
Good
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "mbedWSEsbc.h" 00003 #define PI (3.14159) 00004 00005 // Declare objects (if necessary) 00006 Ticker Controller; // declare Ticker object named "Controller" 00007 DigitalOut myled(LED1); // LED, flash lights for debugging 00008 00009 00010 // variables for data handling and storage 00011 float TotalTime; // Total run time 00012 float Time; // Current elapsed time 00013 float Ts = 0.0083; // Control update period (seconds) (120 Hz equivalent) 00014 float Tstrm = 0.01; // Data streaming period (seconds) (100 Hz equivalent) 00015 float ang,angp,speed; // variables for approximating speed from encoder measurements 00016 float DCAmp; // duty cycle applied to motor 00017 float dc; // duty cycle 00018 long enc1; // encoder variable 00019 float lowDC; 00020 00021 00022 // Function definition Prototypes (declarations) 00023 void twoStepCode(); // declare that a separate (other than main) function named "ctrCode" exists 00024 00025 // Enter main function 00026 int main () 00027 { 00028 // Initializes mbed to access functionality of encoder, A/D, driver, etc. chipsets 00029 // Input is baud rate for PC communication 00030 mbedWSEsbcInit(115200); // also initializes timer object t 00031 mot_en1.period(0.020); // sets PWM period to 0.02 seconds for best DC motor operation 00032 00033 while(1) { 00034 // Scan serial port for user input to begin experiment 00035 pc.scanf("%f,%f",&TotalTime,&lowDC); //&DCAmp,&lowDC); 00036 // perform necessary functions to time the experiment 00037 Time = 0.0; // reset time variable 00038 t.reset(); // reset timer object 00039 // Attach the ctrCode function to ticker object specified with period Ts 00040 Controller.attach(&twoStepCode,Ts); 00041 t.start(); // start measuring elapsed time 00042 // perform operations while the elapsed time is less than the desired total time 00043 while(Time <= TotalTime) { 00044 // send data over serial port 00045 pc.printf("%f,%f,%f\n",Time,speed,dc); 00046 wait(Tstrm); // print data at approximately 50 Hz 00047 } // end while(Time<=Ttime) 00048 Controller.detach(); // detach ticker to turn off controller 00049 // Turn motor off at end of experiment 00050 mot_control(1,0.0); 00051 }// end while(1) 00052 }// end main 00053 // Additional function definitions 00054 void twoStepCode() // function to attach to ticker 00055 { 00056 myled = !myled; // toggle LED 2 to indicate control update 00057 // read current elapsed time 00058 Time = t.read(); 00059 // Read encoder 00060 enc1 = LS7366_read_counter(1); // input is the encoder channel 00061 // Convert from counts to radians 00062 ang = 2.0*PI*enc1/6400.0; 00063 // Estimate speed 00064 speed = (ang-angp)/Ts; 00065 // Age variables 00066 angp = ang; 00067 // compute duty cycle for motor (will be changed later!) 00068 //dc = DCAmp*sin(2.0*PI/5.0*Time); // user-specified duty cycle 00069 // Lab3 DC 00070 if(Time<0.1) { 00071 dc = 0.0; 00072 } else if(Time<0.55) { 00073 dc = lowDC; 00074 } else { 00075 dc = 0.10; 00076 } 00077 // motor control 00078 mot_control(1,dc); // first input is the motor channel, second is duty cycle 00079 } // end ctrCode()
Generated on Wed Jul 13 2022 23:19:02 by
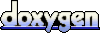