
Bumped Mbed FW version to 01.20.0080
admw_api.h File Reference
: ADMW Host Library Application Programming Interface (API) ----------------------------------------------------------------------------- More...
Go to the source code of this file.
Data Structures | |
struct | ADMW_CONNECTION |
struct | ADMW_STATUS |
struct | ADMW_DATA_SAMPLE |
Typedefs | |
typedef void * | ADMW_DEVICE_HANDLE |
Enumerations | |
enum | ADMW_CONNECTION_TYPE { ADMW_CONNECTION_TYPE_SPI = 1 } |
enum | ADMW_DEVICE_STATUS_FLAGS { ADMW_DEVICE_STATUS_BUSY = (1 << 0), ADMW_DEVICE_STATUS_DATAREADY = (1 << 1), ADMW_DEVICE_STATUS_ERROR = (1 << 2), ADMW_DEVICE_STATUS_ALERT = (1 << 3), ADMW_DEVICE_STATUS_FIFO_ERROR = (1 << 4), ADMW_DEVICE_STATUS_CONFIG_ERROR = (1 << 5), ADMW_DEVICE_STATUS_LUT_ERROR = (1 << 6) } |
enum | ADMW_CHANNEL_ALERT_FLAGS { ADMW_ALERT_DETAIL_CH_RESULT_VALID = (1 << 0), ADMW_ALERT_DETAIL_CH_ADC_NEAR_OVERRANGE = (1 << 1), ADMW_ALERT_DETAIL_CH_SENSOR_UNDERRANGE = (1 << 2), ADMW_ALERT_DETAIL_CH_SENSOR_OVERRANGE = (1 << 3), ADMW_ALERT_DETAIL_CH_CJ_SOFT_FAULT = (1 << 4), ADMW_ALERT_DETAIL_CH_CJ_HARD_FAULT = (1 << 5), ADMW_ALERT_DETAIL_CH_ADC_INPUT_OVERRANGE = (1 << 6), ADMW_ALERT_DETAIL_CH_SENSOR_HARDFAULT = (1 << 7) } |
enum | ADMW_MEASUREMENT_MODE { ADMW_MEASUREMENT_MODE_NORMAL = 0, ADMW_MEASUREMENT_MODE_OMIT_RAW } |
enum | ADMW_USER_CONFIG_SLOT |
enum | ADMW_USER_LUT_CONFIG_SLOT |
Functions | |
ADMW_RESULT | admw_Open (unsigned const nDeviceIndex, ADMW_CONNECTION *const pConnectionInfo, ADMW_DEVICE_HANDLE *const phDevice) |
Open ADMW device handle and set up communication interface. | |
ADMW_RESULT | admw_Close (ADMW_DEVICE_HANDLE const hDevice) |
Close ADMW device context and free resources. | |
ADMW_RESULT | admw_GetGpioState (ADMW_DEVICE_HANDLE const hDevice, ADMW_GPIO_PIN const ePinId, bool *const pbAsserted) |
Get the current state of the specified GPIO input signal. | |
ADMW_RESULT | admw_RegisterGpioCallback (ADMW_DEVICE_HANDLE const hDevice, ADMW_GPIO_PIN const ePinId, ADMW_GPIO_CALLBACK const callbackFunction, void *const pCallbackParam) |
Register an application-defined callback function for GPIO interrupts. | |
ADMW_RESULT | admw_Shutdown (ADMW_DEVICE_HANDLE const hDevice) |
Trigger a shut down of the device. | |
ADMW_RESULT | admw_Reset (ADMW_DEVICE_HANDLE const hDevice) |
Reset the ADMW device. | |
ADMW_RESULT | admw_GetDeviceReadyState (ADMW_DEVICE_HANDLE const hDevice, bool *const pbReady) |
Check if the device is ready, following power-up or a reset. | |
ADMW_RESULT | admw_GetProductID (ADMW_DEVICE_HANDLE const hDevice, ADMW_PRODUCT_ID *const pProductId) |
Obtain the product ID from the device. | |
ADMW_RESULT | admw_SetConfig (ADMW_DEVICE_HANDLE const hDevice, ADMW_CONFIG *const pConfig) |
Write full configuration settings to the device registers. | |
ADMW_RESULT | admw_ApplyConfigUpdates (ADMW_DEVICE_HANDLE const hDevice) |
Apply the configuration settings currently stored in device registers. | |
ADMW_RESULT | admw_SaveConfig (ADMW_DEVICE_HANDLE const hDevice, ADMW_USER_CONFIG_SLOT const eSlotId) |
Store the configuration settings to persistent memory on the device. | |
ADMW_RESULT | admw_RestoreConfig (ADMW_DEVICE_HANDLE const hDevice, ADMW_USER_CONFIG_SLOT const eSlotId) |
Restore configuration settings from persistent memory on the device. | |
ADMW_RESULT | admw_SaveLutData (ADMW_DEVICE_HANDLE const hDevice) |
Store the LUT data to persistent memory on the device. | |
ADMW_RESULT | admw_RestoreLutData (ADMW_DEVICE_HANDLE const hDevice) |
Restore LUT data from persistent memory on the device. | |
ADMW_RESULT | admw_StartMeasurement (ADMW_DEVICE_HANDLE const hDevice, ADMW_MEASUREMENT_MODE const eMeasurementMode) |
Start the measurement cycles on the device. | |
ADMW_RESULT | admw_StopMeasurement (ADMW_DEVICE_HANDLE const hDevice) |
Stop the measurement cycles on the device. | |
ADMW_RESULT | admw_RunDiagnostics (ADMW_DEVICE_HANDLE const hDevice) |
Run built-in diagnostic checks on the device. | |
ADMW_RESULT | admw_RunCalibration (ADMW_DEVICE_HANDLE const hDevice) |
Run built-in calibration on the device. | |
ADMW_RESULT | admw_RunDigitalCalibration (ADMW_DEVICE_HANDLE const hDevice) |
Run built-in digital calibration on the device. | |
ADMW_RESULT | admw_GetStatus (ADMW_DEVICE_HANDLE const hDevice, ADMW_STATUS *const pStatus) |
Read the current status from the device registers. | |
ADMW_RESULT | admw1001_AssembleLutData (ADMW1001_LUT *pLutBuffer, unsigned nLutBufferSize, unsigned const nNumTables, ADMW1001_LUT_DESCRIPTOR *const ppDesc[], ADMW1001_LUT_TABLE_DATA *const ppData[]) |
Assemble a list of separate Look-Up Tables into a single buffer. | |
ADMW_RESULT | admw1001_SetLutData (ADMW_DEVICE_HANDLE hDevice, ADMW1001_LUT *const pLutData) |
Write Look-Up Table data to the device memory. | |
ADMW_RESULT | admw1001_SetLutDataRaw (ADMW_DEVICE_HANDLE hDevice, ADMW1001_LUT_RAW *const pLutData) |
Write Look-Up Table raw data to the device memory. | |
ADMW_RESULT | admw_GetData (ADMW_DEVICE_HANDLE const hDevice, ADMW_MEASUREMENT_MODE const eMeasurementMode, ADMW_DATA_SAMPLE *const pSamples, uint8_t const nBytesPerSample, uint32_t const nRequested, uint32_t *const pnReturned) |
Read measurement data samples from the device registers. | |
ADMW_RESULT | admw_GetCommandRunningState (ADMW_DEVICE_HANDLE hDevice, bool *pbCommandRunning) |
Check if a command is currently running on the device. |
Detailed Description
: ADMW Host Library Application Programming Interface (API) -----------------------------------------------------------------------------
Definition in file admw_api.h.
Generated on Thu Jul 14 2022 10:33:00 by
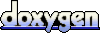