
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
Go to the documentation of this file.
00001 00002 /* 00003 Copyright 2019 (c) Analog Devices, Inc. 00004 00005 All rights reserved. 00006 00007 Redistribution and use in source and binary forms, with or without 00008 modification, are permitted provided that the following conditions are met: 00009 - Redistributions of source code must retain the above copyright 00010 notice, this list of conditions and the following disclaimer. 00011 - Redistributions in binary form must reproduce the above copyright 00012 notice, this list of conditions and the following disclaimer in 00013 the documentation and/or other materials provided with the 00014 distribution. 00015 - Neither the name of Analog Devices, Inc. nor the names of its 00016 contributors may be used to endorse or promote products derived 00017 from this software without specific prior written permission. 00018 - The use of this software may or may not infringe the patent rights 00019 of one or more patent holders. This license does not release you 00020 from the requirement that you obtain separate licenses from these 00021 patent holders to use this software. 00022 - Use of the software either in source or binary form, must be run 00023 on or directly connected to an Analog Devices Inc. component. 00024 00025 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00026 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00027 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00028 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00029 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00030 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 00036 *****************************************************************************/ 00037 /*! 00038 ****************************************************************************** 00039 * @file: 00040 *----------------------------------------------------------------------------- 00041 * 00042 */ 00043 #include "mbed.h" 00044 #include "admw_api.h" 00045 #include "admw1001/admw1001_api.h" 00046 #include "admw_log.h" 00047 #include "common/utils.h" 00048 #include "common/platform.h" 00049 #include "admw1001/ADMW1001_REGISTERS_typedefs.h" 00050 #include "admw1001/ADMW1001_REGISTERS.h" 00051 #include "admw1001/admw1001_lut_data.h" 00052 #include "admw1001/admw1001_host_comms.h" 00053 #include "mbedVersion.h" 00054 00055 extern ADMW_CONFIG thermocouple_typeK_cjc0_config; 00056 extern ADMW_CONFIG thermocouple_typeT_cjc0_config; 00057 extern ADMW_CONFIG thermocouple_typeJ_cjc0_config; 00058 extern ADMW_CONFIG rtd_3w_pt100_config; 00059 extern ADMW_CONFIG rtd_4w_config; 00060 extern ADMW_CONFIG bridge_4w_load_cell_config; 00061 extern ADMW_CONFIG i2c0_sensirionSHT3X_config; 00062 /* Use the following config if adding a configuration file from measureware designer*/ 00063 extern ADMW_CONFIG measureware_config; 00064 00065 /* Change the following pointer to load or change the configurations */ 00066 static ADMW_CONFIG *pSelectedConfig = &thermocouple_typeK_cjc0_config; 00067 00068 /* use the following to load LUT */ 00069 extern ADMW1001_LUT_DESCRIPTOR *lut_desc_list[]; 00070 extern ADMW1001_LUT_TABLE_DATA *lut_data_list[]; 00071 extern unsigned lut_num_tables; 00072 00073 static ADMW_CONNECTION connectionInfo = PLATFORM_CONNECTION_INFO; 00074 00075 00076 int main() 00077 { 00078 ADMW_RESULT res; 00079 ADMW_STATUS status; 00080 ADMW_DEVICE_HANDLE hDevice; 00081 ADMW_MEASUREMENT_MODE eMeasurementMode = ADMW_MEASUREMENT_MODE_NORMAL ; 00082 bool bDeviceReady; 00083 00084 /* 00085 * Open an ADMW1001 device instance. 00086 */ 00087 res = admw_Open(0, &connectionInfo, &hDevice); 00088 if (res != ADMW_SUCCESS ) { 00089 ADMW_LOG_ERROR("Failed to open device instance"); 00090 return res; 00091 } 00092 ADMW_LOG_INFO("Mbed Firmware version is %X.%X.%X",MBED_HOST_MAJOR,MBED_HOST_MINOR,MBED_HOST_BUILD); 00093 /* 00094 * Reset the given ADMW1001 device.... 00095 */ 00096 ADMW_LOG_INFO("Resetting ADMW1001 device, please wait..."); 00097 res = admw_Reset(hDevice); 00098 if (res != ADMW_SUCCESS ) { 00099 ADMW_LOG_ERROR("Failed to reset device"); 00100 return res; 00101 } 00102 /* 00103 * ...and wait until the device is ready. 00104 */ 00105 do { 00106 wait_ms(100); 00107 res = admw_GetDeviceReadyState(hDevice, &bDeviceReady); 00108 if (res != ADMW_SUCCESS ) { 00109 ADMW_LOG_ERROR("Failed to get device ready-state"); 00110 return res; 00111 } 00112 } while (! bDeviceReady); 00113 ADMW_LOG_INFO("ADMW1001 device ready"); 00114 /* 00115 * Write configuration settings to the device registers. 00116 * Settings are not applied until admw_ApplyConfigUpdates() is called. 00117 */ 00118 ADMW_LOG_INFO("Setting device configuration"); 00119 res = admw_SetConfig(hDevice, pSelectedConfig); 00120 if (res != ADMW_SUCCESS ) { 00121 ADMW_LOG_ERROR("Failed to set device configuration"); 00122 return res; 00123 } 00124 unsigned lutBufferSize = ADMW_LUT_MAX_SIZE; 00125 ADMW1001_LUT *pLutBuffer = (ADMW1001_LUT *) malloc(lutBufferSize); 00126 if (pLutBuffer == NULL) { 00127 return 1; 00128 } 00129 00130 res=admw1001_AssembleLutData(pLutBuffer, lutBufferSize, 00131 lut_num_tables, 00132 lut_desc_list, 00133 lut_data_list); 00134 if( res != ADMW_SUCCESS ) { 00135 ADMW_LOG_ERROR("Failed to assemble user-defined LUT data"); 00136 return res; 00137 } 00138 00139 res=admw1001_SetLutData(hDevice, 00140 pLutBuffer); 00141 if( res != ADMW_SUCCESS ) { 00142 ADMW_LOG_ERROR("Failed to set user-defined LUT data"); 00143 } 00144 00145 free( pLutBuffer ); 00146 res = admw_ApplyConfigUpdates(hDevice); 00147 if (res != ADMW_SUCCESS ) { 00148 ADMW_LOG_ERROR("Failed to apply device configuration"); 00149 return res; 00150 } 00151 /* 00152 * Check device status after updating the configuration 00153 */ 00154 res = admw_GetStatus(hDevice, &status); 00155 admw_deviceInformation(hDevice); 00156 if (res != ADMW_SUCCESS ) { 00157 ADMW_LOG_ERROR("Failed to retrieve device status"); 00158 return res; 00159 } 00160 if (status.deviceStatus & 00161 (ADMW_DEVICE_STATUS_ERROR | ADMW_DEVICE_STATUS_ALERT )) { 00162 utils_printStatus(&status); 00163 } 00164 00165 /* 00166 * Kick off the measurement cycle here 00167 */ 00168 ADMW_LOG_INFO("Configuration completed, starting measurement cycles"); 00169 utils_runMeasurement(hDevice, eMeasurementMode); 00170 00171 /* 00172 * Clean up and exit 00173 */ 00174 res = admw_Close(hDevice); 00175 if (res != ADMW_SUCCESS ) { 00176 ADMW_LOG_ERROR("Failed to close device instance"); 00177 return res; 00178 } 00179 00180 return 0; 00181 } 00182
Generated on Thu Jul 14 2022 10:33:00 by
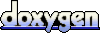