CANMessage is the primitive CAN message object. It supports creation, parsing, formatting of messages. Can be easily integrated with CANPort and CANQueue libraries.
CANMessage.h
00001 /// @file CANMessage.h 00002 /// CANMessage is the primitive CAN message object. It supports creation, 00003 /// parsing, formatting, of messages. 00004 /// 00005 /// @version 1.0 00006 /// 00007 /// @note Copyright &copr; 2011 by Smartware Computing, all rights reserved. 00008 /// Individuals may use this application for evaluation or non-commercial 00009 /// purposes. Within this restriction, changes may be made to this application 00010 /// as long as this copyright notice is retained. The user shall make 00011 /// clear that their work is a derived work, and not the original. 00012 /// Users of this application and sources accept this application "as is" and 00013 /// shall hold harmless Smartware Computing, for any undesired results while 00014 /// using this application - whether real or imagined. 00015 /// 00016 /// @author David Smart, Smartware Computing 00017 /// 00018 00019 #ifndef CANMESSAGE_H 00020 #define CANMESSAGE_H 00021 #include "mbed.h" 00022 00023 /// CAN direction, in the set {rcv, xmt} 00024 typedef enum { 00025 rcv, 00026 xmt 00027 } CANDIR_T; 00028 00029 00030 /// CAN Channel in the set {CH1, CH2} of CANCHANNELS 00031 typedef enum { 00032 CH1, 00033 CH2, 00034 CANCHANNELS 00035 } CANCHANNEL_T; 00036 00037 00038 // CAN mode - active(acknowledge) or passive(monitor) 00039 //typedef enum { 00040 // ACTIVE, 00041 // PASSIVE 00042 //} CANMODE_T; 00043 00044 00045 /// A super CAN message object, which has additional capabilities 00046 /// 00047 /// This object is derived from CANMessage, however it adds 00048 /// a timestamp and other useful methods. 00049 /// 00050 class CANmsg : public CANMessage 00051 { 00052 public: 00053 /// Constructor for a CANmsg object, which is a superclass of CANMessage 00054 /// 00055 /// The CANmsg object included additional information, including 00056 /// direction, channel number, timestamp, and the ability to format 00057 /// into ASCII 00058 /// 00059 CANmsg(); 00060 00061 /// Constructor for a CANmsg object, which is a superclass of CANMessage 00062 /// 00063 /// The CANmsg object included additional information, including 00064 /// direction, channel number, timestamp, and the ability to format 00065 /// into ASCII. 00066 /// @param ch is the channel number (CH1, CH2) 00067 /// @param dir is the direction (xmt, rcv) 00068 /// @param msg is a CANMessage object from which to construct this object 00069 /// 00070 CANmsg(CANCHANNEL_T ch, CANDIR_T dir, CANMessage msg); 00071 00072 /// Constructor for a CANmsg object, which is a superclass of CANMessage 00073 /// 00074 /// The CANmsg object included additional information, including 00075 /// direction, channel number, timestamp, and the ability to format 00076 /// into ASCII. 00077 /// This constructor creates a message from an ascii buffer with 00078 /// the standard message format in it. 00079 /// 00080 /// @todo Improve the parser, it may not handle malformed input well 00081 /// 00082 /// @verbatim 00083 /// t xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 00084 /// If the first letter is not 't' or 'r', transmit is assumed 00085 /// xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 00086 /// @endverbatim 00087 /// 00088 /// @param p is a pointer to a text string of the message contents, 00089 /// in the same format as what would be output with the 00090 /// FormatCANMessage method. 00091 /// 00092 CANmsg(char *p); 00093 00094 /// Destructor for the CANmsg object 00095 ~CANmsg(); 00096 00097 /// Parse a text string into the CANmsg object, which is a superclass of CANMessage 00098 /// 00099 /// The CANmsg object included additional information, including 00100 /// direction, channel number, timestamp, and the ability to format 00101 /// into ASCII. 00102 /// This constructor creates a message from an ascii buffer with 00103 /// the standard message format in it. 00104 /// 00105 /// @todo Improve the parser, it may not handle malformed input well 00106 /// 00107 /// @verbatim 00108 /// t xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 00109 /// If the first letter is not 't' or 'r', transmit is assumed 00110 /// xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 00111 /// @endverbatim 00112 /// 00113 /// @param p is a pointer to a text string of the message contents, 00114 /// in the same format as what would be output with the 00115 /// FormatCANMessage method. 00116 /// @returns true 00117 /// 00118 bool ParseCANMessage(char *p); 00119 00120 /// Formats this CAN message into a text buffer - which should be at least 70 00121 /// 00122 /// @todo buflen is currently ignored, which is unsafe 00123 /// 00124 /// @verbatim 00125 /// t xtd 02 1CF00400 08 11 22 33 44 55 66 77 88 0 0 1234.567890 00126 /// @endverbatim 00127 /// 00128 /// @param buffer is a pointer to the buffer to fill 00129 /// @param buflen is the size of the buffer (minimum 70 recommended) 00130 /// 00131 /// @returns nothing 00132 void FormatCANMessage(char *buffer, int buflen); 00133 00134 /// Overrides the timestamp in this message with the current time 00135 void SetTimestamp(); 00136 00137 /// Gets the timestamp of this message 00138 /// @returns time in microseconds 00139 uint64_t GetTimestamp() { return timestamp; }; 00140 00141 /// direction of this CAN message - rcv or xmt 00142 CANDIR_T dir; 00143 00144 /// channel number of this CAN message 00145 CANCHANNEL_T ch; 00146 00147 private: 00148 /// internally held timestamp 00149 uint64_t timestamp; 00150 00151 // internally held mode 00152 //CANMODE_T mode; 00153 }; 00154 00155 #endif // CANMESSAGE_H
Generated on Wed Jul 13 2022 04:27:02 by
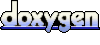