Conversions for the LCD display in FRDM-KL46Z
Dependents: eem202a_display eem202a_resolutedreamer_1
convert.cpp
00001 #include "convert.h" 00002 00003 /*------------------------------------------------------------- 00004 00005 (c) W.D. 2014 00006 00007 -------------------------------------------------------------*/ 00008 00009 Convert::Convert() 00010 { 00011 this->prepare(); 00012 00013 return; 00014 } 00015 00016 void Convert::prepare(void) 00017 { 00018 this->blink(-1); 00019 this->clear(); 00020 this->DP1(0); 00021 this->DP2(0); 00022 this->DP3(0); 00023 this->Colon(0); 00024 this->Home(); 00025 00026 return; 00027 } 00028 00029 void Convert::display_digits(unsigned int number, bool negate) 00030 { 00031 register unsigned short int c1 = ((unsigned int) number / 1000); 00032 register unsigned short int c2 = ((unsigned int) number / 100) - (c1 * 10); 00033 register unsigned short int c3 = ((unsigned int) number / 10) - ((c1 *100) + (c2 *10)); 00034 register unsigned short int c4 = number - ((c1 *1000) + (c2 *100) + (c3 *10)); 00035 00036 this->blink(-1); 00037 this->Home(); 00038 if (negate) 00039 this->putc('-'); 00040 else 00041 this->putc('0'+c1); 00042 this->putc('0'+c2); 00043 this->putc('0'+c3); 00044 this->putc('0'+c4); 00045 00046 return; 00047 } 00048 00049 bool Convert::display(unsigned int number) 00050 { 00051 this->DP1(false); 00052 this->DP2(false); 00053 this->DP3(false); 00054 this->Colon(false); 00055 00056 if (number > 9999) 00057 { 00058 this->blink(2); 00059 this->display(" OFL"); 00060 return true; 00061 } 00062 else 00063 { 00064 this->display_digits(number); 00065 return false; 00066 } 00067 } 00068 00069 bool Convert::display(int number) 00070 { 00071 this->DP1(false); 00072 this->DP2(false); 00073 this->DP3(false); 00074 this->Colon(false); 00075 00076 if (number < 0) 00077 { 00078 if (number < -999) 00079 { 00080 this->blink(3); 00081 this->display("-OFL"); 00082 return true; 00083 } 00084 else 00085 { 00086 this->display_digits ((unsigned int) -number, true); 00087 return false; 00088 } 00089 } 00090 else if (number > 9999) 00091 { 00092 this->blink(3); 00093 this->display(" OFL"); 00094 return true; 00095 } 00096 else 00097 { 00098 this->display_digits ((unsigned int) number, false); 00099 return false; 00100 } 00101 } 00102 00103 bool Convert::display(double number) 00104 { 00105 this->Colon(false); 00106 // >=10000 OVL 00107 if (number >= 10000.) 00108 { 00109 this->blink(3); 00110 this->display(" OFL"); 00111 return true; 00112 } 00113 // >=1000 1234 00114 else if (number >= 1000.) 00115 { 00116 this->DP1(false); 00117 this->DP2(false); 00118 this->DP3(false); 00119 this->display_digits ((unsigned int) number); 00120 return false; 00121 } 00122 // >=100 123.4 00123 else if (number >= 100.) 00124 { 00125 this->DP1(false); 00126 this->DP2(false); 00127 this->DP3(true); 00128 this->display_digits ((unsigned int) (number*10.)); 00129 return false; 00130 } 00131 // >=10 12.34 00132 else if (number >= 10.) 00133 { 00134 this->DP1(false); 00135 this->DP2(true); 00136 this->DP3(false); 00137 this->display_digits ((unsigned int) (number*100.)); 00138 return false; 00139 } 00140 // >=0 1.234 00141 else if (number >= 0.) 00142 { 00143 this->DP1(true); 00144 this->DP2(false); 00145 this->DP3(false); 00146 this->display_digits ((unsigned int) (number*1000.)); 00147 return false; 00148 } 00149 // <0 00150 // <=-1 -1.23 00151 else if (number >= -10.) 00152 { 00153 this->DP1(false); 00154 this->DP2(true); 00155 this->DP3(false); 00156 this->display_digits ((unsigned int) (number*-100.), true); 00157 return false; 00158 } 00159 // <=-10 -12.3 00160 else if (number >= -100.) 00161 { 00162 this->DP1(false); 00163 this->DP2(false); 00164 this->DP3(true); 00165 this->display_digits ((unsigned int) (number*-10.), true); 00166 return false; 00167 } 00168 // <=-100 -123 00169 else if (number >= -1000.) 00170 { 00171 this->DP1(false); 00172 this->DP2(false); 00173 this->DP3(false); 00174 this->display_digits ((unsigned int) (-number), true); 00175 return false; 00176 } 00177 else 00178 // <=-1000 -OVL 00179 { 00180 this->blink(3); 00181 this->display("-OFL"); 00182 return true; 00183 } 00184 00185 } 00186 00187 bool Convert::display(float number) 00188 { 00189 return this->display((double) number); 00190 } 00191 00192 bool Convert::display(char *text) 00193 { 00194 this->DP1(false); 00195 this->DP2(false); 00196 this->DP3(false); 00197 this->Colon(false); 00198 this->Home(); 00199 for(int i=0; i<4; i++) 00200 { 00201 if (text[i]==0) 00202 break; 00203 else 00204 this->putc(text[i]); 00205 } 00206 return false; 00207 }
Generated on Sun Aug 14 2022 16:13:48 by
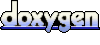