077 dst++; 00078 do 00079 *dst++ = *src; 00080 while (*src++); 00081 } 00082 00083 /// myisprint exists because not all compiler libraries have this function 00084 /// 00085 /// This function tests a character to see if it is printable (a member 00086 /// of the standard ASCII set). 00087 /// 00088 /// @param c is the character to test 00089 /// @returns TRUE if the character is printable 00090 /// @returns FALSE if the character is not printable 00091 /// 00092 int myisprint(int c) { 00093 if (c >= ' ' && c <= '~') 00094 return TRUE; 00095 else 00096 return FALSE; 00097 } 00098 00099 00100 /// hextoi converts a hex string to an unsigned integer 00101 /// 00102 /// This functions converts text to an unsigned integer, as long as 00103 /// the stream contains hex-ASCII characters 00104 /// 00105 /// @param p is a pointer to the string 00106 /// @returns unsigned long integer value 00107 /// 00108 unsigned long hextoul(char *p) { 00109 unsigned long x = 0; 00110 while (*p) { 00111 x <<= 4; 00112 if (*p >= '0' && *p <= '9') 00113 x += *p - '0'; 00114 else if (*p >= 'a' && *p <= 'f') 00115 x += *p - 'a' + 10; 00116 else if (*p >= 'A' && *p <= 'F') 00117 x += *p - 'A' + 10; 00118 p++; 00119 } 00120 return x; 00121 } 00122 00123 00124 00125 #ifdef WIN32 00126 //####################################################################### 00127 //####################################################################### 00128 //####################################################################### 00129 // 00130 // Everything from here forward is intended to "satisfy" the Visual 00131 // Studio 2010 compiler. It isn't intended to necessarily run, since 00132 // we're faking out hardware for the most part. 00133 // 00134 00135 LPC_WDT_BASE * LPC_WDT; 00136 00137 LPC_CAN_BASE * LPC_CAN1; 00138 LPC_CAN_BASE * LPC_CAN2; 00139 00140 00141 void wait(float t) { 00142 (void)t; 00143 } 00144 00145 00146 CANMessage::CANMessage() { 00147 } 00148 00149 CANMessage::CANMessage(uint32_t _id, char *_pdata, int _len, int _type, int _format) { 00150 id = _id; 00151 dir = 0; 00152 len = _len; 00153 format = _format; 00154 type = _type; 00155 for (int i=0; i<len; i++) 00156 data[i] = _pdata[i]; 00157 } 00158 00159 CANMessage::~CANMessage() { 00160 } 00161 00162 00163 CAN::CAN(PinName r, PinName t) { 00164 (void)r; 00165 (void)t; 00166 } 00167 CAN::~CAN() { 00168 } 00169 int CAN::write(CANMessage msg) { 00170 (void)msg; 00171 return 1; 00172 } 00173 int CAN::read(CANMessage &msg) { 00174 (void)msg; 00175 return 1; 00176 } 00177 void CAN::attach(void(*fp)(void)) { 00178 (void)fp; 00179 } 00180 void CAN::monitor(bool t) { 00181 (void)t; 00182 } 00183 bool CAN::frequency(uint32_t f) { 00184 (void)f; 00185 return true; 00186 } 00187 uint32_t CAN::tderror() { 00188 return 0; 00189 } 00190 uint32_t CAN::rderror() { 00191 return 0; 00192 } 00193 void CAN::reset() { 00194 } 00195 00196 PwmOut::PwmOut(PinName p) { 00197 (void)p; 00198 } 00199 PwmOut::~PwmOut() { 00200 } 00201 double &PwmOut::operator=(double _p) { 00202 return p = _p; 00203 } 00204 00205 Timeout::Timeout() { 00206 } 00207 Timeout::~Timeout() { 00208 } 00209 00210 DigitalInOut::DigitalInOut(PinName p) { 00211 (void)p; 00212 } 00213 DigitalInOut::~DigitalInOut() { 00214 } 00215 void DigitalInOut::output() { 00216 } 00217 void DigitalInOut::input() { 00218 } 00219 void DigitalInOut::write(bool v) { 00220 (void)v; 00221 } 00222 void DigitalInOut::mode(int m) { 00223 (void)m; 00224 } 00225 00226 Serial::Serial(PinName t, PinName r) { 00227 (void)t; 00228 (void)r; 00229 } 00230 Serial::~Serial() { 00231 } 00232 void Serial::baud(uint32_t freq) { 00233 (void)freq; 00234 } 00235 int Serial::getc() { 00236 return _getch(); 00237 } 00238 int Serial::putc(int c) { 00239 return _putch(c); 00240 } 00241 int Serial::readable() { 00242 return _kbhit(); 00243 } 00244 int Serial::printf(char * fmt, ...) { 00245 return ::printf(fmt); 00246 } 00247 00248 Timer::Timer() { 00249 } 00250 Timer::~Timer() { 00251 } 00252 void Timer::start() { 00253 } 00254 uint32_t Timer::read_ms() { 00255 return 0; 00256 } 00257 uint32_t Timer::read_us() { 00258 return 0; 00259 } 00260 00261 00262 extern "C" { 00263 void mbed_reset() { 00264 } 00265 } 00266 00267 #endif // WIN32
Generated on Thu Jul 14 2022 04:09:08 by
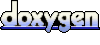