Conversions for the LCD display in FRDM-KL46Z
Dependents: eem202a_display eem202a_resolutedreamer_1
convert.h
00001 #pragma once 00002 00003 #include "SLCD.h" 00004 00005 /*------------------------------------------------------------- 00006 00007 (c) W.D. 2014 00008 00009 -------------------------------------------------------------*/ 00010 00011 /* ------ sample usage------ 00012 Convert slcd; 00013 00014 while (true) 00015 { 00016 wait(1.); 00017 slcd.display(99999); // OFL 00018 wait(2.); 00019 slcd.display(9999); // 9999 00020 wait(1.); 00021 slcd.display(-999); // -999 00022 wait(1.); 00023 slcd.display(-99999); // -OFL 00024 wait(2.); 00025 slcd.display(99999.0); // OFL 00026 wait(1.); 00027 slcd.display(1234.5); // 1000 00028 wait(1.); 00029 slcd.display(234.5); // 200.0 00030 wait(1.); 00031 slcd.display(34.56); // 30.00 00032 wait(1.); 00033 slcd.display(4.567); // 4.000 00034 wait(1.); 00035 slcd.display((float)0.56789); // 0.500 00036 wait(1.); 00037 slcd.display((double)0.0); // 0 00038 wait(1.); 00039 slcd.display((float)-0.67890); // -0.60 00040 wait(1.); 00041 slcd.display((double)-7.891); // -7.00 00042 wait(1.); 00043 slcd.display((float)-89.123); // -80.0 00044 wait(1.); 00045 slcd.display((double)-912.34); // -900 00046 wait(1.); 00047 slcd.display(-12345.0); // -OFL 00048 wait(1.); 00049 } 00050 */ 00051 00052 class Convert : public SLCD 00053 { 00054 public: 00055 Convert(); 00056 bool display(unsigned int number); 00057 bool display(int number); 00058 bool display(double number); 00059 bool display(float number); 00060 bool display(char *text); 00061 private: 00062 void display_digits(unsigned int number, bool negate=false); 00063 void prepare(void); 00064 };
Generated on Sun Aug 14 2022 16:13:48 by
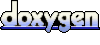