
123123123123123123123123123
Embed:
(wiki syntax)
Show/hide line numbers
port.h
00001 /****************************************************** 00002 00003 ****┏┓ ┏┓ 00004 **┏┛┻━━━━━━┛┻┓ 00005 **┃ ┃ 00006 **┃ ━━━ ┃ 00007 **┃ ┳┛ ┗┳ ┃ 00008 **┃ ┃ 00009 **┃ ''' ┻ ''' ┃ 00010 **┃ ┃ 00011 **┗━━┓ ┏━━┛ 00012 *******┃ ┃ 00013 *******┃ ┃ 00014 *******┃ ┃ 00015 *******┃ ┗━━━━━━━━┓ 00016 *******┃ ┃━┓ 00017 *******┃ NO BUG ┏━┛ 00018 *******┃ ┃ 00019 *******┗━┓ ┓ ┏━┏━┓ ━┛ 00020 ***********┃ ┛ ┛ ┃ ┛ ┛ 00021 ***********┃ ┃ ┃ ┃ ┃ ┃ 00022 ***********┗━┛━┛ ┗━┛━┛ 00023 00024 This part is added by project ESDC2014 of CUHK team. 00025 All the code with this header are under GPL open source license. 00026 This program is running on Mbed Platform 'mbed LPC1768' avaliable in 'http://mbed.org'. 00027 **********************************************************/ 00028 #include "mbed.h" 00029 #include "define.h" 00030 00031 #ifndef _PORT_H 00032 #define _PORT_H 00033 00034 MyDigitalOut IntelToMbed_LED(LED1); //uart port LED between Intel Board and Mbed 00035 MyDigitalOut MbedToArduino_LED(LED2); //uart port LED between Mbed and Arduino 00036 MySerial DEBUG(USBTX, USBRX); //usb serial port between computer and Mbed 00037 MySerial CompassData(p9, p10); ////uart port between compass and Mbed 00038 MySerial IntelToMbed(p13, p14); //uart port between Intel Board and Mbed 00039 MySerial MbedToArduino(p28, p27); //uart port between Mbed and Arduino 00040 MyPwmOut lifter_pwmUp(p21); 00041 MyPwmOut lifter_pwmDown(p22); 00042 MyDigitalOut lifter_enable(p23); 00043 MyInterruptIn lifter_encoder_A(p18); 00044 MyDigitalIn lifter_encoder_B(p17); 00045 00046 MyPwmOut camera_platform_pwmRoll(p24);//roll min counter clockwise, max clockwise 00047 MyPwmOut camera_platform_pwmPitch(p26);//pitch min down, max up, mid 1700 00048 MyPwmOut camera_platform_pwmYaw(p25);//yaw min right, max left, mid 1500 00049 00050 MyDigitalOut buzzer_pin(p29); 00051 Buzzer buzzer(&buzzer_pin); 00052 COMPASS compass(&CompassData); 00053 00054 Communication com(&DEBUG, &IntelToMbed, &MbedToArduino, &compass); 00055 Lifter lifter(&lifter_enable, &lifter_pwmUp, &lifter_pwmDown, &lifter_encoder_A, &lifter_encoder_B); 00056 Camera_platform camera_platform(&camera_platform_pwmRoll, &camera_platform_pwmPitch, &camera_platform_pwmYaw); 00057 00058 void IntelToMbedRxHandler() 00059 { 00060 //__disable_irq();//disable interupt when receiving data from XBEE_UART 00061 uint8_t _x = IntelToMbed.getc(); 00062 com.putToBuffer(_x, 0); //function inside Communication:: 00063 //__enable_irq(); 00064 } 00065 void MbedToArduinoRxHandler() 00066 { 00067 //__disable_irq();//disable interupt when receiving data from XBEE_UART 00068 uint8_t _x = MbedToArduino.getc(); 00069 com.putToBuffer(_x, 1); //function inside Communication:: 00070 //__enable_irq(); 00071 } 00072 void compassHandler() 00073 { 00074 //__disable_irq();//disable interupt when receiving data from XBEE_UART 00075 uint8_t _x = CompassData.getc(); 00076 compass.putToBuffer(_x); //function inside Communication:: 00077 //__enable_irq(); 00078 } 00079 00080 void LifterPulseHandler() 00081 { 00082 if(lifter.pulseCount < lifter.targetPulseCount) 00083 { 00084 lifter.pulseCount++; 00085 if(lifter.getDir() == 0) //up 00086 { 00087 lifter.setLifterUp(); 00088 } 00089 else if(lifter.getDir() == 2)//down 00090 { 00091 lifter.setLifterDown(); 00092 } 00093 } 00094 else 00095 { 00096 lifter.targetPulseCount = 0; 00097 lifter.pulseCount = 0; 00098 lifter.setLifterStop(); 00099 } 00100 } 00101 00102 void init_PORT() //used in main() function 00103 { 00104 DEBUG.baud(9600); 00105 00106 IntelToMbed.baud(9600); 00107 IntelToMbed.attach(&IntelToMbedRxHandler); //serial interrupt function 00108 00109 MbedToArduino.baud(9600); 00110 MbedToArduino.attach(&MbedToArduinoRxHandler); //serial interrupt function 00111 00112 CompassData.baud(56000); 00113 CompassData.attach(&compassHandler); 00114 00115 lifter_encoder_A.fall(&LifterPulseHandler); //interrupt 00116 00117 camera_platform_pwmRoll.period_ms(20); //20ms periodic, 1000us to 2000us 00118 camera_platform_pwmPitch.period_ms(20); //20ms periodic, 1000us to 2000us 00119 camera_platform_pwmYaw.period_ms(20); //20ms periodic, 1000us to 2000us 00120 00121 camera_platform_pwmRoll.pulsewidth_us(ROLL_MID); 00122 camera_platform_pwmPitch.pulsewidth_us(PITCH_MID); 00123 camera_platform_pwmYaw.pulsewidth_us(YAW_MID); 00124 } 00125 00126 #endif
Generated on Thu Jul 14 2022 22:42:47 by
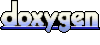