
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw_api.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: admw_api.h 00039 * @brief: ADMW Host Library Application Programming Interface (API) 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef _ADMW_API_H__ 00044 #define _ADMW_API_H__ 00045 00046 #include "inc/admw_types.h" 00047 #include "inc/admw_config_types.h" 00048 #include "inc/admw_platform.h" 00049 #include "inc/admw_gpio.h" 00050 #include "inc/admw_spi.h" 00051 #include "inc/admw_log.h" 00052 #include "inc/admw_time.h" 00053 #include "inc/admw1001/admw1001_sensor_types.h" 00054 #include "inc/admw1001/admw1001_lut_data.h" 00055 #include "ADMW1001_REGISTERS_typedefs.h" 00056 /*! @defgroup ADMW_Api ADMW Host Library API 00057 * Host library API common to the ADMW product family. 00058 * @{ 00059 */ 00060 00061 #ifdef __cplusplus 00062 extern "C" { 00063 #endif 00064 00065 /*! A handle used in all API functions to identify the ADMW device. */ 00066 typedef void* ADMW_DEVICE_HANDLE ; 00067 00068 #define SPI_BUFFER_SIZE 100u 00069 00070 #define SFL_READ_STATUS_HDR_SIZE 14u 00071 00072 #define SFL_READ_STATUS_RESPONSE_SIZE 42u 00073 00074 /*! Supported connection types for communication with the ADMW device. */ 00075 typedef enum 00076 { 00077 ADMW_CONNECTION_TYPE_SPI = 1, 00078 /*!< Serial Peripheral Interface (SPI) connection type */ 00079 00080 } ADMW_CONNECTION_TYPE ; 00081 00082 /*! Connection details for communication with a ADMW device instance. */ 00083 typedef struct 00084 { 00085 ADMW_CONNECTION_TYPE type; 00086 /*!< Connection type selection */ 00087 ADMW_PLATFORM_SPI_CONFIG spi; 00088 /*!< SPI connection parameters, required if SPI connection type is used */ 00089 ADMW_PLATFORM_GPIO_CONFIG gpio; 00090 /*!< GPIO connection parameters, for device reset and status I/O signals */ 00091 ADMW_PLATFORM_LOG_CONFIG log; 00092 /*!< Log interface connection parameters, for display/routing of log messages */ 00093 00094 } ADMW_CONNECTION ; 00095 00096 /*! Bit masks (flags) for the different device status indicators. */ 00097 typedef enum 00098 { 00099 ADMW_DEVICE_STATUS_BUSY = (1 << 0), 00100 /*!< Indicates that a command is currently running on the device */ 00101 ADMW_DEVICE_STATUS_DATAREADY = (1 << 1), 00102 /*!< Indicates the availability of measurement data for retrieval */ 00103 ADMW_DEVICE_STATUS_ERROR = (1 << 2), 00104 /*!< Indicates that an error condition has been detected by the device */ 00105 ADMW_DEVICE_STATUS_ALERT = (1 << 3), 00106 /*!< Indicates that an alert condition has been detected by the device */ 00107 ADMW_DEVICE_STATUS_FIFO_ERROR = (1 << 4), 00108 /*!< Indicates that a FIFO error condition has been detected by the device */ 00109 ADMW_DEVICE_STATUS_CONFIG_ERROR = (1 << 5), 00110 /*!< Indicates that a configuration error condition has been detected by the device */ 00111 ADMW_DEVICE_STATUS_LUT_ERROR = (1 << 6), 00112 /*!< Indicates that a look-up table error condition has been detected by the device */ 00113 00114 } ADMW_DEVICE_STATUS_FLAGS ; 00115 00116 00117 /*! Bit masks (flags) for the different channel alert indicators. */ 00118 typedef enum 00119 { 00120 ADMW_ALERT_DETAIL_CH_RESULT_VALID = (1 << 0), 00121 /*!< Indicates valid result in the channel */ 00122 ADMW_ALERT_DETAIL_CH_ADC_NEAR_OVERRANGE = (1 << 1), 00123 /*!< Indicates ADC is near overrange in the channel */ 00124 ADMW_ALERT_DETAIL_CH_SENSOR_UNDERRANGE = (1 << 2), 00125 /*!< Indicates sensor underrange in the channel */ 00126 ADMW_ALERT_DETAIL_CH_SENSOR_OVERRANGE = (1 << 3), 00127 /*!< Indicates sensor overrange in the channel */ 00128 ADMW_ALERT_DETAIL_CH_CJ_SOFT_FAULT = (1 << 4), 00129 /*!< Indicates Cold Junction soft fault in the channel */ 00130 ADMW_ALERT_DETAIL_CH_CJ_HARD_FAULT = (1 << 5), 00131 /*!< Indicates Cold Junction Hard Fault in the channel */ 00132 ADMW_ALERT_DETAIL_CH_ADC_INPUT_OVERRANGE = (1 << 6), 00133 /*!< Indicates ADC Input overrange in the channel */ 00134 ADMW_ALERT_DETAIL_CH_SENSOR_HARDFAULT = (1 << 7), 00135 /*!< Indicates Hard Fault in the channel */ 00136 } ADMW_CHANNEL_ALERT_FLAGS ; 00137 00138 /*! Status details retreived from the ADMW device. */ 00139 typedef struct 00140 { 00141 ADMW_DEVICE_STATUS_FLAGS deviceStatus; 00142 /*!< General summary status information from the device */ 00143 ADMW_CHANNEL_ALERT_FLAGS channelAlerts[ADMW1001_MAX_CHANNELS ]; 00144 /*!< Per-channel alert status information from the device */ 00145 uint32_t errorCode; 00146 /*!< Code identifying the last error signalled by the device */ 00147 uint32_t alertCode; 00148 /*!< Code identifying the last alert signalled by the device */ 00149 uint32_t debugCode; 00150 /*!< Supplementary code related to the last error/alert */ 00151 uint32_t channelAlertCodes[ADMW1001_MAX_CHANNELS ]; 00152 /*!< Per-channel code identifying the last alert signalled for each channel */ 00153 00154 } ADMW_STATUS ; 00155 00156 /*! Data sample details retreived from the ADMW device. */ 00157 typedef struct 00158 { 00159 float32_t processedValue; 00160 /*!< The processed value obtained from the measurement channel, as a final 00161 * measurement value, following calibration and linearisation correction, 00162 * and conversion into an appropriate unit of measurement. 00163 */ 00164 uint16_t Channel_ID : 4; /**< Indicates which channel this result corresponds to */ 00165 uint16_t Ch_Error : 1; /**< Indicates Error on channel */ 00166 uint16_t Ch_Alert : 1; /**< Indicates Alert on channel */ 00167 uint16_t Ch_Raw : 1; /**< Indicates if Raw sensor data field is valid */ 00168 uint16_t Ch_Valid : 1; /**< Indicates if this Result structure is valid */ 00169 uint16_t Reserved : 8; /**< Reserved for future use */ 00170 ADMW_CORE_Alert_Detail_Ch_t Status; /**< Indicates Status of this measurement */ 00171 float32_t Raw_Sample; 00172 /*!< The raw value obtained from the measurement channel, before 00173 * calibration and linearisation correction, and conversion. 00174 */ 00175 00176 } ADMW_DATA_SAMPLE ; 00177 00178 /*! Measurement mode options for the ADMW device. 00179 * @ref admw_StartMeasurement 00180 */ 00181 typedef enum 00182 { 00183 ADMW_MEASUREMENT_MODE_NORMAL = 0, 00184 /*!< In this mode, normal measurement cycle(s) are executed and data samples 00185 * are returned with raw measurement values included. */ 00186 ADMW_MEASUREMENT_MODE_OMIT_RAW , 00187 /*!< In this mode, normal measurement cycle(s) are executed and data samples 00188 * are returned with raw measurement values omitted for efficiency. */ 00189 00190 } ADMW_MEASUREMENT_MODE ; 00191 00192 /*! Identifiers for the user configuration slots in persistent memory. */ 00193 typedef enum 00194 { 00195 ADMW_FLASH_CONFIG_1, 00196 00197 } ADMW_USER_CONFIG_SLOT ; 00198 00199 /*! Identifiers for the user configuration slots in persistent memory. */ 00200 typedef enum 00201 { 00202 ADMW_FLASH_LUT_CHANNEL_0=0, 00203 ADMW_FLASH_LUT_CHANNEL_1, 00204 ADMW_FLASH_LUT_CHANNEL_2, 00205 ADMW_FLASH_LUT_CHANNEL_3, 00206 00207 00208 } ADMW_USER_LUT_CONFIG_SLOT ; 00209 00210 typedef struct 00211 { 00212 unsigned nDeviceIndex; 00213 ADMW_SPI_HANDLE hSpi; 00214 ADMW_GPIO_HANDLE hGpio; 00215 } ADMW_DEVICE_CONTEXT; 00216 00217 /****************************************************************************** 00218 * ADMW High-Level API function prototypes 00219 *****************************************************************************/ 00220 00221 /*! 00222 * @brief Open ADMW device handle and set up communication interface. 00223 * 00224 * @param[in] nDeviceIndex Zero-based index number identifying this device 00225 * instance. Note that this will be used to 00226 * retrieve a specific device configuration for 00227 * this device (see @ref admw_SetConfig 00228 * and @ref ADMW_CONFIG) 00229 * @param[in] pConnectionInfo Host-specific connection details (e.g. SPI, GPIO) 00230 * @param[out] phDevice Pointer to return an ADMW device handle 00231 * 00232 * @return Status 00233 * - #ADMW_SUCCESS Call completed successfully. 00234 * - #ADMW_NO_MEM Failed to allocate memory resources. 00235 * - #ADMW_INVALID_DEVICE_NUM Invalid device index specified 00236 * 00237 * @details Configure and initialise the Log interface and the SPI/GPIO 00238 * communication interface to the ADISense module. 00239 */ 00240 ADMW_RESULT admw_Open( 00241 unsigned const nDeviceIndex, 00242 ADMW_CONNECTION * const pConnectionInfo, 00243 ADMW_DEVICE_HANDLE * const phDevice); 00244 00245 /*! 00246 * @brief Close ADMW device context and free resources. 00247 * 00248 * @param[in] hDevice ADMW device context handle 00249 * 00250 * @return Status 00251 * - #ADMW_SUCCESS Call completed successfully. 00252 */ 00253 ADMW_RESULT admw_Close( 00254 ADMW_DEVICE_HANDLE const hDevice); 00255 00256 /*! 00257 * @brief Get the current state of the specified GPIO input signal. 00258 * 00259 * @param[in] hDevice ADMW device context handle 00260 * @param[in] ePinId GPIO pin to query 00261 * @param[out] pbAsserted Pointer to return the state of the status signal GPIO pin 00262 * 00263 * @return Status 00264 * - #ADMW_SUCCESS Call completed successfully. 00265 * - #ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified. 00266 * 00267 * @details Sets *pbAsserted to true if the status signal is asserted, or false 00268 * otherwise. 00269 */ 00270 ADMW_RESULT admw_GetGpioState( 00271 ADMW_DEVICE_HANDLE const hDevice, 00272 ADMW_GPIO_PIN const ePinId, 00273 bool * const pbAsserted); 00274 00275 /*! 00276 * @brief Register an application-defined callback function for GPIO interrupts 00277 * 00278 * @param[in] hDevice ADMW context handle (@ref admw_Open) 00279 * @param[in] ePinId GPIO pin on which to enable/disable interrupts 00280 * @param[in] callbackFunction Function to be called when an interrupt occurs. 00281 * Specify NULL here to disable interrupts. 00282 * @param[in] pCallbackParam Optional opaque parameter passed to the callback 00283 * 00284 * @return Status 00285 * - #ADMW_SUCCESS Call completed successfully. 00286 * - #ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified. 00287 */ 00288 ADMW_RESULT admw_RegisterGpioCallback( 00289 ADMW_DEVICE_HANDLE const hDevice, 00290 ADMW_GPIO_PIN const ePinId, 00291 ADMW_GPIO_CALLBACK const callbackFunction, 00292 void * const pCallbackParam); 00293 00294 /*! 00295 * @brief Trigger a shut down of the device. 00296 * 00297 * @param[in] hDevice ADMW device context handle 00298 * 00299 * @return Status 00300 * - #ADMW_SUCCESS Call completed successfully. 00301 * 00302 * @details Instructs the ADMW device to initiate a shut down, 00303 * typically used to conserve power when the device is not 00304 * in use. The device may be restarted by calling 00305 * @ref admw_Reset(). Note that active configuration 00306 * settings are not preserved during shutdown and must be 00307 * reloaded after the device has become ready again. 00308 * 00309 * @note No other command must be running when this is called. 00310 */ 00311 ADMW_RESULT admw_Shutdown( 00312 ADMW_DEVICE_HANDLE const hDevice); 00313 00314 /*! 00315 * @brief Reset the ADMW device. 00316 * 00317 * @param[in] hDevice ADMW device context handle 00318 * 00319 * @return Status 00320 * - #ADMW_SUCCESS Call completed successfully. 00321 * 00322 * @details Trigger a hardware-reset of the ADMW device. 00323 * 00324 * @note The device may require several seconds before it is ready for use 00325 * again. @ref admw_GetDeviceReadyState may be used to check if 00326 * the device is ready. 00327 */ 00328 ADMW_RESULT admw_Reset( 00329 ADMW_DEVICE_HANDLE const hDevice); 00330 00331 /*! 00332 * @brief Check if the device is ready, following power-up or a reset. 00333 * 00334 * @param[in] hDevice ADMW device context handle 00335 * @param[out] pbReady Pointer to return true if the device is ready, or false 00336 * otherwise 00337 * 00338 * @return Status 00339 * - #ADMW_SUCCESS Call completed successfully. 00340 * 00341 * @details This function attempts to read a fixed-value device register via 00342 * the communication interface. 00343 */ 00344 ADMW_RESULT admw_GetDeviceReadyState( 00345 ADMW_DEVICE_HANDLE const hDevice, 00346 bool * const pbReady); 00347 00348 /*! 00349 * @brief Obtain the product ID from the device. 00350 * 00351 * @param[in] hDevice ADMW device context handle 00352 * @param[out] pProductId Pointer to return the product ID value 00353 * 00354 * @return Status 00355 * - #ADMW_SUCCESS Call completed successfully. 00356 * 00357 * @details Reads the product ID registers on the device and returns the value. 00358 */ 00359 ADMW_RESULT admw_GetProductID( 00360 ADMW_DEVICE_HANDLE const hDevice, 00361 ADMW_PRODUCT_ID * const pProductId); 00362 00363 /*! 00364 * @brief Write full configuration settings to the device registers. 00365 * 00366 * @param[in] hDevice ADMW device context handle 00367 * @param[out] pConfig Pointer to the configuration data structure 00368 * 00369 * @return Status 00370 * - #ADMW_SUCCESS Call completed successfully. 00371 * 00372 * @details Translates configuration details provided into device-specific 00373 * register settings and updates device configuration registers. 00374 * 00375 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00376 */ 00377 ADMW_RESULT admw_SetConfig( 00378 ADMW_DEVICE_HANDLE const hDevice, 00379 ADMW_CONFIG * const pConfig); 00380 00381 /*! 00382 * @brief Apply the configuration settings currently stored in device registers 00383 * 00384 * @param[in] hDevice ADMW device context handle 00385 * 00386 * @return Status 00387 * - #ADMW_SUCCESS Call completed successfully. 00388 * 00389 * @details Instructs the ADMW device to reload and apply configuration 00390 * from the device configuration registers. Changes to configuration 00391 * registers are ignored by the device until this function is called. 00392 * 00393 * @note No other command must be running when this is called. 00394 */ 00395 ADMW_RESULT admw_ApplyConfigUpdates( 00396 ADMW_DEVICE_HANDLE const hDevice); 00397 00398 /*! 00399 * @brief Store the configuration settings to persistent memory on the device. 00400 * 00401 * @param[in] hDevice ADMW device context handle 00402 * @param[in] eSlotId User configuration slot in persistent memory 00403 * 00404 * @return Status 00405 * - #ADMW_SUCCESS Call completed successfully. 00406 * 00407 * @details Instructs the ADMW device to save the current contents of its 00408 * device configuration registers to non-volatile memory. 00409 * 00410 * @note No other command must be running when this is called. 00411 * @note Do not power down the device while this command is running. 00412 */ 00413 ADMW_RESULT admw_SaveConfig( 00414 ADMW_DEVICE_HANDLE const hDevice, 00415 ADMW_USER_CONFIG_SLOT const eSlotId); 00416 00417 /*! 00418 * @brief Restore configuration settings from persistent memory on the device. 00419 * 00420 * @param[in] hDevice ADMW device context handle 00421 * @param[in] eSlotId User configuration slot in persistent memory 00422 * 00423 * @return Status 00424 * - #ADMW_SUCCESS Call completed successfully. 00425 * 00426 * @details Instructs the ADMW device to restore the contents of its 00427 * device configuration registers from non-volatile memory. 00428 * 00429 * @note No other command must be running when this is called. 00430 */ 00431 ADMW_RESULT admw_RestoreConfig( 00432 ADMW_DEVICE_HANDLE const hDevice, 00433 ADMW_USER_CONFIG_SLOT const eSlotId); 00434 00435 /*! 00436 * @brief Store the LUT data to persistent memory on the device. 00437 * 00438 * @param[in] hDevice ADMW device context handle 00439 * 00440 * @return Status 00441 * - #ADMW_SUCCESS Call completed successfully. 00442 * 00443 * @details Instructs the ADMW device to save the current contents of its 00444 * LUT data buffer, set using @ref admw_SetLutData, to 00445 * non-volatile memory. 00446 * 00447 * @note No other command must be running when this is called. 00448 * @note Do not power down the device while this command is running. 00449 */ 00450 ADMW_RESULT admw_SaveLutData( 00451 ADMW_DEVICE_HANDLE const hDevice); 00452 00453 /*! 00454 * @brief Restore LUT data from persistent memory on the device. 00455 * 00456 * @param[in] hDevice ADMW device context handle 00457 * 00458 * @return Status 00459 * - #ADMW_SUCCESS Call completed successfully. 00460 * 00461 * @details Instructs the ADMW device to restore the contents of its 00462 * LUT data, previously stored with @ref admw_SaveLutData, from 00463 * non-volatile memory. 00464 * 00465 * @note No other command must be running when this is called. 00466 */ 00467 ADMW_RESULT admw_RestoreLutData( 00468 ADMW_DEVICE_HANDLE const hDevice); 00469 00470 /*! 00471 * @brief Start the measurement cycles on the device. 00472 * 00473 * @param[in] hDevice ADMW device context handle 00474 * @param[in] eMeasurementMode Allows a choice of special modes for the 00475 * measurement. See @ref ADMW_MEASUREMENT_MODE 00476 * for further information. 00477 * 00478 * @return Status 00479 * - #ADMW_SUCCESS Call completed successfully. 00480 * 00481 * @details Instructs the ADMW device to start executing measurement cycles 00482 * according to the current applied configuration settings. The 00483 * DATAREADY status signal will be asserted whenever new measurement 00484 * data is published, according to selected settings. 00485 * Measurement cycles may be stopped by calling @ref 00486 * admw_StopMeasurement. 00487 * 00488 * @note No other command must be running when this is called. 00489 */ 00490 ADMW_RESULT admw_StartMeasurement( 00491 ADMW_DEVICE_HANDLE const hDevice, 00492 ADMW_MEASUREMENT_MODE const eMeasurementMode); 00493 00494 /*! 00495 * @brief Stop the measurement cycles on the device. 00496 * 00497 * @param[in] hDevice ADMW device context handle 00498 * 00499 * @return Status 00500 * - #ADMW_SUCCESS Call completed successfully. 00501 * 00502 * @details Instructs the ADMW device to stop executing measurement cycles. 00503 * The command may be delayed until the current conversion, if any, has 00504 * been completed and published. 00505 * 00506 * @note To be used only if a measurement command is currently running. 00507 */ 00508 ADMW_RESULT admw_StopMeasurement( 00509 ADMW_DEVICE_HANDLE const hDevice); 00510 00511 /*! 00512 * @brief Run built-in diagnostic checks on the device. 00513 * 00514 * @param[in] hDevice ADMW device context handle 00515 * 00516 * @return Status 00517 * - #ADMW_SUCCESS Call completed successfully. 00518 * 00519 * @details Instructs the ADMW device to execute its built-in diagnostic 00520 * tests, on any enabled measurement channels, according to the current 00521 * applied configuration settings. Device status registers will be 00522 * updated to indicate if any errors were detected by the diagnostics. 00523 * 00524 * @note No other command must be running when this is called. 00525 */ 00526 ADMW_RESULT admw_RunDiagnostics( 00527 ADMW_DEVICE_HANDLE const hDevice); 00528 00529 /*! 00530 * @brief Run built-in calibration on the device. 00531 * 00532 * @param[in] hDevice ADMW device context handle 00533 * 00534 * @return Status 00535 * - #ADMW_SUCCESS Call completed successfully. 00536 * 00537 * @details Instructs the ADMW device to execute its self-calibration 00538 * routines, on any enabled measurement channels, according to the 00539 * current applied configuration settings. Device status registers 00540 * will be updated to indicate if any errors were detected. 00541 * 00542 * @note No other command must be running when this is called. 00543 */ 00544 ADMW_RESULT admw_RunCalibration( 00545 ADMW_DEVICE_HANDLE const hDevice); 00546 00547 /*! 00548 * @brief Run built-in digital calibration on the device. 00549 * 00550 * @param[in] hDevice ADMW device context handle 00551 * 00552 * @return Status 00553 * - #ADMW_SUCCESS Call completed successfully. 00554 * 00555 * @details Instructs the ADMW device to execute its calibration 00556 * routines, on any enabled digital channels, according to the 00557 * current applied configuration settings. Device status registers 00558 * will be updated to indicate if any errors were detected. 00559 * 00560 * @note No other command must be running when this is called. 00561 */ 00562 ADMW_RESULT admw_RunDigitalCalibration( 00563 ADMW_DEVICE_HANDLE const hDevice); 00564 00565 /*! 00566 * @brief Read the current status from the device registers. 00567 * 00568 * @param[in] hDevice ADMW device context handle 00569 * @param[out] pStatus Pointer to return the status summary obtained from the 00570 * device. 00571 * 00572 * @return Status 00573 * - #ADMW_SUCCESS Call completed successfully. 00574 * 00575 * @details Reads the status registers and extracts the relevant information 00576 * to return to the caller. 00577 * 00578 * @note This may be called at any time, assuming the device is ready. 00579 */ 00580 ADMW_RESULT admw_GetStatus( 00581 ADMW_DEVICE_HANDLE const hDevice, 00582 ADMW_STATUS * const pStatus); 00583 00584 /*! 00585 * @brief Assemble a list of separate Look-Up Tables into a single buffer 00586 * 00587 * @param[out] pLutBuffer Pointer to the Look-Up Table data buffer where 00588 * the assembled Look-Up Table data will be placed 00589 * @param[in] nLutBufferSize Allocated size, in bytes, of the output data buffer 00590 * @param[in] nNumTables Number of tables to add to the Look-Up Table buffer 00591 * @param[in] ppDesc Array of pointers to the table descriptors to be added 00592 * @param[in] ppData Array of pointers to the table data to be added 00593 * 00594 * @return Status 00595 * - #ADMW_SUCCESS Call completed successfully. 00596 * 00597 * @details This utiliity function fills the Look-up Table header fields; then 00598 * walks through the array of individual table descriptor and data 00599 * pointers provided, appending (copying) each one to the Look-Up Table 00600 * data buffer. The length and crc16 fields of each table descriptor 00601 * will be calculated and filled by this function, but other fields in 00602 * the descriptor structure must be filled by the caller beforehand. 00603 * 00604 * @note The assembled LUT data buffer filled by this function can then be 00605 * written to the device memory using @ref admw1001_SetLutData. 00606 */ 00607 ADMW_RESULT admw1001_AssembleLutData( 00608 ADMW1001_LUT *pLutBuffer, 00609 unsigned nLutBufferSize, 00610 unsigned const nNumTables, 00611 ADMW1001_LUT_DESCRIPTOR *const ppDesc[], 00612 ADMW1001_LUT_TABLE_DATA *const ppData[]); 00613 00614 /*! 00615 * @brief Write Look-Up Table data to the device memory 00616 * 00617 * @param[in] hDevice ADMW1001 device context handle 00618 * @param[out] pLutData Pointer to the Look-Up Table data structure 00619 * 00620 * @return Status 00621 * - #ADMW_SUCCESS Call completed successfully. 00622 * 00623 * @details Validates the Look-Up Table data format and loads it into 00624 * device memory via dedicated keyhole registers. 00625 * 00626 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00627 */ 00628 ADMW_RESULT admw1001_SetLutData( 00629 ADMW_DEVICE_HANDLE hDevice, 00630 ADMW1001_LUT *const pLutData); 00631 00632 /*! 00633 * @brief Write Look-Up Table raw data to the device memory 00634 * 00635 * @param[in] hDevice ADMW device context handle 00636 * @param[out] pLutData Pointer to the Look-Up Table raw data structure 00637 * 00638 * @return Status 00639 * - #ADMW_SUCCESS Call completed successfully. 00640 * 00641 * @details This can be used instead of @ref admw1001_SetLutData for 00642 * loading LUT data from the alternative raw data format. See 00643 * @ref admw1001_SetLutData for more information. 00644 * 00645 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00646 */ 00647 ADMW_RESULT admw1001_SetLutDataRaw( 00648 ADMW_DEVICE_HANDLE hDevice, 00649 ADMW1001_LUT_RAW *const pLutData); 00650 /*! 00651 * @brief Read measurement data samples from the device registers. 00652 * 00653 * @param[in] hDevice ADMW device context handle 00654 * @param[in] eMeasurementMode Must be set to the same value used for @ref 00655 * admw_StartMeasurement(). 00656 * @param[out] pSamples Pointer to return a set of requested data samples. 00657 * @param[in] nBytesPerSample The size, in bytes, of each sample. 00658 * @param[in] nRequested Number of requested data samples. 00659 * @param[out] pnReturned Number of valid data samples successfully retrieved. 00660 * 00661 * @return Status 00662 * - #ADMW_SUCCESS Call completed successfully. 00663 * 00664 * @details Reads the status registers and extracts the relevant information 00665 * to return to the caller. 00666 * 00667 * @note This is intended to be called only when the DATAREADY status signal 00668 * is asserted. 00669 */ 00670 ADMW_RESULT admw_GetData( 00671 ADMW_DEVICE_HANDLE const hDevice, 00672 ADMW_MEASUREMENT_MODE const eMeasurementMode, 00673 ADMW_DATA_SAMPLE * const pSamples, 00674 uint8_t const nBytesPerSample, 00675 uint32_t const nRequested, 00676 uint32_t * const pnReturned); 00677 00678 /*! 00679 * @brief Check if a command is currently running on the device. 00680 * 00681 * @param[in] hDevice ADMW device context handle 00682 * @param[out] pbCommandRunning Pointer to return the command running status 00683 * 00684 * @return Status 00685 * - #ADMW_SUCCESS Call completed successfully. 00686 * 00687 * @details Reads the device status register to check if a command is running. 00688 */ 00689 ADMW_RESULT admw_GetCommandRunningState( 00690 ADMW_DEVICE_HANDLE hDevice, 00691 bool *pbCommandRunning); 00692 00693 ADMW_RESULT admw1001_sendRun( ADMW_DEVICE_HANDLE const hDevice); 00694 ADMW_RESULT admw_deviceInformation(ADMW_DEVICE_HANDLE hDevice); 00695 00696 #ifdef __cplusplus 00697 } 00698 #endif 00699 00700 /*! 00701 * @} 00702 */ 00703 00704 #endif /* _ADMW_API_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
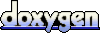