CAN Queue mechanism permitting creation and management of CAN messages through a queueing
Embed:
(wiki syntax)
Show/hide line numbers
CANQueue.h
Go to the documentation of this file.
00001 /// @file CANQueue.h 00002 /// The CAN Queue mechanism permits the creation and management of 00003 /// messages through a CAN queue. 00004 /// 00005 /// @version 1.02 00006 /// 00007 /// @note Copyright &copr; 2011 by Smartware Computing, all rights reserved. 00008 /// Individuals may use this application for evaluation or non-commercial 00009 /// purposes. Within this restriction, changes may be made to this application 00010 /// as long as this copyright notice is retained. The user shall make 00011 /// clear that their work is a derived work, and not the original. 00012 /// Users of this application and sources accept this application "as is" and 00013 /// shall hold harmless Smartware Computing, for any undesired results while 00014 /// using this application - whether real or imagined. 00015 /// 00016 /// @author David Smart, Smartware Computing 00017 /// 00018 /// v1.02 - 20111030 00019 /// \li Added QueueSize api to retrieve the defined size 00020 /// v1.01 - 29 May 2011 00021 /// \li Added QueueMax to find the highest water mark 00022 /// \li Added delete in the destructor 00023 /// \li Added [global] interrupt disable around the critical sections 00024 /// v1.00 - no date 00025 /// \li original version 00026 00027 #ifndef CANQUEUE_H 00028 #define CANQUEUE_H 00029 #include "CANMessage.h" 00030 00031 /// This is a simple queue mechanism for CANmsg messages 00032 /// 00033 /// CANQueue is a circular queue that is designed for CAN messages. 00034 /// It can be used, for example, between a CAN receive interrupt 00035 /// and a background process to offload those received messages. 00036 /// In this way, the receive interrupt is light weight. 00037 /// 00038 class CANQueue 00039 { 00040 public: 00041 /// CANQueue constructor, which sets the queue size 00042 /// 00043 /// This sets the queue size and initializes the data elements 00044 /// 00045 /// @param Size is the size in entries for this queue 00046 /// 00047 CANQueue(int Size = 3); 00048 00049 /// CANQueue destructor 00050 /// 00051 /// This destroys the queue 00052 /// 00053 ~CANQueue(); 00054 00055 /// Enqueue a new message into the queue 00056 /// 00057 /// If we enqueue until we overwrite the dequeue location, then 00058 /// we have lost the oldest message and must force the dequeue 00059 /// forward by one position. 00060 /// 00061 /// @param msg is the message to be enqueued 00062 /// @return true if no overwrite of the oldest message 00063 /// @return false if the oldest message was overwritten 00064 /// 00065 bool Enqueue(CANmsg msg); 00066 00067 /// Enqueue a new message into the queue 00068 /// 00069 /// If we enqueue until we overwrite the dequeue location, then 00070 /// we have lost the oldest message and must force the dequeue 00071 /// forward by one position. 00072 /// 00073 /// @param msg is the message to be enqueued 00074 /// @return true if no overwrite of the oldest message 00075 /// @return false if the oldest message was overwritten 00076 /// 00077 bool Enqueue(CANmsg *msg); 00078 00079 /// Dequeue a message from the queue 00080 /// 00081 /// If there is a message we'll copy it out to the callers message 00082 /// to which they provided the handle. 00083 /// 00084 /// @param msg is a pointer to the callers memory to copy the message into 00085 /// @returns true if a message was dequeued 00086 /// @returns false if the queue was empty 00087 /// 00088 bool Dequeue(CANmsg *msg); 00089 00090 /// Gets the count of the number of items in the queue 00091 /// 00092 /// @returns number of queue entries 00093 /// 00094 int QueueCount() { return queueCount; } 00095 00096 /// Get the maximum number of items that were ever in the queue 00097 /// 00098 /// @returns maximum as the high water mark 00099 /// 00100 int QueueMax() { return queueMaxCount; } 00101 00102 /// Get the defined queue size 00103 /// 00104 /// @returns size of the queue as defined 00105 /// 00106 int QueueSize() { return queueSize; } 00107 00108 private: 00109 CANmsg * queue; ///<!- the queue 00110 int queueSize; ///<!- the size of the queue in entries 00111 int queueCount; ///<!- the current number of items in the queue 00112 int queueMaxCount; ///<!- the maximum ever held in the queue 00113 int enqueuePosition; ///<!- where the next item will be enqueued 00114 int dequeuePosition; ///<!- where the next item will be dequeued 00115 }; 00116 00117 #endif // CANQUEUE_H
Generated on Wed Jul 13 2022 01:23:29 by
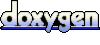